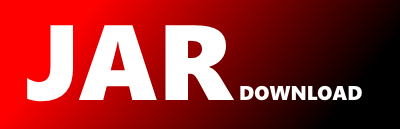
org.cattleframework.webmvc.WebMvcAutoConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cattle-webmvc Show documentation
Show all versions of cattle-webmvc Show documentation
Cattle framework webmvc component pom
/*
* Copyright (C) 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.cattleframework.webmvc;
import java.util.List;
import java.util.Set;
import java.util.Vector;
import org.apache.commons.collections4.CollectionUtils;
import org.cattleframework.exception.web.ExceptionProcessCustomizer;
import org.cattleframework.exception.web.ExceptionProcessResponse;
import org.cattleframework.web.WebProperties;
import org.cattleframework.webmvc.exception.ExceptionAdvice;
import org.cattleframework.webmvc.exception.ExceptionController;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.boot.autoconfigure.AutoConfiguration;
import org.springframework.boot.autoconfigure.condition.ConditionalOnMissingBean;
import org.springframework.boot.autoconfigure.web.servlet.error.ErrorMvcAutoConfiguration;
import org.springframework.boot.web.servlet.error.ErrorAttributes;
import org.springframework.context.annotation.Bean;
import org.springframework.lang.Nullable;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityCustomizer;
import org.springframework.security.web.firewall.HttpFirewall;
import org.springframework.security.web.firewall.HttpStatusRequestRejectedHandler;
import org.springframework.security.web.firewall.RequestRejectedHandler;
import org.springframework.security.web.firewall.StrictHttpFirewall;
import org.springframework.security.web.util.matcher.AntPathRequestMatcher;
import org.springframework.security.web.util.matcher.RequestMatcher;
import org.springframework.web.ErrorResponse;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
/**
* Web Mvc自动配置
*
* @author orange
*
*/
@AutoConfiguration(before = ErrorMvcAutoConfiguration.class)
@EnableWebMvc
@EnableWebSecurity
public class WebMvcAutoConfiguration {
@Value("${server.error.path:${error.path:/error}}")
private String errorPath;
@Bean
public WebMvcConfigurer webMvcConfigurer(WebProperties webProperties) {
return new CattleWebMvcConfigurer(webProperties);
}
@Bean("errorWebSecurity")
public WebSecurityCustomizer webSecurityCustomizer(WebProperties webProperties) {
Set securityIgnorePaths = webProperties.getSecurityIgnorePaths();
List requestMatchers = new Vector();
requestMatchers.add(AntPathRequestMatcher.antMatcher(errorPath));
requestMatchers.add(AntPathRequestMatcher.antMatcher("/favicon.ico"));
if (CollectionUtils.isNotEmpty(securityIgnorePaths)) {
for (String securityIgnorePath : securityIgnorePaths) {
requestMatchers.add(AntPathRequestMatcher.antMatcher(securityIgnorePath));
}
}
return (web) -> web.ignoring().requestMatchers(requestMatchers.toArray(new RequestMatcher[0]));
}
@Bean
@ConditionalOnMissingBean
public HttpFirewall httpFirewall(WebProperties webProperties) {
StrictHttpFirewall firewall = new StrictHttpFirewall();
if (CollectionUtils.isNotEmpty(webProperties.getAllowedHttpMethods())) {
firewall.setAllowedHttpMethods(webProperties.getAllowedHttpMethods());
}
return firewall;
}
@Bean
@ConditionalOnMissingBean
public RequestRejectedHandler requestRejectedHandler() {
return new HttpStatusRequestRejectedHandler();
}
@Bean
public ExceptionProcessCustomizer errorResponseProcessCustomizer() {
return new ExceptionProcessCustomizer() {
@Override
public Class> getType() {
return ErrorResponse.class;
}
@Override
public ExceptionProcessResponse customize(Throwable exception) {
return new ExceptionProcessResponse(((ErrorResponse) exception).getStatusCode().value(),
exception.getMessage());
}
};
}
@Bean
@ConditionalOnMissingBean
public ExceptionAdvice exceptionAdvice(ErrorAttributes errorAttributes, WebProperties webProperties,
@Nullable ExceptionProcessCustomizer[] exceptionProcessCustomizers) {
return new ExceptionAdvice(errorAttributes, webProperties, exceptionProcessCustomizers);
}
@Bean
@ConditionalOnMissingBean
public ExceptionController exceptionController(ErrorAttributes errorAttributes, WebProperties webProperties,
@Nullable ExceptionProcessCustomizer[] exceptionProcessCustomizers) {
return new ExceptionController(errorAttributes, webProperties, exceptionProcessCustomizers);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy