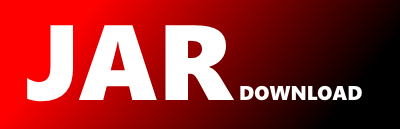
io.gridgo.connector.jdbc.support.Helper Maven / Gradle / Ivy
package io.gridgo.connector.jdbc.support;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.jdbi.v3.core.statement.SqlStatement;
import io.gridgo.bean.BArray;
import io.gridgo.bean.BObject;
import io.gridgo.bean.BValue;
import lombok.extern.slf4j.Slf4j;
@Slf4j
public class Helper {
private Helper() {
}
public static List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy