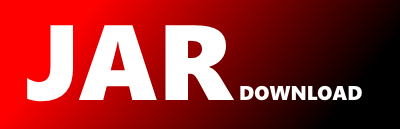
org.cattleframework.utils.auxiliary.NetUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cattle-utils Show documentation
Show all versions of cattle-utils Show documentation
Cattle framework utils component pom
/*
* Copyright (C) 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.cattleframework.utils.auxiliary;
import java.io.IOException;
import java.net.Inet4Address;
import java.net.Inet6Address;
import java.net.InetAddress;
import java.net.NetworkInterface;
import java.net.SocketException;
import java.net.UnknownHostException;
import java.util.Enumeration;
import java.util.HashSet;
import java.util.Set;
/**
* 网络工具
*
* @author orange
*
*/
public final class NetUtils {
private static final String DEFAULT_INET4_ADDRESS = "127.0.0.1";
private NetUtils() {
}
public static Set getInet4Addresses() {
Set inetAddresses = new HashSet();
try {
for (Enumeration nics = NetworkInterface.getNetworkInterfaces(); nics
.hasMoreElements();) {
NetworkInterface ifc = nics.nextElement();
if (ifc.isUp()) {
for (Enumeration addrs = ifc.getInetAddresses(); addrs.hasMoreElements();) {
InetAddress address = addrs.nextElement();
if (address instanceof Inet4Address && !address.isLoopbackAddress()) {
inetAddresses.add(address);
}
}
}
}
} catch (SocketException e) {
}
return inetAddresses;
}
public static Set getInet6Addresses() {
Set inetAddresses = new HashSet();
try {
for (Enumeration nics = NetworkInterface.getNetworkInterfaces(); nics
.hasMoreElements();) {
NetworkInterface ifc = nics.nextElement();
if (ifc.isUp() || !ifc.isVirtual() || !ifc.isLoopback()) {
for (Enumeration addrs = ifc.getInetAddresses(); addrs.hasMoreElements();) {
InetAddress address = addrs.nextElement();
if (address instanceof Inet6Address
// filter ::1
&& !address.isLoopbackAddress()
// filter fe80::/10
&& !address.isLinkLocalAddress()
// filter ::/128
&& !address.isAnyLocalAddress()
// filter fec0::/10,which was discarded, but some
// address may be deployed.
&& !address.isSiteLocalAddress()
// filter fd00::/8
&& !isUniqueLocalAddress(address)) {
inetAddresses.add(address);
}
}
}
}
} catch (IOException e) {
}
return inetAddresses;
}
public static InetAddress findFirstInet4Address() {
InetAddress result = null;
try {
int lowest = Integer.MAX_VALUE;
for (Enumeration nics = NetworkInterface.getNetworkInterfaces(); nics
.hasMoreElements();) {
NetworkInterface ifc = nics.nextElement();
if (ifc.isUp()) {
if (ifc.getIndex() < lowest || result == null) {
lowest = ifc.getIndex();
} else {
continue;
}
for (Enumeration addrs = ifc.getInetAddresses(); addrs.hasMoreElements();) {
InetAddress address = addrs.nextElement();
if (address instanceof Inet4Address && !address.isLoopbackAddress()) {
result = address;
}
}
}
}
} catch (IOException e) {
}
if (result != null) {
return result;
}
try {
return InetAddress.getLocalHost();
} catch (UnknownHostException e) {
}
return null;
}
public static InetAddress findFirstInet6Address() {
InetAddress result = null;
try {
for (Enumeration nics = NetworkInterface.getNetworkInterfaces(); nics
.hasMoreElements();) {
NetworkInterface ifc = nics.nextElement();
if (ifc.isUp() || !ifc.isVirtual() || !ifc.isLoopback()) {
for (Enumeration addrs = ifc.getInetAddresses(); addrs.hasMoreElements();) {
InetAddress inetAddress = addrs.nextElement();
if (inetAddress instanceof Inet6Address
// filter ::1
&& !inetAddress.isLoopbackAddress()
// filter fe80::/10
&& !inetAddress.isLinkLocalAddress()
// filter ::/128
&& !inetAddress.isAnyLocalAddress()
// filter fec0::/10,which was discarded, but some
// address may be deployed.
&& !inetAddress.isSiteLocalAddress()
// filter fd00::/8
&& !isUniqueLocalAddress(inetAddress)) {
result = inetAddress;
break;
}
}
}
}
} catch (IOException e) {
}
if (result != null) {
return result;
}
try {
return InetAddress.getLocalHost();
} catch (UnknownHostException e) {
}
return null;
}
private static boolean isUniqueLocalAddress(InetAddress inetAddress) {
byte[] ip = inetAddress.getAddress();
return (ip[0] & 0xff) == 0xfd;
}
public static InetAddress getLocalHost() {
InetAddress localHost = null;
try {
localHost = InetAddress.getLocalHost();
} catch (UnknownHostException e) {
}
return localHost;
}
public static String getHostAddress(InetAddress inetAddress) {
if (inetAddress == null) {
return DEFAULT_INET4_ADDRESS;
}
String hostAddress = inetAddress.getHostAddress();
if (inetAddress instanceof Inet6Address) {
int index = hostAddress.indexOf("%");
if (index != -1) {
hostAddress = hostAddress.substring(0, index);
}
}
return hostAddress;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy