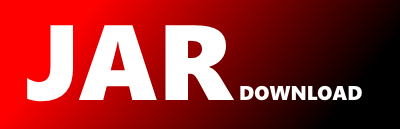
io.gravitee.common.util.ListUtils Maven / Gradle / Ivy
/*
* Copyright © 2015 The Gravitee team (http://gravitee.io)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.gravitee.common.util;
import io.vertx.core.json.Json;
import io.vertx.core.json.JsonArray;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.regex.Pattern;
import java.util.stream.Collectors;
/**
* @author Yann TAVERNIER (yann.tavernier at graviteesource.com)
* @author GraviteeSource Team
*/
public class ListUtils {
private static final String COMMA_SEPARATOR = ",";
private static final Pattern COMMA_SPLITTER = Pattern.compile(COMMA_SEPARATOR);
/**
* Return the object as an immutable {@link List}.
* This method acts differently depending on the type of the object's value.
* Elements of the {@link List} are mapped as follows:
*
* For String:
*
* - comma separated string are spitted and returned trimmed
* - parsable JSON Array elements are returned as strings (including objects)
* - if none of the above, the string is simply wrapped and trimmed
*
*
*
* For Collection:
*
* - Elements of the collection are wrapped in a new List
*
*
*
* For Array:
*
* - Each elements of the array is wrapped into the returned List
*
*
*
* For any other cases: the value is simply wrapped into the returned List.
* Notes:
* Returns an immutable collection
*
*
* @param the expected type of the value. Specify {@link Object} if you expect value of any type.
* @return an immutable List with the attribute values.
*/
public static List toList(Object object) {
if (object == null) {
return null;
}
if (object instanceof String string) {
if (isJSONArray(string)) {
JsonArray jsonArray = (JsonArray) Json.decodeValue(string);
return (List) jsonArray.getList().stream().map(Objects::toString).collect(Collectors.toUnmodifiableList());
} else {
final String[] splits = COMMA_SPLITTER.split(string);
for (int i = 0; i < splits.length; i++) {
splits[i] = splits[i].trim();
}
return (List) List.of(splits);
}
}
if (object instanceof Collection) {
// copy to immutable list
return List.copyOf((Collection extends T>) object);
}
if (object.getClass().isArray()) {
return List.of((T[]) object);
}
return List.of((T) object);
}
private static boolean isJSONArray(String jsonCandidate) {
final String trimmedJsonCandidate = jsonCandidate.trim();
return trimmedJsonCandidate.startsWith("[") && trimmedJsonCandidate.endsWith("]");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy