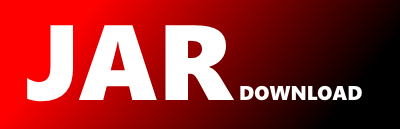
io.grafeas.v1.Occurrence Maven / Gradle / Ivy
/*
* Copyright 2024 The Grafeas Authors. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: grafeas/v1/grafeas.proto
// Protobuf Java Version: 3.25.5
package io.grafeas.v1;
/**
*
*
*
* An instance of an analysis type that has been found on a resource.
*
*
* Protobuf type {@code grafeas.v1.Occurrence}
*/
public final class Occurrence extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:grafeas.v1.Occurrence)
OccurrenceOrBuilder {
private static final long serialVersionUID = 0L;
// Use Occurrence.newBuilder() to construct.
private Occurrence(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Occurrence() {
name_ = "";
resourceUri_ = "";
noteName_ = "";
kind_ = 0;
remediation_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new Occurrence();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return io.grafeas.v1.GrafeasOuterClass.internal_static_grafeas_v1_Occurrence_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.grafeas.v1.GrafeasOuterClass.internal_static_grafeas_v1_Occurrence_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.grafeas.v1.Occurrence.class, io.grafeas.v1.Occurrence.Builder.class);
}
private int bitField0_;
private int detailsCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object details_;
public enum DetailsCase
implements
com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
VULNERABILITY(8),
BUILD(9),
IMAGE(10),
PACKAGE(11),
DEPLOYMENT(12),
DISCOVERY(13),
ATTESTATION(14),
UPGRADE(15),
COMPLIANCE(16),
DSSE_ATTESTATION(17),
SBOM_REFERENCE(19),
DETAILS_NOT_SET(0);
private final int value;
private DetailsCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static DetailsCase valueOf(int value) {
return forNumber(value);
}
public static DetailsCase forNumber(int value) {
switch (value) {
case 8:
return VULNERABILITY;
case 9:
return BUILD;
case 10:
return IMAGE;
case 11:
return PACKAGE;
case 12:
return DEPLOYMENT;
case 13:
return DISCOVERY;
case 14:
return ATTESTATION;
case 15:
return UPGRADE;
case 16:
return COMPLIANCE;
case 17:
return DSSE_ATTESTATION;
case 19:
return SBOM_REFERENCE;
case 0:
return DETAILS_NOT_SET;
default:
return null;
}
}
public int getNumber() {
return this.value;
}
};
public DetailsCase getDetailsCase() {
return DetailsCase.forNumber(detailsCase_);
}
public static final int NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
*
*
* Output only. The name of the occurrence in the form of
* `projects/[PROJECT_ID]/occurrences/[OCCURRENCE_ID]`.
*
*
* string name = 1;
*
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
*
*
* Output only. The name of the occurrence in the form of
* `projects/[PROJECT_ID]/occurrences/[OCCURRENCE_ID]`.
*
*
* string name = 1;
*
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RESOURCE_URI_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object resourceUri_ = "";
/**
*
*
*
* Required. Immutable. A URI that represents the resource for which the
* occurrence applies. For example,
* `https://gcr.io/project/image@sha256:123abc` for a Docker image.
*
*
* string resource_uri = 2;
*
* @return The resourceUri.
*/
@java.lang.Override
public java.lang.String getResourceUri() {
java.lang.Object ref = resourceUri_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceUri_ = s;
return s;
}
}
/**
*
*
*
* Required. Immutable. A URI that represents the resource for which the
* occurrence applies. For example,
* `https://gcr.io/project/image@sha256:123abc` for a Docker image.
*
*
* string resource_uri = 2;
*
* @return The bytes for resourceUri.
*/
@java.lang.Override
public com.google.protobuf.ByteString getResourceUriBytes() {
java.lang.Object ref = resourceUri_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
resourceUri_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NOTE_NAME_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object noteName_ = "";
/**
*
*
*
* Required. Immutable. The analysis note associated with this occurrence, in
* the form of `projects/[PROVIDER_ID]/notes/[NOTE_ID]`. This field can be
* used as a filter in list requests.
*
*
* string note_name = 3;
*
* @return The noteName.
*/
@java.lang.Override
public java.lang.String getNoteName() {
java.lang.Object ref = noteName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
noteName_ = s;
return s;
}
}
/**
*
*
*
* Required. Immutable. The analysis note associated with this occurrence, in
* the form of `projects/[PROVIDER_ID]/notes/[NOTE_ID]`. This field can be
* used as a filter in list requests.
*
*
* string note_name = 3;
*
* @return The bytes for noteName.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNoteNameBytes() {
java.lang.Object ref = noteName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
noteName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int KIND_FIELD_NUMBER = 4;
private int kind_ = 0;
/**
*
*
*
* Output only. This explicitly denotes which of the occurrence details are
* specified. This field can be used as a filter in list requests.
*
*
* .grafeas.v1.NoteKind kind = 4;
*
* @return The enum numeric value on the wire for kind.
*/
@java.lang.Override
public int getKindValue() {
return kind_;
}
/**
*
*
*
* Output only. This explicitly denotes which of the occurrence details are
* specified. This field can be used as a filter in list requests.
*
*
* .grafeas.v1.NoteKind kind = 4;
*
* @return The kind.
*/
@java.lang.Override
public io.grafeas.v1.NoteKind getKind() {
io.grafeas.v1.NoteKind result = io.grafeas.v1.NoteKind.forNumber(kind_);
return result == null ? io.grafeas.v1.NoteKind.UNRECOGNIZED : result;
}
public static final int REMEDIATION_FIELD_NUMBER = 5;
@SuppressWarnings("serial")
private volatile java.lang.Object remediation_ = "";
/**
*
*
*
* A description of actions that can be taken to remedy the note.
*
*
* string remediation = 5;
*
* @return The remediation.
*/
@java.lang.Override
public java.lang.String getRemediation() {
java.lang.Object ref = remediation_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
remediation_ = s;
return s;
}
}
/**
*
*
*
* A description of actions that can be taken to remedy the note.
*
*
* string remediation = 5;
*
* @return The bytes for remediation.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRemediationBytes() {
java.lang.Object ref = remediation_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
remediation_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CREATE_TIME_FIELD_NUMBER = 6;
private com.google.protobuf.Timestamp createTime_;
/**
*
*
*
* Output only. The time this occurrence was created.
*
*
* .google.protobuf.Timestamp create_time = 6;
*
* @return Whether the createTime field is set.
*/
@java.lang.Override
public boolean hasCreateTime() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Output only. The time this occurrence was created.
*
*
* .google.protobuf.Timestamp create_time = 6;
*
* @return The createTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getCreateTime() {
return createTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : createTime_;
}
/**
*
*
*
* Output only. The time this occurrence was created.
*
*
* .google.protobuf.Timestamp create_time = 6;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getCreateTimeOrBuilder() {
return createTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : createTime_;
}
public static final int UPDATE_TIME_FIELD_NUMBER = 7;
private com.google.protobuf.Timestamp updateTime_;
/**
*
*
*
* Output only. The time this occurrence was last updated.
*
*
* .google.protobuf.Timestamp update_time = 7;
*
* @return Whether the updateTime field is set.
*/
@java.lang.Override
public boolean hasUpdateTime() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Output only. The time this occurrence was last updated.
*
*
* .google.protobuf.Timestamp update_time = 7;
*
* @return The updateTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getUpdateTime() {
return updateTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : updateTime_;
}
/**
*
*
*
* Output only. The time this occurrence was last updated.
*
*
* .google.protobuf.Timestamp update_time = 7;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getUpdateTimeOrBuilder() {
return updateTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : updateTime_;
}
public static final int VULNERABILITY_FIELD_NUMBER = 8;
/**
*
*
*
* Describes a security vulnerability.
*
*
* .grafeas.v1.VulnerabilityOccurrence vulnerability = 8;
*
* @return Whether the vulnerability field is set.
*/
@java.lang.Override
public boolean hasVulnerability() {
return detailsCase_ == 8;
}
/**
*
*
*
* Describes a security vulnerability.
*
*
* .grafeas.v1.VulnerabilityOccurrence vulnerability = 8;
*
* @return The vulnerability.
*/
@java.lang.Override
public io.grafeas.v1.VulnerabilityOccurrence getVulnerability() {
if (detailsCase_ == 8) {
return (io.grafeas.v1.VulnerabilityOccurrence) details_;
}
return io.grafeas.v1.VulnerabilityOccurrence.getDefaultInstance();
}
/**
*
*
*
* Describes a security vulnerability.
*
*
* .grafeas.v1.VulnerabilityOccurrence vulnerability = 8;
*/
@java.lang.Override
public io.grafeas.v1.VulnerabilityOccurrenceOrBuilder getVulnerabilityOrBuilder() {
if (detailsCase_ == 8) {
return (io.grafeas.v1.VulnerabilityOccurrence) details_;
}
return io.grafeas.v1.VulnerabilityOccurrence.getDefaultInstance();
}
public static final int BUILD_FIELD_NUMBER = 9;
/**
*
*
*
* Describes a verifiable build.
*
*
* .grafeas.v1.BuildOccurrence build = 9;
*
* @return Whether the build field is set.
*/
@java.lang.Override
public boolean hasBuild() {
return detailsCase_ == 9;
}
/**
*
*
*
* Describes a verifiable build.
*
*
* .grafeas.v1.BuildOccurrence build = 9;
*
* @return The build.
*/
@java.lang.Override
public io.grafeas.v1.BuildOccurrence getBuild() {
if (detailsCase_ == 9) {
return (io.grafeas.v1.BuildOccurrence) details_;
}
return io.grafeas.v1.BuildOccurrence.getDefaultInstance();
}
/**
*
*
*
* Describes a verifiable build.
*
*
* .grafeas.v1.BuildOccurrence build = 9;
*/
@java.lang.Override
public io.grafeas.v1.BuildOccurrenceOrBuilder getBuildOrBuilder() {
if (detailsCase_ == 9) {
return (io.grafeas.v1.BuildOccurrence) details_;
}
return io.grafeas.v1.BuildOccurrence.getDefaultInstance();
}
public static final int IMAGE_FIELD_NUMBER = 10;
/**
*
*
*
* Describes how this resource derives from the basis in the associated
* note.
*
*
* .grafeas.v1.ImageOccurrence image = 10;
*
* @return Whether the image field is set.
*/
@java.lang.Override
public boolean hasImage() {
return detailsCase_ == 10;
}
/**
*
*
*
* Describes how this resource derives from the basis in the associated
* note.
*
*
* .grafeas.v1.ImageOccurrence image = 10;
*
* @return The image.
*/
@java.lang.Override
public io.grafeas.v1.ImageOccurrence getImage() {
if (detailsCase_ == 10) {
return (io.grafeas.v1.ImageOccurrence) details_;
}
return io.grafeas.v1.ImageOccurrence.getDefaultInstance();
}
/**
*
*
*
* Describes how this resource derives from the basis in the associated
* note.
*
*
* .grafeas.v1.ImageOccurrence image = 10;
*/
@java.lang.Override
public io.grafeas.v1.ImageOccurrenceOrBuilder getImageOrBuilder() {
if (detailsCase_ == 10) {
return (io.grafeas.v1.ImageOccurrence) details_;
}
return io.grafeas.v1.ImageOccurrence.getDefaultInstance();
}
public static final int PACKAGE_FIELD_NUMBER = 11;
/**
*
*
*
* Describes the installation of a package on the linked resource.
*
*
* .grafeas.v1.PackageOccurrence package = 11;
*
* @return Whether the package field is set.
*/
@java.lang.Override
public boolean hasPackage() {
return detailsCase_ == 11;
}
/**
*
*
*
* Describes the installation of a package on the linked resource.
*
*
* .grafeas.v1.PackageOccurrence package = 11;
*
* @return The package.
*/
@java.lang.Override
public io.grafeas.v1.PackageOccurrence getPackage() {
if (detailsCase_ == 11) {
return (io.grafeas.v1.PackageOccurrence) details_;
}
return io.grafeas.v1.PackageOccurrence.getDefaultInstance();
}
/**
*
*
*
* Describes the installation of a package on the linked resource.
*
*
* .grafeas.v1.PackageOccurrence package = 11;
*/
@java.lang.Override
public io.grafeas.v1.PackageOccurrenceOrBuilder getPackageOrBuilder() {
if (detailsCase_ == 11) {
return (io.grafeas.v1.PackageOccurrence) details_;
}
return io.grafeas.v1.PackageOccurrence.getDefaultInstance();
}
public static final int DEPLOYMENT_FIELD_NUMBER = 12;
/**
*
*
*
* Describes the deployment of an artifact on a runtime.
*
*
* .grafeas.v1.DeploymentOccurrence deployment = 12;
*
* @return Whether the deployment field is set.
*/
@java.lang.Override
public boolean hasDeployment() {
return detailsCase_ == 12;
}
/**
*
*
*
* Describes the deployment of an artifact on a runtime.
*
*
* .grafeas.v1.DeploymentOccurrence deployment = 12;
*
* @return The deployment.
*/
@java.lang.Override
public io.grafeas.v1.DeploymentOccurrence getDeployment() {
if (detailsCase_ == 12) {
return (io.grafeas.v1.DeploymentOccurrence) details_;
}
return io.grafeas.v1.DeploymentOccurrence.getDefaultInstance();
}
/**
*
*
*
* Describes the deployment of an artifact on a runtime.
*
*
* .grafeas.v1.DeploymentOccurrence deployment = 12;
*/
@java.lang.Override
public io.grafeas.v1.DeploymentOccurrenceOrBuilder getDeploymentOrBuilder() {
if (detailsCase_ == 12) {
return (io.grafeas.v1.DeploymentOccurrence) details_;
}
return io.grafeas.v1.DeploymentOccurrence.getDefaultInstance();
}
public static final int DISCOVERY_FIELD_NUMBER = 13;
/**
*
*
*
* Describes when a resource was discovered.
*
*
* .grafeas.v1.DiscoveryOccurrence discovery = 13;
*
* @return Whether the discovery field is set.
*/
@java.lang.Override
public boolean hasDiscovery() {
return detailsCase_ == 13;
}
/**
*
*
*
* Describes when a resource was discovered.
*
*
* .grafeas.v1.DiscoveryOccurrence discovery = 13;
*
* @return The discovery.
*/
@java.lang.Override
public io.grafeas.v1.DiscoveryOccurrence getDiscovery() {
if (detailsCase_ == 13) {
return (io.grafeas.v1.DiscoveryOccurrence) details_;
}
return io.grafeas.v1.DiscoveryOccurrence.getDefaultInstance();
}
/**
*
*
*
* Describes when a resource was discovered.
*
*
* .grafeas.v1.DiscoveryOccurrence discovery = 13;
*/
@java.lang.Override
public io.grafeas.v1.DiscoveryOccurrenceOrBuilder getDiscoveryOrBuilder() {
if (detailsCase_ == 13) {
return (io.grafeas.v1.DiscoveryOccurrence) details_;
}
return io.grafeas.v1.DiscoveryOccurrence.getDefaultInstance();
}
public static final int ATTESTATION_FIELD_NUMBER = 14;
/**
*
*
*
* Describes an attestation of an artifact.
*
*
* .grafeas.v1.AttestationOccurrence attestation = 14;
*
* @return Whether the attestation field is set.
*/
@java.lang.Override
public boolean hasAttestation() {
return detailsCase_ == 14;
}
/**
*
*
*
* Describes an attestation of an artifact.
*
*
* .grafeas.v1.AttestationOccurrence attestation = 14;
*
* @return The attestation.
*/
@java.lang.Override
public io.grafeas.v1.AttestationOccurrence getAttestation() {
if (detailsCase_ == 14) {
return (io.grafeas.v1.AttestationOccurrence) details_;
}
return io.grafeas.v1.AttestationOccurrence.getDefaultInstance();
}
/**
*
*
*
* Describes an attestation of an artifact.
*
*
* .grafeas.v1.AttestationOccurrence attestation = 14;
*/
@java.lang.Override
public io.grafeas.v1.AttestationOccurrenceOrBuilder getAttestationOrBuilder() {
if (detailsCase_ == 14) {
return (io.grafeas.v1.AttestationOccurrence) details_;
}
return io.grafeas.v1.AttestationOccurrence.getDefaultInstance();
}
public static final int UPGRADE_FIELD_NUMBER = 15;
/**
*
*
*
* Describes an available package upgrade on the linked resource.
*
*
* .grafeas.v1.UpgradeOccurrence upgrade = 15;
*
* @return Whether the upgrade field is set.
*/
@java.lang.Override
public boolean hasUpgrade() {
return detailsCase_ == 15;
}
/**
*
*
*
* Describes an available package upgrade on the linked resource.
*
*
* .grafeas.v1.UpgradeOccurrence upgrade = 15;
*
* @return The upgrade.
*/
@java.lang.Override
public io.grafeas.v1.UpgradeOccurrence getUpgrade() {
if (detailsCase_ == 15) {
return (io.grafeas.v1.UpgradeOccurrence) details_;
}
return io.grafeas.v1.UpgradeOccurrence.getDefaultInstance();
}
/**
*
*
*
* Describes an available package upgrade on the linked resource.
*
*
* .grafeas.v1.UpgradeOccurrence upgrade = 15;
*/
@java.lang.Override
public io.grafeas.v1.UpgradeOccurrenceOrBuilder getUpgradeOrBuilder() {
if (detailsCase_ == 15) {
return (io.grafeas.v1.UpgradeOccurrence) details_;
}
return io.grafeas.v1.UpgradeOccurrence.getDefaultInstance();
}
public static final int COMPLIANCE_FIELD_NUMBER = 16;
/**
*
*
*
* Describes a compliance violation on a linked resource.
*
*
* .grafeas.v1.ComplianceOccurrence compliance = 16;
*
* @return Whether the compliance field is set.
*/
@java.lang.Override
public boolean hasCompliance() {
return detailsCase_ == 16;
}
/**
*
*
*
* Describes a compliance violation on a linked resource.
*
*
* .grafeas.v1.ComplianceOccurrence compliance = 16;
*
* @return The compliance.
*/
@java.lang.Override
public io.grafeas.v1.ComplianceOccurrence getCompliance() {
if (detailsCase_ == 16) {
return (io.grafeas.v1.ComplianceOccurrence) details_;
}
return io.grafeas.v1.ComplianceOccurrence.getDefaultInstance();
}
/**
*
*
*
* Describes a compliance violation on a linked resource.
*
*
* .grafeas.v1.ComplianceOccurrence compliance = 16;
*/
@java.lang.Override
public io.grafeas.v1.ComplianceOccurrenceOrBuilder getComplianceOrBuilder() {
if (detailsCase_ == 16) {
return (io.grafeas.v1.ComplianceOccurrence) details_;
}
return io.grafeas.v1.ComplianceOccurrence.getDefaultInstance();
}
public static final int DSSE_ATTESTATION_FIELD_NUMBER = 17;
/**
*
*
*
* Describes an attestation of an artifact using dsse.
*
*
* .grafeas.v1.DSSEAttestationOccurrence dsse_attestation = 17;
*
* @return Whether the dsseAttestation field is set.
*/
@java.lang.Override
public boolean hasDsseAttestation() {
return detailsCase_ == 17;
}
/**
*
*
*
* Describes an attestation of an artifact using dsse.
*
*
* .grafeas.v1.DSSEAttestationOccurrence dsse_attestation = 17;
*
* @return The dsseAttestation.
*/
@java.lang.Override
public io.grafeas.v1.DSSEAttestationOccurrence getDsseAttestation() {
if (detailsCase_ == 17) {
return (io.grafeas.v1.DSSEAttestationOccurrence) details_;
}
return io.grafeas.v1.DSSEAttestationOccurrence.getDefaultInstance();
}
/**
*
*
*
* Describes an attestation of an artifact using dsse.
*
*
* .grafeas.v1.DSSEAttestationOccurrence dsse_attestation = 17;
*/
@java.lang.Override
public io.grafeas.v1.DSSEAttestationOccurrenceOrBuilder getDsseAttestationOrBuilder() {
if (detailsCase_ == 17) {
return (io.grafeas.v1.DSSEAttestationOccurrence) details_;
}
return io.grafeas.v1.DSSEAttestationOccurrence.getDefaultInstance();
}
public static final int SBOM_REFERENCE_FIELD_NUMBER = 19;
/**
*
*
*
* Describes a specific SBOM reference occurrences.
*
*
* .grafeas.v1.SBOMReferenceOccurrence sbom_reference = 19;
*
* @return Whether the sbomReference field is set.
*/
@java.lang.Override
public boolean hasSbomReference() {
return detailsCase_ == 19;
}
/**
*
*
*
* Describes a specific SBOM reference occurrences.
*
*
* .grafeas.v1.SBOMReferenceOccurrence sbom_reference = 19;
*
* @return The sbomReference.
*/
@java.lang.Override
public io.grafeas.v1.SBOMReferenceOccurrence getSbomReference() {
if (detailsCase_ == 19) {
return (io.grafeas.v1.SBOMReferenceOccurrence) details_;
}
return io.grafeas.v1.SBOMReferenceOccurrence.getDefaultInstance();
}
/**
*
*
*
* Describes a specific SBOM reference occurrences.
*
*
* .grafeas.v1.SBOMReferenceOccurrence sbom_reference = 19;
*/
@java.lang.Override
public io.grafeas.v1.SBOMReferenceOccurrenceOrBuilder getSbomReferenceOrBuilder() {
if (detailsCase_ == 19) {
return (io.grafeas.v1.SBOMReferenceOccurrence) details_;
}
return io.grafeas.v1.SBOMReferenceOccurrence.getDefaultInstance();
}
public static final int ENVELOPE_FIELD_NUMBER = 18;
private io.grafeas.v1.Envelope envelope_;
/**
*
*
*
* https://github.com/secure-systems-lab/dsse
*
*
* .grafeas.v1.Envelope envelope = 18;
*
* @return Whether the envelope field is set.
*/
@java.lang.Override
public boolean hasEnvelope() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* https://github.com/secure-systems-lab/dsse
*
*
* .grafeas.v1.Envelope envelope = 18;
*
* @return The envelope.
*/
@java.lang.Override
public io.grafeas.v1.Envelope getEnvelope() {
return envelope_ == null ? io.grafeas.v1.Envelope.getDefaultInstance() : envelope_;
}
/**
*
*
*
* https://github.com/secure-systems-lab/dsse
*
*
* .grafeas.v1.Envelope envelope = 18;
*/
@java.lang.Override
public io.grafeas.v1.EnvelopeOrBuilder getEnvelopeOrBuilder() {
return envelope_ == null ? io.grafeas.v1.Envelope.getDefaultInstance() : envelope_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceUri_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, resourceUri_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(noteName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, noteName_);
}
if (kind_ != io.grafeas.v1.NoteKind.NOTE_KIND_UNSPECIFIED.getNumber()) {
output.writeEnum(4, kind_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(remediation_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, remediation_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(6, getCreateTime());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(7, getUpdateTime());
}
if (detailsCase_ == 8) {
output.writeMessage(8, (io.grafeas.v1.VulnerabilityOccurrence) details_);
}
if (detailsCase_ == 9) {
output.writeMessage(9, (io.grafeas.v1.BuildOccurrence) details_);
}
if (detailsCase_ == 10) {
output.writeMessage(10, (io.grafeas.v1.ImageOccurrence) details_);
}
if (detailsCase_ == 11) {
output.writeMessage(11, (io.grafeas.v1.PackageOccurrence) details_);
}
if (detailsCase_ == 12) {
output.writeMessage(12, (io.grafeas.v1.DeploymentOccurrence) details_);
}
if (detailsCase_ == 13) {
output.writeMessage(13, (io.grafeas.v1.DiscoveryOccurrence) details_);
}
if (detailsCase_ == 14) {
output.writeMessage(14, (io.grafeas.v1.AttestationOccurrence) details_);
}
if (detailsCase_ == 15) {
output.writeMessage(15, (io.grafeas.v1.UpgradeOccurrence) details_);
}
if (detailsCase_ == 16) {
output.writeMessage(16, (io.grafeas.v1.ComplianceOccurrence) details_);
}
if (detailsCase_ == 17) {
output.writeMessage(17, (io.grafeas.v1.DSSEAttestationOccurrence) details_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(18, getEnvelope());
}
if (detailsCase_ == 19) {
output.writeMessage(19, (io.grafeas.v1.SBOMReferenceOccurrence) details_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceUri_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, resourceUri_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(noteName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, noteName_);
}
if (kind_ != io.grafeas.v1.NoteKind.NOTE_KIND_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream.computeEnumSize(4, kind_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(remediation_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, remediation_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(6, getCreateTime());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(7, getUpdateTime());
}
if (detailsCase_ == 8) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
8, (io.grafeas.v1.VulnerabilityOccurrence) details_);
}
if (detailsCase_ == 9) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
9, (io.grafeas.v1.BuildOccurrence) details_);
}
if (detailsCase_ == 10) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
10, (io.grafeas.v1.ImageOccurrence) details_);
}
if (detailsCase_ == 11) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
11, (io.grafeas.v1.PackageOccurrence) details_);
}
if (detailsCase_ == 12) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
12, (io.grafeas.v1.DeploymentOccurrence) details_);
}
if (detailsCase_ == 13) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
13, (io.grafeas.v1.DiscoveryOccurrence) details_);
}
if (detailsCase_ == 14) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
14, (io.grafeas.v1.AttestationOccurrence) details_);
}
if (detailsCase_ == 15) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
15, (io.grafeas.v1.UpgradeOccurrence) details_);
}
if (detailsCase_ == 16) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
16, (io.grafeas.v1.ComplianceOccurrence) details_);
}
if (detailsCase_ == 17) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
17, (io.grafeas.v1.DSSEAttestationOccurrence) details_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(18, getEnvelope());
}
if (detailsCase_ == 19) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
19, (io.grafeas.v1.SBOMReferenceOccurrence) details_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.grafeas.v1.Occurrence)) {
return super.equals(obj);
}
io.grafeas.v1.Occurrence other = (io.grafeas.v1.Occurrence) obj;
if (!getName().equals(other.getName())) return false;
if (!getResourceUri().equals(other.getResourceUri())) return false;
if (!getNoteName().equals(other.getNoteName())) return false;
if (kind_ != other.kind_) return false;
if (!getRemediation().equals(other.getRemediation())) return false;
if (hasCreateTime() != other.hasCreateTime()) return false;
if (hasCreateTime()) {
if (!getCreateTime().equals(other.getCreateTime())) return false;
}
if (hasUpdateTime() != other.hasUpdateTime()) return false;
if (hasUpdateTime()) {
if (!getUpdateTime().equals(other.getUpdateTime())) return false;
}
if (hasEnvelope() != other.hasEnvelope()) return false;
if (hasEnvelope()) {
if (!getEnvelope().equals(other.getEnvelope())) return false;
}
if (!getDetailsCase().equals(other.getDetailsCase())) return false;
switch (detailsCase_) {
case 8:
if (!getVulnerability().equals(other.getVulnerability())) return false;
break;
case 9:
if (!getBuild().equals(other.getBuild())) return false;
break;
case 10:
if (!getImage().equals(other.getImage())) return false;
break;
case 11:
if (!getPackage().equals(other.getPackage())) return false;
break;
case 12:
if (!getDeployment().equals(other.getDeployment())) return false;
break;
case 13:
if (!getDiscovery().equals(other.getDiscovery())) return false;
break;
case 14:
if (!getAttestation().equals(other.getAttestation())) return false;
break;
case 15:
if (!getUpgrade().equals(other.getUpgrade())) return false;
break;
case 16:
if (!getCompliance().equals(other.getCompliance())) return false;
break;
case 17:
if (!getDsseAttestation().equals(other.getDsseAttestation())) return false;
break;
case 19:
if (!getSbomReference().equals(other.getSbomReference())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + RESOURCE_URI_FIELD_NUMBER;
hash = (53 * hash) + getResourceUri().hashCode();
hash = (37 * hash) + NOTE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getNoteName().hashCode();
hash = (37 * hash) + KIND_FIELD_NUMBER;
hash = (53 * hash) + kind_;
hash = (37 * hash) + REMEDIATION_FIELD_NUMBER;
hash = (53 * hash) + getRemediation().hashCode();
if (hasCreateTime()) {
hash = (37 * hash) + CREATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getCreateTime().hashCode();
}
if (hasUpdateTime()) {
hash = (37 * hash) + UPDATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getUpdateTime().hashCode();
}
if (hasEnvelope()) {
hash = (37 * hash) + ENVELOPE_FIELD_NUMBER;
hash = (53 * hash) + getEnvelope().hashCode();
}
switch (detailsCase_) {
case 8:
hash = (37 * hash) + VULNERABILITY_FIELD_NUMBER;
hash = (53 * hash) + getVulnerability().hashCode();
break;
case 9:
hash = (37 * hash) + BUILD_FIELD_NUMBER;
hash = (53 * hash) + getBuild().hashCode();
break;
case 10:
hash = (37 * hash) + IMAGE_FIELD_NUMBER;
hash = (53 * hash) + getImage().hashCode();
break;
case 11:
hash = (37 * hash) + PACKAGE_FIELD_NUMBER;
hash = (53 * hash) + getPackage().hashCode();
break;
case 12:
hash = (37 * hash) + DEPLOYMENT_FIELD_NUMBER;
hash = (53 * hash) + getDeployment().hashCode();
break;
case 13:
hash = (37 * hash) + DISCOVERY_FIELD_NUMBER;
hash = (53 * hash) + getDiscovery().hashCode();
break;
case 14:
hash = (37 * hash) + ATTESTATION_FIELD_NUMBER;
hash = (53 * hash) + getAttestation().hashCode();
break;
case 15:
hash = (37 * hash) + UPGRADE_FIELD_NUMBER;
hash = (53 * hash) + getUpgrade().hashCode();
break;
case 16:
hash = (37 * hash) + COMPLIANCE_FIELD_NUMBER;
hash = (53 * hash) + getCompliance().hashCode();
break;
case 17:
hash = (37 * hash) + DSSE_ATTESTATION_FIELD_NUMBER;
hash = (53 * hash) + getDsseAttestation().hashCode();
break;
case 19:
hash = (37 * hash) + SBOM_REFERENCE_FIELD_NUMBER;
hash = (53 * hash) + getSbomReference().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.grafeas.v1.Occurrence parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grafeas.v1.Occurrence parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grafeas.v1.Occurrence parseFrom(com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grafeas.v1.Occurrence parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grafeas.v1.Occurrence parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grafeas.v1.Occurrence parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grafeas.v1.Occurrence parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static io.grafeas.v1.Occurrence parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static io.grafeas.v1.Occurrence parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static io.grafeas.v1.Occurrence parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static io.grafeas.v1.Occurrence parseFrom(com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static io.grafeas.v1.Occurrence parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.grafeas.v1.Occurrence prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* An instance of an analysis type that has been found on a resource.
*
*
* Protobuf type {@code grafeas.v1.Occurrence}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:grafeas.v1.Occurrence)
io.grafeas.v1.OccurrenceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return io.grafeas.v1.GrafeasOuterClass.internal_static_grafeas_v1_Occurrence_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.grafeas.v1.GrafeasOuterClass
.internal_static_grafeas_v1_Occurrence_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.grafeas.v1.Occurrence.class, io.grafeas.v1.Occurrence.Builder.class);
}
// Construct using io.grafeas.v1.Occurrence.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getCreateTimeFieldBuilder();
getUpdateTimeFieldBuilder();
getEnvelopeFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
name_ = "";
resourceUri_ = "";
noteName_ = "";
kind_ = 0;
remediation_ = "";
createTime_ = null;
if (createTimeBuilder_ != null) {
createTimeBuilder_.dispose();
createTimeBuilder_ = null;
}
updateTime_ = null;
if (updateTimeBuilder_ != null) {
updateTimeBuilder_.dispose();
updateTimeBuilder_ = null;
}
if (vulnerabilityBuilder_ != null) {
vulnerabilityBuilder_.clear();
}
if (buildBuilder_ != null) {
buildBuilder_.clear();
}
if (imageBuilder_ != null) {
imageBuilder_.clear();
}
if (packageBuilder_ != null) {
packageBuilder_.clear();
}
if (deploymentBuilder_ != null) {
deploymentBuilder_.clear();
}
if (discoveryBuilder_ != null) {
discoveryBuilder_.clear();
}
if (attestationBuilder_ != null) {
attestationBuilder_.clear();
}
if (upgradeBuilder_ != null) {
upgradeBuilder_.clear();
}
if (complianceBuilder_ != null) {
complianceBuilder_.clear();
}
if (dsseAttestationBuilder_ != null) {
dsseAttestationBuilder_.clear();
}
if (sbomReferenceBuilder_ != null) {
sbomReferenceBuilder_.clear();
}
envelope_ = null;
if (envelopeBuilder_ != null) {
envelopeBuilder_.dispose();
envelopeBuilder_ = null;
}
detailsCase_ = 0;
details_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return io.grafeas.v1.GrafeasOuterClass.internal_static_grafeas_v1_Occurrence_descriptor;
}
@java.lang.Override
public io.grafeas.v1.Occurrence getDefaultInstanceForType() {
return io.grafeas.v1.Occurrence.getDefaultInstance();
}
@java.lang.Override
public io.grafeas.v1.Occurrence build() {
io.grafeas.v1.Occurrence result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.grafeas.v1.Occurrence buildPartial() {
io.grafeas.v1.Occurrence result = new io.grafeas.v1.Occurrence(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(io.grafeas.v1.Occurrence result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.name_ = name_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.resourceUri_ = resourceUri_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.noteName_ = noteName_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.kind_ = kind_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.remediation_ = remediation_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000020) != 0)) {
result.createTime_ = createTimeBuilder_ == null ? createTime_ : createTimeBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.updateTime_ = updateTimeBuilder_ == null ? updateTime_ : updateTimeBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00040000) != 0)) {
result.envelope_ = envelopeBuilder_ == null ? envelope_ : envelopeBuilder_.build();
to_bitField0_ |= 0x00000004;
}
result.bitField0_ |= to_bitField0_;
}
private void buildPartialOneofs(io.grafeas.v1.Occurrence result) {
result.detailsCase_ = detailsCase_;
result.details_ = this.details_;
if (detailsCase_ == 8 && vulnerabilityBuilder_ != null) {
result.details_ = vulnerabilityBuilder_.build();
}
if (detailsCase_ == 9 && buildBuilder_ != null) {
result.details_ = buildBuilder_.build();
}
if (detailsCase_ == 10 && imageBuilder_ != null) {
result.details_ = imageBuilder_.build();
}
if (detailsCase_ == 11 && packageBuilder_ != null) {
result.details_ = packageBuilder_.build();
}
if (detailsCase_ == 12 && deploymentBuilder_ != null) {
result.details_ = deploymentBuilder_.build();
}
if (detailsCase_ == 13 && discoveryBuilder_ != null) {
result.details_ = discoveryBuilder_.build();
}
if (detailsCase_ == 14 && attestationBuilder_ != null) {
result.details_ = attestationBuilder_.build();
}
if (detailsCase_ == 15 && upgradeBuilder_ != null) {
result.details_ = upgradeBuilder_.build();
}
if (detailsCase_ == 16 && complianceBuilder_ != null) {
result.details_ = complianceBuilder_.build();
}
if (detailsCase_ == 17 && dsseAttestationBuilder_ != null) {
result.details_ = dsseAttestationBuilder_.build();
}
if (detailsCase_ == 19 && sbomReferenceBuilder_ != null) {
result.details_ = sbomReferenceBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.grafeas.v1.Occurrence) {
return mergeFrom((io.grafeas.v1.Occurrence) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.grafeas.v1.Occurrence other) {
if (other == io.grafeas.v1.Occurrence.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getResourceUri().isEmpty()) {
resourceUri_ = other.resourceUri_;
bitField0_ |= 0x00000002;
onChanged();
}
if (!other.getNoteName().isEmpty()) {
noteName_ = other.noteName_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.kind_ != 0) {
setKindValue(other.getKindValue());
}
if (!other.getRemediation().isEmpty()) {
remediation_ = other.remediation_;
bitField0_ |= 0x00000010;
onChanged();
}
if (other.hasCreateTime()) {
mergeCreateTime(other.getCreateTime());
}
if (other.hasUpdateTime()) {
mergeUpdateTime(other.getUpdateTime());
}
if (other.hasEnvelope()) {
mergeEnvelope(other.getEnvelope());
}
switch (other.getDetailsCase()) {
case VULNERABILITY:
{
mergeVulnerability(other.getVulnerability());
break;
}
case BUILD:
{
mergeBuild(other.getBuild());
break;
}
case IMAGE:
{
mergeImage(other.getImage());
break;
}
case PACKAGE:
{
mergePackage(other.getPackage());
break;
}
case DEPLOYMENT:
{
mergeDeployment(other.getDeployment());
break;
}
case DISCOVERY:
{
mergeDiscovery(other.getDiscovery());
break;
}
case ATTESTATION:
{
mergeAttestation(other.getAttestation());
break;
}
case UPGRADE:
{
mergeUpgrade(other.getUpgrade());
break;
}
case COMPLIANCE:
{
mergeCompliance(other.getCompliance());
break;
}
case DSSE_ATTESTATION:
{
mergeDsseAttestation(other.getDsseAttestation());
break;
}
case SBOM_REFERENCE:
{
mergeSbomReference(other.getSbomReference());
break;
}
case DETAILS_NOT_SET:
{
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18:
{
resourceUri_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26:
{
noteName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 26
case 32:
{
kind_ = input.readEnum();
bitField0_ |= 0x00000008;
break;
} // case 32
case 42:
{
remediation_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 42
case 50:
{
input.readMessage(getCreateTimeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000020;
break;
} // case 50
case 58:
{
input.readMessage(getUpdateTimeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000040;
break;
} // case 58
case 66:
{
input.readMessage(getVulnerabilityFieldBuilder().getBuilder(), extensionRegistry);
detailsCase_ = 8;
break;
} // case 66
case 74:
{
input.readMessage(getBuildFieldBuilder().getBuilder(), extensionRegistry);
detailsCase_ = 9;
break;
} // case 74
case 82:
{
input.readMessage(getImageFieldBuilder().getBuilder(), extensionRegistry);
detailsCase_ = 10;
break;
} // case 82
case 90:
{
input.readMessage(getPackageFieldBuilder().getBuilder(), extensionRegistry);
detailsCase_ = 11;
break;
} // case 90
case 98:
{
input.readMessage(getDeploymentFieldBuilder().getBuilder(), extensionRegistry);
detailsCase_ = 12;
break;
} // case 98
case 106:
{
input.readMessage(getDiscoveryFieldBuilder().getBuilder(), extensionRegistry);
detailsCase_ = 13;
break;
} // case 106
case 114:
{
input.readMessage(getAttestationFieldBuilder().getBuilder(), extensionRegistry);
detailsCase_ = 14;
break;
} // case 114
case 122:
{
input.readMessage(getUpgradeFieldBuilder().getBuilder(), extensionRegistry);
detailsCase_ = 15;
break;
} // case 122
case 130:
{
input.readMessage(getComplianceFieldBuilder().getBuilder(), extensionRegistry);
detailsCase_ = 16;
break;
} // case 130
case 138:
{
input.readMessage(getDsseAttestationFieldBuilder().getBuilder(), extensionRegistry);
detailsCase_ = 17;
break;
} // case 138
case 146:
{
input.readMessage(getEnvelopeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00040000;
break;
} // case 146
case 154:
{
input.readMessage(getSbomReferenceFieldBuilder().getBuilder(), extensionRegistry);
detailsCase_ = 19;
break;
} // case 154
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int detailsCase_ = 0;
private java.lang.Object details_;
public DetailsCase getDetailsCase() {
return DetailsCase.forNumber(detailsCase_);
}
public Builder clearDetails() {
detailsCase_ = 0;
details_ = null;
onChanged();
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
*
*
*
* Output only. The name of the occurrence in the form of
* `projects/[PROJECT_ID]/occurrences/[OCCURRENCE_ID]`.
*
*
* string name = 1;
*
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Output only. The name of the occurrence in the form of
* `projects/[PROJECT_ID]/occurrences/[OCCURRENCE_ID]`.
*
*
* string name = 1;
*
* @return The bytes for name.
*/
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Output only. The name of the occurrence in the form of
* `projects/[PROJECT_ID]/occurrences/[OCCURRENCE_ID]`.
*
*
* string name = 1;
*
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Output only. The name of the occurrence in the form of
* `projects/[PROJECT_ID]/occurrences/[OCCURRENCE_ID]`.
*
*
* string name = 1;
*
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* Output only. The name of the occurrence in the form of
* `projects/[PROJECT_ID]/occurrences/[OCCURRENCE_ID]`.
*
*
* string name = 1;
*
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object resourceUri_ = "";
/**
*
*
*
* Required. Immutable. A URI that represents the resource for which the
* occurrence applies. For example,
* `https://gcr.io/project/image@sha256:123abc` for a Docker image.
*
*
* string resource_uri = 2;
*
* @return The resourceUri.
*/
public java.lang.String getResourceUri() {
java.lang.Object ref = resourceUri_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceUri_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Required. Immutable. A URI that represents the resource for which the
* occurrence applies. For example,
* `https://gcr.io/project/image@sha256:123abc` for a Docker image.
*
*
* string resource_uri = 2;
*
* @return The bytes for resourceUri.
*/
public com.google.protobuf.ByteString getResourceUriBytes() {
java.lang.Object ref = resourceUri_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
resourceUri_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Required. Immutable. A URI that represents the resource for which the
* occurrence applies. For example,
* `https://gcr.io/project/image@sha256:123abc` for a Docker image.
*
*
* string resource_uri = 2;
*
* @param value The resourceUri to set.
* @return This builder for chaining.
*/
public Builder setResourceUri(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
resourceUri_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Required. Immutable. A URI that represents the resource for which the
* occurrence applies. For example,
* `https://gcr.io/project/image@sha256:123abc` for a Docker image.
*
*
* string resource_uri = 2;
*
* @return This builder for chaining.
*/
public Builder clearResourceUri() {
resourceUri_ = getDefaultInstance().getResourceUri();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
* Required. Immutable. A URI that represents the resource for which the
* occurrence applies. For example,
* `https://gcr.io/project/image@sha256:123abc` for a Docker image.
*
*
* string resource_uri = 2;
*
* @param value The bytes for resourceUri to set.
* @return This builder for chaining.
*/
public Builder setResourceUriBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
resourceUri_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object noteName_ = "";
/**
*
*
*
* Required. Immutable. The analysis note associated with this occurrence, in
* the form of `projects/[PROVIDER_ID]/notes/[NOTE_ID]`. This field can be
* used as a filter in list requests.
*
*
* string note_name = 3;
*
* @return The noteName.
*/
public java.lang.String getNoteName() {
java.lang.Object ref = noteName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
noteName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Required. Immutable. The analysis note associated with this occurrence, in
* the form of `projects/[PROVIDER_ID]/notes/[NOTE_ID]`. This field can be
* used as a filter in list requests.
*
*
* string note_name = 3;
*
* @return The bytes for noteName.
*/
public com.google.protobuf.ByteString getNoteNameBytes() {
java.lang.Object ref = noteName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
noteName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Required. Immutable. The analysis note associated with this occurrence, in
* the form of `projects/[PROVIDER_ID]/notes/[NOTE_ID]`. This field can be
* used as a filter in list requests.
*
*
* string note_name = 3;
*
* @param value The noteName to set.
* @return This builder for chaining.
*/
public Builder setNoteName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
noteName_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Required. Immutable. The analysis note associated with this occurrence, in
* the form of `projects/[PROVIDER_ID]/notes/[NOTE_ID]`. This field can be
* used as a filter in list requests.
*
*
* string note_name = 3;
*
* @return This builder for chaining.
*/
public Builder clearNoteName() {
noteName_ = getDefaultInstance().getNoteName();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
*
*
* Required. Immutable. The analysis note associated with this occurrence, in
* the form of `projects/[PROVIDER_ID]/notes/[NOTE_ID]`. This field can be
* used as a filter in list requests.
*
*
* string note_name = 3;
*
* @param value The bytes for noteName to set.
* @return This builder for chaining.
*/
public Builder setNoteNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
noteName_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private int kind_ = 0;
/**
*
*
*
* Output only. This explicitly denotes which of the occurrence details are
* specified. This field can be used as a filter in list requests.
*
*
* .grafeas.v1.NoteKind kind = 4;
*
* @return The enum numeric value on the wire for kind.
*/
@java.lang.Override
public int getKindValue() {
return kind_;
}
/**
*
*
*
* Output only. This explicitly denotes which of the occurrence details are
* specified. This field can be used as a filter in list requests.
*
*
* .grafeas.v1.NoteKind kind = 4;
*
* @param value The enum numeric value on the wire for kind to set.
* @return This builder for chaining.
*/
public Builder setKindValue(int value) {
kind_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Output only. This explicitly denotes which of the occurrence details are
* specified. This field can be used as a filter in list requests.
*
*
* .grafeas.v1.NoteKind kind = 4;
*
* @return The kind.
*/
@java.lang.Override
public io.grafeas.v1.NoteKind getKind() {
io.grafeas.v1.NoteKind result = io.grafeas.v1.NoteKind.forNumber(kind_);
return result == null ? io.grafeas.v1.NoteKind.UNRECOGNIZED : result;
}
/**
*
*
*
* Output only. This explicitly denotes which of the occurrence details are
* specified. This field can be used as a filter in list requests.
*
*
* .grafeas.v1.NoteKind kind = 4;
*
* @param value The kind to set.
* @return This builder for chaining.
*/
public Builder setKind(io.grafeas.v1.NoteKind value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
kind_ = value.getNumber();
onChanged();
return this;
}
/**
*
*
*
* Output only. This explicitly denotes which of the occurrence details are
* specified. This field can be used as a filter in list requests.
*
*
* .grafeas.v1.NoteKind kind = 4;
*
* @return This builder for chaining.
*/
public Builder clearKind() {
bitField0_ = (bitField0_ & ~0x00000008);
kind_ = 0;
onChanged();
return this;
}
private java.lang.Object remediation_ = "";
/**
*
*
*
* A description of actions that can be taken to remedy the note.
*
*
* string remediation = 5;
*
* @return The remediation.
*/
public java.lang.String getRemediation() {
java.lang.Object ref = remediation_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
remediation_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* A description of actions that can be taken to remedy the note.
*
*
* string remediation = 5;
*
* @return The bytes for remediation.
*/
public com.google.protobuf.ByteString getRemediationBytes() {
java.lang.Object ref = remediation_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
remediation_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* A description of actions that can be taken to remedy the note.
*
*
* string remediation = 5;
*
* @param value The remediation to set.
* @return This builder for chaining.
*/
public Builder setRemediation(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
remediation_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* A description of actions that can be taken to remedy the note.
*
*
* string remediation = 5;
*
* @return This builder for chaining.
*/
public Builder clearRemediation() {
remediation_ = getDefaultInstance().getRemediation();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
*
*
*
* A description of actions that can be taken to remedy the note.
*
*
* string remediation = 5;
*
* @param value The bytes for remediation to set.
* @return This builder for chaining.
*/
public Builder setRemediationBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
remediation_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private com.google.protobuf.Timestamp createTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
createTimeBuilder_;
/**
*
*
*
* Output only. The time this occurrence was created.
*
*
* .google.protobuf.Timestamp create_time = 6;
*
* @return Whether the createTime field is set.
*/
public boolean hasCreateTime() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Output only. The time this occurrence was created.
*
*
* .google.protobuf.Timestamp create_time = 6;
*
* @return The createTime.
*/
public com.google.protobuf.Timestamp getCreateTime() {
if (createTimeBuilder_ == null) {
return createTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: createTime_;
} else {
return createTimeBuilder_.getMessage();
}
}
/**
*
*
*
* Output only. The time this occurrence was created.
*
*
* .google.protobuf.Timestamp create_time = 6;
*/
public Builder setCreateTime(com.google.protobuf.Timestamp value) {
if (createTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
createTime_ = value;
} else {
createTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Output only. The time this occurrence was created.
*
*
* .google.protobuf.Timestamp create_time = 6;
*/
public Builder setCreateTime(com.google.protobuf.Timestamp.Builder builderForValue) {
if (createTimeBuilder_ == null) {
createTime_ = builderForValue.build();
} else {
createTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Output only. The time this occurrence was created.
*
*
* .google.protobuf.Timestamp create_time = 6;
*/
public Builder mergeCreateTime(com.google.protobuf.Timestamp value) {
if (createTimeBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)
&& createTime_ != null
&& createTime_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getCreateTimeBuilder().mergeFrom(value);
} else {
createTime_ = value;
}
} else {
createTimeBuilder_.mergeFrom(value);
}
if (createTime_ != null) {
bitField0_ |= 0x00000020;
onChanged();
}
return this;
}
/**
*
*
*
* Output only. The time this occurrence was created.
*
*
* .google.protobuf.Timestamp create_time = 6;
*/
public Builder clearCreateTime() {
bitField0_ = (bitField0_ & ~0x00000020);
createTime_ = null;
if (createTimeBuilder_ != null) {
createTimeBuilder_.dispose();
createTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Output only. The time this occurrence was created.
*
*
* .google.protobuf.Timestamp create_time = 6;
*/
public com.google.protobuf.Timestamp.Builder getCreateTimeBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getCreateTimeFieldBuilder().getBuilder();
}
/**
*
*
*
* Output only. The time this occurrence was created.
*
*
* .google.protobuf.Timestamp create_time = 6;
*/
public com.google.protobuf.TimestampOrBuilder getCreateTimeOrBuilder() {
if (createTimeBuilder_ != null) {
return createTimeBuilder_.getMessageOrBuilder();
} else {
return createTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: createTime_;
}
}
/**
*
*
*
* Output only. The time this occurrence was created.
*
*
* .google.protobuf.Timestamp create_time = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
getCreateTimeFieldBuilder() {
if (createTimeBuilder_ == null) {
createTimeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>(
getCreateTime(), getParentForChildren(), isClean());
createTime_ = null;
}
return createTimeBuilder_;
}
private com.google.protobuf.Timestamp updateTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
updateTimeBuilder_;
/**
*
*
*
* Output only. The time this occurrence was last updated.
*
*
* .google.protobuf.Timestamp update_time = 7;
*
* @return Whether the updateTime field is set.
*/
public boolean hasUpdateTime() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Output only. The time this occurrence was last updated.
*
*
* .google.protobuf.Timestamp update_time = 7;
*
* @return The updateTime.
*/
public com.google.protobuf.Timestamp getUpdateTime() {
if (updateTimeBuilder_ == null) {
return updateTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: updateTime_;
} else {
return updateTimeBuilder_.getMessage();
}
}
/**
*
*
*
* Output only. The time this occurrence was last updated.
*
*
* .google.protobuf.Timestamp update_time = 7;
*/
public Builder setUpdateTime(com.google.protobuf.Timestamp value) {
if (updateTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
updateTime_ = value;
} else {
updateTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Output only. The time this occurrence was last updated.
*
*
* .google.protobuf.Timestamp update_time = 7;
*/
public Builder setUpdateTime(com.google.protobuf.Timestamp.Builder builderForValue) {
if (updateTimeBuilder_ == null) {
updateTime_ = builderForValue.build();
} else {
updateTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Output only. The time this occurrence was last updated.
*
*
* .google.protobuf.Timestamp update_time = 7;
*/
public Builder mergeUpdateTime(com.google.protobuf.Timestamp value) {
if (updateTimeBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0)
&& updateTime_ != null
&& updateTime_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getUpdateTimeBuilder().mergeFrom(value);
} else {
updateTime_ = value;
}
} else {
updateTimeBuilder_.mergeFrom(value);
}
if (updateTime_ != null) {
bitField0_ |= 0x00000040;
onChanged();
}
return this;
}
/**
*
*
*
* Output only. The time this occurrence was last updated.
*
*
* .google.protobuf.Timestamp update_time = 7;
*/
public Builder clearUpdateTime() {
bitField0_ = (bitField0_ & ~0x00000040);
updateTime_ = null;
if (updateTimeBuilder_ != null) {
updateTimeBuilder_.dispose();
updateTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Output only. The time this occurrence was last updated.
*
*
* .google.protobuf.Timestamp update_time = 7;
*/
public com.google.protobuf.Timestamp.Builder getUpdateTimeBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getUpdateTimeFieldBuilder().getBuilder();
}
/**
*
*
*
* Output only. The time this occurrence was last updated.
*
*
* .google.protobuf.Timestamp update_time = 7;
*/
public com.google.protobuf.TimestampOrBuilder getUpdateTimeOrBuilder() {
if (updateTimeBuilder_ != null) {
return updateTimeBuilder_.getMessageOrBuilder();
} else {
return updateTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: updateTime_;
}
}
/**
*
*
*
* Output only. The time this occurrence was last updated.
*
*
* .google.protobuf.Timestamp update_time = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
getUpdateTimeFieldBuilder() {
if (updateTimeBuilder_ == null) {
updateTimeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>(
getUpdateTime(), getParentForChildren(), isClean());
updateTime_ = null;
}
return updateTimeBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.VulnerabilityOccurrence,
io.grafeas.v1.VulnerabilityOccurrence.Builder,
io.grafeas.v1.VulnerabilityOccurrenceOrBuilder>
vulnerabilityBuilder_;
/**
*
*
*
* Describes a security vulnerability.
*
*
* .grafeas.v1.VulnerabilityOccurrence vulnerability = 8;
*
* @return Whether the vulnerability field is set.
*/
@java.lang.Override
public boolean hasVulnerability() {
return detailsCase_ == 8;
}
/**
*
*
*
* Describes a security vulnerability.
*
*
* .grafeas.v1.VulnerabilityOccurrence vulnerability = 8;
*
* @return The vulnerability.
*/
@java.lang.Override
public io.grafeas.v1.VulnerabilityOccurrence getVulnerability() {
if (vulnerabilityBuilder_ == null) {
if (detailsCase_ == 8) {
return (io.grafeas.v1.VulnerabilityOccurrence) details_;
}
return io.grafeas.v1.VulnerabilityOccurrence.getDefaultInstance();
} else {
if (detailsCase_ == 8) {
return vulnerabilityBuilder_.getMessage();
}
return io.grafeas.v1.VulnerabilityOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes a security vulnerability.
*
*
* .grafeas.v1.VulnerabilityOccurrence vulnerability = 8;
*/
public Builder setVulnerability(io.grafeas.v1.VulnerabilityOccurrence value) {
if (vulnerabilityBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
details_ = value;
onChanged();
} else {
vulnerabilityBuilder_.setMessage(value);
}
detailsCase_ = 8;
return this;
}
/**
*
*
*
* Describes a security vulnerability.
*
*
* .grafeas.v1.VulnerabilityOccurrence vulnerability = 8;
*/
public Builder setVulnerability(io.grafeas.v1.VulnerabilityOccurrence.Builder builderForValue) {
if (vulnerabilityBuilder_ == null) {
details_ = builderForValue.build();
onChanged();
} else {
vulnerabilityBuilder_.setMessage(builderForValue.build());
}
detailsCase_ = 8;
return this;
}
/**
*
*
*
* Describes a security vulnerability.
*
*
* .grafeas.v1.VulnerabilityOccurrence vulnerability = 8;
*/
public Builder mergeVulnerability(io.grafeas.v1.VulnerabilityOccurrence value) {
if (vulnerabilityBuilder_ == null) {
if (detailsCase_ == 8
&& details_ != io.grafeas.v1.VulnerabilityOccurrence.getDefaultInstance()) {
details_ =
io.grafeas.v1.VulnerabilityOccurrence.newBuilder(
(io.grafeas.v1.VulnerabilityOccurrence) details_)
.mergeFrom(value)
.buildPartial();
} else {
details_ = value;
}
onChanged();
} else {
if (detailsCase_ == 8) {
vulnerabilityBuilder_.mergeFrom(value);
} else {
vulnerabilityBuilder_.setMessage(value);
}
}
detailsCase_ = 8;
return this;
}
/**
*
*
*
* Describes a security vulnerability.
*
*
* .grafeas.v1.VulnerabilityOccurrence vulnerability = 8;
*/
public Builder clearVulnerability() {
if (vulnerabilityBuilder_ == null) {
if (detailsCase_ == 8) {
detailsCase_ = 0;
details_ = null;
onChanged();
}
} else {
if (detailsCase_ == 8) {
detailsCase_ = 0;
details_ = null;
}
vulnerabilityBuilder_.clear();
}
return this;
}
/**
*
*
*
* Describes a security vulnerability.
*
*
* .grafeas.v1.VulnerabilityOccurrence vulnerability = 8;
*/
public io.grafeas.v1.VulnerabilityOccurrence.Builder getVulnerabilityBuilder() {
return getVulnerabilityFieldBuilder().getBuilder();
}
/**
*
*
*
* Describes a security vulnerability.
*
*
* .grafeas.v1.VulnerabilityOccurrence vulnerability = 8;
*/
@java.lang.Override
public io.grafeas.v1.VulnerabilityOccurrenceOrBuilder getVulnerabilityOrBuilder() {
if ((detailsCase_ == 8) && (vulnerabilityBuilder_ != null)) {
return vulnerabilityBuilder_.getMessageOrBuilder();
} else {
if (detailsCase_ == 8) {
return (io.grafeas.v1.VulnerabilityOccurrence) details_;
}
return io.grafeas.v1.VulnerabilityOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes a security vulnerability.
*
*
* .grafeas.v1.VulnerabilityOccurrence vulnerability = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.VulnerabilityOccurrence,
io.grafeas.v1.VulnerabilityOccurrence.Builder,
io.grafeas.v1.VulnerabilityOccurrenceOrBuilder>
getVulnerabilityFieldBuilder() {
if (vulnerabilityBuilder_ == null) {
if (!(detailsCase_ == 8)) {
details_ = io.grafeas.v1.VulnerabilityOccurrence.getDefaultInstance();
}
vulnerabilityBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.VulnerabilityOccurrence,
io.grafeas.v1.VulnerabilityOccurrence.Builder,
io.grafeas.v1.VulnerabilityOccurrenceOrBuilder>(
(io.grafeas.v1.VulnerabilityOccurrence) details_,
getParentForChildren(),
isClean());
details_ = null;
}
detailsCase_ = 8;
onChanged();
return vulnerabilityBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.BuildOccurrence,
io.grafeas.v1.BuildOccurrence.Builder,
io.grafeas.v1.BuildOccurrenceOrBuilder>
buildBuilder_;
/**
*
*
*
* Describes a verifiable build.
*
*
* .grafeas.v1.BuildOccurrence build = 9;
*
* @return Whether the build field is set.
*/
@java.lang.Override
public boolean hasBuild() {
return detailsCase_ == 9;
}
/**
*
*
*
* Describes a verifiable build.
*
*
* .grafeas.v1.BuildOccurrence build = 9;
*
* @return The build.
*/
@java.lang.Override
public io.grafeas.v1.BuildOccurrence getBuild() {
if (buildBuilder_ == null) {
if (detailsCase_ == 9) {
return (io.grafeas.v1.BuildOccurrence) details_;
}
return io.grafeas.v1.BuildOccurrence.getDefaultInstance();
} else {
if (detailsCase_ == 9) {
return buildBuilder_.getMessage();
}
return io.grafeas.v1.BuildOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes a verifiable build.
*
*
* .grafeas.v1.BuildOccurrence build = 9;
*/
public Builder setBuild(io.grafeas.v1.BuildOccurrence value) {
if (buildBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
details_ = value;
onChanged();
} else {
buildBuilder_.setMessage(value);
}
detailsCase_ = 9;
return this;
}
/**
*
*
*
* Describes a verifiable build.
*
*
* .grafeas.v1.BuildOccurrence build = 9;
*/
public Builder setBuild(io.grafeas.v1.BuildOccurrence.Builder builderForValue) {
if (buildBuilder_ == null) {
details_ = builderForValue.build();
onChanged();
} else {
buildBuilder_.setMessage(builderForValue.build());
}
detailsCase_ = 9;
return this;
}
/**
*
*
*
* Describes a verifiable build.
*
*
* .grafeas.v1.BuildOccurrence build = 9;
*/
public Builder mergeBuild(io.grafeas.v1.BuildOccurrence value) {
if (buildBuilder_ == null) {
if (detailsCase_ == 9 && details_ != io.grafeas.v1.BuildOccurrence.getDefaultInstance()) {
details_ =
io.grafeas.v1.BuildOccurrence.newBuilder((io.grafeas.v1.BuildOccurrence) details_)
.mergeFrom(value)
.buildPartial();
} else {
details_ = value;
}
onChanged();
} else {
if (detailsCase_ == 9) {
buildBuilder_.mergeFrom(value);
} else {
buildBuilder_.setMessage(value);
}
}
detailsCase_ = 9;
return this;
}
/**
*
*
*
* Describes a verifiable build.
*
*
* .grafeas.v1.BuildOccurrence build = 9;
*/
public Builder clearBuild() {
if (buildBuilder_ == null) {
if (detailsCase_ == 9) {
detailsCase_ = 0;
details_ = null;
onChanged();
}
} else {
if (detailsCase_ == 9) {
detailsCase_ = 0;
details_ = null;
}
buildBuilder_.clear();
}
return this;
}
/**
*
*
*
* Describes a verifiable build.
*
*
* .grafeas.v1.BuildOccurrence build = 9;
*/
public io.grafeas.v1.BuildOccurrence.Builder getBuildBuilder() {
return getBuildFieldBuilder().getBuilder();
}
/**
*
*
*
* Describes a verifiable build.
*
*
* .grafeas.v1.BuildOccurrence build = 9;
*/
@java.lang.Override
public io.grafeas.v1.BuildOccurrenceOrBuilder getBuildOrBuilder() {
if ((detailsCase_ == 9) && (buildBuilder_ != null)) {
return buildBuilder_.getMessageOrBuilder();
} else {
if (detailsCase_ == 9) {
return (io.grafeas.v1.BuildOccurrence) details_;
}
return io.grafeas.v1.BuildOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes a verifiable build.
*
*
* .grafeas.v1.BuildOccurrence build = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.BuildOccurrence,
io.grafeas.v1.BuildOccurrence.Builder,
io.grafeas.v1.BuildOccurrenceOrBuilder>
getBuildFieldBuilder() {
if (buildBuilder_ == null) {
if (!(detailsCase_ == 9)) {
details_ = io.grafeas.v1.BuildOccurrence.getDefaultInstance();
}
buildBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.BuildOccurrence,
io.grafeas.v1.BuildOccurrence.Builder,
io.grafeas.v1.BuildOccurrenceOrBuilder>(
(io.grafeas.v1.BuildOccurrence) details_, getParentForChildren(), isClean());
details_ = null;
}
detailsCase_ = 9;
onChanged();
return buildBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.ImageOccurrence,
io.grafeas.v1.ImageOccurrence.Builder,
io.grafeas.v1.ImageOccurrenceOrBuilder>
imageBuilder_;
/**
*
*
*
* Describes how this resource derives from the basis in the associated
* note.
*
*
* .grafeas.v1.ImageOccurrence image = 10;
*
* @return Whether the image field is set.
*/
@java.lang.Override
public boolean hasImage() {
return detailsCase_ == 10;
}
/**
*
*
*
* Describes how this resource derives from the basis in the associated
* note.
*
*
* .grafeas.v1.ImageOccurrence image = 10;
*
* @return The image.
*/
@java.lang.Override
public io.grafeas.v1.ImageOccurrence getImage() {
if (imageBuilder_ == null) {
if (detailsCase_ == 10) {
return (io.grafeas.v1.ImageOccurrence) details_;
}
return io.grafeas.v1.ImageOccurrence.getDefaultInstance();
} else {
if (detailsCase_ == 10) {
return imageBuilder_.getMessage();
}
return io.grafeas.v1.ImageOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes how this resource derives from the basis in the associated
* note.
*
*
* .grafeas.v1.ImageOccurrence image = 10;
*/
public Builder setImage(io.grafeas.v1.ImageOccurrence value) {
if (imageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
details_ = value;
onChanged();
} else {
imageBuilder_.setMessage(value);
}
detailsCase_ = 10;
return this;
}
/**
*
*
*
* Describes how this resource derives from the basis in the associated
* note.
*
*
* .grafeas.v1.ImageOccurrence image = 10;
*/
public Builder setImage(io.grafeas.v1.ImageOccurrence.Builder builderForValue) {
if (imageBuilder_ == null) {
details_ = builderForValue.build();
onChanged();
} else {
imageBuilder_.setMessage(builderForValue.build());
}
detailsCase_ = 10;
return this;
}
/**
*
*
*
* Describes how this resource derives from the basis in the associated
* note.
*
*
* .grafeas.v1.ImageOccurrence image = 10;
*/
public Builder mergeImage(io.grafeas.v1.ImageOccurrence value) {
if (imageBuilder_ == null) {
if (detailsCase_ == 10 && details_ != io.grafeas.v1.ImageOccurrence.getDefaultInstance()) {
details_ =
io.grafeas.v1.ImageOccurrence.newBuilder((io.grafeas.v1.ImageOccurrence) details_)
.mergeFrom(value)
.buildPartial();
} else {
details_ = value;
}
onChanged();
} else {
if (detailsCase_ == 10) {
imageBuilder_.mergeFrom(value);
} else {
imageBuilder_.setMessage(value);
}
}
detailsCase_ = 10;
return this;
}
/**
*
*
*
* Describes how this resource derives from the basis in the associated
* note.
*
*
* .grafeas.v1.ImageOccurrence image = 10;
*/
public Builder clearImage() {
if (imageBuilder_ == null) {
if (detailsCase_ == 10) {
detailsCase_ = 0;
details_ = null;
onChanged();
}
} else {
if (detailsCase_ == 10) {
detailsCase_ = 0;
details_ = null;
}
imageBuilder_.clear();
}
return this;
}
/**
*
*
*
* Describes how this resource derives from the basis in the associated
* note.
*
*
* .grafeas.v1.ImageOccurrence image = 10;
*/
public io.grafeas.v1.ImageOccurrence.Builder getImageBuilder() {
return getImageFieldBuilder().getBuilder();
}
/**
*
*
*
* Describes how this resource derives from the basis in the associated
* note.
*
*
* .grafeas.v1.ImageOccurrence image = 10;
*/
@java.lang.Override
public io.grafeas.v1.ImageOccurrenceOrBuilder getImageOrBuilder() {
if ((detailsCase_ == 10) && (imageBuilder_ != null)) {
return imageBuilder_.getMessageOrBuilder();
} else {
if (detailsCase_ == 10) {
return (io.grafeas.v1.ImageOccurrence) details_;
}
return io.grafeas.v1.ImageOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes how this resource derives from the basis in the associated
* note.
*
*
* .grafeas.v1.ImageOccurrence image = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.ImageOccurrence,
io.grafeas.v1.ImageOccurrence.Builder,
io.grafeas.v1.ImageOccurrenceOrBuilder>
getImageFieldBuilder() {
if (imageBuilder_ == null) {
if (!(detailsCase_ == 10)) {
details_ = io.grafeas.v1.ImageOccurrence.getDefaultInstance();
}
imageBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.ImageOccurrence,
io.grafeas.v1.ImageOccurrence.Builder,
io.grafeas.v1.ImageOccurrenceOrBuilder>(
(io.grafeas.v1.ImageOccurrence) details_, getParentForChildren(), isClean());
details_ = null;
}
detailsCase_ = 10;
onChanged();
return imageBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.PackageOccurrence,
io.grafeas.v1.PackageOccurrence.Builder,
io.grafeas.v1.PackageOccurrenceOrBuilder>
packageBuilder_;
/**
*
*
*
* Describes the installation of a package on the linked resource.
*
*
* .grafeas.v1.PackageOccurrence package = 11;
*
* @return Whether the package field is set.
*/
@java.lang.Override
public boolean hasPackage() {
return detailsCase_ == 11;
}
/**
*
*
*
* Describes the installation of a package on the linked resource.
*
*
* .grafeas.v1.PackageOccurrence package = 11;
*
* @return The package.
*/
@java.lang.Override
public io.grafeas.v1.PackageOccurrence getPackage() {
if (packageBuilder_ == null) {
if (detailsCase_ == 11) {
return (io.grafeas.v1.PackageOccurrence) details_;
}
return io.grafeas.v1.PackageOccurrence.getDefaultInstance();
} else {
if (detailsCase_ == 11) {
return packageBuilder_.getMessage();
}
return io.grafeas.v1.PackageOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes the installation of a package on the linked resource.
*
*
* .grafeas.v1.PackageOccurrence package = 11;
*/
public Builder setPackage(io.grafeas.v1.PackageOccurrence value) {
if (packageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
details_ = value;
onChanged();
} else {
packageBuilder_.setMessage(value);
}
detailsCase_ = 11;
return this;
}
/**
*
*
*
* Describes the installation of a package on the linked resource.
*
*
* .grafeas.v1.PackageOccurrence package = 11;
*/
public Builder setPackage(io.grafeas.v1.PackageOccurrence.Builder builderForValue) {
if (packageBuilder_ == null) {
details_ = builderForValue.build();
onChanged();
} else {
packageBuilder_.setMessage(builderForValue.build());
}
detailsCase_ = 11;
return this;
}
/**
*
*
*
* Describes the installation of a package on the linked resource.
*
*
* .grafeas.v1.PackageOccurrence package = 11;
*/
public Builder mergePackage(io.grafeas.v1.PackageOccurrence value) {
if (packageBuilder_ == null) {
if (detailsCase_ == 11
&& details_ != io.grafeas.v1.PackageOccurrence.getDefaultInstance()) {
details_ =
io.grafeas.v1.PackageOccurrence.newBuilder((io.grafeas.v1.PackageOccurrence) details_)
.mergeFrom(value)
.buildPartial();
} else {
details_ = value;
}
onChanged();
} else {
if (detailsCase_ == 11) {
packageBuilder_.mergeFrom(value);
} else {
packageBuilder_.setMessage(value);
}
}
detailsCase_ = 11;
return this;
}
/**
*
*
*
* Describes the installation of a package on the linked resource.
*
*
* .grafeas.v1.PackageOccurrence package = 11;
*/
public Builder clearPackage() {
if (packageBuilder_ == null) {
if (detailsCase_ == 11) {
detailsCase_ = 0;
details_ = null;
onChanged();
}
} else {
if (detailsCase_ == 11) {
detailsCase_ = 0;
details_ = null;
}
packageBuilder_.clear();
}
return this;
}
/**
*
*
*
* Describes the installation of a package on the linked resource.
*
*
* .grafeas.v1.PackageOccurrence package = 11;
*/
public io.grafeas.v1.PackageOccurrence.Builder getPackageBuilder() {
return getPackageFieldBuilder().getBuilder();
}
/**
*
*
*
* Describes the installation of a package on the linked resource.
*
*
* .grafeas.v1.PackageOccurrence package = 11;
*/
@java.lang.Override
public io.grafeas.v1.PackageOccurrenceOrBuilder getPackageOrBuilder() {
if ((detailsCase_ == 11) && (packageBuilder_ != null)) {
return packageBuilder_.getMessageOrBuilder();
} else {
if (detailsCase_ == 11) {
return (io.grafeas.v1.PackageOccurrence) details_;
}
return io.grafeas.v1.PackageOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes the installation of a package on the linked resource.
*
*
* .grafeas.v1.PackageOccurrence package = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.PackageOccurrence,
io.grafeas.v1.PackageOccurrence.Builder,
io.grafeas.v1.PackageOccurrenceOrBuilder>
getPackageFieldBuilder() {
if (packageBuilder_ == null) {
if (!(detailsCase_ == 11)) {
details_ = io.grafeas.v1.PackageOccurrence.getDefaultInstance();
}
packageBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.PackageOccurrence,
io.grafeas.v1.PackageOccurrence.Builder,
io.grafeas.v1.PackageOccurrenceOrBuilder>(
(io.grafeas.v1.PackageOccurrence) details_, getParentForChildren(), isClean());
details_ = null;
}
detailsCase_ = 11;
onChanged();
return packageBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.DeploymentOccurrence,
io.grafeas.v1.DeploymentOccurrence.Builder,
io.grafeas.v1.DeploymentOccurrenceOrBuilder>
deploymentBuilder_;
/**
*
*
*
* Describes the deployment of an artifact on a runtime.
*
*
* .grafeas.v1.DeploymentOccurrence deployment = 12;
*
* @return Whether the deployment field is set.
*/
@java.lang.Override
public boolean hasDeployment() {
return detailsCase_ == 12;
}
/**
*
*
*
* Describes the deployment of an artifact on a runtime.
*
*
* .grafeas.v1.DeploymentOccurrence deployment = 12;
*
* @return The deployment.
*/
@java.lang.Override
public io.grafeas.v1.DeploymentOccurrence getDeployment() {
if (deploymentBuilder_ == null) {
if (detailsCase_ == 12) {
return (io.grafeas.v1.DeploymentOccurrence) details_;
}
return io.grafeas.v1.DeploymentOccurrence.getDefaultInstance();
} else {
if (detailsCase_ == 12) {
return deploymentBuilder_.getMessage();
}
return io.grafeas.v1.DeploymentOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes the deployment of an artifact on a runtime.
*
*
* .grafeas.v1.DeploymentOccurrence deployment = 12;
*/
public Builder setDeployment(io.grafeas.v1.DeploymentOccurrence value) {
if (deploymentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
details_ = value;
onChanged();
} else {
deploymentBuilder_.setMessage(value);
}
detailsCase_ = 12;
return this;
}
/**
*
*
*
* Describes the deployment of an artifact on a runtime.
*
*
* .grafeas.v1.DeploymentOccurrence deployment = 12;
*/
public Builder setDeployment(io.grafeas.v1.DeploymentOccurrence.Builder builderForValue) {
if (deploymentBuilder_ == null) {
details_ = builderForValue.build();
onChanged();
} else {
deploymentBuilder_.setMessage(builderForValue.build());
}
detailsCase_ = 12;
return this;
}
/**
*
*
*
* Describes the deployment of an artifact on a runtime.
*
*
* .grafeas.v1.DeploymentOccurrence deployment = 12;
*/
public Builder mergeDeployment(io.grafeas.v1.DeploymentOccurrence value) {
if (deploymentBuilder_ == null) {
if (detailsCase_ == 12
&& details_ != io.grafeas.v1.DeploymentOccurrence.getDefaultInstance()) {
details_ =
io.grafeas.v1.DeploymentOccurrence.newBuilder(
(io.grafeas.v1.DeploymentOccurrence) details_)
.mergeFrom(value)
.buildPartial();
} else {
details_ = value;
}
onChanged();
} else {
if (detailsCase_ == 12) {
deploymentBuilder_.mergeFrom(value);
} else {
deploymentBuilder_.setMessage(value);
}
}
detailsCase_ = 12;
return this;
}
/**
*
*
*
* Describes the deployment of an artifact on a runtime.
*
*
* .grafeas.v1.DeploymentOccurrence deployment = 12;
*/
public Builder clearDeployment() {
if (deploymentBuilder_ == null) {
if (detailsCase_ == 12) {
detailsCase_ = 0;
details_ = null;
onChanged();
}
} else {
if (detailsCase_ == 12) {
detailsCase_ = 0;
details_ = null;
}
deploymentBuilder_.clear();
}
return this;
}
/**
*
*
*
* Describes the deployment of an artifact on a runtime.
*
*
* .grafeas.v1.DeploymentOccurrence deployment = 12;
*/
public io.grafeas.v1.DeploymentOccurrence.Builder getDeploymentBuilder() {
return getDeploymentFieldBuilder().getBuilder();
}
/**
*
*
*
* Describes the deployment of an artifact on a runtime.
*
*
* .grafeas.v1.DeploymentOccurrence deployment = 12;
*/
@java.lang.Override
public io.grafeas.v1.DeploymentOccurrenceOrBuilder getDeploymentOrBuilder() {
if ((detailsCase_ == 12) && (deploymentBuilder_ != null)) {
return deploymentBuilder_.getMessageOrBuilder();
} else {
if (detailsCase_ == 12) {
return (io.grafeas.v1.DeploymentOccurrence) details_;
}
return io.grafeas.v1.DeploymentOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes the deployment of an artifact on a runtime.
*
*
* .grafeas.v1.DeploymentOccurrence deployment = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.DeploymentOccurrence,
io.grafeas.v1.DeploymentOccurrence.Builder,
io.grafeas.v1.DeploymentOccurrenceOrBuilder>
getDeploymentFieldBuilder() {
if (deploymentBuilder_ == null) {
if (!(detailsCase_ == 12)) {
details_ = io.grafeas.v1.DeploymentOccurrence.getDefaultInstance();
}
deploymentBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.DeploymentOccurrence,
io.grafeas.v1.DeploymentOccurrence.Builder,
io.grafeas.v1.DeploymentOccurrenceOrBuilder>(
(io.grafeas.v1.DeploymentOccurrence) details_, getParentForChildren(), isClean());
details_ = null;
}
detailsCase_ = 12;
onChanged();
return deploymentBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.DiscoveryOccurrence,
io.grafeas.v1.DiscoveryOccurrence.Builder,
io.grafeas.v1.DiscoveryOccurrenceOrBuilder>
discoveryBuilder_;
/**
*
*
*
* Describes when a resource was discovered.
*
*
* .grafeas.v1.DiscoveryOccurrence discovery = 13;
*
* @return Whether the discovery field is set.
*/
@java.lang.Override
public boolean hasDiscovery() {
return detailsCase_ == 13;
}
/**
*
*
*
* Describes when a resource was discovered.
*
*
* .grafeas.v1.DiscoveryOccurrence discovery = 13;
*
* @return The discovery.
*/
@java.lang.Override
public io.grafeas.v1.DiscoveryOccurrence getDiscovery() {
if (discoveryBuilder_ == null) {
if (detailsCase_ == 13) {
return (io.grafeas.v1.DiscoveryOccurrence) details_;
}
return io.grafeas.v1.DiscoveryOccurrence.getDefaultInstance();
} else {
if (detailsCase_ == 13) {
return discoveryBuilder_.getMessage();
}
return io.grafeas.v1.DiscoveryOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes when a resource was discovered.
*
*
* .grafeas.v1.DiscoveryOccurrence discovery = 13;
*/
public Builder setDiscovery(io.grafeas.v1.DiscoveryOccurrence value) {
if (discoveryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
details_ = value;
onChanged();
} else {
discoveryBuilder_.setMessage(value);
}
detailsCase_ = 13;
return this;
}
/**
*
*
*
* Describes when a resource was discovered.
*
*
* .grafeas.v1.DiscoveryOccurrence discovery = 13;
*/
public Builder setDiscovery(io.grafeas.v1.DiscoveryOccurrence.Builder builderForValue) {
if (discoveryBuilder_ == null) {
details_ = builderForValue.build();
onChanged();
} else {
discoveryBuilder_.setMessage(builderForValue.build());
}
detailsCase_ = 13;
return this;
}
/**
*
*
*
* Describes when a resource was discovered.
*
*
* .grafeas.v1.DiscoveryOccurrence discovery = 13;
*/
public Builder mergeDiscovery(io.grafeas.v1.DiscoveryOccurrence value) {
if (discoveryBuilder_ == null) {
if (detailsCase_ == 13
&& details_ != io.grafeas.v1.DiscoveryOccurrence.getDefaultInstance()) {
details_ =
io.grafeas.v1.DiscoveryOccurrence.newBuilder(
(io.grafeas.v1.DiscoveryOccurrence) details_)
.mergeFrom(value)
.buildPartial();
} else {
details_ = value;
}
onChanged();
} else {
if (detailsCase_ == 13) {
discoveryBuilder_.mergeFrom(value);
} else {
discoveryBuilder_.setMessage(value);
}
}
detailsCase_ = 13;
return this;
}
/**
*
*
*
* Describes when a resource was discovered.
*
*
* .grafeas.v1.DiscoveryOccurrence discovery = 13;
*/
public Builder clearDiscovery() {
if (discoveryBuilder_ == null) {
if (detailsCase_ == 13) {
detailsCase_ = 0;
details_ = null;
onChanged();
}
} else {
if (detailsCase_ == 13) {
detailsCase_ = 0;
details_ = null;
}
discoveryBuilder_.clear();
}
return this;
}
/**
*
*
*
* Describes when a resource was discovered.
*
*
* .grafeas.v1.DiscoveryOccurrence discovery = 13;
*/
public io.grafeas.v1.DiscoveryOccurrence.Builder getDiscoveryBuilder() {
return getDiscoveryFieldBuilder().getBuilder();
}
/**
*
*
*
* Describes when a resource was discovered.
*
*
* .grafeas.v1.DiscoveryOccurrence discovery = 13;
*/
@java.lang.Override
public io.grafeas.v1.DiscoveryOccurrenceOrBuilder getDiscoveryOrBuilder() {
if ((detailsCase_ == 13) && (discoveryBuilder_ != null)) {
return discoveryBuilder_.getMessageOrBuilder();
} else {
if (detailsCase_ == 13) {
return (io.grafeas.v1.DiscoveryOccurrence) details_;
}
return io.grafeas.v1.DiscoveryOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes when a resource was discovered.
*
*
* .grafeas.v1.DiscoveryOccurrence discovery = 13;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.DiscoveryOccurrence,
io.grafeas.v1.DiscoveryOccurrence.Builder,
io.grafeas.v1.DiscoveryOccurrenceOrBuilder>
getDiscoveryFieldBuilder() {
if (discoveryBuilder_ == null) {
if (!(detailsCase_ == 13)) {
details_ = io.grafeas.v1.DiscoveryOccurrence.getDefaultInstance();
}
discoveryBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.DiscoveryOccurrence,
io.grafeas.v1.DiscoveryOccurrence.Builder,
io.grafeas.v1.DiscoveryOccurrenceOrBuilder>(
(io.grafeas.v1.DiscoveryOccurrence) details_, getParentForChildren(), isClean());
details_ = null;
}
detailsCase_ = 13;
onChanged();
return discoveryBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.AttestationOccurrence,
io.grafeas.v1.AttestationOccurrence.Builder,
io.grafeas.v1.AttestationOccurrenceOrBuilder>
attestationBuilder_;
/**
*
*
*
* Describes an attestation of an artifact.
*
*
* .grafeas.v1.AttestationOccurrence attestation = 14;
*
* @return Whether the attestation field is set.
*/
@java.lang.Override
public boolean hasAttestation() {
return detailsCase_ == 14;
}
/**
*
*
*
* Describes an attestation of an artifact.
*
*
* .grafeas.v1.AttestationOccurrence attestation = 14;
*
* @return The attestation.
*/
@java.lang.Override
public io.grafeas.v1.AttestationOccurrence getAttestation() {
if (attestationBuilder_ == null) {
if (detailsCase_ == 14) {
return (io.grafeas.v1.AttestationOccurrence) details_;
}
return io.grafeas.v1.AttestationOccurrence.getDefaultInstance();
} else {
if (detailsCase_ == 14) {
return attestationBuilder_.getMessage();
}
return io.grafeas.v1.AttestationOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes an attestation of an artifact.
*
*
* .grafeas.v1.AttestationOccurrence attestation = 14;
*/
public Builder setAttestation(io.grafeas.v1.AttestationOccurrence value) {
if (attestationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
details_ = value;
onChanged();
} else {
attestationBuilder_.setMessage(value);
}
detailsCase_ = 14;
return this;
}
/**
*
*
*
* Describes an attestation of an artifact.
*
*
* .grafeas.v1.AttestationOccurrence attestation = 14;
*/
public Builder setAttestation(io.grafeas.v1.AttestationOccurrence.Builder builderForValue) {
if (attestationBuilder_ == null) {
details_ = builderForValue.build();
onChanged();
} else {
attestationBuilder_.setMessage(builderForValue.build());
}
detailsCase_ = 14;
return this;
}
/**
*
*
*
* Describes an attestation of an artifact.
*
*
* .grafeas.v1.AttestationOccurrence attestation = 14;
*/
public Builder mergeAttestation(io.grafeas.v1.AttestationOccurrence value) {
if (attestationBuilder_ == null) {
if (detailsCase_ == 14
&& details_ != io.grafeas.v1.AttestationOccurrence.getDefaultInstance()) {
details_ =
io.grafeas.v1.AttestationOccurrence.newBuilder(
(io.grafeas.v1.AttestationOccurrence) details_)
.mergeFrom(value)
.buildPartial();
} else {
details_ = value;
}
onChanged();
} else {
if (detailsCase_ == 14) {
attestationBuilder_.mergeFrom(value);
} else {
attestationBuilder_.setMessage(value);
}
}
detailsCase_ = 14;
return this;
}
/**
*
*
*
* Describes an attestation of an artifact.
*
*
* .grafeas.v1.AttestationOccurrence attestation = 14;
*/
public Builder clearAttestation() {
if (attestationBuilder_ == null) {
if (detailsCase_ == 14) {
detailsCase_ = 0;
details_ = null;
onChanged();
}
} else {
if (detailsCase_ == 14) {
detailsCase_ = 0;
details_ = null;
}
attestationBuilder_.clear();
}
return this;
}
/**
*
*
*
* Describes an attestation of an artifact.
*
*
* .grafeas.v1.AttestationOccurrence attestation = 14;
*/
public io.grafeas.v1.AttestationOccurrence.Builder getAttestationBuilder() {
return getAttestationFieldBuilder().getBuilder();
}
/**
*
*
*
* Describes an attestation of an artifact.
*
*
* .grafeas.v1.AttestationOccurrence attestation = 14;
*/
@java.lang.Override
public io.grafeas.v1.AttestationOccurrenceOrBuilder getAttestationOrBuilder() {
if ((detailsCase_ == 14) && (attestationBuilder_ != null)) {
return attestationBuilder_.getMessageOrBuilder();
} else {
if (detailsCase_ == 14) {
return (io.grafeas.v1.AttestationOccurrence) details_;
}
return io.grafeas.v1.AttestationOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes an attestation of an artifact.
*
*
* .grafeas.v1.AttestationOccurrence attestation = 14;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.AttestationOccurrence,
io.grafeas.v1.AttestationOccurrence.Builder,
io.grafeas.v1.AttestationOccurrenceOrBuilder>
getAttestationFieldBuilder() {
if (attestationBuilder_ == null) {
if (!(detailsCase_ == 14)) {
details_ = io.grafeas.v1.AttestationOccurrence.getDefaultInstance();
}
attestationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.AttestationOccurrence,
io.grafeas.v1.AttestationOccurrence.Builder,
io.grafeas.v1.AttestationOccurrenceOrBuilder>(
(io.grafeas.v1.AttestationOccurrence) details_, getParentForChildren(), isClean());
details_ = null;
}
detailsCase_ = 14;
onChanged();
return attestationBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.UpgradeOccurrence,
io.grafeas.v1.UpgradeOccurrence.Builder,
io.grafeas.v1.UpgradeOccurrenceOrBuilder>
upgradeBuilder_;
/**
*
*
*
* Describes an available package upgrade on the linked resource.
*
*
* .grafeas.v1.UpgradeOccurrence upgrade = 15;
*
* @return Whether the upgrade field is set.
*/
@java.lang.Override
public boolean hasUpgrade() {
return detailsCase_ == 15;
}
/**
*
*
*
* Describes an available package upgrade on the linked resource.
*
*
* .grafeas.v1.UpgradeOccurrence upgrade = 15;
*
* @return The upgrade.
*/
@java.lang.Override
public io.grafeas.v1.UpgradeOccurrence getUpgrade() {
if (upgradeBuilder_ == null) {
if (detailsCase_ == 15) {
return (io.grafeas.v1.UpgradeOccurrence) details_;
}
return io.grafeas.v1.UpgradeOccurrence.getDefaultInstance();
} else {
if (detailsCase_ == 15) {
return upgradeBuilder_.getMessage();
}
return io.grafeas.v1.UpgradeOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes an available package upgrade on the linked resource.
*
*
* .grafeas.v1.UpgradeOccurrence upgrade = 15;
*/
public Builder setUpgrade(io.grafeas.v1.UpgradeOccurrence value) {
if (upgradeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
details_ = value;
onChanged();
} else {
upgradeBuilder_.setMessage(value);
}
detailsCase_ = 15;
return this;
}
/**
*
*
*
* Describes an available package upgrade on the linked resource.
*
*
* .grafeas.v1.UpgradeOccurrence upgrade = 15;
*/
public Builder setUpgrade(io.grafeas.v1.UpgradeOccurrence.Builder builderForValue) {
if (upgradeBuilder_ == null) {
details_ = builderForValue.build();
onChanged();
} else {
upgradeBuilder_.setMessage(builderForValue.build());
}
detailsCase_ = 15;
return this;
}
/**
*
*
*
* Describes an available package upgrade on the linked resource.
*
*
* .grafeas.v1.UpgradeOccurrence upgrade = 15;
*/
public Builder mergeUpgrade(io.grafeas.v1.UpgradeOccurrence value) {
if (upgradeBuilder_ == null) {
if (detailsCase_ == 15
&& details_ != io.grafeas.v1.UpgradeOccurrence.getDefaultInstance()) {
details_ =
io.grafeas.v1.UpgradeOccurrence.newBuilder((io.grafeas.v1.UpgradeOccurrence) details_)
.mergeFrom(value)
.buildPartial();
} else {
details_ = value;
}
onChanged();
} else {
if (detailsCase_ == 15) {
upgradeBuilder_.mergeFrom(value);
} else {
upgradeBuilder_.setMessage(value);
}
}
detailsCase_ = 15;
return this;
}
/**
*
*
*
* Describes an available package upgrade on the linked resource.
*
*
* .grafeas.v1.UpgradeOccurrence upgrade = 15;
*/
public Builder clearUpgrade() {
if (upgradeBuilder_ == null) {
if (detailsCase_ == 15) {
detailsCase_ = 0;
details_ = null;
onChanged();
}
} else {
if (detailsCase_ == 15) {
detailsCase_ = 0;
details_ = null;
}
upgradeBuilder_.clear();
}
return this;
}
/**
*
*
*
* Describes an available package upgrade on the linked resource.
*
*
* .grafeas.v1.UpgradeOccurrence upgrade = 15;
*/
public io.grafeas.v1.UpgradeOccurrence.Builder getUpgradeBuilder() {
return getUpgradeFieldBuilder().getBuilder();
}
/**
*
*
*
* Describes an available package upgrade on the linked resource.
*
*
* .grafeas.v1.UpgradeOccurrence upgrade = 15;
*/
@java.lang.Override
public io.grafeas.v1.UpgradeOccurrenceOrBuilder getUpgradeOrBuilder() {
if ((detailsCase_ == 15) && (upgradeBuilder_ != null)) {
return upgradeBuilder_.getMessageOrBuilder();
} else {
if (detailsCase_ == 15) {
return (io.grafeas.v1.UpgradeOccurrence) details_;
}
return io.grafeas.v1.UpgradeOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes an available package upgrade on the linked resource.
*
*
* .grafeas.v1.UpgradeOccurrence upgrade = 15;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.UpgradeOccurrence,
io.grafeas.v1.UpgradeOccurrence.Builder,
io.grafeas.v1.UpgradeOccurrenceOrBuilder>
getUpgradeFieldBuilder() {
if (upgradeBuilder_ == null) {
if (!(detailsCase_ == 15)) {
details_ = io.grafeas.v1.UpgradeOccurrence.getDefaultInstance();
}
upgradeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.UpgradeOccurrence,
io.grafeas.v1.UpgradeOccurrence.Builder,
io.grafeas.v1.UpgradeOccurrenceOrBuilder>(
(io.grafeas.v1.UpgradeOccurrence) details_, getParentForChildren(), isClean());
details_ = null;
}
detailsCase_ = 15;
onChanged();
return upgradeBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.ComplianceOccurrence,
io.grafeas.v1.ComplianceOccurrence.Builder,
io.grafeas.v1.ComplianceOccurrenceOrBuilder>
complianceBuilder_;
/**
*
*
*
* Describes a compliance violation on a linked resource.
*
*
* .grafeas.v1.ComplianceOccurrence compliance = 16;
*
* @return Whether the compliance field is set.
*/
@java.lang.Override
public boolean hasCompliance() {
return detailsCase_ == 16;
}
/**
*
*
*
* Describes a compliance violation on a linked resource.
*
*
* .grafeas.v1.ComplianceOccurrence compliance = 16;
*
* @return The compliance.
*/
@java.lang.Override
public io.grafeas.v1.ComplianceOccurrence getCompliance() {
if (complianceBuilder_ == null) {
if (detailsCase_ == 16) {
return (io.grafeas.v1.ComplianceOccurrence) details_;
}
return io.grafeas.v1.ComplianceOccurrence.getDefaultInstance();
} else {
if (detailsCase_ == 16) {
return complianceBuilder_.getMessage();
}
return io.grafeas.v1.ComplianceOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes a compliance violation on a linked resource.
*
*
* .grafeas.v1.ComplianceOccurrence compliance = 16;
*/
public Builder setCompliance(io.grafeas.v1.ComplianceOccurrence value) {
if (complianceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
details_ = value;
onChanged();
} else {
complianceBuilder_.setMessage(value);
}
detailsCase_ = 16;
return this;
}
/**
*
*
*
* Describes a compliance violation on a linked resource.
*
*
* .grafeas.v1.ComplianceOccurrence compliance = 16;
*/
public Builder setCompliance(io.grafeas.v1.ComplianceOccurrence.Builder builderForValue) {
if (complianceBuilder_ == null) {
details_ = builderForValue.build();
onChanged();
} else {
complianceBuilder_.setMessage(builderForValue.build());
}
detailsCase_ = 16;
return this;
}
/**
*
*
*
* Describes a compliance violation on a linked resource.
*
*
* .grafeas.v1.ComplianceOccurrence compliance = 16;
*/
public Builder mergeCompliance(io.grafeas.v1.ComplianceOccurrence value) {
if (complianceBuilder_ == null) {
if (detailsCase_ == 16
&& details_ != io.grafeas.v1.ComplianceOccurrence.getDefaultInstance()) {
details_ =
io.grafeas.v1.ComplianceOccurrence.newBuilder(
(io.grafeas.v1.ComplianceOccurrence) details_)
.mergeFrom(value)
.buildPartial();
} else {
details_ = value;
}
onChanged();
} else {
if (detailsCase_ == 16) {
complianceBuilder_.mergeFrom(value);
} else {
complianceBuilder_.setMessage(value);
}
}
detailsCase_ = 16;
return this;
}
/**
*
*
*
* Describes a compliance violation on a linked resource.
*
*
* .grafeas.v1.ComplianceOccurrence compliance = 16;
*/
public Builder clearCompliance() {
if (complianceBuilder_ == null) {
if (detailsCase_ == 16) {
detailsCase_ = 0;
details_ = null;
onChanged();
}
} else {
if (detailsCase_ == 16) {
detailsCase_ = 0;
details_ = null;
}
complianceBuilder_.clear();
}
return this;
}
/**
*
*
*
* Describes a compliance violation on a linked resource.
*
*
* .grafeas.v1.ComplianceOccurrence compliance = 16;
*/
public io.grafeas.v1.ComplianceOccurrence.Builder getComplianceBuilder() {
return getComplianceFieldBuilder().getBuilder();
}
/**
*
*
*
* Describes a compliance violation on a linked resource.
*
*
* .grafeas.v1.ComplianceOccurrence compliance = 16;
*/
@java.lang.Override
public io.grafeas.v1.ComplianceOccurrenceOrBuilder getComplianceOrBuilder() {
if ((detailsCase_ == 16) && (complianceBuilder_ != null)) {
return complianceBuilder_.getMessageOrBuilder();
} else {
if (detailsCase_ == 16) {
return (io.grafeas.v1.ComplianceOccurrence) details_;
}
return io.grafeas.v1.ComplianceOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes a compliance violation on a linked resource.
*
*
* .grafeas.v1.ComplianceOccurrence compliance = 16;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.ComplianceOccurrence,
io.grafeas.v1.ComplianceOccurrence.Builder,
io.grafeas.v1.ComplianceOccurrenceOrBuilder>
getComplianceFieldBuilder() {
if (complianceBuilder_ == null) {
if (!(detailsCase_ == 16)) {
details_ = io.grafeas.v1.ComplianceOccurrence.getDefaultInstance();
}
complianceBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.ComplianceOccurrence,
io.grafeas.v1.ComplianceOccurrence.Builder,
io.grafeas.v1.ComplianceOccurrenceOrBuilder>(
(io.grafeas.v1.ComplianceOccurrence) details_, getParentForChildren(), isClean());
details_ = null;
}
detailsCase_ = 16;
onChanged();
return complianceBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.DSSEAttestationOccurrence,
io.grafeas.v1.DSSEAttestationOccurrence.Builder,
io.grafeas.v1.DSSEAttestationOccurrenceOrBuilder>
dsseAttestationBuilder_;
/**
*
*
*
* Describes an attestation of an artifact using dsse.
*
*
* .grafeas.v1.DSSEAttestationOccurrence dsse_attestation = 17;
*
* @return Whether the dsseAttestation field is set.
*/
@java.lang.Override
public boolean hasDsseAttestation() {
return detailsCase_ == 17;
}
/**
*
*
*
* Describes an attestation of an artifact using dsse.
*
*
* .grafeas.v1.DSSEAttestationOccurrence dsse_attestation = 17;
*
* @return The dsseAttestation.
*/
@java.lang.Override
public io.grafeas.v1.DSSEAttestationOccurrence getDsseAttestation() {
if (dsseAttestationBuilder_ == null) {
if (detailsCase_ == 17) {
return (io.grafeas.v1.DSSEAttestationOccurrence) details_;
}
return io.grafeas.v1.DSSEAttestationOccurrence.getDefaultInstance();
} else {
if (detailsCase_ == 17) {
return dsseAttestationBuilder_.getMessage();
}
return io.grafeas.v1.DSSEAttestationOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes an attestation of an artifact using dsse.
*
*
* .grafeas.v1.DSSEAttestationOccurrence dsse_attestation = 17;
*/
public Builder setDsseAttestation(io.grafeas.v1.DSSEAttestationOccurrence value) {
if (dsseAttestationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
details_ = value;
onChanged();
} else {
dsseAttestationBuilder_.setMessage(value);
}
detailsCase_ = 17;
return this;
}
/**
*
*
*
* Describes an attestation of an artifact using dsse.
*
*
* .grafeas.v1.DSSEAttestationOccurrence dsse_attestation = 17;
*/
public Builder setDsseAttestation(
io.grafeas.v1.DSSEAttestationOccurrence.Builder builderForValue) {
if (dsseAttestationBuilder_ == null) {
details_ = builderForValue.build();
onChanged();
} else {
dsseAttestationBuilder_.setMessage(builderForValue.build());
}
detailsCase_ = 17;
return this;
}
/**
*
*
*
* Describes an attestation of an artifact using dsse.
*
*
* .grafeas.v1.DSSEAttestationOccurrence dsse_attestation = 17;
*/
public Builder mergeDsseAttestation(io.grafeas.v1.DSSEAttestationOccurrence value) {
if (dsseAttestationBuilder_ == null) {
if (detailsCase_ == 17
&& details_ != io.grafeas.v1.DSSEAttestationOccurrence.getDefaultInstance()) {
details_ =
io.grafeas.v1.DSSEAttestationOccurrence.newBuilder(
(io.grafeas.v1.DSSEAttestationOccurrence) details_)
.mergeFrom(value)
.buildPartial();
} else {
details_ = value;
}
onChanged();
} else {
if (detailsCase_ == 17) {
dsseAttestationBuilder_.mergeFrom(value);
} else {
dsseAttestationBuilder_.setMessage(value);
}
}
detailsCase_ = 17;
return this;
}
/**
*
*
*
* Describes an attestation of an artifact using dsse.
*
*
* .grafeas.v1.DSSEAttestationOccurrence dsse_attestation = 17;
*/
public Builder clearDsseAttestation() {
if (dsseAttestationBuilder_ == null) {
if (detailsCase_ == 17) {
detailsCase_ = 0;
details_ = null;
onChanged();
}
} else {
if (detailsCase_ == 17) {
detailsCase_ = 0;
details_ = null;
}
dsseAttestationBuilder_.clear();
}
return this;
}
/**
*
*
*
* Describes an attestation of an artifact using dsse.
*
*
* .grafeas.v1.DSSEAttestationOccurrence dsse_attestation = 17;
*/
public io.grafeas.v1.DSSEAttestationOccurrence.Builder getDsseAttestationBuilder() {
return getDsseAttestationFieldBuilder().getBuilder();
}
/**
*
*
*
* Describes an attestation of an artifact using dsse.
*
*
* .grafeas.v1.DSSEAttestationOccurrence dsse_attestation = 17;
*/
@java.lang.Override
public io.grafeas.v1.DSSEAttestationOccurrenceOrBuilder getDsseAttestationOrBuilder() {
if ((detailsCase_ == 17) && (dsseAttestationBuilder_ != null)) {
return dsseAttestationBuilder_.getMessageOrBuilder();
} else {
if (detailsCase_ == 17) {
return (io.grafeas.v1.DSSEAttestationOccurrence) details_;
}
return io.grafeas.v1.DSSEAttestationOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes an attestation of an artifact using dsse.
*
*
* .grafeas.v1.DSSEAttestationOccurrence dsse_attestation = 17;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.DSSEAttestationOccurrence,
io.grafeas.v1.DSSEAttestationOccurrence.Builder,
io.grafeas.v1.DSSEAttestationOccurrenceOrBuilder>
getDsseAttestationFieldBuilder() {
if (dsseAttestationBuilder_ == null) {
if (!(detailsCase_ == 17)) {
details_ = io.grafeas.v1.DSSEAttestationOccurrence.getDefaultInstance();
}
dsseAttestationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.DSSEAttestationOccurrence,
io.grafeas.v1.DSSEAttestationOccurrence.Builder,
io.grafeas.v1.DSSEAttestationOccurrenceOrBuilder>(
(io.grafeas.v1.DSSEAttestationOccurrence) details_,
getParentForChildren(),
isClean());
details_ = null;
}
detailsCase_ = 17;
onChanged();
return dsseAttestationBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.SBOMReferenceOccurrence,
io.grafeas.v1.SBOMReferenceOccurrence.Builder,
io.grafeas.v1.SBOMReferenceOccurrenceOrBuilder>
sbomReferenceBuilder_;
/**
*
*
*
* Describes a specific SBOM reference occurrences.
*
*
* .grafeas.v1.SBOMReferenceOccurrence sbom_reference = 19;
*
* @return Whether the sbomReference field is set.
*/
@java.lang.Override
public boolean hasSbomReference() {
return detailsCase_ == 19;
}
/**
*
*
*
* Describes a specific SBOM reference occurrences.
*
*
* .grafeas.v1.SBOMReferenceOccurrence sbom_reference = 19;
*
* @return The sbomReference.
*/
@java.lang.Override
public io.grafeas.v1.SBOMReferenceOccurrence getSbomReference() {
if (sbomReferenceBuilder_ == null) {
if (detailsCase_ == 19) {
return (io.grafeas.v1.SBOMReferenceOccurrence) details_;
}
return io.grafeas.v1.SBOMReferenceOccurrence.getDefaultInstance();
} else {
if (detailsCase_ == 19) {
return sbomReferenceBuilder_.getMessage();
}
return io.grafeas.v1.SBOMReferenceOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes a specific SBOM reference occurrences.
*
*
* .grafeas.v1.SBOMReferenceOccurrence sbom_reference = 19;
*/
public Builder setSbomReference(io.grafeas.v1.SBOMReferenceOccurrence value) {
if (sbomReferenceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
details_ = value;
onChanged();
} else {
sbomReferenceBuilder_.setMessage(value);
}
detailsCase_ = 19;
return this;
}
/**
*
*
*
* Describes a specific SBOM reference occurrences.
*
*
* .grafeas.v1.SBOMReferenceOccurrence sbom_reference = 19;
*/
public Builder setSbomReference(io.grafeas.v1.SBOMReferenceOccurrence.Builder builderForValue) {
if (sbomReferenceBuilder_ == null) {
details_ = builderForValue.build();
onChanged();
} else {
sbomReferenceBuilder_.setMessage(builderForValue.build());
}
detailsCase_ = 19;
return this;
}
/**
*
*
*
* Describes a specific SBOM reference occurrences.
*
*
* .grafeas.v1.SBOMReferenceOccurrence sbom_reference = 19;
*/
public Builder mergeSbomReference(io.grafeas.v1.SBOMReferenceOccurrence value) {
if (sbomReferenceBuilder_ == null) {
if (detailsCase_ == 19
&& details_ != io.grafeas.v1.SBOMReferenceOccurrence.getDefaultInstance()) {
details_ =
io.grafeas.v1.SBOMReferenceOccurrence.newBuilder(
(io.grafeas.v1.SBOMReferenceOccurrence) details_)
.mergeFrom(value)
.buildPartial();
} else {
details_ = value;
}
onChanged();
} else {
if (detailsCase_ == 19) {
sbomReferenceBuilder_.mergeFrom(value);
} else {
sbomReferenceBuilder_.setMessage(value);
}
}
detailsCase_ = 19;
return this;
}
/**
*
*
*
* Describes a specific SBOM reference occurrences.
*
*
* .grafeas.v1.SBOMReferenceOccurrence sbom_reference = 19;
*/
public Builder clearSbomReference() {
if (sbomReferenceBuilder_ == null) {
if (detailsCase_ == 19) {
detailsCase_ = 0;
details_ = null;
onChanged();
}
} else {
if (detailsCase_ == 19) {
detailsCase_ = 0;
details_ = null;
}
sbomReferenceBuilder_.clear();
}
return this;
}
/**
*
*
*
* Describes a specific SBOM reference occurrences.
*
*
* .grafeas.v1.SBOMReferenceOccurrence sbom_reference = 19;
*/
public io.grafeas.v1.SBOMReferenceOccurrence.Builder getSbomReferenceBuilder() {
return getSbomReferenceFieldBuilder().getBuilder();
}
/**
*
*
*
* Describes a specific SBOM reference occurrences.
*
*
* .grafeas.v1.SBOMReferenceOccurrence sbom_reference = 19;
*/
@java.lang.Override
public io.grafeas.v1.SBOMReferenceOccurrenceOrBuilder getSbomReferenceOrBuilder() {
if ((detailsCase_ == 19) && (sbomReferenceBuilder_ != null)) {
return sbomReferenceBuilder_.getMessageOrBuilder();
} else {
if (detailsCase_ == 19) {
return (io.grafeas.v1.SBOMReferenceOccurrence) details_;
}
return io.grafeas.v1.SBOMReferenceOccurrence.getDefaultInstance();
}
}
/**
*
*
*
* Describes a specific SBOM reference occurrences.
*
*
* .grafeas.v1.SBOMReferenceOccurrence sbom_reference = 19;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.SBOMReferenceOccurrence,
io.grafeas.v1.SBOMReferenceOccurrence.Builder,
io.grafeas.v1.SBOMReferenceOccurrenceOrBuilder>
getSbomReferenceFieldBuilder() {
if (sbomReferenceBuilder_ == null) {
if (!(detailsCase_ == 19)) {
details_ = io.grafeas.v1.SBOMReferenceOccurrence.getDefaultInstance();
}
sbomReferenceBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.SBOMReferenceOccurrence,
io.grafeas.v1.SBOMReferenceOccurrence.Builder,
io.grafeas.v1.SBOMReferenceOccurrenceOrBuilder>(
(io.grafeas.v1.SBOMReferenceOccurrence) details_,
getParentForChildren(),
isClean());
details_ = null;
}
detailsCase_ = 19;
onChanged();
return sbomReferenceBuilder_;
}
private io.grafeas.v1.Envelope envelope_;
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.Envelope, io.grafeas.v1.Envelope.Builder, io.grafeas.v1.EnvelopeOrBuilder>
envelopeBuilder_;
/**
*
*
*
* https://github.com/secure-systems-lab/dsse
*
*
* .grafeas.v1.Envelope envelope = 18;
*
* @return Whether the envelope field is set.
*/
public boolean hasEnvelope() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
*
*
*
* https://github.com/secure-systems-lab/dsse
*
*
* .grafeas.v1.Envelope envelope = 18;
*
* @return The envelope.
*/
public io.grafeas.v1.Envelope getEnvelope() {
if (envelopeBuilder_ == null) {
return envelope_ == null ? io.grafeas.v1.Envelope.getDefaultInstance() : envelope_;
} else {
return envelopeBuilder_.getMessage();
}
}
/**
*
*
*
* https://github.com/secure-systems-lab/dsse
*
*
* .grafeas.v1.Envelope envelope = 18;
*/
public Builder setEnvelope(io.grafeas.v1.Envelope value) {
if (envelopeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
envelope_ = value;
} else {
envelopeBuilder_.setMessage(value);
}
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
*
*
*
* https://github.com/secure-systems-lab/dsse
*
*
* .grafeas.v1.Envelope envelope = 18;
*/
public Builder setEnvelope(io.grafeas.v1.Envelope.Builder builderForValue) {
if (envelopeBuilder_ == null) {
envelope_ = builderForValue.build();
} else {
envelopeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
*
*
*
* https://github.com/secure-systems-lab/dsse
*
*
* .grafeas.v1.Envelope envelope = 18;
*/
public Builder mergeEnvelope(io.grafeas.v1.Envelope value) {
if (envelopeBuilder_ == null) {
if (((bitField0_ & 0x00040000) != 0)
&& envelope_ != null
&& envelope_ != io.grafeas.v1.Envelope.getDefaultInstance()) {
getEnvelopeBuilder().mergeFrom(value);
} else {
envelope_ = value;
}
} else {
envelopeBuilder_.mergeFrom(value);
}
if (envelope_ != null) {
bitField0_ |= 0x00040000;
onChanged();
}
return this;
}
/**
*
*
*
* https://github.com/secure-systems-lab/dsse
*
*
* .grafeas.v1.Envelope envelope = 18;
*/
public Builder clearEnvelope() {
bitField0_ = (bitField0_ & ~0x00040000);
envelope_ = null;
if (envelopeBuilder_ != null) {
envelopeBuilder_.dispose();
envelopeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* https://github.com/secure-systems-lab/dsse
*
*
* .grafeas.v1.Envelope envelope = 18;
*/
public io.grafeas.v1.Envelope.Builder getEnvelopeBuilder() {
bitField0_ |= 0x00040000;
onChanged();
return getEnvelopeFieldBuilder().getBuilder();
}
/**
*
*
*
* https://github.com/secure-systems-lab/dsse
*
*
* .grafeas.v1.Envelope envelope = 18;
*/
public io.grafeas.v1.EnvelopeOrBuilder getEnvelopeOrBuilder() {
if (envelopeBuilder_ != null) {
return envelopeBuilder_.getMessageOrBuilder();
} else {
return envelope_ == null ? io.grafeas.v1.Envelope.getDefaultInstance() : envelope_;
}
}
/**
*
*
*
* https://github.com/secure-systems-lab/dsse
*
*
* .grafeas.v1.Envelope envelope = 18;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.Envelope, io.grafeas.v1.Envelope.Builder, io.grafeas.v1.EnvelopeOrBuilder>
getEnvelopeFieldBuilder() {
if (envelopeBuilder_ == null) {
envelopeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
io.grafeas.v1.Envelope,
io.grafeas.v1.Envelope.Builder,
io.grafeas.v1.EnvelopeOrBuilder>(getEnvelope(), getParentForChildren(), isClean());
envelope_ = null;
}
return envelopeBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:grafeas.v1.Occurrence)
}
// @@protoc_insertion_point(class_scope:grafeas.v1.Occurrence)
private static final io.grafeas.v1.Occurrence DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.grafeas.v1.Occurrence();
}
public static io.grafeas.v1.Occurrence getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Occurrence parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.grafeas.v1.Occurrence getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy