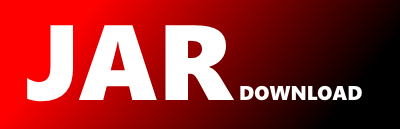
io.gravitee.gateway.reactive.api.context.http.HttpMessageResponse Maven / Gradle / Ivy
/*
* Copyright © 2015 The Gravitee team (http://gravitee.io)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.gravitee.gateway.reactive.api.context.http;
import io.gravitee.gateway.reactive.api.context.base.BaseMessageResponse;
import io.gravitee.gateway.reactive.api.message.Message;
import io.reactivex.rxjava3.core.Completable;
import io.reactivex.rxjava3.core.Flowable;
import io.reactivex.rxjava3.core.FlowableTransformer;
import io.reactivex.rxjava3.core.Maybe;
import java.util.function.Function;
/**
* Represents a response that can manipulate a flow of messages.
*
* @author Guillaume LAMIRAND (guillaume.lamirand at graviteesource.com)
* @author GraviteeSource Team
*/
public interface HttpMessageResponse extends BaseMessageResponse, HttpBaseResponse {
/**
* Get the response message flow as a {@link Flowable} of {@link Message}.
* WARN: you should not keep a direct reference on the message flow as it could be overridden by others at anytime.
*
* @return a {@link Flowable} of {@link Message}.
*/
Flowable messages();
/**
* Set the response message flow.
* WARN:
*
* - Replacing the message flow DOES NOT take care of the previous message flow in place.
* - You MUST ensure to consume the previous message flow when using it.
* - You SHOULD consider using {@link #onMessages(FlowableTransformer)} or {@link #onMessage(Function)} that may be more appropriate for message transformation.
*
*
* @see #onMessage(Function)
* @see #onMessages(FlowableTransformer)
*/
void messages(final Flowable messages);
/**
* Applies a given transformation on each message.
* Ex:
*
* response.onMessages(messages -> messages.flatMap(message -> transformMyMessage(message)));
*
*
* @param onMessages the transformer that will be applied on each message.
* @return a {@link Completable} that completes once the message transformation has been set up on the message flow (not executed).
*/
Completable onMessages(final FlowableTransformer onMessages);
/**
* Applies a given transformation on each message.
* Ex:
* Discard a message:
*
* response.onMessage(message -> Maybe.empty());
*
*
* Update a message:
*
* response.onMessage(message -> transformMyMessage(message));
*
*
* @param onMessage the transformer that will be applied on each message.
* @return a {@link Completable} that completes once the message transformation has been set up on the message flow (not executed).
*/
default Completable onMessage(Function> onMessage) {
return onMessages(upstream -> upstream.concatMapMaybe(onMessage::apply));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy