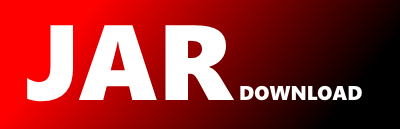
io.gravitee.rest.api.service.swagger.converter.extension.XGraviteeIODefinition Maven / Gradle / Ivy
package io.gravitee.rest.api.service.swagger.converter.extension;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonValue;
/**
* xGraviteeIODefinition
*
* Definition of the Gravitee vendor extension for Swagger/OpenAPI descriptors : x-graviteeio-definition
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"categories",
"virtualHosts",
"groups",
"labels",
"metadata",
"picture",
"properties",
"tags",
"visibility"
})
public class XGraviteeIODefinition {
/**
* List of categories the API belongs to.
*
*/
@JsonProperty("categories")
@JsonPropertyDescription("List of categories the API belongs to.")
private List categories = new ArrayList();
/**
* List of virtual hosts for this API.
*
*/
@JsonProperty("virtualHosts")
@JsonPropertyDescription("List of virtual hosts for this API.")
private List virtualHosts = new ArrayList();
/**
* List of groups attached to this API.
*
*/
@JsonProperty("groups")
@JsonPropertyDescription("List of groups attached to this API.")
private List groups = new ArrayList();
/**
* List of labels attached to this API.
*
*/
@JsonProperty("labels")
@JsonPropertyDescription("List of labels attached to this API.")
private List labels = new ArrayList();
/**
* List of data about API.
*
*/
@JsonProperty("metadata")
@JsonPropertyDescription("List of data about API.")
private List metadata = new ArrayList();
/**
* A picture in data-URI format.
*
*/
@JsonProperty("picture")
@JsonPropertyDescription("A picture in data-URI format.")
private String picture;
/**
* A list of properties about the API.
*
*/
@JsonProperty("properties")
@JsonPropertyDescription("A list of properties about the API.")
private List properties = new ArrayList();
/**
* List of the sharding tags of the API. Used for deployment on Gateway.
*
*/
@JsonProperty("tags")
@JsonPropertyDescription("List of the sharding tags of the API. Used for deployment on Gateway.")
private List tags = new ArrayList();
/**
* The visibility of the API.
*
*/
@JsonProperty("visibility")
@JsonPropertyDescription("The visibility of the API.")
private XGraviteeIODefinition.Visibility visibility;
@JsonIgnore
private Map additionalProperties = new HashMap();
/**
* List of categories the API belongs to.
*
*/
@JsonProperty("categories")
public List getCategories() {
return categories;
}
/**
* List of categories the API belongs to.
*
*/
@JsonProperty("categories")
public void setCategories(List categories) {
this.categories = categories;
}
/**
* List of virtual hosts for this API.
*
*/
@JsonProperty("virtualHosts")
public List getVirtualHosts() {
return virtualHosts;
}
/**
* List of virtual hosts for this API.
*
*/
@JsonProperty("virtualHosts")
public void setVirtualHosts(List virtualHosts) {
this.virtualHosts = virtualHosts;
}
/**
* List of groups attached to this API.
*
*/
@JsonProperty("groups")
public List getGroups() {
return groups;
}
/**
* List of groups attached to this API.
*
*/
@JsonProperty("groups")
public void setGroups(List groups) {
this.groups = groups;
}
/**
* List of labels attached to this API.
*
*/
@JsonProperty("labels")
public List getLabels() {
return labels;
}
/**
* List of labels attached to this API.
*
*/
@JsonProperty("labels")
public void setLabels(List labels) {
this.labels = labels;
}
/**
* List of data about API.
*
*/
@JsonProperty("metadata")
public List getMetadata() {
return metadata;
}
/**
* List of data about API.
*
*/
@JsonProperty("metadata")
public void setMetadata(List metadata) {
this.metadata = metadata;
}
/**
* A picture in data-URI format.
*
*/
@JsonProperty("picture")
public String getPicture() {
return picture;
}
/**
* A picture in data-URI format.
*
*/
@JsonProperty("picture")
public void setPicture(String picture) {
this.picture = picture;
}
/**
* A list of properties about the API.
*
*/
@JsonProperty("properties")
public List getProperties() {
return properties;
}
/**
* A list of properties about the API.
*
*/
@JsonProperty("properties")
public void setProperties(List properties) {
this.properties = properties;
}
/**
* List of the sharding tags of the API. Used for deployment on Gateway.
*
*/
@JsonProperty("tags")
public List getTags() {
return tags;
}
/**
* List of the sharding tags of the API. Used for deployment on Gateway.
*
*/
@JsonProperty("tags")
public void setTags(List tags) {
this.tags = tags;
}
/**
* The visibility of the API.
*
*/
@JsonProperty("visibility")
public XGraviteeIODefinition.Visibility getVisibility() {
return visibility;
}
/**
* The visibility of the API.
*
*/
@JsonProperty("visibility")
public void setVisibility(XGraviteeIODefinition.Visibility visibility) {
this.visibility = visibility;
}
@JsonAnyGetter
public Map getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append(XGraviteeIODefinition.class.getName()).append('@').append(Integer.toHexString(System.identityHashCode(this))).append('[');
sb.append("categories");
sb.append('=');
sb.append(((this.categories == null)?"":this.categories));
sb.append(',');
sb.append("virtualHosts");
sb.append('=');
sb.append(((this.virtualHosts == null)?"":this.virtualHosts));
sb.append(',');
sb.append("groups");
sb.append('=');
sb.append(((this.groups == null)?"":this.groups));
sb.append(',');
sb.append("labels");
sb.append('=');
sb.append(((this.labels == null)?"":this.labels));
sb.append(',');
sb.append("metadata");
sb.append('=');
sb.append(((this.metadata == null)?"":this.metadata));
sb.append(',');
sb.append("picture");
sb.append('=');
sb.append(((this.picture == null)?"":this.picture));
sb.append(',');
sb.append("properties");
sb.append('=');
sb.append(((this.properties == null)?"":this.properties));
sb.append(',');
sb.append("tags");
sb.append('=');
sb.append(((this.tags == null)?"":this.tags));
sb.append(',');
sb.append("visibility");
sb.append('=');
sb.append(((this.visibility == null)?"":this.visibility));
sb.append(',');
sb.append("additionalProperties");
sb.append('=');
sb.append(((this.additionalProperties == null)?"":this.additionalProperties));
sb.append(',');
if (sb.charAt((sb.length()- 1)) == ',') {
sb.setCharAt((sb.length()- 1), ']');
} else {
sb.append(']');
}
return sb.toString();
}
@Override
public int hashCode() {
int result = 1;
result = ((result* 31)+((this.virtualHosts == null)? 0 :this.virtualHosts.hashCode()));
result = ((result* 31)+((this.metadata == null)? 0 :this.metadata.hashCode()));
result = ((result* 31)+((this.visibility == null)? 0 :this.visibility.hashCode()));
result = ((result* 31)+((this.groups == null)? 0 :this.groups.hashCode()));
result = ((result* 31)+((this.categories == null)? 0 :this.categories.hashCode()));
result = ((result* 31)+((this.additionalProperties == null)? 0 :this.additionalProperties.hashCode()));
result = ((result* 31)+((this.picture == null)? 0 :this.picture.hashCode()));
result = ((result* 31)+((this.properties == null)? 0 :this.properties.hashCode()));
result = ((result* 31)+((this.labels == null)? 0 :this.labels.hashCode()));
result = ((result* 31)+((this.tags == null)? 0 :this.tags.hashCode()));
return result;
}
@Override
public boolean equals(Object other) {
if (other == this) {
return true;
}
if ((other instanceof XGraviteeIODefinition) == false) {
return false;
}
XGraviteeIODefinition rhs = ((XGraviteeIODefinition) other);
return (((((((((((this.virtualHosts == rhs.virtualHosts)||((this.virtualHosts!= null)&&this.virtualHosts.equals(rhs.virtualHosts)))&&((this.metadata == rhs.metadata)||((this.metadata!= null)&&this.metadata.equals(rhs.metadata))))&&((this.visibility == rhs.visibility)||((this.visibility!= null)&&this.visibility.equals(rhs.visibility))))&&((this.groups == rhs.groups)||((this.groups!= null)&&this.groups.equals(rhs.groups))))&&((this.categories == rhs.categories)||((this.categories!= null)&&this.categories.equals(rhs.categories))))&&((this.additionalProperties == rhs.additionalProperties)||((this.additionalProperties!= null)&&this.additionalProperties.equals(rhs.additionalProperties))))&&((this.picture == rhs.picture)||((this.picture!= null)&&this.picture.equals(rhs.picture))))&&((this.properties == rhs.properties)||((this.properties!= null)&&this.properties.equals(rhs.properties))))&&((this.labels == rhs.labels)||((this.labels!= null)&&this.labels.equals(rhs.labels))))&&((this.tags == rhs.tags)||((this.tags!= null)&&this.tags.equals(rhs.tags))));
}
/**
* The visibility of the API.
*
*/
public enum Visibility {
PUBLIC("PUBLIC"),
PRIVATE("PRIVATE");
private final String value;
private final static Map CONSTANTS = new HashMap();
static {
for (XGraviteeIODefinition.Visibility c: values()) {
CONSTANTS.put(c.value, c);
}
}
private Visibility(String value) {
this.value = value;
}
@Override
public String toString() {
return this.value;
}
@JsonValue
public String value() {
return this.value;
}
@JsonCreator
public static XGraviteeIODefinition.Visibility fromValue(String value) {
XGraviteeIODefinition.Visibility constant = CONSTANTS.get(value);
if (constant == null) {
throw new IllegalArgumentException(value);
} else {
return constant;
}
}
}
}