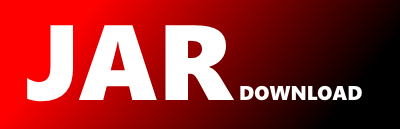
io.grpc.alts.internal.StartServerHandshakeReqOrBuilder Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: grpc/gcp/handshaker.proto
// Protobuf Java Version: 3.25.1
package io.grpc.alts.internal;
public interface StartServerHandshakeReqOrBuilder extends
// @@protoc_insertion_point(interface_extends:grpc.gcp.StartServerHandshakeReq)
com.google.protobuf.MessageOrBuilder {
/**
*
* The application protocols supported by the server, e.g., "h2" (for http2),
* "grpc".
*
*
* repeated string application_protocols = 1;
* @return A list containing the applicationProtocols.
*/
java.util.List
getApplicationProtocolsList();
/**
*
* The application protocols supported by the server, e.g., "h2" (for http2),
* "grpc".
*
*
* repeated string application_protocols = 1;
* @return The count of applicationProtocols.
*/
int getApplicationProtocolsCount();
/**
*
* The application protocols supported by the server, e.g., "h2" (for http2),
* "grpc".
*
*
* repeated string application_protocols = 1;
* @param index The index of the element to return.
* @return The applicationProtocols at the given index.
*/
java.lang.String getApplicationProtocols(int index);
/**
*
* The application protocols supported by the server, e.g., "h2" (for http2),
* "grpc".
*
*
* repeated string application_protocols = 1;
* @param index The index of the value to return.
* @return The bytes of the applicationProtocols at the given index.
*/
com.google.protobuf.ByteString
getApplicationProtocolsBytes(int index);
/**
*
* Handshake parameters (record protocols and local identities supported by
* the server) mapped by the handshake protocol. Each handshake security
* protocol (e.g., TLS or ALTS) has its own set of record protocols and local
* identities. Since protobuf does not support enum as key to the map, the key
* to handshake_parameters is the integer value of HandshakeProtocol enum.
*
*
* map<int32, .grpc.gcp.ServerHandshakeParameters> handshake_parameters = 2;
*/
int getHandshakeParametersCount();
/**
*
* Handshake parameters (record protocols and local identities supported by
* the server) mapped by the handshake protocol. Each handshake security
* protocol (e.g., TLS or ALTS) has its own set of record protocols and local
* identities. Since protobuf does not support enum as key to the map, the key
* to handshake_parameters is the integer value of HandshakeProtocol enum.
*
*
* map<int32, .grpc.gcp.ServerHandshakeParameters> handshake_parameters = 2;
*/
boolean containsHandshakeParameters(
int key);
/**
* Use {@link #getHandshakeParametersMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getHandshakeParameters();
/**
*
* Handshake parameters (record protocols and local identities supported by
* the server) mapped by the handshake protocol. Each handshake security
* protocol (e.g., TLS or ALTS) has its own set of record protocols and local
* identities. Since protobuf does not support enum as key to the map, the key
* to handshake_parameters is the integer value of HandshakeProtocol enum.
*
*
* map<int32, .grpc.gcp.ServerHandshakeParameters> handshake_parameters = 2;
*/
java.util.Map
getHandshakeParametersMap();
/**
*
* Handshake parameters (record protocols and local identities supported by
* the server) mapped by the handshake protocol. Each handshake security
* protocol (e.g., TLS or ALTS) has its own set of record protocols and local
* identities. Since protobuf does not support enum as key to the map, the key
* to handshake_parameters is the integer value of HandshakeProtocol enum.
*
*
* map<int32, .grpc.gcp.ServerHandshakeParameters> handshake_parameters = 2;
*/
/* nullable */
io.grpc.alts.internal.ServerHandshakeParameters getHandshakeParametersOrDefault(
int key,
/* nullable */
io.grpc.alts.internal.ServerHandshakeParameters defaultValue);
/**
*
* Handshake parameters (record protocols and local identities supported by
* the server) mapped by the handshake protocol. Each handshake security
* protocol (e.g., TLS or ALTS) has its own set of record protocols and local
* identities. Since protobuf does not support enum as key to the map, the key
* to handshake_parameters is the integer value of HandshakeProtocol enum.
*
*
* map<int32, .grpc.gcp.ServerHandshakeParameters> handshake_parameters = 2;
*/
io.grpc.alts.internal.ServerHandshakeParameters getHandshakeParametersOrThrow(
int key);
/**
*
* Bytes in out_frames returned from the peer's HandshakerResp. It is possible
* that the peer's out_frames are split into multiple HandshakReq messages.
*
*
* bytes in_bytes = 3;
* @return The inBytes.
*/
com.google.protobuf.ByteString getInBytes();
/**
*
* (Optional) Local endpoint information of the connection to the client,
* such as local IP address, port number, and network protocol.
*
*
* .grpc.gcp.Endpoint local_endpoint = 4;
* @return Whether the localEndpoint field is set.
*/
boolean hasLocalEndpoint();
/**
*
* (Optional) Local endpoint information of the connection to the client,
* such as local IP address, port number, and network protocol.
*
*
* .grpc.gcp.Endpoint local_endpoint = 4;
* @return The localEndpoint.
*/
io.grpc.alts.internal.Endpoint getLocalEndpoint();
/**
*
* (Optional) Local endpoint information of the connection to the client,
* such as local IP address, port number, and network protocol.
*
*
* .grpc.gcp.Endpoint local_endpoint = 4;
*/
io.grpc.alts.internal.EndpointOrBuilder getLocalEndpointOrBuilder();
/**
*
* (Optional) Endpoint information of the remote client, such as IP address,
* port number, and network protocol.
*
*
* .grpc.gcp.Endpoint remote_endpoint = 5;
* @return Whether the remoteEndpoint field is set.
*/
boolean hasRemoteEndpoint();
/**
*
* (Optional) Endpoint information of the remote client, such as IP address,
* port number, and network protocol.
*
*
* .grpc.gcp.Endpoint remote_endpoint = 5;
* @return The remoteEndpoint.
*/
io.grpc.alts.internal.Endpoint getRemoteEndpoint();
/**
*
* (Optional) Endpoint information of the remote client, such as IP address,
* port number, and network protocol.
*
*
* .grpc.gcp.Endpoint remote_endpoint = 5;
*/
io.grpc.alts.internal.EndpointOrBuilder getRemoteEndpointOrBuilder();
/**
*
* (Optional) RPC protocol versions supported by the server.
*
*
* .grpc.gcp.RpcProtocolVersions rpc_versions = 6;
* @return Whether the rpcVersions field is set.
*/
boolean hasRpcVersions();
/**
*
* (Optional) RPC protocol versions supported by the server.
*
*
* .grpc.gcp.RpcProtocolVersions rpc_versions = 6;
* @return The rpcVersions.
*/
io.grpc.alts.internal.RpcProtocolVersions getRpcVersions();
/**
*
* (Optional) RPC protocol versions supported by the server.
*
*
* .grpc.gcp.RpcProtocolVersions rpc_versions = 6;
*/
io.grpc.alts.internal.RpcProtocolVersionsOrBuilder getRpcVersionsOrBuilder();
/**
*
* (Optional) Maximum frame size supported by the server.
*
*
* uint32 max_frame_size = 7;
* @return The maxFrameSize.
*/
int getMaxFrameSize();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy