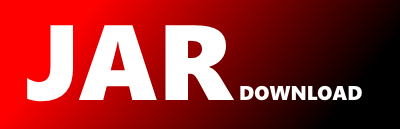
org.cattleframework.utils.auxiliary.JacksonUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cattle-utils Show documentation
Show all versions of cattle-utils Show documentation
Cattle framework utils component pom
/*
* Copyright (C) 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.cattleframework.utils.auxiliary;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.lang.reflect.Type;
import org.cattleframework.exception.ExceptionWrapUtils;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.ObjectWriter;
/**
* Jackson工具
*
* @author orange
*
*/
public final class JacksonUtils {
private static final ObjectMapper MAPPER = new ObjectMapper();
private JacksonUtils() {
}
static {
MAPPER.disable(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES);
MAPPER.setSerializationInclusion(Include.NON_NULL);
}
public static String toJson(T object) {
return toJson(object, false);
}
public static String toJson(T object, boolean pretty) {
try {
if (pretty) {
ObjectWriter objectWriter = MAPPER.writerWithDefaultPrettyPrinter();
return objectWriter.writeValueAsString(object);
} else {
return MAPPER.writeValueAsString(object);
}
} catch (JsonProcessingException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static byte[] toJsonBytes(T object) {
return toJsonBytes(object, false);
}
public static byte[] toJsonBytes(T object, boolean pretty) {
try {
if (pretty) {
ObjectWriter objectWriter = MAPPER.writerWithDefaultPrettyPrinter();
return objectWriter.writeValueAsBytes(object);
} else {
return MAPPER.writeValueAsBytes(object);
}
} catch (JsonProcessingException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static void toJsonOutputStream(T object, OutputStream outputStream) {
toJsonOutputStream(object, outputStream, false);
}
public static void toJsonOutputStream(T object, OutputStream outputStream, boolean pretty) {
try {
if (pretty) {
ObjectWriter objectWriter = MAPPER.writerWithDefaultPrettyPrinter();
objectWriter.writeValue(outputStream, object);
} else {
MAPPER.writeValue(outputStream, object);
}
} catch (IOException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static T toObject(String json, Class type) {
try {
return MAPPER.readValue(json, type);
} catch (JsonProcessingException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static T toObject(String json, Type type) {
try {
return MAPPER.readValue(json, MAPPER.constructType(type));
} catch (JsonProcessingException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static T toObject(String json, TypeReference typeReference) {
try {
return MAPPER.readValue(json, typeReference);
} catch (JsonProcessingException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static T toObject(byte[] jsonBytes, Class type) {
try {
return MAPPER.readValue(jsonBytes, type);
} catch (IOException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static T toObject(byte[] jsonBytes, Type type) {
try {
return MAPPER.readValue(jsonBytes, MAPPER.constructType(type));
} catch (IOException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static T toObject(byte[] jsonBytes, TypeReference typeReference) {
try {
return MAPPER.readValue(jsonBytes, typeReference);
} catch (IOException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static T toObject(InputStream inputStream, Class type) {
try {
return MAPPER.readValue(inputStream, type);
} catch (IOException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static T toObject(InputStream inputStream, Type type) {
try {
return MAPPER.readValue(inputStream, MAPPER.constructType(type));
} catch (IOException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
public static T toObject(InputStream inputStream, TypeReference typeReference) {
try {
return MAPPER.readValue(inputStream, typeReference);
} catch (IOException e) {
throw ExceptionWrapUtils.wrap(e);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy