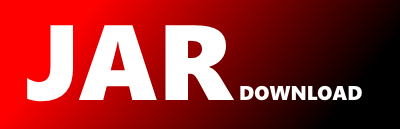
io.gravitee.repository.jdbc.management.JdbcHelper Maven / Gradle / Ivy
/*
* Copyright © 2015 The Gravitee team (http://gravitee.io)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.gravitee.repository.jdbc.management;
import static org.springframework.util.CollectionUtils.isEmpty;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.*;
import org.springframework.jdbc.core.RowCallbackHandler;
import org.springframework.jdbc.core.RowMapper;
/**
*
* @author njt
*/
public class JdbcHelper {
public static final String AND_CLAUSE = " and ";
public static final String WHERE_CLAUSE = " where ";
@FunctionalInterface
public interface ChildAdder {
void addChild(T parent, ResultSet rs) throws SQLException;
}
public static class CollatingRowMapper implements RowCallbackHandler {
private final RowMapper mapper;
private final ChildAdder childAdder;
private final String idColumn;
private final Map, T> rows;
CollatingRowMapper(RowMapper mapper, ChildAdder childAdder, String idColumn) {
this.mapper = mapper;
this.childAdder = childAdder;
this.idColumn = idColumn;
this.rows = new LinkedHashMap<>();
}
@Override
public void processRow(ResultSet rs) throws SQLException {
Comparable> currentId = (Comparable>) rs.getObject(idColumn);
T current = rows.computeIfAbsent(
currentId,
id -> {
try {
return mapper.mapRow(rs, rows.size() + 1);
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
);
childAdder.addChild(current, rs);
}
public List getRows() {
return new ArrayList<>(rows.values());
}
}
static boolean addCondition(
final boolean first,
final StringBuilder builder,
final String propName,
final Object propVal,
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy