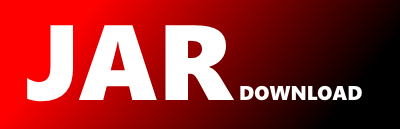
io.gravitee.gateway.reactive.api.message.DefaultMessage Maven / Gradle / Ivy
/*
* Copyright © 2015 The Gravitee team (http://gravitee.io)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.gravitee.gateway.reactive.api.message;
import io.gravitee.common.util.ListUtils;
import io.gravitee.gateway.api.buffer.Buffer;
import io.gravitee.gateway.api.http.HttpHeaders;
import io.gravitee.gateway.reactive.api.tracing.message.TracingMessage;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.UUID;
import lombok.AccessLevel;
import lombok.AllArgsConstructor;
import lombok.Builder;
import lombok.Data;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.Setter;
import lombok.experimental.Accessors;
@Data
@Builder
@AllArgsConstructor
@NoArgsConstructor
@Accessors(fluent = true)
public class DefaultMessage implements TracingMessage {
public static final String SOURCE_TIMESTAMP = "sourceTimestamp";
private String id;
@Builder.Default
private String correlationId = UUID.randomUUID().toString();
private String parentCorrelationId;
@Builder.Default
private long timestamp = System.currentTimeMillis();
@Getter(AccessLevel.NONE)
@Setter(AccessLevel.NONE)
private long sourceTimestamp = timestamp;
private Map attributes;
private Map internalAttributes;
private Map metadata;
private HttpHeaders headers;
private Buffer content;
private boolean error;
private Runnable ackRunnable;
private Runnable endRunnable;
private boolean ended;
private Map tracingAttributes;
public DefaultMessage(final String content) {
this(); // Due to @Builder.Default annotation
if (content != null) {
this.content = Buffer.buffer(content);
}
}
private static Map unmodifiableMetadata(final Map metadata) {
if (metadata != null) {
return Collections.unmodifiableMap(metadata);
} else {
return Map.of();
}
}
@Override
public T attribute(final String name) {
return (T) getOrInitAttribute().get(name);
}
@Override
public List attributeAsList(String name) {
return ListUtils.toList(getOrInitAttribute().get(name));
}
@Override
public DefaultMessage attribute(final String name, final Object value) {
getOrInitAttribute().put(name, value);
return this;
}
@Override
public DefaultMessage removeAttribute(final String name) {
getOrInitAttribute().remove(name);
return this;
}
@Override
public Set attributeNames() {
return getOrInitAttribute().keySet();
}
@Override
public Map attributes() {
return Collections.unmodifiableMap((Map) getOrInitAttribute());
}
@Override
public T internalAttribute(final String name) {
return (T) getOrInitInternalAttribute().get(name);
}
@Override
public DefaultMessage internalAttribute(final String name, final Object value) {
getOrInitInternalAttribute().put(name, value);
return this;
}
@Override
public DefaultMessage removeInternalAttribute(final String name) {
getOrInitInternalAttribute().remove(name);
return this;
}
@Override
public Set internalAttributeNames() {
return getOrInitInternalAttribute().keySet();
}
@Override
public Map internalAttributes() {
return Collections.unmodifiableMap((Map) getOrInitInternalAttribute());
}
@Override
public Map metadata() {
if (metadata == null) {
metadata = Map.of();
}
return metadata;
}
@Override
public HttpHeaders headers() {
if (headers == null) {
headers = HttpHeaders.create();
}
return headers;
}
@Override
public DefaultMessage content(Buffer content) {
this.content = content;
return this;
}
@Override
public DefaultMessage content(String content) {
if (content != null) {
this.content(Buffer.buffer(content));
} else {
this.content((Buffer) null);
}
return this;
}
public DefaultMessage metadata(Map metadata) {
this.metadata = unmodifiableMetadata(metadata);
return this;
}
@Override
public void ack() {
if (ackRunnable != null) {
ackRunnable.run();
}
if (!this.ended) {
this.end();
}
}
@Override
public DefaultMessage doOnEnd(final Runnable runnable) {
this.endRunnable = runnable;
return this;
}
@Override
public void end() {
if (this.endRunnable != null) {
this.endRunnable.run();
}
this.ended = true;
}
@Override
public Map tracingAttributes() {
if (this.tracingAttributes == null) {
this.tracingAttributes = new HashMap<>();
}
return tracingAttributes;
}
@Override
public void addTracingAttribute(final String key, final String value) {
if (key == null || value == null) {
throw new IllegalArgumentException("Key or value of tracing attribute cannot be null");
}
tracingAttributes.put(key, value);
}
public static DefaultMessageBuilder builder() {
return new DefaultMessageBuilder() {
@Override
public DefaultMessage build() {
prebuild();
return super.build();
}
};
}
public static class DefaultMessageBuilder {
// Insert sourceTimestamp into metadata before building the object
void prebuild() {
if (!timestamp$set) {
timestamp(System.currentTimeMillis());
}
if (metadata == null) {
metadata = new HashMap<>();
}
if (!metadata.containsKey(SOURCE_TIMESTAMP)) {
metadata.put(SOURCE_TIMESTAMP, sourceTimestamp != 0L ? sourceTimestamp : timestamp$value);
}
metadata = unmodifiableMetadata(metadata);
}
}
private Map getOrInitAttribute() {
if (this.attributes == null) {
this.attributes = new HashMap<>();
}
return this.attributes;
}
private Map getOrInitInternalAttribute() {
if (this.internalAttributes == null) {
this.internalAttributes = new HashMap<>();
}
return this.internalAttributes;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy