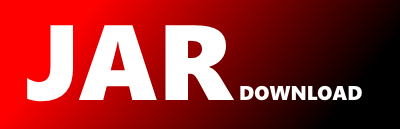
org.cattleframework.utils.auxiliary.HttpUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cattle-utils Show documentation
Show all versions of cattle-utils Show documentation
Cattle framework utils component pom
/*
* Copyright (C) 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.cattleframework.utils.auxiliary;
import java.util.Enumeration;
import java.util.Iterator;
import org.apache.commons.lang3.StringUtils;
import org.cattleframework.exception.CattleException;
import org.springframework.http.HttpHeaders;
import org.springframework.http.server.ServerHttpRequest;
import jakarta.servlet.http.Cookie;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
/**
* Http工具
*
* @author orange
*
*/
public final class HttpUtils {
private static final String HTTP_PREFIX = "http://";
private static final String HTTPS_PREFIX = "https://";
private static final String UNKNOWN = "unknown";
private static final String LOCAL_HOST_IP_V6 = "0:0:0:0:0:0:0:1";
private static final String COMMA = ",";
private HttpUtils() {
}
public static boolean isSsl(String url) {
String lUrl = url.toLowerCase();
if (lUrl.startsWith(HTTPS_PREFIX) || lUrl.startsWith(HTTP_PREFIX)) {
return lUrl.startsWith("https://");
}
throw new CattleException("无效的URL地址:" + url);
}
public static String getCookieValue(HttpServletRequest request, String name) {
String str = null;
if (request.getCookies() != null) {
for (int i = 0; i < request.getCookies().length; i++) {
if (!request.getCookies()[i].getName().equalsIgnoreCase(name)) {
continue;
}
str = request.getCookies()[i].getValue();
break;
}
}
return str;
}
public static String getHeaderValue(HttpServletRequest request, String name) {
String result = null;
Enumeration headerNames = request.getHeaderNames();
while (headerNames.hasMoreElements()) {
String headerName = headerNames.nextElement();
if (headerName.equalsIgnoreCase(name)) {
result = request.getHeader(headerName);
break;
}
}
return result;
}
public static String getIpAddress(HttpServletRequest request) {
String ip = getHeaderValue(request, "x-forwarded-for");
if (ip != null && ip.length() != 0 && !UNKNOWN.equalsIgnoreCase(ip)) {
if (ip.indexOf(COMMA) != -1) {
ip = ip.split(COMMA)[0];
}
}
if (ip == null || ip.length() == 0 || UNKNOWN.equalsIgnoreCase(ip)) {
ip = getHeaderValue(request, "Proxy-Client-IP");
}
if (ip == null || ip.length() == 0 || UNKNOWN.equalsIgnoreCase(ip)) {
ip = getHeaderValue(request, "WL-Proxy-Client-IP");
}
if (ip == null || ip.length() == 0 || UNKNOWN.equalsIgnoreCase(ip)) {
ip = getHeaderValue(request, "HTTP_CLIENT_IP");
}
if (ip == null || ip.length() == 0 || UNKNOWN.equalsIgnoreCase(ip)) {
ip = getHeaderValue(request, "HTTP_X_FORWARDED_FOR");
}
if (ip == null || ip.length() == 0 || UNKNOWN.equalsIgnoreCase(ip)) {
ip = getHeaderValue(request, "X-Real-IP");
}
if (ip == null || ip.length() == 0 || UNKNOWN.equalsIgnoreCase(ip)) {
ip = request.getRemoteAddr();
}
if (LOCAL_HOST_IP_V6.equals(ip)) {
ip = "localhost";
}
return ip;
}
public static String getHeaderValue(ServerHttpRequest request, String name) {
String result = null;
HttpHeaders headers = request.getHeaders();
Iterator iterator = headers.keySet().iterator();
while (iterator.hasNext()) {
String headerName = iterator.next();
if (headerName.equalsIgnoreCase(name)) {
result = headers.getFirst(headerName);
break;
}
}
return result;
}
public static String getIpAddress(ServerHttpRequest request) {
String ip = getHeaderValue(request, "x-forwarded-for");
if (ip != null && ip.length() != 0 && !UNKNOWN.equalsIgnoreCase(ip)) {
if (ip.indexOf(COMMA) != -1) {
ip = ip.split(COMMA)[0];
}
}
if (ip == null || ip.length() == 0 || UNKNOWN.equalsIgnoreCase(ip)) {
ip = getHeaderValue(request, "Proxy-Client-IP");
}
if (ip == null || ip.length() == 0 || UNKNOWN.equalsIgnoreCase(ip)) {
ip = getHeaderValue(request, "WL-Proxy-Client-IP");
}
if (ip == null || ip.length() == 0 || UNKNOWN.equalsIgnoreCase(ip)) {
ip = getHeaderValue(request, "HTTP_CLIENT_IP");
}
if (ip == null || ip.length() == 0 || UNKNOWN.equalsIgnoreCase(ip)) {
ip = getHeaderValue(request, "HTTP_X_FORWARDED_FOR");
}
if (ip == null || ip.length() == 0 || UNKNOWN.equalsIgnoreCase(ip)) {
ip = getHeaderValue(request, "X-Real-IP");
}
if (ip == null || ip.length() == 0 || UNKNOWN.equalsIgnoreCase(ip)) {
ip = request.getRemoteAddress().getAddress().getHostAddress();
}
if (LOCAL_HOST_IP_V6.equals(ip)) {
ip = "localhost";
}
return ip;
}
public static void saveCookie(HttpServletRequest request, HttpServletResponse response, boolean httpOnly,
String name, String value) {
saveCookie(request, response, httpOnly, -1, name, value);
}
public static void saveCookie(HttpServletRequest request, HttpServletResponse response, boolean httpOnly,
int expiry, String name, String value) {
if (expiry != -1 && expiry <= 0) {
throw new CattleException("Cookie有效期间秒数必须大于0");
}
Cookie savedRequestCookie = new Cookie(name, value);
savedRequestCookie.setSecure(request.isSecure());
savedRequestCookie.setHttpOnly(httpOnly);
savedRequestCookie.setPath(getCookiePath(request));
savedRequestCookie.setMaxAge(expiry);
response.addCookie(savedRequestCookie);
}
public static void removeCookie(HttpServletRequest request, HttpServletResponse response, boolean httpOnly,
String name) {
Cookie removeSavedRequestCookie = new Cookie(name, "");
removeSavedRequestCookie.setSecure(request.isSecure());
removeSavedRequestCookie.setHttpOnly(httpOnly);
removeSavedRequestCookie.setPath(getCookiePath(request));
removeSavedRequestCookie.setMaxAge(0);
response.addCookie(removeSavedRequestCookie);
}
private static String getCookiePath(HttpServletRequest request) {
String contextPath = request.getContextPath();
return StringUtils.isNotBlank(contextPath) ? contextPath : "/";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy