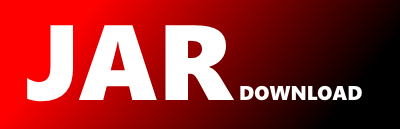
io.gravitee.node.api.license.License Maven / Gradle / Ivy
package io.gravitee.node.api.license;
import java.util.Date;
import java.util.Map;
import java.util.Set;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
/**
* Represents a license structure that can be used to check enabled features.
*
* @author Jeoffrey HAEYAERT (jeoffrey.haeyaert at graviteesource.com)
* @author GraviteeSource Team
*/
public interface License {
String REFERENCE_TYPE_PLATFORM = "PLATFORM";
String REFERENCE_ID_PLATFORM = "PLATFORM";
String REFERENCE_TYPE_ORGANIZATION = "ORGANIZATION";
/**
* The reference type the license is associated to (e.g. PLATFORM, ORGANIZATION, ...)
* @return the reference type the license is associated to.
*/
@Nonnull
String getReferenceType();
/**
* The reference id the license is associated to (e.g. organization identifier when associated to an organization)
* @return the reference type the license is associated to.
*/
@Nonnull
String getReferenceId();
/**
* Return the tier associated with this license.
*
* @return the tier or null
if no tier is assigned for this license.
*/
@Nullable
String getTier();
/**
* Return the list of all packs allowed by this license.
*
* @return the list of all packs allowed by this license or empty if no packs is assigned.
*/
@Nonnull
Set getPacks();
/**
* Return the list of all features allowed for this license. The list of the features is built from the tier, packs and all individual features assigned.
*
* @return the list of all features allowed for this license.
*/
@Nonnull
Set getFeatures();
/**
* Indicates if a feature is enabled or not for this license.
*
* @param feature the feature to check.
*
* @return true
if the feature is allowed, false
else.
*/
boolean isFeatureEnabled(String feature);
/**
* Verify that the license is valid. This checks both the signature and the expiration date.
*
* @throws InvalidLicenseException if the license is expired or invalid.
*/
void verify() throws InvalidLicenseException;
/**
* Return the expiration date of the license or null
if the license has no expiration date.
*
* @return the license expiration date.
*/
@Nullable
Date getExpirationDate();
/**
* Indicates if the license is expired or not.
* Having a null
expiration date means no expiration.
*
* @return true
if the license has expired, false
else.
*/
boolean isExpired();
/**
* Return a map of all the attributes of the license.
*
* @return a map of all the attributes of the license.
*/
@Nonnull
Map getAttributes();
/**
* Return a map of all the raw information of the license.
*
* @return a map of all the raw information of the license.
*/
@Nonnull
Map getRawAttributes();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy