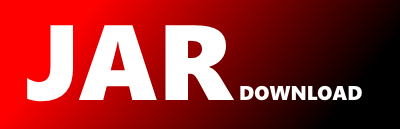
org.cattleframework.utils.http.RestTemplateUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cattle-utils Show documentation
Show all versions of cattle-utils Show documentation
Cattle framework utils component pom
/*
* Copyright (C) 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.cattleframework.utils.http;
import java.util.Iterator;
import java.util.Map;
import org.apache.commons.collections4.MapUtils;
import org.cattleframework.exception.CattleException;
import org.cattleframework.exception.CattleResponseException;
import org.cattleframework.exception.ExceptionConstants;
import org.cattleframework.exception.ExceptionWrapUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.core.ParameterizedTypeReference;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestClientResponseException;
import org.springframework.web.client.RestTemplate;
/**
* Rest模板工具
*
* @author orange
*
*/
public class RestTemplateUtils {
private static final Logger logger = LoggerFactory.getLogger(RestTemplateUtils.class);
private static final String MESSAGE = "message";
private static final String EXCEPTION_CODE = "error-code";
private static final String EXCEPTION_RESPONSE = "response";
private static final String MESSAGE_SOURCE = "source";
private static final String SOURCE_OWNER = "cattle";
private final RestTemplate restTemplate;
public RestTemplateUtils(RestTemplate restTemplate) {
this.restTemplate = restTemplate;
}
public T post(String url, MediaType type, Map headers, R request,
ParameterizedTypeReference responseType) {
HttpHeaders httpHeaders = getHeaders(type, headers);
HttpEntity requestEntity = new HttpEntity(request, httpHeaders);
try {
ResponseEntity responseEntity = restTemplate.exchange(url, HttpMethod.POST, requestEntity, responseType);
return responseEntity.getBody();
} catch (Throwable e) {
throw throwException(e);
}
}
public T post(String url, MediaType type, Map headers, R request, Class responseType) {
HttpHeaders httpHeaders = getHeaders(type, headers);
HttpEntity requestEntity = new HttpEntity(request, httpHeaders);
try {
ResponseEntity responseEntity = restTemplate.exchange(url, HttpMethod.POST, requestEntity, responseType);
return responseEntity.getBody();
} catch (Throwable e) {
throw throwException(e);
}
}
private HttpHeaders getHeaders(MediaType type, Map headers) {
HttpHeaders httpHeaders = new HttpHeaders();
if (type != null) {
httpHeaders.setContentType(type);
}
if (MapUtils.isNotEmpty(headers)) {
Iterator iterator = headers.keySet().iterator();
while (iterator.hasNext()) {
String header = iterator.next();
httpHeaders.add(header, headers.get(header));
}
}
return httpHeaders;
}
public T get(String url, MediaType type, Map headers,
ParameterizedTypeReference responseType) {
return get(url, type, headers, responseType, null);
}
public T get(String url, MediaType type, Map headers,
ParameterizedTypeReference responseType, Map uriVariables) {
HttpHeaders httpHeaders = getHeaders(type, headers);
HttpEntity
© 2015 - 2024 Weber Informatics LLC | Privacy Policy