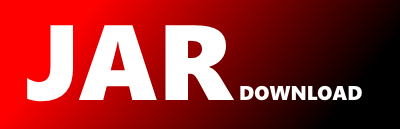
org.cattleframework.utils.auxiliary.StringUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cattle-utils Show documentation
Show all versions of cattle-utils Show documentation
Cattle framework utils component pom
/*
* Copyright (C) 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.cattleframework.utils.auxiliary;
import java.math.BigInteger;
import org.apache.commons.codec.digest.DigestUtils;
import org.apache.commons.text.RandomStringGenerator;
import com.google.common.base.CaseFormat;
/**
* 文本工具
*
* @author orange
*
*/
public final class StringUtils {
private static final char WILDCARD_MARK = '*';
private StringUtils() {
}
public static String replaceOnce(String template, String placeholder, String replacement) {
if (template == null) {
return null;
}
int loc = template.indexOf(placeholder);
if (loc < 0) {
return template;
}
return template.substring(0, loc) + replacement + template.substring(loc + placeholder.length());
}
public static boolean equals(final CharSequence cs1, final CharSequence cs2) {
if (org.apache.commons.lang3.StringUtils.isEmpty(cs1) && org.apache.commons.lang3.StringUtils.isEmpty(cs2)) {
return true;
}
return org.apache.commons.lang3.StringUtils.equals(cs1, cs2);
}
public static String replace(String template, String placeholder, String replacement) {
return replace(template, placeholder, replacement, false);
}
public static String replace(String template, String placeholder, String replacement, boolean wholeWords) {
int loc = template.indexOf(placeholder);
if (loc < 0) {
return template;
}
boolean actuallyReplace = !wholeWords || ((loc + placeholder.length()) == template.length())
|| !Character.isJavaIdentifierPart(template.charAt(loc + placeholder.length()));
String actualReplacement = actuallyReplace ? replacement : placeholder;
return new StringBuffer(template.substring(0, loc)).append(actualReplacement)
.append(replace(template.substring(loc + placeholder.length()), placeholder, replacement, wholeWords))
.toString();
}
public static String getRandomString(int length) {
return getRandomString(false, length);
}
public static String getRandomString(boolean onlyNumber, int length) {
char[][] pairs = { { 'a', 'z' }, { 'A', 'Z' }, { '0', '9' } };
RandomStringGenerator generator = onlyNumber ? new RandomStringGenerator.Builder().withinRange('0', '9').get()
: new RandomStringGenerator.Builder().withinRange(pairs).get();
return generator.generate(length);
}
/**
* 字符串是否匹配(通配符匹配,?代表任意一位 *代表0-N位)
*
* @param s 被匹配字符串
* @param p 匹配字符串
* @return 是否匹配
*/
public static boolean isMatch(String s, String p) {
int idxs = 0;
int idxp = 0;
int idxstar = -1;
int idxmatch = 0;
while (idxs < s.length()) {
if (idxp < p.length()) {
if (s.charAt(idxs) == p.charAt(idxp) || p.charAt(idxp) == '?') {
idxp++;
idxs++;
continue;
} else if (p.charAt(idxp) == WILDCARD_MARK) {
idxstar = idxp;
idxp++;
idxmatch = idxs;
continue;
}
}
if (idxstar != -1) {
// 用上一个*来匹配,那我们p的指针也应该退回至上一个*的后面
idxp = idxstar + 1;
// 用*匹配到的位置递增
idxmatch++;
// s的指针退回至用*匹配到位置
idxs = idxmatch;
} else {
return false;
}
}
// 因为1个*能匹配无限序列,如果p末尾有多个*,我们都要跳过
while (idxp < p.length() && p.charAt(idxp) == WILDCARD_MARK) {
idxp++;
}
// 如果p匹配完了,说明匹配成功
return idxp == p.length();
}
public static String getHashName(String str) {
byte[] bytes = DigestUtils.md5(str);
BigInteger bigInteger = new BigInteger(1, bytes);
return bigInteger.toString(35).toUpperCase();
}
public static String hyphen2Camel(String str) {
return str.indexOf("-") >= 0 ? CaseFormat.LOWER_HYPHEN.to(CaseFormat.LOWER_CAMEL, str) : str;
}
public static String camel2Hyphen(String str) {
return str.indexOf("-") >= 0 ? str : CaseFormat.LOWER_CAMEL.to(CaseFormat.LOWER_HYPHEN, str);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy