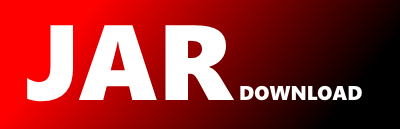
io.envoyproxy.envoy.config.cluster.v3.OutlierDetection Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: envoy/config/cluster/v3/outlier_detection.proto
// Protobuf Java Version: 3.25.5
package io.envoyproxy.envoy.config.cluster.v3;
/**
*
* See the :ref:`architecture overview <arch_overview_outlier_detection>` for
* more information on outlier detection.
* [#next-free-field: 26]
*
*
* Protobuf type {@code envoy.config.cluster.v3.OutlierDetection}
*/
public final class OutlierDetection extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:envoy.config.cluster.v3.OutlierDetection)
OutlierDetectionOrBuilder {
private static final long serialVersionUID = 0L;
// Use OutlierDetection.newBuilder() to construct.
private OutlierDetection(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private OutlierDetection() {
monitors_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new OutlierDetection();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.envoyproxy.envoy.config.cluster.v3.OutlierDetectionProto.internal_static_envoy_config_cluster_v3_OutlierDetection_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.envoyproxy.envoy.config.cluster.v3.OutlierDetectionProto.internal_static_envoy_config_cluster_v3_OutlierDetection_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.envoyproxy.envoy.config.cluster.v3.OutlierDetection.class, io.envoyproxy.envoy.config.cluster.v3.OutlierDetection.Builder.class);
}
private int bitField0_;
public static final int CONSECUTIVE_5XX_FIELD_NUMBER = 1;
private com.google.protobuf.UInt32Value consecutive5Xx_;
/**
*
* The number of consecutive server-side error responses (for HTTP traffic,
* 5xx responses; for TCP traffic, connection failures; for Redis, failure to
* respond PONG; etc.) before a consecutive 5xx ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_5xx = 1;
* @return Whether the consecutive5xx field is set.
*/
@java.lang.Override
public boolean hasConsecutive5Xx() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The number of consecutive server-side error responses (for HTTP traffic,
* 5xx responses; for TCP traffic, connection failures; for Redis, failure to
* respond PONG; etc.) before a consecutive 5xx ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_5xx = 1;
* @return The consecutive5xx.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getConsecutive5Xx() {
return consecutive5Xx_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : consecutive5Xx_;
}
/**
*
* The number of consecutive server-side error responses (for HTTP traffic,
* 5xx responses; for TCP traffic, connection failures; for Redis, failure to
* respond PONG; etc.) before a consecutive 5xx ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_5xx = 1;
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getConsecutive5XxOrBuilder() {
return consecutive5Xx_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : consecutive5Xx_;
}
public static final int INTERVAL_FIELD_NUMBER = 2;
private com.google.protobuf.Duration interval_;
/**
*
* The time interval between ejection analysis sweeps. This can result in
* both new ejections as well as hosts being returned to service. Defaults
* to 10000ms or 10s.
*
*
* .google.protobuf.Duration interval = 2 [(.validate.rules) = { ... }
* @return Whether the interval field is set.
*/
@java.lang.Override
public boolean hasInterval() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The time interval between ejection analysis sweeps. This can result in
* both new ejections as well as hosts being returned to service. Defaults
* to 10000ms or 10s.
*
*
* .google.protobuf.Duration interval = 2 [(.validate.rules) = { ... }
* @return The interval.
*/
@java.lang.Override
public com.google.protobuf.Duration getInterval() {
return interval_ == null ? com.google.protobuf.Duration.getDefaultInstance() : interval_;
}
/**
*
* The time interval between ejection analysis sweeps. This can result in
* both new ejections as well as hosts being returned to service. Defaults
* to 10000ms or 10s.
*
*
* .google.protobuf.Duration interval = 2 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getIntervalOrBuilder() {
return interval_ == null ? com.google.protobuf.Duration.getDefaultInstance() : interval_;
}
public static final int BASE_EJECTION_TIME_FIELD_NUMBER = 3;
private com.google.protobuf.Duration baseEjectionTime_;
/**
*
* The base time that a host is ejected for. The real time is equal to the
* base time multiplied by the number of times the host has been ejected and is
* capped by :ref:`max_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_time>`.
* Defaults to 30000ms or 30s.
*
*
* .google.protobuf.Duration base_ejection_time = 3 [(.validate.rules) = { ... }
* @return Whether the baseEjectionTime field is set.
*/
@java.lang.Override
public boolean hasBaseEjectionTime() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The base time that a host is ejected for. The real time is equal to the
* base time multiplied by the number of times the host has been ejected and is
* capped by :ref:`max_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_time>`.
* Defaults to 30000ms or 30s.
*
*
* .google.protobuf.Duration base_ejection_time = 3 [(.validate.rules) = { ... }
* @return The baseEjectionTime.
*/
@java.lang.Override
public com.google.protobuf.Duration getBaseEjectionTime() {
return baseEjectionTime_ == null ? com.google.protobuf.Duration.getDefaultInstance() : baseEjectionTime_;
}
/**
*
* The base time that a host is ejected for. The real time is equal to the
* base time multiplied by the number of times the host has been ejected and is
* capped by :ref:`max_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_time>`.
* Defaults to 30000ms or 30s.
*
*
* .google.protobuf.Duration base_ejection_time = 3 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getBaseEjectionTimeOrBuilder() {
return baseEjectionTime_ == null ? com.google.protobuf.Duration.getDefaultInstance() : baseEjectionTime_;
}
public static final int MAX_EJECTION_PERCENT_FIELD_NUMBER = 4;
private com.google.protobuf.UInt32Value maxEjectionPercent_;
/**
*
* The maximum % of an upstream cluster that can be ejected due to outlier detection. Defaults to 10% .
* Will eject at least one host regardless of the value if :ref:`always_eject_one_host<envoy_v3_api_field_config.cluster.v3.OutlierDetection.always_eject_one_host>` is enabled.
*
*
* .google.protobuf.UInt32Value max_ejection_percent = 4 [(.validate.rules) = { ... }
* @return Whether the maxEjectionPercent field is set.
*/
@java.lang.Override
public boolean hasMaxEjectionPercent() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The maximum % of an upstream cluster that can be ejected due to outlier detection. Defaults to 10% .
* Will eject at least one host regardless of the value if :ref:`always_eject_one_host<envoy_v3_api_field_config.cluster.v3.OutlierDetection.always_eject_one_host>` is enabled.
*
*
* .google.protobuf.UInt32Value max_ejection_percent = 4 [(.validate.rules) = { ... }
* @return The maxEjectionPercent.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getMaxEjectionPercent() {
return maxEjectionPercent_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : maxEjectionPercent_;
}
/**
*
* The maximum % of an upstream cluster that can be ejected due to outlier detection. Defaults to 10% .
* Will eject at least one host regardless of the value if :ref:`always_eject_one_host<envoy_v3_api_field_config.cluster.v3.OutlierDetection.always_eject_one_host>` is enabled.
*
*
* .google.protobuf.UInt32Value max_ejection_percent = 4 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getMaxEjectionPercentOrBuilder() {
return maxEjectionPercent_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : maxEjectionPercent_;
}
public static final int ENFORCING_CONSECUTIVE_5XX_FIELD_NUMBER = 5;
private com.google.protobuf.UInt32Value enforcingConsecutive5Xx_;
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive 5xx. This setting can be used to disable
* ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_5xx = 5 [(.validate.rules) = { ... }
* @return Whether the enforcingConsecutive5xx field is set.
*/
@java.lang.Override
public boolean hasEnforcingConsecutive5Xx() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive 5xx. This setting can be used to disable
* ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_5xx = 5 [(.validate.rules) = { ... }
* @return The enforcingConsecutive5xx.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getEnforcingConsecutive5Xx() {
return enforcingConsecutive5Xx_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingConsecutive5Xx_;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive 5xx. This setting can be used to disable
* ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_5xx = 5 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getEnforcingConsecutive5XxOrBuilder() {
return enforcingConsecutive5Xx_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingConsecutive5Xx_;
}
public static final int ENFORCING_SUCCESS_RATE_FIELD_NUMBER = 6;
private com.google.protobuf.UInt32Value enforcingSuccessRate_;
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics. This setting can be used to
* disable ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_success_rate = 6 [(.validate.rules) = { ... }
* @return Whether the enforcingSuccessRate field is set.
*/
@java.lang.Override
public boolean hasEnforcingSuccessRate() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics. This setting can be used to
* disable ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_success_rate = 6 [(.validate.rules) = { ... }
* @return The enforcingSuccessRate.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getEnforcingSuccessRate() {
return enforcingSuccessRate_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingSuccessRate_;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics. This setting can be used to
* disable ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_success_rate = 6 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getEnforcingSuccessRateOrBuilder() {
return enforcingSuccessRate_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingSuccessRate_;
}
public static final int SUCCESS_RATE_MINIMUM_HOSTS_FIELD_NUMBER = 7;
private com.google.protobuf.UInt32Value successRateMinimumHosts_;
/**
*
* The number of hosts in a cluster that must have enough request volume to
* detect success rate outliers. If the number of hosts is less than this
* setting, outlier detection via success rate statistics is not performed
* for any host in the cluster. Defaults to 5.
*
*
* .google.protobuf.UInt32Value success_rate_minimum_hosts = 7;
* @return Whether the successRateMinimumHosts field is set.
*/
@java.lang.Override
public boolean hasSuccessRateMinimumHosts() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* The number of hosts in a cluster that must have enough request volume to
* detect success rate outliers. If the number of hosts is less than this
* setting, outlier detection via success rate statistics is not performed
* for any host in the cluster. Defaults to 5.
*
*
* .google.protobuf.UInt32Value success_rate_minimum_hosts = 7;
* @return The successRateMinimumHosts.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getSuccessRateMinimumHosts() {
return successRateMinimumHosts_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : successRateMinimumHosts_;
}
/**
*
* The number of hosts in a cluster that must have enough request volume to
* detect success rate outliers. If the number of hosts is less than this
* setting, outlier detection via success rate statistics is not performed
* for any host in the cluster. Defaults to 5.
*
*
* .google.protobuf.UInt32Value success_rate_minimum_hosts = 7;
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getSuccessRateMinimumHostsOrBuilder() {
return successRateMinimumHosts_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : successRateMinimumHosts_;
}
public static final int SUCCESS_RATE_REQUEST_VOLUME_FIELD_NUMBER = 8;
private com.google.protobuf.UInt32Value successRateRequestVolume_;
/**
*
* The minimum number of total requests that must be collected in one
* interval (as defined by the interval duration above) to include this host
* in success rate based outlier detection. If the volume is lower than this
* setting, outlier detection via success rate statistics is not performed
* for that host. Defaults to 100.
*
*
* .google.protobuf.UInt32Value success_rate_request_volume = 8;
* @return Whether the successRateRequestVolume field is set.
*/
@java.lang.Override
public boolean hasSuccessRateRequestVolume() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* The minimum number of total requests that must be collected in one
* interval (as defined by the interval duration above) to include this host
* in success rate based outlier detection. If the volume is lower than this
* setting, outlier detection via success rate statistics is not performed
* for that host. Defaults to 100.
*
*
* .google.protobuf.UInt32Value success_rate_request_volume = 8;
* @return The successRateRequestVolume.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getSuccessRateRequestVolume() {
return successRateRequestVolume_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : successRateRequestVolume_;
}
/**
*
* The minimum number of total requests that must be collected in one
* interval (as defined by the interval duration above) to include this host
* in success rate based outlier detection. If the volume is lower than this
* setting, outlier detection via success rate statistics is not performed
* for that host. Defaults to 100.
*
*
* .google.protobuf.UInt32Value success_rate_request_volume = 8;
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getSuccessRateRequestVolumeOrBuilder() {
return successRateRequestVolume_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : successRateRequestVolume_;
}
public static final int SUCCESS_RATE_STDEV_FACTOR_FIELD_NUMBER = 9;
private com.google.protobuf.UInt32Value successRateStdevFactor_;
/**
*
* This factor is used to determine the ejection threshold for success rate
* outlier ejection. The ejection threshold is the difference between the
* mean success rate, and the product of this factor and the standard
* deviation of the mean success rate: mean - (stdev *
* success_rate_stdev_factor). This factor is divided by a thousand to get a
* double. That is, if the desired factor is 1.9, the runtime value should
* be 1900. Defaults to 1900.
*
*
* .google.protobuf.UInt32Value success_rate_stdev_factor = 9;
* @return Whether the successRateStdevFactor field is set.
*/
@java.lang.Override
public boolean hasSuccessRateStdevFactor() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* This factor is used to determine the ejection threshold for success rate
* outlier ejection. The ejection threshold is the difference between the
* mean success rate, and the product of this factor and the standard
* deviation of the mean success rate: mean - (stdev *
* success_rate_stdev_factor). This factor is divided by a thousand to get a
* double. That is, if the desired factor is 1.9, the runtime value should
* be 1900. Defaults to 1900.
*
*
* .google.protobuf.UInt32Value success_rate_stdev_factor = 9;
* @return The successRateStdevFactor.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getSuccessRateStdevFactor() {
return successRateStdevFactor_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : successRateStdevFactor_;
}
/**
*
* This factor is used to determine the ejection threshold for success rate
* outlier ejection. The ejection threshold is the difference between the
* mean success rate, and the product of this factor and the standard
* deviation of the mean success rate: mean - (stdev *
* success_rate_stdev_factor). This factor is divided by a thousand to get a
* double. That is, if the desired factor is 1.9, the runtime value should
* be 1900. Defaults to 1900.
*
*
* .google.protobuf.UInt32Value success_rate_stdev_factor = 9;
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getSuccessRateStdevFactorOrBuilder() {
return successRateStdevFactor_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : successRateStdevFactor_;
}
public static final int CONSECUTIVE_GATEWAY_FAILURE_FIELD_NUMBER = 10;
private com.google.protobuf.UInt32Value consecutiveGatewayFailure_;
/**
*
* The number of consecutive gateway failures (502, 503, 504 status codes)
* before a consecutive gateway failure ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_gateway_failure = 10;
* @return Whether the consecutiveGatewayFailure field is set.
*/
@java.lang.Override
public boolean hasConsecutiveGatewayFailure() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
* The number of consecutive gateway failures (502, 503, 504 status codes)
* before a consecutive gateway failure ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_gateway_failure = 10;
* @return The consecutiveGatewayFailure.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getConsecutiveGatewayFailure() {
return consecutiveGatewayFailure_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : consecutiveGatewayFailure_;
}
/**
*
* The number of consecutive gateway failures (502, 503, 504 status codes)
* before a consecutive gateway failure ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_gateway_failure = 10;
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getConsecutiveGatewayFailureOrBuilder() {
return consecutiveGatewayFailure_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : consecutiveGatewayFailure_;
}
public static final int ENFORCING_CONSECUTIVE_GATEWAY_FAILURE_FIELD_NUMBER = 11;
private com.google.protobuf.UInt32Value enforcingConsecutiveGatewayFailure_;
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive gateway failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_gateway_failure = 11 [(.validate.rules) = { ... }
* @return Whether the enforcingConsecutiveGatewayFailure field is set.
*/
@java.lang.Override
public boolean hasEnforcingConsecutiveGatewayFailure() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive gateway failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_gateway_failure = 11 [(.validate.rules) = { ... }
* @return The enforcingConsecutiveGatewayFailure.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getEnforcingConsecutiveGatewayFailure() {
return enforcingConsecutiveGatewayFailure_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingConsecutiveGatewayFailure_;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive gateway failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_gateway_failure = 11 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getEnforcingConsecutiveGatewayFailureOrBuilder() {
return enforcingConsecutiveGatewayFailure_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingConsecutiveGatewayFailure_;
}
public static final int SPLIT_EXTERNAL_LOCAL_ORIGIN_ERRORS_FIELD_NUMBER = 12;
private boolean splitExternalLocalOriginErrors_ = false;
/**
*
* Determines whether to distinguish local origin failures from external errors. If set to true
* the following configuration parameters are taken into account:
* :ref:`consecutive_local_origin_failure<envoy_v3_api_field_config.cluster.v3.OutlierDetection.consecutive_local_origin_failure>`,
* :ref:`enforcing_consecutive_local_origin_failure<envoy_v3_api_field_config.cluster.v3.OutlierDetection.enforcing_consecutive_local_origin_failure>`
* and
* :ref:`enforcing_local_origin_success_rate<envoy_v3_api_field_config.cluster.v3.OutlierDetection.enforcing_local_origin_success_rate>`.
* Defaults to false.
*
*
* bool split_external_local_origin_errors = 12;
* @return The splitExternalLocalOriginErrors.
*/
@java.lang.Override
public boolean getSplitExternalLocalOriginErrors() {
return splitExternalLocalOriginErrors_;
}
public static final int CONSECUTIVE_LOCAL_ORIGIN_FAILURE_FIELD_NUMBER = 13;
private com.google.protobuf.UInt32Value consecutiveLocalOriginFailure_;
/**
*
* The number of consecutive locally originated failures before ejection
* occurs. Defaults to 5. Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value consecutive_local_origin_failure = 13;
* @return Whether the consecutiveLocalOriginFailure field is set.
*/
@java.lang.Override
public boolean hasConsecutiveLocalOriginFailure() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
* The number of consecutive locally originated failures before ejection
* occurs. Defaults to 5. Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value consecutive_local_origin_failure = 13;
* @return The consecutiveLocalOriginFailure.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getConsecutiveLocalOriginFailure() {
return consecutiveLocalOriginFailure_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : consecutiveLocalOriginFailure_;
}
/**
*
* The number of consecutive locally originated failures before ejection
* occurs. Defaults to 5. Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value consecutive_local_origin_failure = 13;
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getConsecutiveLocalOriginFailureOrBuilder() {
return consecutiveLocalOriginFailure_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : consecutiveLocalOriginFailure_;
}
public static final int ENFORCING_CONSECUTIVE_LOCAL_ORIGIN_FAILURE_FIELD_NUMBER = 14;
private com.google.protobuf.UInt32Value enforcingConsecutiveLocalOriginFailure_;
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive locally originated failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_local_origin_failure = 14 [(.validate.rules) = { ... }
* @return Whether the enforcingConsecutiveLocalOriginFailure field is set.
*/
@java.lang.Override
public boolean hasEnforcingConsecutiveLocalOriginFailure() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive locally originated failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_local_origin_failure = 14 [(.validate.rules) = { ... }
* @return The enforcingConsecutiveLocalOriginFailure.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getEnforcingConsecutiveLocalOriginFailure() {
return enforcingConsecutiveLocalOriginFailure_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingConsecutiveLocalOriginFailure_;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive locally originated failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_local_origin_failure = 14 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getEnforcingConsecutiveLocalOriginFailureOrBuilder() {
return enforcingConsecutiveLocalOriginFailure_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingConsecutiveLocalOriginFailure_;
}
public static final int ENFORCING_LOCAL_ORIGIN_SUCCESS_RATE_FIELD_NUMBER = 15;
private com.google.protobuf.UInt32Value enforcingLocalOriginSuccessRate_;
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics for locally originated errors.
* This setting can be used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_local_origin_success_rate = 15 [(.validate.rules) = { ... }
* @return Whether the enforcingLocalOriginSuccessRate field is set.
*/
@java.lang.Override
public boolean hasEnforcingLocalOriginSuccessRate() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics for locally originated errors.
* This setting can be used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_local_origin_success_rate = 15 [(.validate.rules) = { ... }
* @return The enforcingLocalOriginSuccessRate.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getEnforcingLocalOriginSuccessRate() {
return enforcingLocalOriginSuccessRate_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingLocalOriginSuccessRate_;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics for locally originated errors.
* This setting can be used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_local_origin_success_rate = 15 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getEnforcingLocalOriginSuccessRateOrBuilder() {
return enforcingLocalOriginSuccessRate_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingLocalOriginSuccessRate_;
}
public static final int FAILURE_PERCENTAGE_THRESHOLD_FIELD_NUMBER = 16;
private com.google.protobuf.UInt32Value failurePercentageThreshold_;
/**
*
* The failure percentage to use when determining failure percentage-based outlier detection. If
* the failure percentage of a given host is greater than or equal to this value, it will be
* ejected. Defaults to 85.
*
*
* .google.protobuf.UInt32Value failure_percentage_threshold = 16 [(.validate.rules) = { ... }
* @return Whether the failurePercentageThreshold field is set.
*/
@java.lang.Override
public boolean hasFailurePercentageThreshold() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
* The failure percentage to use when determining failure percentage-based outlier detection. If
* the failure percentage of a given host is greater than or equal to this value, it will be
* ejected. Defaults to 85.
*
*
* .google.protobuf.UInt32Value failure_percentage_threshold = 16 [(.validate.rules) = { ... }
* @return The failurePercentageThreshold.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getFailurePercentageThreshold() {
return failurePercentageThreshold_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : failurePercentageThreshold_;
}
/**
*
* The failure percentage to use when determining failure percentage-based outlier detection. If
* the failure percentage of a given host is greater than or equal to this value, it will be
* ejected. Defaults to 85.
*
*
* .google.protobuf.UInt32Value failure_percentage_threshold = 16 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getFailurePercentageThresholdOrBuilder() {
return failurePercentageThreshold_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : failurePercentageThreshold_;
}
public static final int ENFORCING_FAILURE_PERCENTAGE_FIELD_NUMBER = 17;
private com.google.protobuf.UInt32Value enforcingFailurePercentage_;
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* failure percentage statistics. This setting can be used to disable ejection or to ramp it up
* slowly. Defaults to 0.
*
* [#next-major-version: setting this without setting failure_percentage_threshold should be
* invalid in v4.]
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage = 17 [(.validate.rules) = { ... }
* @return Whether the enforcingFailurePercentage field is set.
*/
@java.lang.Override
public boolean hasEnforcingFailurePercentage() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* failure percentage statistics. This setting can be used to disable ejection or to ramp it up
* slowly. Defaults to 0.
*
* [#next-major-version: setting this without setting failure_percentage_threshold should be
* invalid in v4.]
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage = 17 [(.validate.rules) = { ... }
* @return The enforcingFailurePercentage.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getEnforcingFailurePercentage() {
return enforcingFailurePercentage_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingFailurePercentage_;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* failure percentage statistics. This setting can be used to disable ejection or to ramp it up
* slowly. Defaults to 0.
*
* [#next-major-version: setting this without setting failure_percentage_threshold should be
* invalid in v4.]
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage = 17 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getEnforcingFailurePercentageOrBuilder() {
return enforcingFailurePercentage_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingFailurePercentage_;
}
public static final int ENFORCING_FAILURE_PERCENTAGE_LOCAL_ORIGIN_FIELD_NUMBER = 18;
private com.google.protobuf.UInt32Value enforcingFailurePercentageLocalOrigin_;
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* local-origin failure percentage statistics. This setting can be used to disable ejection or to
* ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage_local_origin = 18 [(.validate.rules) = { ... }
* @return Whether the enforcingFailurePercentageLocalOrigin field is set.
*/
@java.lang.Override
public boolean hasEnforcingFailurePercentageLocalOrigin() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* local-origin failure percentage statistics. This setting can be used to disable ejection or to
* ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage_local_origin = 18 [(.validate.rules) = { ... }
* @return The enforcingFailurePercentageLocalOrigin.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getEnforcingFailurePercentageLocalOrigin() {
return enforcingFailurePercentageLocalOrigin_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingFailurePercentageLocalOrigin_;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* local-origin failure percentage statistics. This setting can be used to disable ejection or to
* ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage_local_origin = 18 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getEnforcingFailurePercentageLocalOriginOrBuilder() {
return enforcingFailurePercentageLocalOrigin_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingFailurePercentageLocalOrigin_;
}
public static final int FAILURE_PERCENTAGE_MINIMUM_HOSTS_FIELD_NUMBER = 19;
private com.google.protobuf.UInt32Value failurePercentageMinimumHosts_;
/**
*
* The minimum number of hosts in a cluster in order to perform failure percentage-based ejection.
* If the total number of hosts in the cluster is less than this value, failure percentage-based
* ejection will not be performed. Defaults to 5.
*
*
* .google.protobuf.UInt32Value failure_percentage_minimum_hosts = 19;
* @return Whether the failurePercentageMinimumHosts field is set.
*/
@java.lang.Override
public boolean hasFailurePercentageMinimumHosts() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
*
* The minimum number of hosts in a cluster in order to perform failure percentage-based ejection.
* If the total number of hosts in the cluster is less than this value, failure percentage-based
* ejection will not be performed. Defaults to 5.
*
*
* .google.protobuf.UInt32Value failure_percentage_minimum_hosts = 19;
* @return The failurePercentageMinimumHosts.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getFailurePercentageMinimumHosts() {
return failurePercentageMinimumHosts_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : failurePercentageMinimumHosts_;
}
/**
*
* The minimum number of hosts in a cluster in order to perform failure percentage-based ejection.
* If the total number of hosts in the cluster is less than this value, failure percentage-based
* ejection will not be performed. Defaults to 5.
*
*
* .google.protobuf.UInt32Value failure_percentage_minimum_hosts = 19;
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getFailurePercentageMinimumHostsOrBuilder() {
return failurePercentageMinimumHosts_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : failurePercentageMinimumHosts_;
}
public static final int FAILURE_PERCENTAGE_REQUEST_VOLUME_FIELD_NUMBER = 20;
private com.google.protobuf.UInt32Value failurePercentageRequestVolume_;
/**
*
* The minimum number of total requests that must be collected in one interval (as defined by the
* interval duration above) to perform failure percentage-based ejection for this host. If the
* volume is lower than this setting, failure percentage-based ejection will not be performed for
* this host. Defaults to 50.
*
*
* .google.protobuf.UInt32Value failure_percentage_request_volume = 20;
* @return Whether the failurePercentageRequestVolume field is set.
*/
@java.lang.Override
public boolean hasFailurePercentageRequestVolume() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
*
* The minimum number of total requests that must be collected in one interval (as defined by the
* interval duration above) to perform failure percentage-based ejection for this host. If the
* volume is lower than this setting, failure percentage-based ejection will not be performed for
* this host. Defaults to 50.
*
*
* .google.protobuf.UInt32Value failure_percentage_request_volume = 20;
* @return The failurePercentageRequestVolume.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getFailurePercentageRequestVolume() {
return failurePercentageRequestVolume_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : failurePercentageRequestVolume_;
}
/**
*
* The minimum number of total requests that must be collected in one interval (as defined by the
* interval duration above) to perform failure percentage-based ejection for this host. If the
* volume is lower than this setting, failure percentage-based ejection will not be performed for
* this host. Defaults to 50.
*
*
* .google.protobuf.UInt32Value failure_percentage_request_volume = 20;
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getFailurePercentageRequestVolumeOrBuilder() {
return failurePercentageRequestVolume_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : failurePercentageRequestVolume_;
}
public static final int MAX_EJECTION_TIME_FIELD_NUMBER = 21;
private com.google.protobuf.Duration maxEjectionTime_;
/**
*
* The maximum time that a host is ejected for. See :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* for more information. If not specified, the default value (300000ms or 300s) or
* :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>` value is applied, whatever is larger.
*
*
* .google.protobuf.Duration max_ejection_time = 21 [(.validate.rules) = { ... }
* @return Whether the maxEjectionTime field is set.
*/
@java.lang.Override
public boolean hasMaxEjectionTime() {
return ((bitField0_ & 0x00080000) != 0);
}
/**
*
* The maximum time that a host is ejected for. See :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* for more information. If not specified, the default value (300000ms or 300s) or
* :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>` value is applied, whatever is larger.
*
*
* .google.protobuf.Duration max_ejection_time = 21 [(.validate.rules) = { ... }
* @return The maxEjectionTime.
*/
@java.lang.Override
public com.google.protobuf.Duration getMaxEjectionTime() {
return maxEjectionTime_ == null ? com.google.protobuf.Duration.getDefaultInstance() : maxEjectionTime_;
}
/**
*
* The maximum time that a host is ejected for. See :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* for more information. If not specified, the default value (300000ms or 300s) or
* :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>` value is applied, whatever is larger.
*
*
* .google.protobuf.Duration max_ejection_time = 21 [(.validate.rules) = { ... }
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getMaxEjectionTimeOrBuilder() {
return maxEjectionTime_ == null ? com.google.protobuf.Duration.getDefaultInstance() : maxEjectionTime_;
}
public static final int MAX_EJECTION_TIME_JITTER_FIELD_NUMBER = 22;
private com.google.protobuf.Duration maxEjectionTimeJitter_;
/**
*
* The maximum amount of jitter to add to the ejection time, in order to prevent
* a 'thundering herd' effect where all proxies try to reconnect to host at the same time.
* See :ref:`max_ejection_time_jitter<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* Defaults to 0s.
*
*
* .google.protobuf.Duration max_ejection_time_jitter = 22;
* @return Whether the maxEjectionTimeJitter field is set.
*/
@java.lang.Override
public boolean hasMaxEjectionTimeJitter() {
return ((bitField0_ & 0x00100000) != 0);
}
/**
*
* The maximum amount of jitter to add to the ejection time, in order to prevent
* a 'thundering herd' effect where all proxies try to reconnect to host at the same time.
* See :ref:`max_ejection_time_jitter<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* Defaults to 0s.
*
*
* .google.protobuf.Duration max_ejection_time_jitter = 22;
* @return The maxEjectionTimeJitter.
*/
@java.lang.Override
public com.google.protobuf.Duration getMaxEjectionTimeJitter() {
return maxEjectionTimeJitter_ == null ? com.google.protobuf.Duration.getDefaultInstance() : maxEjectionTimeJitter_;
}
/**
*
* The maximum amount of jitter to add to the ejection time, in order to prevent
* a 'thundering herd' effect where all proxies try to reconnect to host at the same time.
* See :ref:`max_ejection_time_jitter<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* Defaults to 0s.
*
*
* .google.protobuf.Duration max_ejection_time_jitter = 22;
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getMaxEjectionTimeJitterOrBuilder() {
return maxEjectionTimeJitter_ == null ? com.google.protobuf.Duration.getDefaultInstance() : maxEjectionTimeJitter_;
}
public static final int SUCCESSFUL_ACTIVE_HEALTH_CHECK_UNEJECT_HOST_FIELD_NUMBER = 23;
private com.google.protobuf.BoolValue successfulActiveHealthCheckUnejectHost_;
/**
*
* If active health checking is enabled and a host is ejected by outlier detection, a successful active health check
* unejects the host by default and considers it as healthy. Unejection also clears all the outlier detection counters.
* To change this default behavior set this config to ``false`` where active health checking will not uneject the host.
* Defaults to true.
*
*
* .google.protobuf.BoolValue successful_active_health_check_uneject_host = 23;
* @return Whether the successfulActiveHealthCheckUnejectHost field is set.
*/
@java.lang.Override
public boolean hasSuccessfulActiveHealthCheckUnejectHost() {
return ((bitField0_ & 0x00200000) != 0);
}
/**
*
* If active health checking is enabled and a host is ejected by outlier detection, a successful active health check
* unejects the host by default and considers it as healthy. Unejection also clears all the outlier detection counters.
* To change this default behavior set this config to ``false`` where active health checking will not uneject the host.
* Defaults to true.
*
*
* .google.protobuf.BoolValue successful_active_health_check_uneject_host = 23;
* @return The successfulActiveHealthCheckUnejectHost.
*/
@java.lang.Override
public com.google.protobuf.BoolValue getSuccessfulActiveHealthCheckUnejectHost() {
return successfulActiveHealthCheckUnejectHost_ == null ? com.google.protobuf.BoolValue.getDefaultInstance() : successfulActiveHealthCheckUnejectHost_;
}
/**
*
* If active health checking is enabled and a host is ejected by outlier detection, a successful active health check
* unejects the host by default and considers it as healthy. Unejection also clears all the outlier detection counters.
* To change this default behavior set this config to ``false`` where active health checking will not uneject the host.
* Defaults to true.
*
*
* .google.protobuf.BoolValue successful_active_health_check_uneject_host = 23;
*/
@java.lang.Override
public com.google.protobuf.BoolValueOrBuilder getSuccessfulActiveHealthCheckUnejectHostOrBuilder() {
return successfulActiveHealthCheckUnejectHost_ == null ? com.google.protobuf.BoolValue.getDefaultInstance() : successfulActiveHealthCheckUnejectHost_;
}
public static final int MONITORS_FIELD_NUMBER = 24;
@SuppressWarnings("serial")
private java.util.List monitors_;
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
@java.lang.Override
public java.util.List getMonitorsList() {
return monitors_;
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
@java.lang.Override
public java.util.List extends io.envoyproxy.envoy.config.core.v3.TypedExtensionConfigOrBuilder>
getMonitorsOrBuilderList() {
return monitors_;
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
@java.lang.Override
public int getMonitorsCount() {
return monitors_.size();
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
@java.lang.Override
public io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig getMonitors(int index) {
return monitors_.get(index);
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
@java.lang.Override
public io.envoyproxy.envoy.config.core.v3.TypedExtensionConfigOrBuilder getMonitorsOrBuilder(
int index) {
return monitors_.get(index);
}
public static final int ALWAYS_EJECT_ONE_HOST_FIELD_NUMBER = 25;
private com.google.protobuf.BoolValue alwaysEjectOneHost_;
/**
*
* If enabled, at least one host is ejected regardless of the value of :ref:`max_ejection_percent<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_percent>`.
* Defaults to false.
*
*
* .google.protobuf.BoolValue always_eject_one_host = 25;
* @return Whether the alwaysEjectOneHost field is set.
*/
@java.lang.Override
public boolean hasAlwaysEjectOneHost() {
return ((bitField0_ & 0x00400000) != 0);
}
/**
*
* If enabled, at least one host is ejected regardless of the value of :ref:`max_ejection_percent<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_percent>`.
* Defaults to false.
*
*
* .google.protobuf.BoolValue always_eject_one_host = 25;
* @return The alwaysEjectOneHost.
*/
@java.lang.Override
public com.google.protobuf.BoolValue getAlwaysEjectOneHost() {
return alwaysEjectOneHost_ == null ? com.google.protobuf.BoolValue.getDefaultInstance() : alwaysEjectOneHost_;
}
/**
*
* If enabled, at least one host is ejected regardless of the value of :ref:`max_ejection_percent<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_percent>`.
* Defaults to false.
*
*
* .google.protobuf.BoolValue always_eject_one_host = 25;
*/
@java.lang.Override
public com.google.protobuf.BoolValueOrBuilder getAlwaysEjectOneHostOrBuilder() {
return alwaysEjectOneHost_ == null ? com.google.protobuf.BoolValue.getDefaultInstance() : alwaysEjectOneHost_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getConsecutive5Xx());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getInterval());
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(3, getBaseEjectionTime());
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(4, getMaxEjectionPercent());
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeMessage(5, getEnforcingConsecutive5Xx());
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(6, getEnforcingSuccessRate());
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeMessage(7, getSuccessRateMinimumHosts());
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeMessage(8, getSuccessRateRequestVolume());
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeMessage(9, getSuccessRateStdevFactor());
}
if (((bitField0_ & 0x00000200) != 0)) {
output.writeMessage(10, getConsecutiveGatewayFailure());
}
if (((bitField0_ & 0x00000400) != 0)) {
output.writeMessage(11, getEnforcingConsecutiveGatewayFailure());
}
if (splitExternalLocalOriginErrors_ != false) {
output.writeBool(12, splitExternalLocalOriginErrors_);
}
if (((bitField0_ & 0x00000800) != 0)) {
output.writeMessage(13, getConsecutiveLocalOriginFailure());
}
if (((bitField0_ & 0x00001000) != 0)) {
output.writeMessage(14, getEnforcingConsecutiveLocalOriginFailure());
}
if (((bitField0_ & 0x00002000) != 0)) {
output.writeMessage(15, getEnforcingLocalOriginSuccessRate());
}
if (((bitField0_ & 0x00004000) != 0)) {
output.writeMessage(16, getFailurePercentageThreshold());
}
if (((bitField0_ & 0x00008000) != 0)) {
output.writeMessage(17, getEnforcingFailurePercentage());
}
if (((bitField0_ & 0x00010000) != 0)) {
output.writeMessage(18, getEnforcingFailurePercentageLocalOrigin());
}
if (((bitField0_ & 0x00020000) != 0)) {
output.writeMessage(19, getFailurePercentageMinimumHosts());
}
if (((bitField0_ & 0x00040000) != 0)) {
output.writeMessage(20, getFailurePercentageRequestVolume());
}
if (((bitField0_ & 0x00080000) != 0)) {
output.writeMessage(21, getMaxEjectionTime());
}
if (((bitField0_ & 0x00100000) != 0)) {
output.writeMessage(22, getMaxEjectionTimeJitter());
}
if (((bitField0_ & 0x00200000) != 0)) {
output.writeMessage(23, getSuccessfulActiveHealthCheckUnejectHost());
}
for (int i = 0; i < monitors_.size(); i++) {
output.writeMessage(24, monitors_.get(i));
}
if (((bitField0_ & 0x00400000) != 0)) {
output.writeMessage(25, getAlwaysEjectOneHost());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getConsecutive5Xx());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getInterval());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getBaseEjectionTime());
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getMaxEjectionPercent());
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getEnforcingConsecutive5Xx());
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getEnforcingSuccessRate());
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getSuccessRateMinimumHosts());
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getSuccessRateRequestVolume());
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getSuccessRateStdevFactor());
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getConsecutiveGatewayFailure());
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getEnforcingConsecutiveGatewayFailure());
}
if (splitExternalLocalOriginErrors_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(12, splitExternalLocalOriginErrors_);
}
if (((bitField0_ & 0x00000800) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, getConsecutiveLocalOriginFailure());
}
if (((bitField0_ & 0x00001000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(14, getEnforcingConsecutiveLocalOriginFailure());
}
if (((bitField0_ & 0x00002000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(15, getEnforcingLocalOriginSuccessRate());
}
if (((bitField0_ & 0x00004000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(16, getFailurePercentageThreshold());
}
if (((bitField0_ & 0x00008000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(17, getEnforcingFailurePercentage());
}
if (((bitField0_ & 0x00010000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(18, getEnforcingFailurePercentageLocalOrigin());
}
if (((bitField0_ & 0x00020000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(19, getFailurePercentageMinimumHosts());
}
if (((bitField0_ & 0x00040000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(20, getFailurePercentageRequestVolume());
}
if (((bitField0_ & 0x00080000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(21, getMaxEjectionTime());
}
if (((bitField0_ & 0x00100000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(22, getMaxEjectionTimeJitter());
}
if (((bitField0_ & 0x00200000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(23, getSuccessfulActiveHealthCheckUnejectHost());
}
for (int i = 0; i < monitors_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(24, monitors_.get(i));
}
if (((bitField0_ & 0x00400000) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(25, getAlwaysEjectOneHost());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.envoyproxy.envoy.config.cluster.v3.OutlierDetection)) {
return super.equals(obj);
}
io.envoyproxy.envoy.config.cluster.v3.OutlierDetection other = (io.envoyproxy.envoy.config.cluster.v3.OutlierDetection) obj;
if (hasConsecutive5Xx() != other.hasConsecutive5Xx()) return false;
if (hasConsecutive5Xx()) {
if (!getConsecutive5Xx()
.equals(other.getConsecutive5Xx())) return false;
}
if (hasInterval() != other.hasInterval()) return false;
if (hasInterval()) {
if (!getInterval()
.equals(other.getInterval())) return false;
}
if (hasBaseEjectionTime() != other.hasBaseEjectionTime()) return false;
if (hasBaseEjectionTime()) {
if (!getBaseEjectionTime()
.equals(other.getBaseEjectionTime())) return false;
}
if (hasMaxEjectionPercent() != other.hasMaxEjectionPercent()) return false;
if (hasMaxEjectionPercent()) {
if (!getMaxEjectionPercent()
.equals(other.getMaxEjectionPercent())) return false;
}
if (hasEnforcingConsecutive5Xx() != other.hasEnforcingConsecutive5Xx()) return false;
if (hasEnforcingConsecutive5Xx()) {
if (!getEnforcingConsecutive5Xx()
.equals(other.getEnforcingConsecutive5Xx())) return false;
}
if (hasEnforcingSuccessRate() != other.hasEnforcingSuccessRate()) return false;
if (hasEnforcingSuccessRate()) {
if (!getEnforcingSuccessRate()
.equals(other.getEnforcingSuccessRate())) return false;
}
if (hasSuccessRateMinimumHosts() != other.hasSuccessRateMinimumHosts()) return false;
if (hasSuccessRateMinimumHosts()) {
if (!getSuccessRateMinimumHosts()
.equals(other.getSuccessRateMinimumHosts())) return false;
}
if (hasSuccessRateRequestVolume() != other.hasSuccessRateRequestVolume()) return false;
if (hasSuccessRateRequestVolume()) {
if (!getSuccessRateRequestVolume()
.equals(other.getSuccessRateRequestVolume())) return false;
}
if (hasSuccessRateStdevFactor() != other.hasSuccessRateStdevFactor()) return false;
if (hasSuccessRateStdevFactor()) {
if (!getSuccessRateStdevFactor()
.equals(other.getSuccessRateStdevFactor())) return false;
}
if (hasConsecutiveGatewayFailure() != other.hasConsecutiveGatewayFailure()) return false;
if (hasConsecutiveGatewayFailure()) {
if (!getConsecutiveGatewayFailure()
.equals(other.getConsecutiveGatewayFailure())) return false;
}
if (hasEnforcingConsecutiveGatewayFailure() != other.hasEnforcingConsecutiveGatewayFailure()) return false;
if (hasEnforcingConsecutiveGatewayFailure()) {
if (!getEnforcingConsecutiveGatewayFailure()
.equals(other.getEnforcingConsecutiveGatewayFailure())) return false;
}
if (getSplitExternalLocalOriginErrors()
!= other.getSplitExternalLocalOriginErrors()) return false;
if (hasConsecutiveLocalOriginFailure() != other.hasConsecutiveLocalOriginFailure()) return false;
if (hasConsecutiveLocalOriginFailure()) {
if (!getConsecutiveLocalOriginFailure()
.equals(other.getConsecutiveLocalOriginFailure())) return false;
}
if (hasEnforcingConsecutiveLocalOriginFailure() != other.hasEnforcingConsecutiveLocalOriginFailure()) return false;
if (hasEnforcingConsecutiveLocalOriginFailure()) {
if (!getEnforcingConsecutiveLocalOriginFailure()
.equals(other.getEnforcingConsecutiveLocalOriginFailure())) return false;
}
if (hasEnforcingLocalOriginSuccessRate() != other.hasEnforcingLocalOriginSuccessRate()) return false;
if (hasEnforcingLocalOriginSuccessRate()) {
if (!getEnforcingLocalOriginSuccessRate()
.equals(other.getEnforcingLocalOriginSuccessRate())) return false;
}
if (hasFailurePercentageThreshold() != other.hasFailurePercentageThreshold()) return false;
if (hasFailurePercentageThreshold()) {
if (!getFailurePercentageThreshold()
.equals(other.getFailurePercentageThreshold())) return false;
}
if (hasEnforcingFailurePercentage() != other.hasEnforcingFailurePercentage()) return false;
if (hasEnforcingFailurePercentage()) {
if (!getEnforcingFailurePercentage()
.equals(other.getEnforcingFailurePercentage())) return false;
}
if (hasEnforcingFailurePercentageLocalOrigin() != other.hasEnforcingFailurePercentageLocalOrigin()) return false;
if (hasEnforcingFailurePercentageLocalOrigin()) {
if (!getEnforcingFailurePercentageLocalOrigin()
.equals(other.getEnforcingFailurePercentageLocalOrigin())) return false;
}
if (hasFailurePercentageMinimumHosts() != other.hasFailurePercentageMinimumHosts()) return false;
if (hasFailurePercentageMinimumHosts()) {
if (!getFailurePercentageMinimumHosts()
.equals(other.getFailurePercentageMinimumHosts())) return false;
}
if (hasFailurePercentageRequestVolume() != other.hasFailurePercentageRequestVolume()) return false;
if (hasFailurePercentageRequestVolume()) {
if (!getFailurePercentageRequestVolume()
.equals(other.getFailurePercentageRequestVolume())) return false;
}
if (hasMaxEjectionTime() != other.hasMaxEjectionTime()) return false;
if (hasMaxEjectionTime()) {
if (!getMaxEjectionTime()
.equals(other.getMaxEjectionTime())) return false;
}
if (hasMaxEjectionTimeJitter() != other.hasMaxEjectionTimeJitter()) return false;
if (hasMaxEjectionTimeJitter()) {
if (!getMaxEjectionTimeJitter()
.equals(other.getMaxEjectionTimeJitter())) return false;
}
if (hasSuccessfulActiveHealthCheckUnejectHost() != other.hasSuccessfulActiveHealthCheckUnejectHost()) return false;
if (hasSuccessfulActiveHealthCheckUnejectHost()) {
if (!getSuccessfulActiveHealthCheckUnejectHost()
.equals(other.getSuccessfulActiveHealthCheckUnejectHost())) return false;
}
if (!getMonitorsList()
.equals(other.getMonitorsList())) return false;
if (hasAlwaysEjectOneHost() != other.hasAlwaysEjectOneHost()) return false;
if (hasAlwaysEjectOneHost()) {
if (!getAlwaysEjectOneHost()
.equals(other.getAlwaysEjectOneHost())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasConsecutive5Xx()) {
hash = (37 * hash) + CONSECUTIVE_5XX_FIELD_NUMBER;
hash = (53 * hash) + getConsecutive5Xx().hashCode();
}
if (hasInterval()) {
hash = (37 * hash) + INTERVAL_FIELD_NUMBER;
hash = (53 * hash) + getInterval().hashCode();
}
if (hasBaseEjectionTime()) {
hash = (37 * hash) + BASE_EJECTION_TIME_FIELD_NUMBER;
hash = (53 * hash) + getBaseEjectionTime().hashCode();
}
if (hasMaxEjectionPercent()) {
hash = (37 * hash) + MAX_EJECTION_PERCENT_FIELD_NUMBER;
hash = (53 * hash) + getMaxEjectionPercent().hashCode();
}
if (hasEnforcingConsecutive5Xx()) {
hash = (37 * hash) + ENFORCING_CONSECUTIVE_5XX_FIELD_NUMBER;
hash = (53 * hash) + getEnforcingConsecutive5Xx().hashCode();
}
if (hasEnforcingSuccessRate()) {
hash = (37 * hash) + ENFORCING_SUCCESS_RATE_FIELD_NUMBER;
hash = (53 * hash) + getEnforcingSuccessRate().hashCode();
}
if (hasSuccessRateMinimumHosts()) {
hash = (37 * hash) + SUCCESS_RATE_MINIMUM_HOSTS_FIELD_NUMBER;
hash = (53 * hash) + getSuccessRateMinimumHosts().hashCode();
}
if (hasSuccessRateRequestVolume()) {
hash = (37 * hash) + SUCCESS_RATE_REQUEST_VOLUME_FIELD_NUMBER;
hash = (53 * hash) + getSuccessRateRequestVolume().hashCode();
}
if (hasSuccessRateStdevFactor()) {
hash = (37 * hash) + SUCCESS_RATE_STDEV_FACTOR_FIELD_NUMBER;
hash = (53 * hash) + getSuccessRateStdevFactor().hashCode();
}
if (hasConsecutiveGatewayFailure()) {
hash = (37 * hash) + CONSECUTIVE_GATEWAY_FAILURE_FIELD_NUMBER;
hash = (53 * hash) + getConsecutiveGatewayFailure().hashCode();
}
if (hasEnforcingConsecutiveGatewayFailure()) {
hash = (37 * hash) + ENFORCING_CONSECUTIVE_GATEWAY_FAILURE_FIELD_NUMBER;
hash = (53 * hash) + getEnforcingConsecutiveGatewayFailure().hashCode();
}
hash = (37 * hash) + SPLIT_EXTERNAL_LOCAL_ORIGIN_ERRORS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSplitExternalLocalOriginErrors());
if (hasConsecutiveLocalOriginFailure()) {
hash = (37 * hash) + CONSECUTIVE_LOCAL_ORIGIN_FAILURE_FIELD_NUMBER;
hash = (53 * hash) + getConsecutiveLocalOriginFailure().hashCode();
}
if (hasEnforcingConsecutiveLocalOriginFailure()) {
hash = (37 * hash) + ENFORCING_CONSECUTIVE_LOCAL_ORIGIN_FAILURE_FIELD_NUMBER;
hash = (53 * hash) + getEnforcingConsecutiveLocalOriginFailure().hashCode();
}
if (hasEnforcingLocalOriginSuccessRate()) {
hash = (37 * hash) + ENFORCING_LOCAL_ORIGIN_SUCCESS_RATE_FIELD_NUMBER;
hash = (53 * hash) + getEnforcingLocalOriginSuccessRate().hashCode();
}
if (hasFailurePercentageThreshold()) {
hash = (37 * hash) + FAILURE_PERCENTAGE_THRESHOLD_FIELD_NUMBER;
hash = (53 * hash) + getFailurePercentageThreshold().hashCode();
}
if (hasEnforcingFailurePercentage()) {
hash = (37 * hash) + ENFORCING_FAILURE_PERCENTAGE_FIELD_NUMBER;
hash = (53 * hash) + getEnforcingFailurePercentage().hashCode();
}
if (hasEnforcingFailurePercentageLocalOrigin()) {
hash = (37 * hash) + ENFORCING_FAILURE_PERCENTAGE_LOCAL_ORIGIN_FIELD_NUMBER;
hash = (53 * hash) + getEnforcingFailurePercentageLocalOrigin().hashCode();
}
if (hasFailurePercentageMinimumHosts()) {
hash = (37 * hash) + FAILURE_PERCENTAGE_MINIMUM_HOSTS_FIELD_NUMBER;
hash = (53 * hash) + getFailurePercentageMinimumHosts().hashCode();
}
if (hasFailurePercentageRequestVolume()) {
hash = (37 * hash) + FAILURE_PERCENTAGE_REQUEST_VOLUME_FIELD_NUMBER;
hash = (53 * hash) + getFailurePercentageRequestVolume().hashCode();
}
if (hasMaxEjectionTime()) {
hash = (37 * hash) + MAX_EJECTION_TIME_FIELD_NUMBER;
hash = (53 * hash) + getMaxEjectionTime().hashCode();
}
if (hasMaxEjectionTimeJitter()) {
hash = (37 * hash) + MAX_EJECTION_TIME_JITTER_FIELD_NUMBER;
hash = (53 * hash) + getMaxEjectionTimeJitter().hashCode();
}
if (hasSuccessfulActiveHealthCheckUnejectHost()) {
hash = (37 * hash) + SUCCESSFUL_ACTIVE_HEALTH_CHECK_UNEJECT_HOST_FIELD_NUMBER;
hash = (53 * hash) + getSuccessfulActiveHealthCheckUnejectHost().hashCode();
}
if (getMonitorsCount() > 0) {
hash = (37 * hash) + MONITORS_FIELD_NUMBER;
hash = (53 * hash) + getMonitorsList().hashCode();
}
if (hasAlwaysEjectOneHost()) {
hash = (37 * hash) + ALWAYS_EJECT_ONE_HOST_FIELD_NUMBER;
hash = (53 * hash) + getAlwaysEjectOneHost().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.envoyproxy.envoy.config.cluster.v3.OutlierDetection parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.envoyproxy.envoy.config.cluster.v3.OutlierDetection parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.envoyproxy.envoy.config.cluster.v3.OutlierDetection parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.envoyproxy.envoy.config.cluster.v3.OutlierDetection parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.envoyproxy.envoy.config.cluster.v3.OutlierDetection parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.envoyproxy.envoy.config.cluster.v3.OutlierDetection parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.envoyproxy.envoy.config.cluster.v3.OutlierDetection parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.envoyproxy.envoy.config.cluster.v3.OutlierDetection parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.envoyproxy.envoy.config.cluster.v3.OutlierDetection parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.envoyproxy.envoy.config.cluster.v3.OutlierDetection parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.envoyproxy.envoy.config.cluster.v3.OutlierDetection parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.envoyproxy.envoy.config.cluster.v3.OutlierDetection parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.envoyproxy.envoy.config.cluster.v3.OutlierDetection prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* See the :ref:`architecture overview <arch_overview_outlier_detection>` for
* more information on outlier detection.
* [#next-free-field: 26]
*
*
* Protobuf type {@code envoy.config.cluster.v3.OutlierDetection}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:envoy.config.cluster.v3.OutlierDetection)
io.envoyproxy.envoy.config.cluster.v3.OutlierDetectionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.envoyproxy.envoy.config.cluster.v3.OutlierDetectionProto.internal_static_envoy_config_cluster_v3_OutlierDetection_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.envoyproxy.envoy.config.cluster.v3.OutlierDetectionProto.internal_static_envoy_config_cluster_v3_OutlierDetection_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.envoyproxy.envoy.config.cluster.v3.OutlierDetection.class, io.envoyproxy.envoy.config.cluster.v3.OutlierDetection.Builder.class);
}
// Construct using io.envoyproxy.envoy.config.cluster.v3.OutlierDetection.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getConsecutive5XxFieldBuilder();
getIntervalFieldBuilder();
getBaseEjectionTimeFieldBuilder();
getMaxEjectionPercentFieldBuilder();
getEnforcingConsecutive5XxFieldBuilder();
getEnforcingSuccessRateFieldBuilder();
getSuccessRateMinimumHostsFieldBuilder();
getSuccessRateRequestVolumeFieldBuilder();
getSuccessRateStdevFactorFieldBuilder();
getConsecutiveGatewayFailureFieldBuilder();
getEnforcingConsecutiveGatewayFailureFieldBuilder();
getConsecutiveLocalOriginFailureFieldBuilder();
getEnforcingConsecutiveLocalOriginFailureFieldBuilder();
getEnforcingLocalOriginSuccessRateFieldBuilder();
getFailurePercentageThresholdFieldBuilder();
getEnforcingFailurePercentageFieldBuilder();
getEnforcingFailurePercentageLocalOriginFieldBuilder();
getFailurePercentageMinimumHostsFieldBuilder();
getFailurePercentageRequestVolumeFieldBuilder();
getMaxEjectionTimeFieldBuilder();
getMaxEjectionTimeJitterFieldBuilder();
getSuccessfulActiveHealthCheckUnejectHostFieldBuilder();
getMonitorsFieldBuilder();
getAlwaysEjectOneHostFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
consecutive5Xx_ = null;
if (consecutive5XxBuilder_ != null) {
consecutive5XxBuilder_.dispose();
consecutive5XxBuilder_ = null;
}
interval_ = null;
if (intervalBuilder_ != null) {
intervalBuilder_.dispose();
intervalBuilder_ = null;
}
baseEjectionTime_ = null;
if (baseEjectionTimeBuilder_ != null) {
baseEjectionTimeBuilder_.dispose();
baseEjectionTimeBuilder_ = null;
}
maxEjectionPercent_ = null;
if (maxEjectionPercentBuilder_ != null) {
maxEjectionPercentBuilder_.dispose();
maxEjectionPercentBuilder_ = null;
}
enforcingConsecutive5Xx_ = null;
if (enforcingConsecutive5XxBuilder_ != null) {
enforcingConsecutive5XxBuilder_.dispose();
enforcingConsecutive5XxBuilder_ = null;
}
enforcingSuccessRate_ = null;
if (enforcingSuccessRateBuilder_ != null) {
enforcingSuccessRateBuilder_.dispose();
enforcingSuccessRateBuilder_ = null;
}
successRateMinimumHosts_ = null;
if (successRateMinimumHostsBuilder_ != null) {
successRateMinimumHostsBuilder_.dispose();
successRateMinimumHostsBuilder_ = null;
}
successRateRequestVolume_ = null;
if (successRateRequestVolumeBuilder_ != null) {
successRateRequestVolumeBuilder_.dispose();
successRateRequestVolumeBuilder_ = null;
}
successRateStdevFactor_ = null;
if (successRateStdevFactorBuilder_ != null) {
successRateStdevFactorBuilder_.dispose();
successRateStdevFactorBuilder_ = null;
}
consecutiveGatewayFailure_ = null;
if (consecutiveGatewayFailureBuilder_ != null) {
consecutiveGatewayFailureBuilder_.dispose();
consecutiveGatewayFailureBuilder_ = null;
}
enforcingConsecutiveGatewayFailure_ = null;
if (enforcingConsecutiveGatewayFailureBuilder_ != null) {
enforcingConsecutiveGatewayFailureBuilder_.dispose();
enforcingConsecutiveGatewayFailureBuilder_ = null;
}
splitExternalLocalOriginErrors_ = false;
consecutiveLocalOriginFailure_ = null;
if (consecutiveLocalOriginFailureBuilder_ != null) {
consecutiveLocalOriginFailureBuilder_.dispose();
consecutiveLocalOriginFailureBuilder_ = null;
}
enforcingConsecutiveLocalOriginFailure_ = null;
if (enforcingConsecutiveLocalOriginFailureBuilder_ != null) {
enforcingConsecutiveLocalOriginFailureBuilder_.dispose();
enforcingConsecutiveLocalOriginFailureBuilder_ = null;
}
enforcingLocalOriginSuccessRate_ = null;
if (enforcingLocalOriginSuccessRateBuilder_ != null) {
enforcingLocalOriginSuccessRateBuilder_.dispose();
enforcingLocalOriginSuccessRateBuilder_ = null;
}
failurePercentageThreshold_ = null;
if (failurePercentageThresholdBuilder_ != null) {
failurePercentageThresholdBuilder_.dispose();
failurePercentageThresholdBuilder_ = null;
}
enforcingFailurePercentage_ = null;
if (enforcingFailurePercentageBuilder_ != null) {
enforcingFailurePercentageBuilder_.dispose();
enforcingFailurePercentageBuilder_ = null;
}
enforcingFailurePercentageLocalOrigin_ = null;
if (enforcingFailurePercentageLocalOriginBuilder_ != null) {
enforcingFailurePercentageLocalOriginBuilder_.dispose();
enforcingFailurePercentageLocalOriginBuilder_ = null;
}
failurePercentageMinimumHosts_ = null;
if (failurePercentageMinimumHostsBuilder_ != null) {
failurePercentageMinimumHostsBuilder_.dispose();
failurePercentageMinimumHostsBuilder_ = null;
}
failurePercentageRequestVolume_ = null;
if (failurePercentageRequestVolumeBuilder_ != null) {
failurePercentageRequestVolumeBuilder_.dispose();
failurePercentageRequestVolumeBuilder_ = null;
}
maxEjectionTime_ = null;
if (maxEjectionTimeBuilder_ != null) {
maxEjectionTimeBuilder_.dispose();
maxEjectionTimeBuilder_ = null;
}
maxEjectionTimeJitter_ = null;
if (maxEjectionTimeJitterBuilder_ != null) {
maxEjectionTimeJitterBuilder_.dispose();
maxEjectionTimeJitterBuilder_ = null;
}
successfulActiveHealthCheckUnejectHost_ = null;
if (successfulActiveHealthCheckUnejectHostBuilder_ != null) {
successfulActiveHealthCheckUnejectHostBuilder_.dispose();
successfulActiveHealthCheckUnejectHostBuilder_ = null;
}
if (monitorsBuilder_ == null) {
monitors_ = java.util.Collections.emptyList();
} else {
monitors_ = null;
monitorsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00800000);
alwaysEjectOneHost_ = null;
if (alwaysEjectOneHostBuilder_ != null) {
alwaysEjectOneHostBuilder_.dispose();
alwaysEjectOneHostBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.envoyproxy.envoy.config.cluster.v3.OutlierDetectionProto.internal_static_envoy_config_cluster_v3_OutlierDetection_descriptor;
}
@java.lang.Override
public io.envoyproxy.envoy.config.cluster.v3.OutlierDetection getDefaultInstanceForType() {
return io.envoyproxy.envoy.config.cluster.v3.OutlierDetection.getDefaultInstance();
}
@java.lang.Override
public io.envoyproxy.envoy.config.cluster.v3.OutlierDetection build() {
io.envoyproxy.envoy.config.cluster.v3.OutlierDetection result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.envoyproxy.envoy.config.cluster.v3.OutlierDetection buildPartial() {
io.envoyproxy.envoy.config.cluster.v3.OutlierDetection result = new io.envoyproxy.envoy.config.cluster.v3.OutlierDetection(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(io.envoyproxy.envoy.config.cluster.v3.OutlierDetection result) {
if (monitorsBuilder_ == null) {
if (((bitField0_ & 0x00800000) != 0)) {
monitors_ = java.util.Collections.unmodifiableList(monitors_);
bitField0_ = (bitField0_ & ~0x00800000);
}
result.monitors_ = monitors_;
} else {
result.monitors_ = monitorsBuilder_.build();
}
}
private void buildPartial0(io.envoyproxy.envoy.config.cluster.v3.OutlierDetection result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.consecutive5Xx_ = consecutive5XxBuilder_ == null
? consecutive5Xx_
: consecutive5XxBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.interval_ = intervalBuilder_ == null
? interval_
: intervalBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.baseEjectionTime_ = baseEjectionTimeBuilder_ == null
? baseEjectionTime_
: baseEjectionTimeBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.maxEjectionPercent_ = maxEjectionPercentBuilder_ == null
? maxEjectionPercent_
: maxEjectionPercentBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.enforcingConsecutive5Xx_ = enforcingConsecutive5XxBuilder_ == null
? enforcingConsecutive5Xx_
: enforcingConsecutive5XxBuilder_.build();
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.enforcingSuccessRate_ = enforcingSuccessRateBuilder_ == null
? enforcingSuccessRate_
: enforcingSuccessRateBuilder_.build();
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.successRateMinimumHosts_ = successRateMinimumHostsBuilder_ == null
? successRateMinimumHosts_
: successRateMinimumHostsBuilder_.build();
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.successRateRequestVolume_ = successRateRequestVolumeBuilder_ == null
? successRateRequestVolume_
: successRateRequestVolumeBuilder_.build();
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.successRateStdevFactor_ = successRateStdevFactorBuilder_ == null
? successRateStdevFactor_
: successRateStdevFactorBuilder_.build();
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.consecutiveGatewayFailure_ = consecutiveGatewayFailureBuilder_ == null
? consecutiveGatewayFailure_
: consecutiveGatewayFailureBuilder_.build();
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.enforcingConsecutiveGatewayFailure_ = enforcingConsecutiveGatewayFailureBuilder_ == null
? enforcingConsecutiveGatewayFailure_
: enforcingConsecutiveGatewayFailureBuilder_.build();
to_bitField0_ |= 0x00000400;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.splitExternalLocalOriginErrors_ = splitExternalLocalOriginErrors_;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.consecutiveLocalOriginFailure_ = consecutiveLocalOriginFailureBuilder_ == null
? consecutiveLocalOriginFailure_
: consecutiveLocalOriginFailureBuilder_.build();
to_bitField0_ |= 0x00000800;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.enforcingConsecutiveLocalOriginFailure_ = enforcingConsecutiveLocalOriginFailureBuilder_ == null
? enforcingConsecutiveLocalOriginFailure_
: enforcingConsecutiveLocalOriginFailureBuilder_.build();
to_bitField0_ |= 0x00001000;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.enforcingLocalOriginSuccessRate_ = enforcingLocalOriginSuccessRateBuilder_ == null
? enforcingLocalOriginSuccessRate_
: enforcingLocalOriginSuccessRateBuilder_.build();
to_bitField0_ |= 0x00002000;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
result.failurePercentageThreshold_ = failurePercentageThresholdBuilder_ == null
? failurePercentageThreshold_
: failurePercentageThresholdBuilder_.build();
to_bitField0_ |= 0x00004000;
}
if (((from_bitField0_ & 0x00010000) != 0)) {
result.enforcingFailurePercentage_ = enforcingFailurePercentageBuilder_ == null
? enforcingFailurePercentage_
: enforcingFailurePercentageBuilder_.build();
to_bitField0_ |= 0x00008000;
}
if (((from_bitField0_ & 0x00020000) != 0)) {
result.enforcingFailurePercentageLocalOrigin_ = enforcingFailurePercentageLocalOriginBuilder_ == null
? enforcingFailurePercentageLocalOrigin_
: enforcingFailurePercentageLocalOriginBuilder_.build();
to_bitField0_ |= 0x00010000;
}
if (((from_bitField0_ & 0x00040000) != 0)) {
result.failurePercentageMinimumHosts_ = failurePercentageMinimumHostsBuilder_ == null
? failurePercentageMinimumHosts_
: failurePercentageMinimumHostsBuilder_.build();
to_bitField0_ |= 0x00020000;
}
if (((from_bitField0_ & 0x00080000) != 0)) {
result.failurePercentageRequestVolume_ = failurePercentageRequestVolumeBuilder_ == null
? failurePercentageRequestVolume_
: failurePercentageRequestVolumeBuilder_.build();
to_bitField0_ |= 0x00040000;
}
if (((from_bitField0_ & 0x00100000) != 0)) {
result.maxEjectionTime_ = maxEjectionTimeBuilder_ == null
? maxEjectionTime_
: maxEjectionTimeBuilder_.build();
to_bitField0_ |= 0x00080000;
}
if (((from_bitField0_ & 0x00200000) != 0)) {
result.maxEjectionTimeJitter_ = maxEjectionTimeJitterBuilder_ == null
? maxEjectionTimeJitter_
: maxEjectionTimeJitterBuilder_.build();
to_bitField0_ |= 0x00100000;
}
if (((from_bitField0_ & 0x00400000) != 0)) {
result.successfulActiveHealthCheckUnejectHost_ = successfulActiveHealthCheckUnejectHostBuilder_ == null
? successfulActiveHealthCheckUnejectHost_
: successfulActiveHealthCheckUnejectHostBuilder_.build();
to_bitField0_ |= 0x00200000;
}
if (((from_bitField0_ & 0x01000000) != 0)) {
result.alwaysEjectOneHost_ = alwaysEjectOneHostBuilder_ == null
? alwaysEjectOneHost_
: alwaysEjectOneHostBuilder_.build();
to_bitField0_ |= 0x00400000;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.envoyproxy.envoy.config.cluster.v3.OutlierDetection) {
return mergeFrom((io.envoyproxy.envoy.config.cluster.v3.OutlierDetection)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.envoyproxy.envoy.config.cluster.v3.OutlierDetection other) {
if (other == io.envoyproxy.envoy.config.cluster.v3.OutlierDetection.getDefaultInstance()) return this;
if (other.hasConsecutive5Xx()) {
mergeConsecutive5Xx(other.getConsecutive5Xx());
}
if (other.hasInterval()) {
mergeInterval(other.getInterval());
}
if (other.hasBaseEjectionTime()) {
mergeBaseEjectionTime(other.getBaseEjectionTime());
}
if (other.hasMaxEjectionPercent()) {
mergeMaxEjectionPercent(other.getMaxEjectionPercent());
}
if (other.hasEnforcingConsecutive5Xx()) {
mergeEnforcingConsecutive5Xx(other.getEnforcingConsecutive5Xx());
}
if (other.hasEnforcingSuccessRate()) {
mergeEnforcingSuccessRate(other.getEnforcingSuccessRate());
}
if (other.hasSuccessRateMinimumHosts()) {
mergeSuccessRateMinimumHosts(other.getSuccessRateMinimumHosts());
}
if (other.hasSuccessRateRequestVolume()) {
mergeSuccessRateRequestVolume(other.getSuccessRateRequestVolume());
}
if (other.hasSuccessRateStdevFactor()) {
mergeSuccessRateStdevFactor(other.getSuccessRateStdevFactor());
}
if (other.hasConsecutiveGatewayFailure()) {
mergeConsecutiveGatewayFailure(other.getConsecutiveGatewayFailure());
}
if (other.hasEnforcingConsecutiveGatewayFailure()) {
mergeEnforcingConsecutiveGatewayFailure(other.getEnforcingConsecutiveGatewayFailure());
}
if (other.getSplitExternalLocalOriginErrors() != false) {
setSplitExternalLocalOriginErrors(other.getSplitExternalLocalOriginErrors());
}
if (other.hasConsecutiveLocalOriginFailure()) {
mergeConsecutiveLocalOriginFailure(other.getConsecutiveLocalOriginFailure());
}
if (other.hasEnforcingConsecutiveLocalOriginFailure()) {
mergeEnforcingConsecutiveLocalOriginFailure(other.getEnforcingConsecutiveLocalOriginFailure());
}
if (other.hasEnforcingLocalOriginSuccessRate()) {
mergeEnforcingLocalOriginSuccessRate(other.getEnforcingLocalOriginSuccessRate());
}
if (other.hasFailurePercentageThreshold()) {
mergeFailurePercentageThreshold(other.getFailurePercentageThreshold());
}
if (other.hasEnforcingFailurePercentage()) {
mergeEnforcingFailurePercentage(other.getEnforcingFailurePercentage());
}
if (other.hasEnforcingFailurePercentageLocalOrigin()) {
mergeEnforcingFailurePercentageLocalOrigin(other.getEnforcingFailurePercentageLocalOrigin());
}
if (other.hasFailurePercentageMinimumHosts()) {
mergeFailurePercentageMinimumHosts(other.getFailurePercentageMinimumHosts());
}
if (other.hasFailurePercentageRequestVolume()) {
mergeFailurePercentageRequestVolume(other.getFailurePercentageRequestVolume());
}
if (other.hasMaxEjectionTime()) {
mergeMaxEjectionTime(other.getMaxEjectionTime());
}
if (other.hasMaxEjectionTimeJitter()) {
mergeMaxEjectionTimeJitter(other.getMaxEjectionTimeJitter());
}
if (other.hasSuccessfulActiveHealthCheckUnejectHost()) {
mergeSuccessfulActiveHealthCheckUnejectHost(other.getSuccessfulActiveHealthCheckUnejectHost());
}
if (monitorsBuilder_ == null) {
if (!other.monitors_.isEmpty()) {
if (monitors_.isEmpty()) {
monitors_ = other.monitors_;
bitField0_ = (bitField0_ & ~0x00800000);
} else {
ensureMonitorsIsMutable();
monitors_.addAll(other.monitors_);
}
onChanged();
}
} else {
if (!other.monitors_.isEmpty()) {
if (monitorsBuilder_.isEmpty()) {
monitorsBuilder_.dispose();
monitorsBuilder_ = null;
monitors_ = other.monitors_;
bitField0_ = (bitField0_ & ~0x00800000);
monitorsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getMonitorsFieldBuilder() : null;
} else {
monitorsBuilder_.addAllMessages(other.monitors_);
}
}
}
if (other.hasAlwaysEjectOneHost()) {
mergeAlwaysEjectOneHost(other.getAlwaysEjectOneHost());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
input.readMessage(
getConsecutive5XxFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
input.readMessage(
getIntervalFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
input.readMessage(
getBaseEjectionTimeFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
input.readMessage(
getMaxEjectionPercentFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
case 42: {
input.readMessage(
getEnforcingConsecutive5XxFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000010;
break;
} // case 42
case 50: {
input.readMessage(
getEnforcingSuccessRateFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000020;
break;
} // case 50
case 58: {
input.readMessage(
getSuccessRateMinimumHostsFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000040;
break;
} // case 58
case 66: {
input.readMessage(
getSuccessRateRequestVolumeFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000080;
break;
} // case 66
case 74: {
input.readMessage(
getSuccessRateStdevFactorFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000100;
break;
} // case 74
case 82: {
input.readMessage(
getConsecutiveGatewayFailureFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000200;
break;
} // case 82
case 90: {
input.readMessage(
getEnforcingConsecutiveGatewayFailureFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000400;
break;
} // case 90
case 96: {
splitExternalLocalOriginErrors_ = input.readBool();
bitField0_ |= 0x00000800;
break;
} // case 96
case 106: {
input.readMessage(
getConsecutiveLocalOriginFailureFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00001000;
break;
} // case 106
case 114: {
input.readMessage(
getEnforcingConsecutiveLocalOriginFailureFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00002000;
break;
} // case 114
case 122: {
input.readMessage(
getEnforcingLocalOriginSuccessRateFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00004000;
break;
} // case 122
case 130: {
input.readMessage(
getFailurePercentageThresholdFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00008000;
break;
} // case 130
case 138: {
input.readMessage(
getEnforcingFailurePercentageFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00010000;
break;
} // case 138
case 146: {
input.readMessage(
getEnforcingFailurePercentageLocalOriginFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00020000;
break;
} // case 146
case 154: {
input.readMessage(
getFailurePercentageMinimumHostsFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00040000;
break;
} // case 154
case 162: {
input.readMessage(
getFailurePercentageRequestVolumeFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00080000;
break;
} // case 162
case 170: {
input.readMessage(
getMaxEjectionTimeFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00100000;
break;
} // case 170
case 178: {
input.readMessage(
getMaxEjectionTimeJitterFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00200000;
break;
} // case 178
case 186: {
input.readMessage(
getSuccessfulActiveHealthCheckUnejectHostFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00400000;
break;
} // case 186
case 194: {
io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig m =
input.readMessage(
io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig.parser(),
extensionRegistry);
if (monitorsBuilder_ == null) {
ensureMonitorsIsMutable();
monitors_.add(m);
} else {
monitorsBuilder_.addMessage(m);
}
break;
} // case 194
case 202: {
input.readMessage(
getAlwaysEjectOneHostFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x01000000;
break;
} // case 202
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.UInt32Value consecutive5Xx_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> consecutive5XxBuilder_;
/**
*
* The number of consecutive server-side error responses (for HTTP traffic,
* 5xx responses; for TCP traffic, connection failures; for Redis, failure to
* respond PONG; etc.) before a consecutive 5xx ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_5xx = 1;
* @return Whether the consecutive5xx field is set.
*/
public boolean hasConsecutive5Xx() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The number of consecutive server-side error responses (for HTTP traffic,
* 5xx responses; for TCP traffic, connection failures; for Redis, failure to
* respond PONG; etc.) before a consecutive 5xx ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_5xx = 1;
* @return The consecutive5xx.
*/
public com.google.protobuf.UInt32Value getConsecutive5Xx() {
if (consecutive5XxBuilder_ == null) {
return consecutive5Xx_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : consecutive5Xx_;
} else {
return consecutive5XxBuilder_.getMessage();
}
}
/**
*
* The number of consecutive server-side error responses (for HTTP traffic,
* 5xx responses; for TCP traffic, connection failures; for Redis, failure to
* respond PONG; etc.) before a consecutive 5xx ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_5xx = 1;
*/
public Builder setConsecutive5Xx(com.google.protobuf.UInt32Value value) {
if (consecutive5XxBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
consecutive5Xx_ = value;
} else {
consecutive5XxBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The number of consecutive server-side error responses (for HTTP traffic,
* 5xx responses; for TCP traffic, connection failures; for Redis, failure to
* respond PONG; etc.) before a consecutive 5xx ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_5xx = 1;
*/
public Builder setConsecutive5Xx(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (consecutive5XxBuilder_ == null) {
consecutive5Xx_ = builderForValue.build();
} else {
consecutive5XxBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The number of consecutive server-side error responses (for HTTP traffic,
* 5xx responses; for TCP traffic, connection failures; for Redis, failure to
* respond PONG; etc.) before a consecutive 5xx ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_5xx = 1;
*/
public Builder mergeConsecutive5Xx(com.google.protobuf.UInt32Value value) {
if (consecutive5XxBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
consecutive5Xx_ != null &&
consecutive5Xx_ != com.google.protobuf.UInt32Value.getDefaultInstance()) {
getConsecutive5XxBuilder().mergeFrom(value);
} else {
consecutive5Xx_ = value;
}
} else {
consecutive5XxBuilder_.mergeFrom(value);
}
if (consecutive5Xx_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
*
* The number of consecutive server-side error responses (for HTTP traffic,
* 5xx responses; for TCP traffic, connection failures; for Redis, failure to
* respond PONG; etc.) before a consecutive 5xx ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_5xx = 1;
*/
public Builder clearConsecutive5Xx() {
bitField0_ = (bitField0_ & ~0x00000001);
consecutive5Xx_ = null;
if (consecutive5XxBuilder_ != null) {
consecutive5XxBuilder_.dispose();
consecutive5XxBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The number of consecutive server-side error responses (for HTTP traffic,
* 5xx responses; for TCP traffic, connection failures; for Redis, failure to
* respond PONG; etc.) before a consecutive 5xx ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_5xx = 1;
*/
public com.google.protobuf.UInt32Value.Builder getConsecutive5XxBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getConsecutive5XxFieldBuilder().getBuilder();
}
/**
*
* The number of consecutive server-side error responses (for HTTP traffic,
* 5xx responses; for TCP traffic, connection failures; for Redis, failure to
* respond PONG; etc.) before a consecutive 5xx ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_5xx = 1;
*/
public com.google.protobuf.UInt32ValueOrBuilder getConsecutive5XxOrBuilder() {
if (consecutive5XxBuilder_ != null) {
return consecutive5XxBuilder_.getMessageOrBuilder();
} else {
return consecutive5Xx_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : consecutive5Xx_;
}
}
/**
*
* The number of consecutive server-side error responses (for HTTP traffic,
* 5xx responses; for TCP traffic, connection failures; for Redis, failure to
* respond PONG; etc.) before a consecutive 5xx ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_5xx = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getConsecutive5XxFieldBuilder() {
if (consecutive5XxBuilder_ == null) {
consecutive5XxBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getConsecutive5Xx(),
getParentForChildren(),
isClean());
consecutive5Xx_ = null;
}
return consecutive5XxBuilder_;
}
private com.google.protobuf.Duration interval_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder> intervalBuilder_;
/**
*
* The time interval between ejection analysis sweeps. This can result in
* both new ejections as well as hosts being returned to service. Defaults
* to 10000ms or 10s.
*
*
* .google.protobuf.Duration interval = 2 [(.validate.rules) = { ... }
* @return Whether the interval field is set.
*/
public boolean hasInterval() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The time interval between ejection analysis sweeps. This can result in
* both new ejections as well as hosts being returned to service. Defaults
* to 10000ms or 10s.
*
*
* .google.protobuf.Duration interval = 2 [(.validate.rules) = { ... }
* @return The interval.
*/
public com.google.protobuf.Duration getInterval() {
if (intervalBuilder_ == null) {
return interval_ == null ? com.google.protobuf.Duration.getDefaultInstance() : interval_;
} else {
return intervalBuilder_.getMessage();
}
}
/**
*
* The time interval between ejection analysis sweeps. This can result in
* both new ejections as well as hosts being returned to service. Defaults
* to 10000ms or 10s.
*
*
* .google.protobuf.Duration interval = 2 [(.validate.rules) = { ... }
*/
public Builder setInterval(com.google.protobuf.Duration value) {
if (intervalBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
interval_ = value;
} else {
intervalBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The time interval between ejection analysis sweeps. This can result in
* both new ejections as well as hosts being returned to service. Defaults
* to 10000ms or 10s.
*
*
* .google.protobuf.Duration interval = 2 [(.validate.rules) = { ... }
*/
public Builder setInterval(
com.google.protobuf.Duration.Builder builderForValue) {
if (intervalBuilder_ == null) {
interval_ = builderForValue.build();
} else {
intervalBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The time interval between ejection analysis sweeps. This can result in
* both new ejections as well as hosts being returned to service. Defaults
* to 10000ms or 10s.
*
*
* .google.protobuf.Duration interval = 2 [(.validate.rules) = { ... }
*/
public Builder mergeInterval(com.google.protobuf.Duration value) {
if (intervalBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
interval_ != null &&
interval_ != com.google.protobuf.Duration.getDefaultInstance()) {
getIntervalBuilder().mergeFrom(value);
} else {
interval_ = value;
}
} else {
intervalBuilder_.mergeFrom(value);
}
if (interval_ != null) {
bitField0_ |= 0x00000002;
onChanged();
}
return this;
}
/**
*
* The time interval between ejection analysis sweeps. This can result in
* both new ejections as well as hosts being returned to service. Defaults
* to 10000ms or 10s.
*
*
* .google.protobuf.Duration interval = 2 [(.validate.rules) = { ... }
*/
public Builder clearInterval() {
bitField0_ = (bitField0_ & ~0x00000002);
interval_ = null;
if (intervalBuilder_ != null) {
intervalBuilder_.dispose();
intervalBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The time interval between ejection analysis sweeps. This can result in
* both new ejections as well as hosts being returned to service. Defaults
* to 10000ms or 10s.
*
*
* .google.protobuf.Duration interval = 2 [(.validate.rules) = { ... }
*/
public com.google.protobuf.Duration.Builder getIntervalBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getIntervalFieldBuilder().getBuilder();
}
/**
*
* The time interval between ejection analysis sweeps. This can result in
* both new ejections as well as hosts being returned to service. Defaults
* to 10000ms or 10s.
*
*
* .google.protobuf.Duration interval = 2 [(.validate.rules) = { ... }
*/
public com.google.protobuf.DurationOrBuilder getIntervalOrBuilder() {
if (intervalBuilder_ != null) {
return intervalBuilder_.getMessageOrBuilder();
} else {
return interval_ == null ?
com.google.protobuf.Duration.getDefaultInstance() : interval_;
}
}
/**
*
* The time interval between ejection analysis sweeps. This can result in
* both new ejections as well as hosts being returned to service. Defaults
* to 10000ms or 10s.
*
*
* .google.protobuf.Duration interval = 2 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>
getIntervalFieldBuilder() {
if (intervalBuilder_ == null) {
intervalBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>(
getInterval(),
getParentForChildren(),
isClean());
interval_ = null;
}
return intervalBuilder_;
}
private com.google.protobuf.Duration baseEjectionTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder> baseEjectionTimeBuilder_;
/**
*
* The base time that a host is ejected for. The real time is equal to the
* base time multiplied by the number of times the host has been ejected and is
* capped by :ref:`max_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_time>`.
* Defaults to 30000ms or 30s.
*
*
* .google.protobuf.Duration base_ejection_time = 3 [(.validate.rules) = { ... }
* @return Whether the baseEjectionTime field is set.
*/
public boolean hasBaseEjectionTime() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The base time that a host is ejected for. The real time is equal to the
* base time multiplied by the number of times the host has been ejected and is
* capped by :ref:`max_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_time>`.
* Defaults to 30000ms or 30s.
*
*
* .google.protobuf.Duration base_ejection_time = 3 [(.validate.rules) = { ... }
* @return The baseEjectionTime.
*/
public com.google.protobuf.Duration getBaseEjectionTime() {
if (baseEjectionTimeBuilder_ == null) {
return baseEjectionTime_ == null ? com.google.protobuf.Duration.getDefaultInstance() : baseEjectionTime_;
} else {
return baseEjectionTimeBuilder_.getMessage();
}
}
/**
*
* The base time that a host is ejected for. The real time is equal to the
* base time multiplied by the number of times the host has been ejected and is
* capped by :ref:`max_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_time>`.
* Defaults to 30000ms or 30s.
*
*
* .google.protobuf.Duration base_ejection_time = 3 [(.validate.rules) = { ... }
*/
public Builder setBaseEjectionTime(com.google.protobuf.Duration value) {
if (baseEjectionTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
baseEjectionTime_ = value;
} else {
baseEjectionTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* The base time that a host is ejected for. The real time is equal to the
* base time multiplied by the number of times the host has been ejected and is
* capped by :ref:`max_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_time>`.
* Defaults to 30000ms or 30s.
*
*
* .google.protobuf.Duration base_ejection_time = 3 [(.validate.rules) = { ... }
*/
public Builder setBaseEjectionTime(
com.google.protobuf.Duration.Builder builderForValue) {
if (baseEjectionTimeBuilder_ == null) {
baseEjectionTime_ = builderForValue.build();
} else {
baseEjectionTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* The base time that a host is ejected for. The real time is equal to the
* base time multiplied by the number of times the host has been ejected and is
* capped by :ref:`max_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_time>`.
* Defaults to 30000ms or 30s.
*
*
* .google.protobuf.Duration base_ejection_time = 3 [(.validate.rules) = { ... }
*/
public Builder mergeBaseEjectionTime(com.google.protobuf.Duration value) {
if (baseEjectionTimeBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0) &&
baseEjectionTime_ != null &&
baseEjectionTime_ != com.google.protobuf.Duration.getDefaultInstance()) {
getBaseEjectionTimeBuilder().mergeFrom(value);
} else {
baseEjectionTime_ = value;
}
} else {
baseEjectionTimeBuilder_.mergeFrom(value);
}
if (baseEjectionTime_ != null) {
bitField0_ |= 0x00000004;
onChanged();
}
return this;
}
/**
*
* The base time that a host is ejected for. The real time is equal to the
* base time multiplied by the number of times the host has been ejected and is
* capped by :ref:`max_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_time>`.
* Defaults to 30000ms or 30s.
*
*
* .google.protobuf.Duration base_ejection_time = 3 [(.validate.rules) = { ... }
*/
public Builder clearBaseEjectionTime() {
bitField0_ = (bitField0_ & ~0x00000004);
baseEjectionTime_ = null;
if (baseEjectionTimeBuilder_ != null) {
baseEjectionTimeBuilder_.dispose();
baseEjectionTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The base time that a host is ejected for. The real time is equal to the
* base time multiplied by the number of times the host has been ejected and is
* capped by :ref:`max_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_time>`.
* Defaults to 30000ms or 30s.
*
*
* .google.protobuf.Duration base_ejection_time = 3 [(.validate.rules) = { ... }
*/
public com.google.protobuf.Duration.Builder getBaseEjectionTimeBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getBaseEjectionTimeFieldBuilder().getBuilder();
}
/**
*
* The base time that a host is ejected for. The real time is equal to the
* base time multiplied by the number of times the host has been ejected and is
* capped by :ref:`max_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_time>`.
* Defaults to 30000ms or 30s.
*
*
* .google.protobuf.Duration base_ejection_time = 3 [(.validate.rules) = { ... }
*/
public com.google.protobuf.DurationOrBuilder getBaseEjectionTimeOrBuilder() {
if (baseEjectionTimeBuilder_ != null) {
return baseEjectionTimeBuilder_.getMessageOrBuilder();
} else {
return baseEjectionTime_ == null ?
com.google.protobuf.Duration.getDefaultInstance() : baseEjectionTime_;
}
}
/**
*
* The base time that a host is ejected for. The real time is equal to the
* base time multiplied by the number of times the host has been ejected and is
* capped by :ref:`max_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_time>`.
* Defaults to 30000ms or 30s.
*
*
* .google.protobuf.Duration base_ejection_time = 3 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>
getBaseEjectionTimeFieldBuilder() {
if (baseEjectionTimeBuilder_ == null) {
baseEjectionTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>(
getBaseEjectionTime(),
getParentForChildren(),
isClean());
baseEjectionTime_ = null;
}
return baseEjectionTimeBuilder_;
}
private com.google.protobuf.UInt32Value maxEjectionPercent_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> maxEjectionPercentBuilder_;
/**
*
* The maximum % of an upstream cluster that can be ejected due to outlier detection. Defaults to 10% .
* Will eject at least one host regardless of the value if :ref:`always_eject_one_host<envoy_v3_api_field_config.cluster.v3.OutlierDetection.always_eject_one_host>` is enabled.
*
*
* .google.protobuf.UInt32Value max_ejection_percent = 4 [(.validate.rules) = { ... }
* @return Whether the maxEjectionPercent field is set.
*/
public boolean hasMaxEjectionPercent() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The maximum % of an upstream cluster that can be ejected due to outlier detection. Defaults to 10% .
* Will eject at least one host regardless of the value if :ref:`always_eject_one_host<envoy_v3_api_field_config.cluster.v3.OutlierDetection.always_eject_one_host>` is enabled.
*
*
* .google.protobuf.UInt32Value max_ejection_percent = 4 [(.validate.rules) = { ... }
* @return The maxEjectionPercent.
*/
public com.google.protobuf.UInt32Value getMaxEjectionPercent() {
if (maxEjectionPercentBuilder_ == null) {
return maxEjectionPercent_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : maxEjectionPercent_;
} else {
return maxEjectionPercentBuilder_.getMessage();
}
}
/**
*
* The maximum % of an upstream cluster that can be ejected due to outlier detection. Defaults to 10% .
* Will eject at least one host regardless of the value if :ref:`always_eject_one_host<envoy_v3_api_field_config.cluster.v3.OutlierDetection.always_eject_one_host>` is enabled.
*
*
* .google.protobuf.UInt32Value max_ejection_percent = 4 [(.validate.rules) = { ... }
*/
public Builder setMaxEjectionPercent(com.google.protobuf.UInt32Value value) {
if (maxEjectionPercentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
maxEjectionPercent_ = value;
} else {
maxEjectionPercentBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* The maximum % of an upstream cluster that can be ejected due to outlier detection. Defaults to 10% .
* Will eject at least one host regardless of the value if :ref:`always_eject_one_host<envoy_v3_api_field_config.cluster.v3.OutlierDetection.always_eject_one_host>` is enabled.
*
*
* .google.protobuf.UInt32Value max_ejection_percent = 4 [(.validate.rules) = { ... }
*/
public Builder setMaxEjectionPercent(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (maxEjectionPercentBuilder_ == null) {
maxEjectionPercent_ = builderForValue.build();
} else {
maxEjectionPercentBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* The maximum % of an upstream cluster that can be ejected due to outlier detection. Defaults to 10% .
* Will eject at least one host regardless of the value if :ref:`always_eject_one_host<envoy_v3_api_field_config.cluster.v3.OutlierDetection.always_eject_one_host>` is enabled.
*
*
* .google.protobuf.UInt32Value max_ejection_percent = 4 [(.validate.rules) = { ... }
*/
public Builder mergeMaxEjectionPercent(com.google.protobuf.UInt32Value value) {
if (maxEjectionPercentBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
maxEjectionPercent_ != null &&
maxEjectionPercent_ != com.google.protobuf.UInt32Value.getDefaultInstance()) {
getMaxEjectionPercentBuilder().mergeFrom(value);
} else {
maxEjectionPercent_ = value;
}
} else {
maxEjectionPercentBuilder_.mergeFrom(value);
}
if (maxEjectionPercent_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
* The maximum % of an upstream cluster that can be ejected due to outlier detection. Defaults to 10% .
* Will eject at least one host regardless of the value if :ref:`always_eject_one_host<envoy_v3_api_field_config.cluster.v3.OutlierDetection.always_eject_one_host>` is enabled.
*
*
* .google.protobuf.UInt32Value max_ejection_percent = 4 [(.validate.rules) = { ... }
*/
public Builder clearMaxEjectionPercent() {
bitField0_ = (bitField0_ & ~0x00000008);
maxEjectionPercent_ = null;
if (maxEjectionPercentBuilder_ != null) {
maxEjectionPercentBuilder_.dispose();
maxEjectionPercentBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The maximum % of an upstream cluster that can be ejected due to outlier detection. Defaults to 10% .
* Will eject at least one host regardless of the value if :ref:`always_eject_one_host<envoy_v3_api_field_config.cluster.v3.OutlierDetection.always_eject_one_host>` is enabled.
*
*
* .google.protobuf.UInt32Value max_ejection_percent = 4 [(.validate.rules) = { ... }
*/
public com.google.protobuf.UInt32Value.Builder getMaxEjectionPercentBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getMaxEjectionPercentFieldBuilder().getBuilder();
}
/**
*
* The maximum % of an upstream cluster that can be ejected due to outlier detection. Defaults to 10% .
* Will eject at least one host regardless of the value if :ref:`always_eject_one_host<envoy_v3_api_field_config.cluster.v3.OutlierDetection.always_eject_one_host>` is enabled.
*
*
* .google.protobuf.UInt32Value max_ejection_percent = 4 [(.validate.rules) = { ... }
*/
public com.google.protobuf.UInt32ValueOrBuilder getMaxEjectionPercentOrBuilder() {
if (maxEjectionPercentBuilder_ != null) {
return maxEjectionPercentBuilder_.getMessageOrBuilder();
} else {
return maxEjectionPercent_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : maxEjectionPercent_;
}
}
/**
*
* The maximum % of an upstream cluster that can be ejected due to outlier detection. Defaults to 10% .
* Will eject at least one host regardless of the value if :ref:`always_eject_one_host<envoy_v3_api_field_config.cluster.v3.OutlierDetection.always_eject_one_host>` is enabled.
*
*
* .google.protobuf.UInt32Value max_ejection_percent = 4 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getMaxEjectionPercentFieldBuilder() {
if (maxEjectionPercentBuilder_ == null) {
maxEjectionPercentBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getMaxEjectionPercent(),
getParentForChildren(),
isClean());
maxEjectionPercent_ = null;
}
return maxEjectionPercentBuilder_;
}
private com.google.protobuf.UInt32Value enforcingConsecutive5Xx_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> enforcingConsecutive5XxBuilder_;
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive 5xx. This setting can be used to disable
* ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_5xx = 5 [(.validate.rules) = { ... }
* @return Whether the enforcingConsecutive5xx field is set.
*/
public boolean hasEnforcingConsecutive5Xx() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive 5xx. This setting can be used to disable
* ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_5xx = 5 [(.validate.rules) = { ... }
* @return The enforcingConsecutive5xx.
*/
public com.google.protobuf.UInt32Value getEnforcingConsecutive5Xx() {
if (enforcingConsecutive5XxBuilder_ == null) {
return enforcingConsecutive5Xx_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingConsecutive5Xx_;
} else {
return enforcingConsecutive5XxBuilder_.getMessage();
}
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive 5xx. This setting can be used to disable
* ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_5xx = 5 [(.validate.rules) = { ... }
*/
public Builder setEnforcingConsecutive5Xx(com.google.protobuf.UInt32Value value) {
if (enforcingConsecutive5XxBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
enforcingConsecutive5Xx_ = value;
} else {
enforcingConsecutive5XxBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive 5xx. This setting can be used to disable
* ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_5xx = 5 [(.validate.rules) = { ... }
*/
public Builder setEnforcingConsecutive5Xx(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (enforcingConsecutive5XxBuilder_ == null) {
enforcingConsecutive5Xx_ = builderForValue.build();
} else {
enforcingConsecutive5XxBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive 5xx. This setting can be used to disable
* ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_5xx = 5 [(.validate.rules) = { ... }
*/
public Builder mergeEnforcingConsecutive5Xx(com.google.protobuf.UInt32Value value) {
if (enforcingConsecutive5XxBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0) &&
enforcingConsecutive5Xx_ != null &&
enforcingConsecutive5Xx_ != com.google.protobuf.UInt32Value.getDefaultInstance()) {
getEnforcingConsecutive5XxBuilder().mergeFrom(value);
} else {
enforcingConsecutive5Xx_ = value;
}
} else {
enforcingConsecutive5XxBuilder_.mergeFrom(value);
}
if (enforcingConsecutive5Xx_ != null) {
bitField0_ |= 0x00000010;
onChanged();
}
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive 5xx. This setting can be used to disable
* ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_5xx = 5 [(.validate.rules) = { ... }
*/
public Builder clearEnforcingConsecutive5Xx() {
bitField0_ = (bitField0_ & ~0x00000010);
enforcingConsecutive5Xx_ = null;
if (enforcingConsecutive5XxBuilder_ != null) {
enforcingConsecutive5XxBuilder_.dispose();
enforcingConsecutive5XxBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive 5xx. This setting can be used to disable
* ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_5xx = 5 [(.validate.rules) = { ... }
*/
public com.google.protobuf.UInt32Value.Builder getEnforcingConsecutive5XxBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getEnforcingConsecutive5XxFieldBuilder().getBuilder();
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive 5xx. This setting can be used to disable
* ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_5xx = 5 [(.validate.rules) = { ... }
*/
public com.google.protobuf.UInt32ValueOrBuilder getEnforcingConsecutive5XxOrBuilder() {
if (enforcingConsecutive5XxBuilder_ != null) {
return enforcingConsecutive5XxBuilder_.getMessageOrBuilder();
} else {
return enforcingConsecutive5Xx_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingConsecutive5Xx_;
}
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive 5xx. This setting can be used to disable
* ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_5xx = 5 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getEnforcingConsecutive5XxFieldBuilder() {
if (enforcingConsecutive5XxBuilder_ == null) {
enforcingConsecutive5XxBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getEnforcingConsecutive5Xx(),
getParentForChildren(),
isClean());
enforcingConsecutive5Xx_ = null;
}
return enforcingConsecutive5XxBuilder_;
}
private com.google.protobuf.UInt32Value enforcingSuccessRate_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> enforcingSuccessRateBuilder_;
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics. This setting can be used to
* disable ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_success_rate = 6 [(.validate.rules) = { ... }
* @return Whether the enforcingSuccessRate field is set.
*/
public boolean hasEnforcingSuccessRate() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics. This setting can be used to
* disable ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_success_rate = 6 [(.validate.rules) = { ... }
* @return The enforcingSuccessRate.
*/
public com.google.protobuf.UInt32Value getEnforcingSuccessRate() {
if (enforcingSuccessRateBuilder_ == null) {
return enforcingSuccessRate_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingSuccessRate_;
} else {
return enforcingSuccessRateBuilder_.getMessage();
}
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics. This setting can be used to
* disable ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_success_rate = 6 [(.validate.rules) = { ... }
*/
public Builder setEnforcingSuccessRate(com.google.protobuf.UInt32Value value) {
if (enforcingSuccessRateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
enforcingSuccessRate_ = value;
} else {
enforcingSuccessRateBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics. This setting can be used to
* disable ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_success_rate = 6 [(.validate.rules) = { ... }
*/
public Builder setEnforcingSuccessRate(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (enforcingSuccessRateBuilder_ == null) {
enforcingSuccessRate_ = builderForValue.build();
} else {
enforcingSuccessRateBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics. This setting can be used to
* disable ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_success_rate = 6 [(.validate.rules) = { ... }
*/
public Builder mergeEnforcingSuccessRate(com.google.protobuf.UInt32Value value) {
if (enforcingSuccessRateBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0) &&
enforcingSuccessRate_ != null &&
enforcingSuccessRate_ != com.google.protobuf.UInt32Value.getDefaultInstance()) {
getEnforcingSuccessRateBuilder().mergeFrom(value);
} else {
enforcingSuccessRate_ = value;
}
} else {
enforcingSuccessRateBuilder_.mergeFrom(value);
}
if (enforcingSuccessRate_ != null) {
bitField0_ |= 0x00000020;
onChanged();
}
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics. This setting can be used to
* disable ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_success_rate = 6 [(.validate.rules) = { ... }
*/
public Builder clearEnforcingSuccessRate() {
bitField0_ = (bitField0_ & ~0x00000020);
enforcingSuccessRate_ = null;
if (enforcingSuccessRateBuilder_ != null) {
enforcingSuccessRateBuilder_.dispose();
enforcingSuccessRateBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics. This setting can be used to
* disable ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_success_rate = 6 [(.validate.rules) = { ... }
*/
public com.google.protobuf.UInt32Value.Builder getEnforcingSuccessRateBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getEnforcingSuccessRateFieldBuilder().getBuilder();
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics. This setting can be used to
* disable ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_success_rate = 6 [(.validate.rules) = { ... }
*/
public com.google.protobuf.UInt32ValueOrBuilder getEnforcingSuccessRateOrBuilder() {
if (enforcingSuccessRateBuilder_ != null) {
return enforcingSuccessRateBuilder_.getMessageOrBuilder();
} else {
return enforcingSuccessRate_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingSuccessRate_;
}
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics. This setting can be used to
* disable ejection or to ramp it up slowly. Defaults to 100.
*
*
* .google.protobuf.UInt32Value enforcing_success_rate = 6 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getEnforcingSuccessRateFieldBuilder() {
if (enforcingSuccessRateBuilder_ == null) {
enforcingSuccessRateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getEnforcingSuccessRate(),
getParentForChildren(),
isClean());
enforcingSuccessRate_ = null;
}
return enforcingSuccessRateBuilder_;
}
private com.google.protobuf.UInt32Value successRateMinimumHosts_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> successRateMinimumHostsBuilder_;
/**
*
* The number of hosts in a cluster that must have enough request volume to
* detect success rate outliers. If the number of hosts is less than this
* setting, outlier detection via success rate statistics is not performed
* for any host in the cluster. Defaults to 5.
*
*
* .google.protobuf.UInt32Value success_rate_minimum_hosts = 7;
* @return Whether the successRateMinimumHosts field is set.
*/
public boolean hasSuccessRateMinimumHosts() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* The number of hosts in a cluster that must have enough request volume to
* detect success rate outliers. If the number of hosts is less than this
* setting, outlier detection via success rate statistics is not performed
* for any host in the cluster. Defaults to 5.
*
*
* .google.protobuf.UInt32Value success_rate_minimum_hosts = 7;
* @return The successRateMinimumHosts.
*/
public com.google.protobuf.UInt32Value getSuccessRateMinimumHosts() {
if (successRateMinimumHostsBuilder_ == null) {
return successRateMinimumHosts_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : successRateMinimumHosts_;
} else {
return successRateMinimumHostsBuilder_.getMessage();
}
}
/**
*
* The number of hosts in a cluster that must have enough request volume to
* detect success rate outliers. If the number of hosts is less than this
* setting, outlier detection via success rate statistics is not performed
* for any host in the cluster. Defaults to 5.
*
*
* .google.protobuf.UInt32Value success_rate_minimum_hosts = 7;
*/
public Builder setSuccessRateMinimumHosts(com.google.protobuf.UInt32Value value) {
if (successRateMinimumHostsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
successRateMinimumHosts_ = value;
} else {
successRateMinimumHostsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* The number of hosts in a cluster that must have enough request volume to
* detect success rate outliers. If the number of hosts is less than this
* setting, outlier detection via success rate statistics is not performed
* for any host in the cluster. Defaults to 5.
*
*
* .google.protobuf.UInt32Value success_rate_minimum_hosts = 7;
*/
public Builder setSuccessRateMinimumHosts(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (successRateMinimumHostsBuilder_ == null) {
successRateMinimumHosts_ = builderForValue.build();
} else {
successRateMinimumHostsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* The number of hosts in a cluster that must have enough request volume to
* detect success rate outliers. If the number of hosts is less than this
* setting, outlier detection via success rate statistics is not performed
* for any host in the cluster. Defaults to 5.
*
*
* .google.protobuf.UInt32Value success_rate_minimum_hosts = 7;
*/
public Builder mergeSuccessRateMinimumHosts(com.google.protobuf.UInt32Value value) {
if (successRateMinimumHostsBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0) &&
successRateMinimumHosts_ != null &&
successRateMinimumHosts_ != com.google.protobuf.UInt32Value.getDefaultInstance()) {
getSuccessRateMinimumHostsBuilder().mergeFrom(value);
} else {
successRateMinimumHosts_ = value;
}
} else {
successRateMinimumHostsBuilder_.mergeFrom(value);
}
if (successRateMinimumHosts_ != null) {
bitField0_ |= 0x00000040;
onChanged();
}
return this;
}
/**
*
* The number of hosts in a cluster that must have enough request volume to
* detect success rate outliers. If the number of hosts is less than this
* setting, outlier detection via success rate statistics is not performed
* for any host in the cluster. Defaults to 5.
*
*
* .google.protobuf.UInt32Value success_rate_minimum_hosts = 7;
*/
public Builder clearSuccessRateMinimumHosts() {
bitField0_ = (bitField0_ & ~0x00000040);
successRateMinimumHosts_ = null;
if (successRateMinimumHostsBuilder_ != null) {
successRateMinimumHostsBuilder_.dispose();
successRateMinimumHostsBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The number of hosts in a cluster that must have enough request volume to
* detect success rate outliers. If the number of hosts is less than this
* setting, outlier detection via success rate statistics is not performed
* for any host in the cluster. Defaults to 5.
*
*
* .google.protobuf.UInt32Value success_rate_minimum_hosts = 7;
*/
public com.google.protobuf.UInt32Value.Builder getSuccessRateMinimumHostsBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getSuccessRateMinimumHostsFieldBuilder().getBuilder();
}
/**
*
* The number of hosts in a cluster that must have enough request volume to
* detect success rate outliers. If the number of hosts is less than this
* setting, outlier detection via success rate statistics is not performed
* for any host in the cluster. Defaults to 5.
*
*
* .google.protobuf.UInt32Value success_rate_minimum_hosts = 7;
*/
public com.google.protobuf.UInt32ValueOrBuilder getSuccessRateMinimumHostsOrBuilder() {
if (successRateMinimumHostsBuilder_ != null) {
return successRateMinimumHostsBuilder_.getMessageOrBuilder();
} else {
return successRateMinimumHosts_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : successRateMinimumHosts_;
}
}
/**
*
* The number of hosts in a cluster that must have enough request volume to
* detect success rate outliers. If the number of hosts is less than this
* setting, outlier detection via success rate statistics is not performed
* for any host in the cluster. Defaults to 5.
*
*
* .google.protobuf.UInt32Value success_rate_minimum_hosts = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getSuccessRateMinimumHostsFieldBuilder() {
if (successRateMinimumHostsBuilder_ == null) {
successRateMinimumHostsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getSuccessRateMinimumHosts(),
getParentForChildren(),
isClean());
successRateMinimumHosts_ = null;
}
return successRateMinimumHostsBuilder_;
}
private com.google.protobuf.UInt32Value successRateRequestVolume_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> successRateRequestVolumeBuilder_;
/**
*
* The minimum number of total requests that must be collected in one
* interval (as defined by the interval duration above) to include this host
* in success rate based outlier detection. If the volume is lower than this
* setting, outlier detection via success rate statistics is not performed
* for that host. Defaults to 100.
*
*
* .google.protobuf.UInt32Value success_rate_request_volume = 8;
* @return Whether the successRateRequestVolume field is set.
*/
public boolean hasSuccessRateRequestVolume() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* The minimum number of total requests that must be collected in one
* interval (as defined by the interval duration above) to include this host
* in success rate based outlier detection. If the volume is lower than this
* setting, outlier detection via success rate statistics is not performed
* for that host. Defaults to 100.
*
*
* .google.protobuf.UInt32Value success_rate_request_volume = 8;
* @return The successRateRequestVolume.
*/
public com.google.protobuf.UInt32Value getSuccessRateRequestVolume() {
if (successRateRequestVolumeBuilder_ == null) {
return successRateRequestVolume_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : successRateRequestVolume_;
} else {
return successRateRequestVolumeBuilder_.getMessage();
}
}
/**
*
* The minimum number of total requests that must be collected in one
* interval (as defined by the interval duration above) to include this host
* in success rate based outlier detection. If the volume is lower than this
* setting, outlier detection via success rate statistics is not performed
* for that host. Defaults to 100.
*
*
* .google.protobuf.UInt32Value success_rate_request_volume = 8;
*/
public Builder setSuccessRateRequestVolume(com.google.protobuf.UInt32Value value) {
if (successRateRequestVolumeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
successRateRequestVolume_ = value;
} else {
successRateRequestVolumeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* The minimum number of total requests that must be collected in one
* interval (as defined by the interval duration above) to include this host
* in success rate based outlier detection. If the volume is lower than this
* setting, outlier detection via success rate statistics is not performed
* for that host. Defaults to 100.
*
*
* .google.protobuf.UInt32Value success_rate_request_volume = 8;
*/
public Builder setSuccessRateRequestVolume(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (successRateRequestVolumeBuilder_ == null) {
successRateRequestVolume_ = builderForValue.build();
} else {
successRateRequestVolumeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* The minimum number of total requests that must be collected in one
* interval (as defined by the interval duration above) to include this host
* in success rate based outlier detection. If the volume is lower than this
* setting, outlier detection via success rate statistics is not performed
* for that host. Defaults to 100.
*
*
* .google.protobuf.UInt32Value success_rate_request_volume = 8;
*/
public Builder mergeSuccessRateRequestVolume(com.google.protobuf.UInt32Value value) {
if (successRateRequestVolumeBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0) &&
successRateRequestVolume_ != null &&
successRateRequestVolume_ != com.google.protobuf.UInt32Value.getDefaultInstance()) {
getSuccessRateRequestVolumeBuilder().mergeFrom(value);
} else {
successRateRequestVolume_ = value;
}
} else {
successRateRequestVolumeBuilder_.mergeFrom(value);
}
if (successRateRequestVolume_ != null) {
bitField0_ |= 0x00000080;
onChanged();
}
return this;
}
/**
*
* The minimum number of total requests that must be collected in one
* interval (as defined by the interval duration above) to include this host
* in success rate based outlier detection. If the volume is lower than this
* setting, outlier detection via success rate statistics is not performed
* for that host. Defaults to 100.
*
*
* .google.protobuf.UInt32Value success_rate_request_volume = 8;
*/
public Builder clearSuccessRateRequestVolume() {
bitField0_ = (bitField0_ & ~0x00000080);
successRateRequestVolume_ = null;
if (successRateRequestVolumeBuilder_ != null) {
successRateRequestVolumeBuilder_.dispose();
successRateRequestVolumeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The minimum number of total requests that must be collected in one
* interval (as defined by the interval duration above) to include this host
* in success rate based outlier detection. If the volume is lower than this
* setting, outlier detection via success rate statistics is not performed
* for that host. Defaults to 100.
*
*
* .google.protobuf.UInt32Value success_rate_request_volume = 8;
*/
public com.google.protobuf.UInt32Value.Builder getSuccessRateRequestVolumeBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getSuccessRateRequestVolumeFieldBuilder().getBuilder();
}
/**
*
* The minimum number of total requests that must be collected in one
* interval (as defined by the interval duration above) to include this host
* in success rate based outlier detection. If the volume is lower than this
* setting, outlier detection via success rate statistics is not performed
* for that host. Defaults to 100.
*
*
* .google.protobuf.UInt32Value success_rate_request_volume = 8;
*/
public com.google.protobuf.UInt32ValueOrBuilder getSuccessRateRequestVolumeOrBuilder() {
if (successRateRequestVolumeBuilder_ != null) {
return successRateRequestVolumeBuilder_.getMessageOrBuilder();
} else {
return successRateRequestVolume_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : successRateRequestVolume_;
}
}
/**
*
* The minimum number of total requests that must be collected in one
* interval (as defined by the interval duration above) to include this host
* in success rate based outlier detection. If the volume is lower than this
* setting, outlier detection via success rate statistics is not performed
* for that host. Defaults to 100.
*
*
* .google.protobuf.UInt32Value success_rate_request_volume = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getSuccessRateRequestVolumeFieldBuilder() {
if (successRateRequestVolumeBuilder_ == null) {
successRateRequestVolumeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getSuccessRateRequestVolume(),
getParentForChildren(),
isClean());
successRateRequestVolume_ = null;
}
return successRateRequestVolumeBuilder_;
}
private com.google.protobuf.UInt32Value successRateStdevFactor_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> successRateStdevFactorBuilder_;
/**
*
* This factor is used to determine the ejection threshold for success rate
* outlier ejection. The ejection threshold is the difference between the
* mean success rate, and the product of this factor and the standard
* deviation of the mean success rate: mean - (stdev *
* success_rate_stdev_factor). This factor is divided by a thousand to get a
* double. That is, if the desired factor is 1.9, the runtime value should
* be 1900. Defaults to 1900.
*
*
* .google.protobuf.UInt32Value success_rate_stdev_factor = 9;
* @return Whether the successRateStdevFactor field is set.
*/
public boolean hasSuccessRateStdevFactor() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* This factor is used to determine the ejection threshold for success rate
* outlier ejection. The ejection threshold is the difference between the
* mean success rate, and the product of this factor and the standard
* deviation of the mean success rate: mean - (stdev *
* success_rate_stdev_factor). This factor is divided by a thousand to get a
* double. That is, if the desired factor is 1.9, the runtime value should
* be 1900. Defaults to 1900.
*
*
* .google.protobuf.UInt32Value success_rate_stdev_factor = 9;
* @return The successRateStdevFactor.
*/
public com.google.protobuf.UInt32Value getSuccessRateStdevFactor() {
if (successRateStdevFactorBuilder_ == null) {
return successRateStdevFactor_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : successRateStdevFactor_;
} else {
return successRateStdevFactorBuilder_.getMessage();
}
}
/**
*
* This factor is used to determine the ejection threshold for success rate
* outlier ejection. The ejection threshold is the difference between the
* mean success rate, and the product of this factor and the standard
* deviation of the mean success rate: mean - (stdev *
* success_rate_stdev_factor). This factor is divided by a thousand to get a
* double. That is, if the desired factor is 1.9, the runtime value should
* be 1900. Defaults to 1900.
*
*
* .google.protobuf.UInt32Value success_rate_stdev_factor = 9;
*/
public Builder setSuccessRateStdevFactor(com.google.protobuf.UInt32Value value) {
if (successRateStdevFactorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
successRateStdevFactor_ = value;
} else {
successRateStdevFactorBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
* This factor is used to determine the ejection threshold for success rate
* outlier ejection. The ejection threshold is the difference between the
* mean success rate, and the product of this factor and the standard
* deviation of the mean success rate: mean - (stdev *
* success_rate_stdev_factor). This factor is divided by a thousand to get a
* double. That is, if the desired factor is 1.9, the runtime value should
* be 1900. Defaults to 1900.
*
*
* .google.protobuf.UInt32Value success_rate_stdev_factor = 9;
*/
public Builder setSuccessRateStdevFactor(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (successRateStdevFactorBuilder_ == null) {
successRateStdevFactor_ = builderForValue.build();
} else {
successRateStdevFactorBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
* This factor is used to determine the ejection threshold for success rate
* outlier ejection. The ejection threshold is the difference between the
* mean success rate, and the product of this factor and the standard
* deviation of the mean success rate: mean - (stdev *
* success_rate_stdev_factor). This factor is divided by a thousand to get a
* double. That is, if the desired factor is 1.9, the runtime value should
* be 1900. Defaults to 1900.
*
*
* .google.protobuf.UInt32Value success_rate_stdev_factor = 9;
*/
public Builder mergeSuccessRateStdevFactor(com.google.protobuf.UInt32Value value) {
if (successRateStdevFactorBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0) &&
successRateStdevFactor_ != null &&
successRateStdevFactor_ != com.google.protobuf.UInt32Value.getDefaultInstance()) {
getSuccessRateStdevFactorBuilder().mergeFrom(value);
} else {
successRateStdevFactor_ = value;
}
} else {
successRateStdevFactorBuilder_.mergeFrom(value);
}
if (successRateStdevFactor_ != null) {
bitField0_ |= 0x00000100;
onChanged();
}
return this;
}
/**
*
* This factor is used to determine the ejection threshold for success rate
* outlier ejection. The ejection threshold is the difference between the
* mean success rate, and the product of this factor and the standard
* deviation of the mean success rate: mean - (stdev *
* success_rate_stdev_factor). This factor is divided by a thousand to get a
* double. That is, if the desired factor is 1.9, the runtime value should
* be 1900. Defaults to 1900.
*
*
* .google.protobuf.UInt32Value success_rate_stdev_factor = 9;
*/
public Builder clearSuccessRateStdevFactor() {
bitField0_ = (bitField0_ & ~0x00000100);
successRateStdevFactor_ = null;
if (successRateStdevFactorBuilder_ != null) {
successRateStdevFactorBuilder_.dispose();
successRateStdevFactorBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* This factor is used to determine the ejection threshold for success rate
* outlier ejection. The ejection threshold is the difference between the
* mean success rate, and the product of this factor and the standard
* deviation of the mean success rate: mean - (stdev *
* success_rate_stdev_factor). This factor is divided by a thousand to get a
* double. That is, if the desired factor is 1.9, the runtime value should
* be 1900. Defaults to 1900.
*
*
* .google.protobuf.UInt32Value success_rate_stdev_factor = 9;
*/
public com.google.protobuf.UInt32Value.Builder getSuccessRateStdevFactorBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getSuccessRateStdevFactorFieldBuilder().getBuilder();
}
/**
*
* This factor is used to determine the ejection threshold for success rate
* outlier ejection. The ejection threshold is the difference between the
* mean success rate, and the product of this factor and the standard
* deviation of the mean success rate: mean - (stdev *
* success_rate_stdev_factor). This factor is divided by a thousand to get a
* double. That is, if the desired factor is 1.9, the runtime value should
* be 1900. Defaults to 1900.
*
*
* .google.protobuf.UInt32Value success_rate_stdev_factor = 9;
*/
public com.google.protobuf.UInt32ValueOrBuilder getSuccessRateStdevFactorOrBuilder() {
if (successRateStdevFactorBuilder_ != null) {
return successRateStdevFactorBuilder_.getMessageOrBuilder();
} else {
return successRateStdevFactor_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : successRateStdevFactor_;
}
}
/**
*
* This factor is used to determine the ejection threshold for success rate
* outlier ejection. The ejection threshold is the difference between the
* mean success rate, and the product of this factor and the standard
* deviation of the mean success rate: mean - (stdev *
* success_rate_stdev_factor). This factor is divided by a thousand to get a
* double. That is, if the desired factor is 1.9, the runtime value should
* be 1900. Defaults to 1900.
*
*
* .google.protobuf.UInt32Value success_rate_stdev_factor = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getSuccessRateStdevFactorFieldBuilder() {
if (successRateStdevFactorBuilder_ == null) {
successRateStdevFactorBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getSuccessRateStdevFactor(),
getParentForChildren(),
isClean());
successRateStdevFactor_ = null;
}
return successRateStdevFactorBuilder_;
}
private com.google.protobuf.UInt32Value consecutiveGatewayFailure_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> consecutiveGatewayFailureBuilder_;
/**
*
* The number of consecutive gateway failures (502, 503, 504 status codes)
* before a consecutive gateway failure ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_gateway_failure = 10;
* @return Whether the consecutiveGatewayFailure field is set.
*/
public boolean hasConsecutiveGatewayFailure() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
* The number of consecutive gateway failures (502, 503, 504 status codes)
* before a consecutive gateway failure ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_gateway_failure = 10;
* @return The consecutiveGatewayFailure.
*/
public com.google.protobuf.UInt32Value getConsecutiveGatewayFailure() {
if (consecutiveGatewayFailureBuilder_ == null) {
return consecutiveGatewayFailure_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : consecutiveGatewayFailure_;
} else {
return consecutiveGatewayFailureBuilder_.getMessage();
}
}
/**
*
* The number of consecutive gateway failures (502, 503, 504 status codes)
* before a consecutive gateway failure ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_gateway_failure = 10;
*/
public Builder setConsecutiveGatewayFailure(com.google.protobuf.UInt32Value value) {
if (consecutiveGatewayFailureBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
consecutiveGatewayFailure_ = value;
} else {
consecutiveGatewayFailureBuilder_.setMessage(value);
}
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
* The number of consecutive gateway failures (502, 503, 504 status codes)
* before a consecutive gateway failure ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_gateway_failure = 10;
*/
public Builder setConsecutiveGatewayFailure(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (consecutiveGatewayFailureBuilder_ == null) {
consecutiveGatewayFailure_ = builderForValue.build();
} else {
consecutiveGatewayFailureBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
* The number of consecutive gateway failures (502, 503, 504 status codes)
* before a consecutive gateway failure ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_gateway_failure = 10;
*/
public Builder mergeConsecutiveGatewayFailure(com.google.protobuf.UInt32Value value) {
if (consecutiveGatewayFailureBuilder_ == null) {
if (((bitField0_ & 0x00000200) != 0) &&
consecutiveGatewayFailure_ != null &&
consecutiveGatewayFailure_ != com.google.protobuf.UInt32Value.getDefaultInstance()) {
getConsecutiveGatewayFailureBuilder().mergeFrom(value);
} else {
consecutiveGatewayFailure_ = value;
}
} else {
consecutiveGatewayFailureBuilder_.mergeFrom(value);
}
if (consecutiveGatewayFailure_ != null) {
bitField0_ |= 0x00000200;
onChanged();
}
return this;
}
/**
*
* The number of consecutive gateway failures (502, 503, 504 status codes)
* before a consecutive gateway failure ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_gateway_failure = 10;
*/
public Builder clearConsecutiveGatewayFailure() {
bitField0_ = (bitField0_ & ~0x00000200);
consecutiveGatewayFailure_ = null;
if (consecutiveGatewayFailureBuilder_ != null) {
consecutiveGatewayFailureBuilder_.dispose();
consecutiveGatewayFailureBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The number of consecutive gateway failures (502, 503, 504 status codes)
* before a consecutive gateway failure ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_gateway_failure = 10;
*/
public com.google.protobuf.UInt32Value.Builder getConsecutiveGatewayFailureBuilder() {
bitField0_ |= 0x00000200;
onChanged();
return getConsecutiveGatewayFailureFieldBuilder().getBuilder();
}
/**
*
* The number of consecutive gateway failures (502, 503, 504 status codes)
* before a consecutive gateway failure ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_gateway_failure = 10;
*/
public com.google.protobuf.UInt32ValueOrBuilder getConsecutiveGatewayFailureOrBuilder() {
if (consecutiveGatewayFailureBuilder_ != null) {
return consecutiveGatewayFailureBuilder_.getMessageOrBuilder();
} else {
return consecutiveGatewayFailure_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : consecutiveGatewayFailure_;
}
}
/**
*
* The number of consecutive gateway failures (502, 503, 504 status codes)
* before a consecutive gateway failure ejection occurs. Defaults to 5.
*
*
* .google.protobuf.UInt32Value consecutive_gateway_failure = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getConsecutiveGatewayFailureFieldBuilder() {
if (consecutiveGatewayFailureBuilder_ == null) {
consecutiveGatewayFailureBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getConsecutiveGatewayFailure(),
getParentForChildren(),
isClean());
consecutiveGatewayFailure_ = null;
}
return consecutiveGatewayFailureBuilder_;
}
private com.google.protobuf.UInt32Value enforcingConsecutiveGatewayFailure_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> enforcingConsecutiveGatewayFailureBuilder_;
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive gateway failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_gateway_failure = 11 [(.validate.rules) = { ... }
* @return Whether the enforcingConsecutiveGatewayFailure field is set.
*/
public boolean hasEnforcingConsecutiveGatewayFailure() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive gateway failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_gateway_failure = 11 [(.validate.rules) = { ... }
* @return The enforcingConsecutiveGatewayFailure.
*/
public com.google.protobuf.UInt32Value getEnforcingConsecutiveGatewayFailure() {
if (enforcingConsecutiveGatewayFailureBuilder_ == null) {
return enforcingConsecutiveGatewayFailure_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingConsecutiveGatewayFailure_;
} else {
return enforcingConsecutiveGatewayFailureBuilder_.getMessage();
}
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive gateway failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_gateway_failure = 11 [(.validate.rules) = { ... }
*/
public Builder setEnforcingConsecutiveGatewayFailure(com.google.protobuf.UInt32Value value) {
if (enforcingConsecutiveGatewayFailureBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
enforcingConsecutiveGatewayFailure_ = value;
} else {
enforcingConsecutiveGatewayFailureBuilder_.setMessage(value);
}
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive gateway failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_gateway_failure = 11 [(.validate.rules) = { ... }
*/
public Builder setEnforcingConsecutiveGatewayFailure(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (enforcingConsecutiveGatewayFailureBuilder_ == null) {
enforcingConsecutiveGatewayFailure_ = builderForValue.build();
} else {
enforcingConsecutiveGatewayFailureBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive gateway failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_gateway_failure = 11 [(.validate.rules) = { ... }
*/
public Builder mergeEnforcingConsecutiveGatewayFailure(com.google.protobuf.UInt32Value value) {
if (enforcingConsecutiveGatewayFailureBuilder_ == null) {
if (((bitField0_ & 0x00000400) != 0) &&
enforcingConsecutiveGatewayFailure_ != null &&
enforcingConsecutiveGatewayFailure_ != com.google.protobuf.UInt32Value.getDefaultInstance()) {
getEnforcingConsecutiveGatewayFailureBuilder().mergeFrom(value);
} else {
enforcingConsecutiveGatewayFailure_ = value;
}
} else {
enforcingConsecutiveGatewayFailureBuilder_.mergeFrom(value);
}
if (enforcingConsecutiveGatewayFailure_ != null) {
bitField0_ |= 0x00000400;
onChanged();
}
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive gateway failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_gateway_failure = 11 [(.validate.rules) = { ... }
*/
public Builder clearEnforcingConsecutiveGatewayFailure() {
bitField0_ = (bitField0_ & ~0x00000400);
enforcingConsecutiveGatewayFailure_ = null;
if (enforcingConsecutiveGatewayFailureBuilder_ != null) {
enforcingConsecutiveGatewayFailureBuilder_.dispose();
enforcingConsecutiveGatewayFailureBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive gateway failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_gateway_failure = 11 [(.validate.rules) = { ... }
*/
public com.google.protobuf.UInt32Value.Builder getEnforcingConsecutiveGatewayFailureBuilder() {
bitField0_ |= 0x00000400;
onChanged();
return getEnforcingConsecutiveGatewayFailureFieldBuilder().getBuilder();
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive gateway failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_gateway_failure = 11 [(.validate.rules) = { ... }
*/
public com.google.protobuf.UInt32ValueOrBuilder getEnforcingConsecutiveGatewayFailureOrBuilder() {
if (enforcingConsecutiveGatewayFailureBuilder_ != null) {
return enforcingConsecutiveGatewayFailureBuilder_.getMessageOrBuilder();
} else {
return enforcingConsecutiveGatewayFailure_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingConsecutiveGatewayFailure_;
}
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive gateway failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_gateway_failure = 11 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getEnforcingConsecutiveGatewayFailureFieldBuilder() {
if (enforcingConsecutiveGatewayFailureBuilder_ == null) {
enforcingConsecutiveGatewayFailureBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getEnforcingConsecutiveGatewayFailure(),
getParentForChildren(),
isClean());
enforcingConsecutiveGatewayFailure_ = null;
}
return enforcingConsecutiveGatewayFailureBuilder_;
}
private boolean splitExternalLocalOriginErrors_ ;
/**
*
* Determines whether to distinguish local origin failures from external errors. If set to true
* the following configuration parameters are taken into account:
* :ref:`consecutive_local_origin_failure<envoy_v3_api_field_config.cluster.v3.OutlierDetection.consecutive_local_origin_failure>`,
* :ref:`enforcing_consecutive_local_origin_failure<envoy_v3_api_field_config.cluster.v3.OutlierDetection.enforcing_consecutive_local_origin_failure>`
* and
* :ref:`enforcing_local_origin_success_rate<envoy_v3_api_field_config.cluster.v3.OutlierDetection.enforcing_local_origin_success_rate>`.
* Defaults to false.
*
*
* bool split_external_local_origin_errors = 12;
* @return The splitExternalLocalOriginErrors.
*/
@java.lang.Override
public boolean getSplitExternalLocalOriginErrors() {
return splitExternalLocalOriginErrors_;
}
/**
*
* Determines whether to distinguish local origin failures from external errors. If set to true
* the following configuration parameters are taken into account:
* :ref:`consecutive_local_origin_failure<envoy_v3_api_field_config.cluster.v3.OutlierDetection.consecutive_local_origin_failure>`,
* :ref:`enforcing_consecutive_local_origin_failure<envoy_v3_api_field_config.cluster.v3.OutlierDetection.enforcing_consecutive_local_origin_failure>`
* and
* :ref:`enforcing_local_origin_success_rate<envoy_v3_api_field_config.cluster.v3.OutlierDetection.enforcing_local_origin_success_rate>`.
* Defaults to false.
*
*
* bool split_external_local_origin_errors = 12;
* @param value The splitExternalLocalOriginErrors to set.
* @return This builder for chaining.
*/
public Builder setSplitExternalLocalOriginErrors(boolean value) {
splitExternalLocalOriginErrors_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
* Determines whether to distinguish local origin failures from external errors. If set to true
* the following configuration parameters are taken into account:
* :ref:`consecutive_local_origin_failure<envoy_v3_api_field_config.cluster.v3.OutlierDetection.consecutive_local_origin_failure>`,
* :ref:`enforcing_consecutive_local_origin_failure<envoy_v3_api_field_config.cluster.v3.OutlierDetection.enforcing_consecutive_local_origin_failure>`
* and
* :ref:`enforcing_local_origin_success_rate<envoy_v3_api_field_config.cluster.v3.OutlierDetection.enforcing_local_origin_success_rate>`.
* Defaults to false.
*
*
* bool split_external_local_origin_errors = 12;
* @return This builder for chaining.
*/
public Builder clearSplitExternalLocalOriginErrors() {
bitField0_ = (bitField0_ & ~0x00000800);
splitExternalLocalOriginErrors_ = false;
onChanged();
return this;
}
private com.google.protobuf.UInt32Value consecutiveLocalOriginFailure_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> consecutiveLocalOriginFailureBuilder_;
/**
*
* The number of consecutive locally originated failures before ejection
* occurs. Defaults to 5. Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value consecutive_local_origin_failure = 13;
* @return Whether the consecutiveLocalOriginFailure field is set.
*/
public boolean hasConsecutiveLocalOriginFailure() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
* The number of consecutive locally originated failures before ejection
* occurs. Defaults to 5. Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value consecutive_local_origin_failure = 13;
* @return The consecutiveLocalOriginFailure.
*/
public com.google.protobuf.UInt32Value getConsecutiveLocalOriginFailure() {
if (consecutiveLocalOriginFailureBuilder_ == null) {
return consecutiveLocalOriginFailure_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : consecutiveLocalOriginFailure_;
} else {
return consecutiveLocalOriginFailureBuilder_.getMessage();
}
}
/**
*
* The number of consecutive locally originated failures before ejection
* occurs. Defaults to 5. Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value consecutive_local_origin_failure = 13;
*/
public Builder setConsecutiveLocalOriginFailure(com.google.protobuf.UInt32Value value) {
if (consecutiveLocalOriginFailureBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
consecutiveLocalOriginFailure_ = value;
} else {
consecutiveLocalOriginFailureBuilder_.setMessage(value);
}
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
* The number of consecutive locally originated failures before ejection
* occurs. Defaults to 5. Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value consecutive_local_origin_failure = 13;
*/
public Builder setConsecutiveLocalOriginFailure(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (consecutiveLocalOriginFailureBuilder_ == null) {
consecutiveLocalOriginFailure_ = builderForValue.build();
} else {
consecutiveLocalOriginFailureBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
* The number of consecutive locally originated failures before ejection
* occurs. Defaults to 5. Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value consecutive_local_origin_failure = 13;
*/
public Builder mergeConsecutiveLocalOriginFailure(com.google.protobuf.UInt32Value value) {
if (consecutiveLocalOriginFailureBuilder_ == null) {
if (((bitField0_ & 0x00001000) != 0) &&
consecutiveLocalOriginFailure_ != null &&
consecutiveLocalOriginFailure_ != com.google.protobuf.UInt32Value.getDefaultInstance()) {
getConsecutiveLocalOriginFailureBuilder().mergeFrom(value);
} else {
consecutiveLocalOriginFailure_ = value;
}
} else {
consecutiveLocalOriginFailureBuilder_.mergeFrom(value);
}
if (consecutiveLocalOriginFailure_ != null) {
bitField0_ |= 0x00001000;
onChanged();
}
return this;
}
/**
*
* The number of consecutive locally originated failures before ejection
* occurs. Defaults to 5. Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value consecutive_local_origin_failure = 13;
*/
public Builder clearConsecutiveLocalOriginFailure() {
bitField0_ = (bitField0_ & ~0x00001000);
consecutiveLocalOriginFailure_ = null;
if (consecutiveLocalOriginFailureBuilder_ != null) {
consecutiveLocalOriginFailureBuilder_.dispose();
consecutiveLocalOriginFailureBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The number of consecutive locally originated failures before ejection
* occurs. Defaults to 5. Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value consecutive_local_origin_failure = 13;
*/
public com.google.protobuf.UInt32Value.Builder getConsecutiveLocalOriginFailureBuilder() {
bitField0_ |= 0x00001000;
onChanged();
return getConsecutiveLocalOriginFailureFieldBuilder().getBuilder();
}
/**
*
* The number of consecutive locally originated failures before ejection
* occurs. Defaults to 5. Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value consecutive_local_origin_failure = 13;
*/
public com.google.protobuf.UInt32ValueOrBuilder getConsecutiveLocalOriginFailureOrBuilder() {
if (consecutiveLocalOriginFailureBuilder_ != null) {
return consecutiveLocalOriginFailureBuilder_.getMessageOrBuilder();
} else {
return consecutiveLocalOriginFailure_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : consecutiveLocalOriginFailure_;
}
}
/**
*
* The number of consecutive locally originated failures before ejection
* occurs. Defaults to 5. Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value consecutive_local_origin_failure = 13;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getConsecutiveLocalOriginFailureFieldBuilder() {
if (consecutiveLocalOriginFailureBuilder_ == null) {
consecutiveLocalOriginFailureBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getConsecutiveLocalOriginFailure(),
getParentForChildren(),
isClean());
consecutiveLocalOriginFailure_ = null;
}
return consecutiveLocalOriginFailureBuilder_;
}
private com.google.protobuf.UInt32Value enforcingConsecutiveLocalOriginFailure_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> enforcingConsecutiveLocalOriginFailureBuilder_;
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive locally originated failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_local_origin_failure = 14 [(.validate.rules) = { ... }
* @return Whether the enforcingConsecutiveLocalOriginFailure field is set.
*/
public boolean hasEnforcingConsecutiveLocalOriginFailure() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive locally originated failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_local_origin_failure = 14 [(.validate.rules) = { ... }
* @return The enforcingConsecutiveLocalOriginFailure.
*/
public com.google.protobuf.UInt32Value getEnforcingConsecutiveLocalOriginFailure() {
if (enforcingConsecutiveLocalOriginFailureBuilder_ == null) {
return enforcingConsecutiveLocalOriginFailure_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingConsecutiveLocalOriginFailure_;
} else {
return enforcingConsecutiveLocalOriginFailureBuilder_.getMessage();
}
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive locally originated failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_local_origin_failure = 14 [(.validate.rules) = { ... }
*/
public Builder setEnforcingConsecutiveLocalOriginFailure(com.google.protobuf.UInt32Value value) {
if (enforcingConsecutiveLocalOriginFailureBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
enforcingConsecutiveLocalOriginFailure_ = value;
} else {
enforcingConsecutiveLocalOriginFailureBuilder_.setMessage(value);
}
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive locally originated failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_local_origin_failure = 14 [(.validate.rules) = { ... }
*/
public Builder setEnforcingConsecutiveLocalOriginFailure(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (enforcingConsecutiveLocalOriginFailureBuilder_ == null) {
enforcingConsecutiveLocalOriginFailure_ = builderForValue.build();
} else {
enforcingConsecutiveLocalOriginFailureBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive locally originated failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_local_origin_failure = 14 [(.validate.rules) = { ... }
*/
public Builder mergeEnforcingConsecutiveLocalOriginFailure(com.google.protobuf.UInt32Value value) {
if (enforcingConsecutiveLocalOriginFailureBuilder_ == null) {
if (((bitField0_ & 0x00002000) != 0) &&
enforcingConsecutiveLocalOriginFailure_ != null &&
enforcingConsecutiveLocalOriginFailure_ != com.google.protobuf.UInt32Value.getDefaultInstance()) {
getEnforcingConsecutiveLocalOriginFailureBuilder().mergeFrom(value);
} else {
enforcingConsecutiveLocalOriginFailure_ = value;
}
} else {
enforcingConsecutiveLocalOriginFailureBuilder_.mergeFrom(value);
}
if (enforcingConsecutiveLocalOriginFailure_ != null) {
bitField0_ |= 0x00002000;
onChanged();
}
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive locally originated failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_local_origin_failure = 14 [(.validate.rules) = { ... }
*/
public Builder clearEnforcingConsecutiveLocalOriginFailure() {
bitField0_ = (bitField0_ & ~0x00002000);
enforcingConsecutiveLocalOriginFailure_ = null;
if (enforcingConsecutiveLocalOriginFailureBuilder_ != null) {
enforcingConsecutiveLocalOriginFailureBuilder_.dispose();
enforcingConsecutiveLocalOriginFailureBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive locally originated failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_local_origin_failure = 14 [(.validate.rules) = { ... }
*/
public com.google.protobuf.UInt32Value.Builder getEnforcingConsecutiveLocalOriginFailureBuilder() {
bitField0_ |= 0x00002000;
onChanged();
return getEnforcingConsecutiveLocalOriginFailureFieldBuilder().getBuilder();
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive locally originated failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_local_origin_failure = 14 [(.validate.rules) = { ... }
*/
public com.google.protobuf.UInt32ValueOrBuilder getEnforcingConsecutiveLocalOriginFailureOrBuilder() {
if (enforcingConsecutiveLocalOriginFailureBuilder_ != null) {
return enforcingConsecutiveLocalOriginFailureBuilder_.getMessageOrBuilder();
} else {
return enforcingConsecutiveLocalOriginFailure_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingConsecutiveLocalOriginFailure_;
}
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through consecutive locally originated failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_consecutive_local_origin_failure = 14 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getEnforcingConsecutiveLocalOriginFailureFieldBuilder() {
if (enforcingConsecutiveLocalOriginFailureBuilder_ == null) {
enforcingConsecutiveLocalOriginFailureBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getEnforcingConsecutiveLocalOriginFailure(),
getParentForChildren(),
isClean());
enforcingConsecutiveLocalOriginFailure_ = null;
}
return enforcingConsecutiveLocalOriginFailureBuilder_;
}
private com.google.protobuf.UInt32Value enforcingLocalOriginSuccessRate_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> enforcingLocalOriginSuccessRateBuilder_;
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics for locally originated errors.
* This setting can be used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_local_origin_success_rate = 15 [(.validate.rules) = { ... }
* @return Whether the enforcingLocalOriginSuccessRate field is set.
*/
public boolean hasEnforcingLocalOriginSuccessRate() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics for locally originated errors.
* This setting can be used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_local_origin_success_rate = 15 [(.validate.rules) = { ... }
* @return The enforcingLocalOriginSuccessRate.
*/
public com.google.protobuf.UInt32Value getEnforcingLocalOriginSuccessRate() {
if (enforcingLocalOriginSuccessRateBuilder_ == null) {
return enforcingLocalOriginSuccessRate_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingLocalOriginSuccessRate_;
} else {
return enforcingLocalOriginSuccessRateBuilder_.getMessage();
}
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics for locally originated errors.
* This setting can be used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_local_origin_success_rate = 15 [(.validate.rules) = { ... }
*/
public Builder setEnforcingLocalOriginSuccessRate(com.google.protobuf.UInt32Value value) {
if (enforcingLocalOriginSuccessRateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
enforcingLocalOriginSuccessRate_ = value;
} else {
enforcingLocalOriginSuccessRateBuilder_.setMessage(value);
}
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics for locally originated errors.
* This setting can be used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_local_origin_success_rate = 15 [(.validate.rules) = { ... }
*/
public Builder setEnforcingLocalOriginSuccessRate(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (enforcingLocalOriginSuccessRateBuilder_ == null) {
enforcingLocalOriginSuccessRate_ = builderForValue.build();
} else {
enforcingLocalOriginSuccessRateBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics for locally originated errors.
* This setting can be used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_local_origin_success_rate = 15 [(.validate.rules) = { ... }
*/
public Builder mergeEnforcingLocalOriginSuccessRate(com.google.protobuf.UInt32Value value) {
if (enforcingLocalOriginSuccessRateBuilder_ == null) {
if (((bitField0_ & 0x00004000) != 0) &&
enforcingLocalOriginSuccessRate_ != null &&
enforcingLocalOriginSuccessRate_ != com.google.protobuf.UInt32Value.getDefaultInstance()) {
getEnforcingLocalOriginSuccessRateBuilder().mergeFrom(value);
} else {
enforcingLocalOriginSuccessRate_ = value;
}
} else {
enforcingLocalOriginSuccessRateBuilder_.mergeFrom(value);
}
if (enforcingLocalOriginSuccessRate_ != null) {
bitField0_ |= 0x00004000;
onChanged();
}
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics for locally originated errors.
* This setting can be used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_local_origin_success_rate = 15 [(.validate.rules) = { ... }
*/
public Builder clearEnforcingLocalOriginSuccessRate() {
bitField0_ = (bitField0_ & ~0x00004000);
enforcingLocalOriginSuccessRate_ = null;
if (enforcingLocalOriginSuccessRateBuilder_ != null) {
enforcingLocalOriginSuccessRateBuilder_.dispose();
enforcingLocalOriginSuccessRateBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics for locally originated errors.
* This setting can be used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_local_origin_success_rate = 15 [(.validate.rules) = { ... }
*/
public com.google.protobuf.UInt32Value.Builder getEnforcingLocalOriginSuccessRateBuilder() {
bitField0_ |= 0x00004000;
onChanged();
return getEnforcingLocalOriginSuccessRateFieldBuilder().getBuilder();
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics for locally originated errors.
* This setting can be used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_local_origin_success_rate = 15 [(.validate.rules) = { ... }
*/
public com.google.protobuf.UInt32ValueOrBuilder getEnforcingLocalOriginSuccessRateOrBuilder() {
if (enforcingLocalOriginSuccessRateBuilder_ != null) {
return enforcingLocalOriginSuccessRateBuilder_.getMessageOrBuilder();
} else {
return enforcingLocalOriginSuccessRate_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingLocalOriginSuccessRate_;
}
}
/**
*
* The % chance that a host will be actually ejected when an outlier status
* is detected through success rate statistics for locally originated errors.
* This setting can be used to disable ejection or to ramp it up slowly. Defaults to 100.
* Parameter takes effect only when
* :ref:`split_external_local_origin_errors<envoy_v3_api_field_config.cluster.v3.OutlierDetection.split_external_local_origin_errors>`
* is set to true.
*
*
* .google.protobuf.UInt32Value enforcing_local_origin_success_rate = 15 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getEnforcingLocalOriginSuccessRateFieldBuilder() {
if (enforcingLocalOriginSuccessRateBuilder_ == null) {
enforcingLocalOriginSuccessRateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getEnforcingLocalOriginSuccessRate(),
getParentForChildren(),
isClean());
enforcingLocalOriginSuccessRate_ = null;
}
return enforcingLocalOriginSuccessRateBuilder_;
}
private com.google.protobuf.UInt32Value failurePercentageThreshold_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> failurePercentageThresholdBuilder_;
/**
*
* The failure percentage to use when determining failure percentage-based outlier detection. If
* the failure percentage of a given host is greater than or equal to this value, it will be
* ejected. Defaults to 85.
*
*
* .google.protobuf.UInt32Value failure_percentage_threshold = 16 [(.validate.rules) = { ... }
* @return Whether the failurePercentageThreshold field is set.
*/
public boolean hasFailurePercentageThreshold() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
*
* The failure percentage to use when determining failure percentage-based outlier detection. If
* the failure percentage of a given host is greater than or equal to this value, it will be
* ejected. Defaults to 85.
*
*
* .google.protobuf.UInt32Value failure_percentage_threshold = 16 [(.validate.rules) = { ... }
* @return The failurePercentageThreshold.
*/
public com.google.protobuf.UInt32Value getFailurePercentageThreshold() {
if (failurePercentageThresholdBuilder_ == null) {
return failurePercentageThreshold_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : failurePercentageThreshold_;
} else {
return failurePercentageThresholdBuilder_.getMessage();
}
}
/**
*
* The failure percentage to use when determining failure percentage-based outlier detection. If
* the failure percentage of a given host is greater than or equal to this value, it will be
* ejected. Defaults to 85.
*
*
* .google.protobuf.UInt32Value failure_percentage_threshold = 16 [(.validate.rules) = { ... }
*/
public Builder setFailurePercentageThreshold(com.google.protobuf.UInt32Value value) {
if (failurePercentageThresholdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
failurePercentageThreshold_ = value;
} else {
failurePercentageThresholdBuilder_.setMessage(value);
}
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
* The failure percentage to use when determining failure percentage-based outlier detection. If
* the failure percentage of a given host is greater than or equal to this value, it will be
* ejected. Defaults to 85.
*
*
* .google.protobuf.UInt32Value failure_percentage_threshold = 16 [(.validate.rules) = { ... }
*/
public Builder setFailurePercentageThreshold(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (failurePercentageThresholdBuilder_ == null) {
failurePercentageThreshold_ = builderForValue.build();
} else {
failurePercentageThresholdBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
* The failure percentage to use when determining failure percentage-based outlier detection. If
* the failure percentage of a given host is greater than or equal to this value, it will be
* ejected. Defaults to 85.
*
*
* .google.protobuf.UInt32Value failure_percentage_threshold = 16 [(.validate.rules) = { ... }
*/
public Builder mergeFailurePercentageThreshold(com.google.protobuf.UInt32Value value) {
if (failurePercentageThresholdBuilder_ == null) {
if (((bitField0_ & 0x00008000) != 0) &&
failurePercentageThreshold_ != null &&
failurePercentageThreshold_ != com.google.protobuf.UInt32Value.getDefaultInstance()) {
getFailurePercentageThresholdBuilder().mergeFrom(value);
} else {
failurePercentageThreshold_ = value;
}
} else {
failurePercentageThresholdBuilder_.mergeFrom(value);
}
if (failurePercentageThreshold_ != null) {
bitField0_ |= 0x00008000;
onChanged();
}
return this;
}
/**
*
* The failure percentage to use when determining failure percentage-based outlier detection. If
* the failure percentage of a given host is greater than or equal to this value, it will be
* ejected. Defaults to 85.
*
*
* .google.protobuf.UInt32Value failure_percentage_threshold = 16 [(.validate.rules) = { ... }
*/
public Builder clearFailurePercentageThreshold() {
bitField0_ = (bitField0_ & ~0x00008000);
failurePercentageThreshold_ = null;
if (failurePercentageThresholdBuilder_ != null) {
failurePercentageThresholdBuilder_.dispose();
failurePercentageThresholdBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The failure percentage to use when determining failure percentage-based outlier detection. If
* the failure percentage of a given host is greater than or equal to this value, it will be
* ejected. Defaults to 85.
*
*
* .google.protobuf.UInt32Value failure_percentage_threshold = 16 [(.validate.rules) = { ... }
*/
public com.google.protobuf.UInt32Value.Builder getFailurePercentageThresholdBuilder() {
bitField0_ |= 0x00008000;
onChanged();
return getFailurePercentageThresholdFieldBuilder().getBuilder();
}
/**
*
* The failure percentage to use when determining failure percentage-based outlier detection. If
* the failure percentage of a given host is greater than or equal to this value, it will be
* ejected. Defaults to 85.
*
*
* .google.protobuf.UInt32Value failure_percentage_threshold = 16 [(.validate.rules) = { ... }
*/
public com.google.protobuf.UInt32ValueOrBuilder getFailurePercentageThresholdOrBuilder() {
if (failurePercentageThresholdBuilder_ != null) {
return failurePercentageThresholdBuilder_.getMessageOrBuilder();
} else {
return failurePercentageThreshold_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : failurePercentageThreshold_;
}
}
/**
*
* The failure percentage to use when determining failure percentage-based outlier detection. If
* the failure percentage of a given host is greater than or equal to this value, it will be
* ejected. Defaults to 85.
*
*
* .google.protobuf.UInt32Value failure_percentage_threshold = 16 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getFailurePercentageThresholdFieldBuilder() {
if (failurePercentageThresholdBuilder_ == null) {
failurePercentageThresholdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getFailurePercentageThreshold(),
getParentForChildren(),
isClean());
failurePercentageThreshold_ = null;
}
return failurePercentageThresholdBuilder_;
}
private com.google.protobuf.UInt32Value enforcingFailurePercentage_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> enforcingFailurePercentageBuilder_;
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* failure percentage statistics. This setting can be used to disable ejection or to ramp it up
* slowly. Defaults to 0.
*
* [#next-major-version: setting this without setting failure_percentage_threshold should be
* invalid in v4.]
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage = 17 [(.validate.rules) = { ... }
* @return Whether the enforcingFailurePercentage field is set.
*/
public boolean hasEnforcingFailurePercentage() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* failure percentage statistics. This setting can be used to disable ejection or to ramp it up
* slowly. Defaults to 0.
*
* [#next-major-version: setting this without setting failure_percentage_threshold should be
* invalid in v4.]
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage = 17 [(.validate.rules) = { ... }
* @return The enforcingFailurePercentage.
*/
public com.google.protobuf.UInt32Value getEnforcingFailurePercentage() {
if (enforcingFailurePercentageBuilder_ == null) {
return enforcingFailurePercentage_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingFailurePercentage_;
} else {
return enforcingFailurePercentageBuilder_.getMessage();
}
}
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* failure percentage statistics. This setting can be used to disable ejection or to ramp it up
* slowly. Defaults to 0.
*
* [#next-major-version: setting this without setting failure_percentage_threshold should be
* invalid in v4.]
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage = 17 [(.validate.rules) = { ... }
*/
public Builder setEnforcingFailurePercentage(com.google.protobuf.UInt32Value value) {
if (enforcingFailurePercentageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
enforcingFailurePercentage_ = value;
} else {
enforcingFailurePercentageBuilder_.setMessage(value);
}
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* failure percentage statistics. This setting can be used to disable ejection or to ramp it up
* slowly. Defaults to 0.
*
* [#next-major-version: setting this without setting failure_percentage_threshold should be
* invalid in v4.]
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage = 17 [(.validate.rules) = { ... }
*/
public Builder setEnforcingFailurePercentage(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (enforcingFailurePercentageBuilder_ == null) {
enforcingFailurePercentage_ = builderForValue.build();
} else {
enforcingFailurePercentageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* failure percentage statistics. This setting can be used to disable ejection or to ramp it up
* slowly. Defaults to 0.
*
* [#next-major-version: setting this without setting failure_percentage_threshold should be
* invalid in v4.]
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage = 17 [(.validate.rules) = { ... }
*/
public Builder mergeEnforcingFailurePercentage(com.google.protobuf.UInt32Value value) {
if (enforcingFailurePercentageBuilder_ == null) {
if (((bitField0_ & 0x00010000) != 0) &&
enforcingFailurePercentage_ != null &&
enforcingFailurePercentage_ != com.google.protobuf.UInt32Value.getDefaultInstance()) {
getEnforcingFailurePercentageBuilder().mergeFrom(value);
} else {
enforcingFailurePercentage_ = value;
}
} else {
enforcingFailurePercentageBuilder_.mergeFrom(value);
}
if (enforcingFailurePercentage_ != null) {
bitField0_ |= 0x00010000;
onChanged();
}
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* failure percentage statistics. This setting can be used to disable ejection or to ramp it up
* slowly. Defaults to 0.
*
* [#next-major-version: setting this without setting failure_percentage_threshold should be
* invalid in v4.]
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage = 17 [(.validate.rules) = { ... }
*/
public Builder clearEnforcingFailurePercentage() {
bitField0_ = (bitField0_ & ~0x00010000);
enforcingFailurePercentage_ = null;
if (enforcingFailurePercentageBuilder_ != null) {
enforcingFailurePercentageBuilder_.dispose();
enforcingFailurePercentageBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* failure percentage statistics. This setting can be used to disable ejection or to ramp it up
* slowly. Defaults to 0.
*
* [#next-major-version: setting this without setting failure_percentage_threshold should be
* invalid in v4.]
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage = 17 [(.validate.rules) = { ... }
*/
public com.google.protobuf.UInt32Value.Builder getEnforcingFailurePercentageBuilder() {
bitField0_ |= 0x00010000;
onChanged();
return getEnforcingFailurePercentageFieldBuilder().getBuilder();
}
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* failure percentage statistics. This setting can be used to disable ejection or to ramp it up
* slowly. Defaults to 0.
*
* [#next-major-version: setting this without setting failure_percentage_threshold should be
* invalid in v4.]
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage = 17 [(.validate.rules) = { ... }
*/
public com.google.protobuf.UInt32ValueOrBuilder getEnforcingFailurePercentageOrBuilder() {
if (enforcingFailurePercentageBuilder_ != null) {
return enforcingFailurePercentageBuilder_.getMessageOrBuilder();
} else {
return enforcingFailurePercentage_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingFailurePercentage_;
}
}
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* failure percentage statistics. This setting can be used to disable ejection or to ramp it up
* slowly. Defaults to 0.
*
* [#next-major-version: setting this without setting failure_percentage_threshold should be
* invalid in v4.]
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage = 17 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getEnforcingFailurePercentageFieldBuilder() {
if (enforcingFailurePercentageBuilder_ == null) {
enforcingFailurePercentageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getEnforcingFailurePercentage(),
getParentForChildren(),
isClean());
enforcingFailurePercentage_ = null;
}
return enforcingFailurePercentageBuilder_;
}
private com.google.protobuf.UInt32Value enforcingFailurePercentageLocalOrigin_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> enforcingFailurePercentageLocalOriginBuilder_;
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* local-origin failure percentage statistics. This setting can be used to disable ejection or to
* ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage_local_origin = 18 [(.validate.rules) = { ... }
* @return Whether the enforcingFailurePercentageLocalOrigin field is set.
*/
public boolean hasEnforcingFailurePercentageLocalOrigin() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* local-origin failure percentage statistics. This setting can be used to disable ejection or to
* ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage_local_origin = 18 [(.validate.rules) = { ... }
* @return The enforcingFailurePercentageLocalOrigin.
*/
public com.google.protobuf.UInt32Value getEnforcingFailurePercentageLocalOrigin() {
if (enforcingFailurePercentageLocalOriginBuilder_ == null) {
return enforcingFailurePercentageLocalOrigin_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingFailurePercentageLocalOrigin_;
} else {
return enforcingFailurePercentageLocalOriginBuilder_.getMessage();
}
}
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* local-origin failure percentage statistics. This setting can be used to disable ejection or to
* ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage_local_origin = 18 [(.validate.rules) = { ... }
*/
public Builder setEnforcingFailurePercentageLocalOrigin(com.google.protobuf.UInt32Value value) {
if (enforcingFailurePercentageLocalOriginBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
enforcingFailurePercentageLocalOrigin_ = value;
} else {
enforcingFailurePercentageLocalOriginBuilder_.setMessage(value);
}
bitField0_ |= 0x00020000;
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* local-origin failure percentage statistics. This setting can be used to disable ejection or to
* ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage_local_origin = 18 [(.validate.rules) = { ... }
*/
public Builder setEnforcingFailurePercentageLocalOrigin(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (enforcingFailurePercentageLocalOriginBuilder_ == null) {
enforcingFailurePercentageLocalOrigin_ = builderForValue.build();
} else {
enforcingFailurePercentageLocalOriginBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00020000;
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* local-origin failure percentage statistics. This setting can be used to disable ejection or to
* ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage_local_origin = 18 [(.validate.rules) = { ... }
*/
public Builder mergeEnforcingFailurePercentageLocalOrigin(com.google.protobuf.UInt32Value value) {
if (enforcingFailurePercentageLocalOriginBuilder_ == null) {
if (((bitField0_ & 0x00020000) != 0) &&
enforcingFailurePercentageLocalOrigin_ != null &&
enforcingFailurePercentageLocalOrigin_ != com.google.protobuf.UInt32Value.getDefaultInstance()) {
getEnforcingFailurePercentageLocalOriginBuilder().mergeFrom(value);
} else {
enforcingFailurePercentageLocalOrigin_ = value;
}
} else {
enforcingFailurePercentageLocalOriginBuilder_.mergeFrom(value);
}
if (enforcingFailurePercentageLocalOrigin_ != null) {
bitField0_ |= 0x00020000;
onChanged();
}
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* local-origin failure percentage statistics. This setting can be used to disable ejection or to
* ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage_local_origin = 18 [(.validate.rules) = { ... }
*/
public Builder clearEnforcingFailurePercentageLocalOrigin() {
bitField0_ = (bitField0_ & ~0x00020000);
enforcingFailurePercentageLocalOrigin_ = null;
if (enforcingFailurePercentageLocalOriginBuilder_ != null) {
enforcingFailurePercentageLocalOriginBuilder_.dispose();
enforcingFailurePercentageLocalOriginBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* local-origin failure percentage statistics. This setting can be used to disable ejection or to
* ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage_local_origin = 18 [(.validate.rules) = { ... }
*/
public com.google.protobuf.UInt32Value.Builder getEnforcingFailurePercentageLocalOriginBuilder() {
bitField0_ |= 0x00020000;
onChanged();
return getEnforcingFailurePercentageLocalOriginFieldBuilder().getBuilder();
}
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* local-origin failure percentage statistics. This setting can be used to disable ejection or to
* ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage_local_origin = 18 [(.validate.rules) = { ... }
*/
public com.google.protobuf.UInt32ValueOrBuilder getEnforcingFailurePercentageLocalOriginOrBuilder() {
if (enforcingFailurePercentageLocalOriginBuilder_ != null) {
return enforcingFailurePercentageLocalOriginBuilder_.getMessageOrBuilder();
} else {
return enforcingFailurePercentageLocalOrigin_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : enforcingFailurePercentageLocalOrigin_;
}
}
/**
*
* The % chance that a host will be actually ejected when an outlier status is detected through
* local-origin failure percentage statistics. This setting can be used to disable ejection or to
* ramp it up slowly. Defaults to 0.
*
*
* .google.protobuf.UInt32Value enforcing_failure_percentage_local_origin = 18 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getEnforcingFailurePercentageLocalOriginFieldBuilder() {
if (enforcingFailurePercentageLocalOriginBuilder_ == null) {
enforcingFailurePercentageLocalOriginBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getEnforcingFailurePercentageLocalOrigin(),
getParentForChildren(),
isClean());
enforcingFailurePercentageLocalOrigin_ = null;
}
return enforcingFailurePercentageLocalOriginBuilder_;
}
private com.google.protobuf.UInt32Value failurePercentageMinimumHosts_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> failurePercentageMinimumHostsBuilder_;
/**
*
* The minimum number of hosts in a cluster in order to perform failure percentage-based ejection.
* If the total number of hosts in the cluster is less than this value, failure percentage-based
* ejection will not be performed. Defaults to 5.
*
*
* .google.protobuf.UInt32Value failure_percentage_minimum_hosts = 19;
* @return Whether the failurePercentageMinimumHosts field is set.
*/
public boolean hasFailurePercentageMinimumHosts() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
*
* The minimum number of hosts in a cluster in order to perform failure percentage-based ejection.
* If the total number of hosts in the cluster is less than this value, failure percentage-based
* ejection will not be performed. Defaults to 5.
*
*
* .google.protobuf.UInt32Value failure_percentage_minimum_hosts = 19;
* @return The failurePercentageMinimumHosts.
*/
public com.google.protobuf.UInt32Value getFailurePercentageMinimumHosts() {
if (failurePercentageMinimumHostsBuilder_ == null) {
return failurePercentageMinimumHosts_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : failurePercentageMinimumHosts_;
} else {
return failurePercentageMinimumHostsBuilder_.getMessage();
}
}
/**
*
* The minimum number of hosts in a cluster in order to perform failure percentage-based ejection.
* If the total number of hosts in the cluster is less than this value, failure percentage-based
* ejection will not be performed. Defaults to 5.
*
*
* .google.protobuf.UInt32Value failure_percentage_minimum_hosts = 19;
*/
public Builder setFailurePercentageMinimumHosts(com.google.protobuf.UInt32Value value) {
if (failurePercentageMinimumHostsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
failurePercentageMinimumHosts_ = value;
} else {
failurePercentageMinimumHostsBuilder_.setMessage(value);
}
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
*
* The minimum number of hosts in a cluster in order to perform failure percentage-based ejection.
* If the total number of hosts in the cluster is less than this value, failure percentage-based
* ejection will not be performed. Defaults to 5.
*
*
* .google.protobuf.UInt32Value failure_percentage_minimum_hosts = 19;
*/
public Builder setFailurePercentageMinimumHosts(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (failurePercentageMinimumHostsBuilder_ == null) {
failurePercentageMinimumHosts_ = builderForValue.build();
} else {
failurePercentageMinimumHostsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
*
* The minimum number of hosts in a cluster in order to perform failure percentage-based ejection.
* If the total number of hosts in the cluster is less than this value, failure percentage-based
* ejection will not be performed. Defaults to 5.
*
*
* .google.protobuf.UInt32Value failure_percentage_minimum_hosts = 19;
*/
public Builder mergeFailurePercentageMinimumHosts(com.google.protobuf.UInt32Value value) {
if (failurePercentageMinimumHostsBuilder_ == null) {
if (((bitField0_ & 0x00040000) != 0) &&
failurePercentageMinimumHosts_ != null &&
failurePercentageMinimumHosts_ != com.google.protobuf.UInt32Value.getDefaultInstance()) {
getFailurePercentageMinimumHostsBuilder().mergeFrom(value);
} else {
failurePercentageMinimumHosts_ = value;
}
} else {
failurePercentageMinimumHostsBuilder_.mergeFrom(value);
}
if (failurePercentageMinimumHosts_ != null) {
bitField0_ |= 0x00040000;
onChanged();
}
return this;
}
/**
*
* The minimum number of hosts in a cluster in order to perform failure percentage-based ejection.
* If the total number of hosts in the cluster is less than this value, failure percentage-based
* ejection will not be performed. Defaults to 5.
*
*
* .google.protobuf.UInt32Value failure_percentage_minimum_hosts = 19;
*/
public Builder clearFailurePercentageMinimumHosts() {
bitField0_ = (bitField0_ & ~0x00040000);
failurePercentageMinimumHosts_ = null;
if (failurePercentageMinimumHostsBuilder_ != null) {
failurePercentageMinimumHostsBuilder_.dispose();
failurePercentageMinimumHostsBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The minimum number of hosts in a cluster in order to perform failure percentage-based ejection.
* If the total number of hosts in the cluster is less than this value, failure percentage-based
* ejection will not be performed. Defaults to 5.
*
*
* .google.protobuf.UInt32Value failure_percentage_minimum_hosts = 19;
*/
public com.google.protobuf.UInt32Value.Builder getFailurePercentageMinimumHostsBuilder() {
bitField0_ |= 0x00040000;
onChanged();
return getFailurePercentageMinimumHostsFieldBuilder().getBuilder();
}
/**
*
* The minimum number of hosts in a cluster in order to perform failure percentage-based ejection.
* If the total number of hosts in the cluster is less than this value, failure percentage-based
* ejection will not be performed. Defaults to 5.
*
*
* .google.protobuf.UInt32Value failure_percentage_minimum_hosts = 19;
*/
public com.google.protobuf.UInt32ValueOrBuilder getFailurePercentageMinimumHostsOrBuilder() {
if (failurePercentageMinimumHostsBuilder_ != null) {
return failurePercentageMinimumHostsBuilder_.getMessageOrBuilder();
} else {
return failurePercentageMinimumHosts_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : failurePercentageMinimumHosts_;
}
}
/**
*
* The minimum number of hosts in a cluster in order to perform failure percentage-based ejection.
* If the total number of hosts in the cluster is less than this value, failure percentage-based
* ejection will not be performed. Defaults to 5.
*
*
* .google.protobuf.UInt32Value failure_percentage_minimum_hosts = 19;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getFailurePercentageMinimumHostsFieldBuilder() {
if (failurePercentageMinimumHostsBuilder_ == null) {
failurePercentageMinimumHostsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getFailurePercentageMinimumHosts(),
getParentForChildren(),
isClean());
failurePercentageMinimumHosts_ = null;
}
return failurePercentageMinimumHostsBuilder_;
}
private com.google.protobuf.UInt32Value failurePercentageRequestVolume_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> failurePercentageRequestVolumeBuilder_;
/**
*
* The minimum number of total requests that must be collected in one interval (as defined by the
* interval duration above) to perform failure percentage-based ejection for this host. If the
* volume is lower than this setting, failure percentage-based ejection will not be performed for
* this host. Defaults to 50.
*
*
* .google.protobuf.UInt32Value failure_percentage_request_volume = 20;
* @return Whether the failurePercentageRequestVolume field is set.
*/
public boolean hasFailurePercentageRequestVolume() {
return ((bitField0_ & 0x00080000) != 0);
}
/**
*
* The minimum number of total requests that must be collected in one interval (as defined by the
* interval duration above) to perform failure percentage-based ejection for this host. If the
* volume is lower than this setting, failure percentage-based ejection will not be performed for
* this host. Defaults to 50.
*
*
* .google.protobuf.UInt32Value failure_percentage_request_volume = 20;
* @return The failurePercentageRequestVolume.
*/
public com.google.protobuf.UInt32Value getFailurePercentageRequestVolume() {
if (failurePercentageRequestVolumeBuilder_ == null) {
return failurePercentageRequestVolume_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : failurePercentageRequestVolume_;
} else {
return failurePercentageRequestVolumeBuilder_.getMessage();
}
}
/**
*
* The minimum number of total requests that must be collected in one interval (as defined by the
* interval duration above) to perform failure percentage-based ejection for this host. If the
* volume is lower than this setting, failure percentage-based ejection will not be performed for
* this host. Defaults to 50.
*
*
* .google.protobuf.UInt32Value failure_percentage_request_volume = 20;
*/
public Builder setFailurePercentageRequestVolume(com.google.protobuf.UInt32Value value) {
if (failurePercentageRequestVolumeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
failurePercentageRequestVolume_ = value;
} else {
failurePercentageRequestVolumeBuilder_.setMessage(value);
}
bitField0_ |= 0x00080000;
onChanged();
return this;
}
/**
*
* The minimum number of total requests that must be collected in one interval (as defined by the
* interval duration above) to perform failure percentage-based ejection for this host. If the
* volume is lower than this setting, failure percentage-based ejection will not be performed for
* this host. Defaults to 50.
*
*
* .google.protobuf.UInt32Value failure_percentage_request_volume = 20;
*/
public Builder setFailurePercentageRequestVolume(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (failurePercentageRequestVolumeBuilder_ == null) {
failurePercentageRequestVolume_ = builderForValue.build();
} else {
failurePercentageRequestVolumeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00080000;
onChanged();
return this;
}
/**
*
* The minimum number of total requests that must be collected in one interval (as defined by the
* interval duration above) to perform failure percentage-based ejection for this host. If the
* volume is lower than this setting, failure percentage-based ejection will not be performed for
* this host. Defaults to 50.
*
*
* .google.protobuf.UInt32Value failure_percentage_request_volume = 20;
*/
public Builder mergeFailurePercentageRequestVolume(com.google.protobuf.UInt32Value value) {
if (failurePercentageRequestVolumeBuilder_ == null) {
if (((bitField0_ & 0x00080000) != 0) &&
failurePercentageRequestVolume_ != null &&
failurePercentageRequestVolume_ != com.google.protobuf.UInt32Value.getDefaultInstance()) {
getFailurePercentageRequestVolumeBuilder().mergeFrom(value);
} else {
failurePercentageRequestVolume_ = value;
}
} else {
failurePercentageRequestVolumeBuilder_.mergeFrom(value);
}
if (failurePercentageRequestVolume_ != null) {
bitField0_ |= 0x00080000;
onChanged();
}
return this;
}
/**
*
* The minimum number of total requests that must be collected in one interval (as defined by the
* interval duration above) to perform failure percentage-based ejection for this host. If the
* volume is lower than this setting, failure percentage-based ejection will not be performed for
* this host. Defaults to 50.
*
*
* .google.protobuf.UInt32Value failure_percentage_request_volume = 20;
*/
public Builder clearFailurePercentageRequestVolume() {
bitField0_ = (bitField0_ & ~0x00080000);
failurePercentageRequestVolume_ = null;
if (failurePercentageRequestVolumeBuilder_ != null) {
failurePercentageRequestVolumeBuilder_.dispose();
failurePercentageRequestVolumeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The minimum number of total requests that must be collected in one interval (as defined by the
* interval duration above) to perform failure percentage-based ejection for this host. If the
* volume is lower than this setting, failure percentage-based ejection will not be performed for
* this host. Defaults to 50.
*
*
* .google.protobuf.UInt32Value failure_percentage_request_volume = 20;
*/
public com.google.protobuf.UInt32Value.Builder getFailurePercentageRequestVolumeBuilder() {
bitField0_ |= 0x00080000;
onChanged();
return getFailurePercentageRequestVolumeFieldBuilder().getBuilder();
}
/**
*
* The minimum number of total requests that must be collected in one interval (as defined by the
* interval duration above) to perform failure percentage-based ejection for this host. If the
* volume is lower than this setting, failure percentage-based ejection will not be performed for
* this host. Defaults to 50.
*
*
* .google.protobuf.UInt32Value failure_percentage_request_volume = 20;
*/
public com.google.protobuf.UInt32ValueOrBuilder getFailurePercentageRequestVolumeOrBuilder() {
if (failurePercentageRequestVolumeBuilder_ != null) {
return failurePercentageRequestVolumeBuilder_.getMessageOrBuilder();
} else {
return failurePercentageRequestVolume_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : failurePercentageRequestVolume_;
}
}
/**
*
* The minimum number of total requests that must be collected in one interval (as defined by the
* interval duration above) to perform failure percentage-based ejection for this host. If the
* volume is lower than this setting, failure percentage-based ejection will not be performed for
* this host. Defaults to 50.
*
*
* .google.protobuf.UInt32Value failure_percentage_request_volume = 20;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getFailurePercentageRequestVolumeFieldBuilder() {
if (failurePercentageRequestVolumeBuilder_ == null) {
failurePercentageRequestVolumeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getFailurePercentageRequestVolume(),
getParentForChildren(),
isClean());
failurePercentageRequestVolume_ = null;
}
return failurePercentageRequestVolumeBuilder_;
}
private com.google.protobuf.Duration maxEjectionTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder> maxEjectionTimeBuilder_;
/**
*
* The maximum time that a host is ejected for. See :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* for more information. If not specified, the default value (300000ms or 300s) or
* :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>` value is applied, whatever is larger.
*
*
* .google.protobuf.Duration max_ejection_time = 21 [(.validate.rules) = { ... }
* @return Whether the maxEjectionTime field is set.
*/
public boolean hasMaxEjectionTime() {
return ((bitField0_ & 0x00100000) != 0);
}
/**
*
* The maximum time that a host is ejected for. See :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* for more information. If not specified, the default value (300000ms or 300s) or
* :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>` value is applied, whatever is larger.
*
*
* .google.protobuf.Duration max_ejection_time = 21 [(.validate.rules) = { ... }
* @return The maxEjectionTime.
*/
public com.google.protobuf.Duration getMaxEjectionTime() {
if (maxEjectionTimeBuilder_ == null) {
return maxEjectionTime_ == null ? com.google.protobuf.Duration.getDefaultInstance() : maxEjectionTime_;
} else {
return maxEjectionTimeBuilder_.getMessage();
}
}
/**
*
* The maximum time that a host is ejected for. See :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* for more information. If not specified, the default value (300000ms or 300s) or
* :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>` value is applied, whatever is larger.
*
*
* .google.protobuf.Duration max_ejection_time = 21 [(.validate.rules) = { ... }
*/
public Builder setMaxEjectionTime(com.google.protobuf.Duration value) {
if (maxEjectionTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
maxEjectionTime_ = value;
} else {
maxEjectionTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00100000;
onChanged();
return this;
}
/**
*
* The maximum time that a host is ejected for. See :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* for more information. If not specified, the default value (300000ms or 300s) or
* :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>` value is applied, whatever is larger.
*
*
* .google.protobuf.Duration max_ejection_time = 21 [(.validate.rules) = { ... }
*/
public Builder setMaxEjectionTime(
com.google.protobuf.Duration.Builder builderForValue) {
if (maxEjectionTimeBuilder_ == null) {
maxEjectionTime_ = builderForValue.build();
} else {
maxEjectionTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00100000;
onChanged();
return this;
}
/**
*
* The maximum time that a host is ejected for. See :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* for more information. If not specified, the default value (300000ms or 300s) or
* :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>` value is applied, whatever is larger.
*
*
* .google.protobuf.Duration max_ejection_time = 21 [(.validate.rules) = { ... }
*/
public Builder mergeMaxEjectionTime(com.google.protobuf.Duration value) {
if (maxEjectionTimeBuilder_ == null) {
if (((bitField0_ & 0x00100000) != 0) &&
maxEjectionTime_ != null &&
maxEjectionTime_ != com.google.protobuf.Duration.getDefaultInstance()) {
getMaxEjectionTimeBuilder().mergeFrom(value);
} else {
maxEjectionTime_ = value;
}
} else {
maxEjectionTimeBuilder_.mergeFrom(value);
}
if (maxEjectionTime_ != null) {
bitField0_ |= 0x00100000;
onChanged();
}
return this;
}
/**
*
* The maximum time that a host is ejected for. See :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* for more information. If not specified, the default value (300000ms or 300s) or
* :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>` value is applied, whatever is larger.
*
*
* .google.protobuf.Duration max_ejection_time = 21 [(.validate.rules) = { ... }
*/
public Builder clearMaxEjectionTime() {
bitField0_ = (bitField0_ & ~0x00100000);
maxEjectionTime_ = null;
if (maxEjectionTimeBuilder_ != null) {
maxEjectionTimeBuilder_.dispose();
maxEjectionTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The maximum time that a host is ejected for. See :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* for more information. If not specified, the default value (300000ms or 300s) or
* :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>` value is applied, whatever is larger.
*
*
* .google.protobuf.Duration max_ejection_time = 21 [(.validate.rules) = { ... }
*/
public com.google.protobuf.Duration.Builder getMaxEjectionTimeBuilder() {
bitField0_ |= 0x00100000;
onChanged();
return getMaxEjectionTimeFieldBuilder().getBuilder();
}
/**
*
* The maximum time that a host is ejected for. See :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* for more information. If not specified, the default value (300000ms or 300s) or
* :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>` value is applied, whatever is larger.
*
*
* .google.protobuf.Duration max_ejection_time = 21 [(.validate.rules) = { ... }
*/
public com.google.protobuf.DurationOrBuilder getMaxEjectionTimeOrBuilder() {
if (maxEjectionTimeBuilder_ != null) {
return maxEjectionTimeBuilder_.getMessageOrBuilder();
} else {
return maxEjectionTime_ == null ?
com.google.protobuf.Duration.getDefaultInstance() : maxEjectionTime_;
}
}
/**
*
* The maximum time that a host is ejected for. See :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* for more information. If not specified, the default value (300000ms or 300s) or
* :ref:`base_ejection_time<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>` value is applied, whatever is larger.
*
*
* .google.protobuf.Duration max_ejection_time = 21 [(.validate.rules) = { ... }
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>
getMaxEjectionTimeFieldBuilder() {
if (maxEjectionTimeBuilder_ == null) {
maxEjectionTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>(
getMaxEjectionTime(),
getParentForChildren(),
isClean());
maxEjectionTime_ = null;
}
return maxEjectionTimeBuilder_;
}
private com.google.protobuf.Duration maxEjectionTimeJitter_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder> maxEjectionTimeJitterBuilder_;
/**
*
* The maximum amount of jitter to add to the ejection time, in order to prevent
* a 'thundering herd' effect where all proxies try to reconnect to host at the same time.
* See :ref:`max_ejection_time_jitter<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* Defaults to 0s.
*
*
* .google.protobuf.Duration max_ejection_time_jitter = 22;
* @return Whether the maxEjectionTimeJitter field is set.
*/
public boolean hasMaxEjectionTimeJitter() {
return ((bitField0_ & 0x00200000) != 0);
}
/**
*
* The maximum amount of jitter to add to the ejection time, in order to prevent
* a 'thundering herd' effect where all proxies try to reconnect to host at the same time.
* See :ref:`max_ejection_time_jitter<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* Defaults to 0s.
*
*
* .google.protobuf.Duration max_ejection_time_jitter = 22;
* @return The maxEjectionTimeJitter.
*/
public com.google.protobuf.Duration getMaxEjectionTimeJitter() {
if (maxEjectionTimeJitterBuilder_ == null) {
return maxEjectionTimeJitter_ == null ? com.google.protobuf.Duration.getDefaultInstance() : maxEjectionTimeJitter_;
} else {
return maxEjectionTimeJitterBuilder_.getMessage();
}
}
/**
*
* The maximum amount of jitter to add to the ejection time, in order to prevent
* a 'thundering herd' effect where all proxies try to reconnect to host at the same time.
* See :ref:`max_ejection_time_jitter<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* Defaults to 0s.
*
*
* .google.protobuf.Duration max_ejection_time_jitter = 22;
*/
public Builder setMaxEjectionTimeJitter(com.google.protobuf.Duration value) {
if (maxEjectionTimeJitterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
maxEjectionTimeJitter_ = value;
} else {
maxEjectionTimeJitterBuilder_.setMessage(value);
}
bitField0_ |= 0x00200000;
onChanged();
return this;
}
/**
*
* The maximum amount of jitter to add to the ejection time, in order to prevent
* a 'thundering herd' effect where all proxies try to reconnect to host at the same time.
* See :ref:`max_ejection_time_jitter<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* Defaults to 0s.
*
*
* .google.protobuf.Duration max_ejection_time_jitter = 22;
*/
public Builder setMaxEjectionTimeJitter(
com.google.protobuf.Duration.Builder builderForValue) {
if (maxEjectionTimeJitterBuilder_ == null) {
maxEjectionTimeJitter_ = builderForValue.build();
} else {
maxEjectionTimeJitterBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00200000;
onChanged();
return this;
}
/**
*
* The maximum amount of jitter to add to the ejection time, in order to prevent
* a 'thundering herd' effect where all proxies try to reconnect to host at the same time.
* See :ref:`max_ejection_time_jitter<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* Defaults to 0s.
*
*
* .google.protobuf.Duration max_ejection_time_jitter = 22;
*/
public Builder mergeMaxEjectionTimeJitter(com.google.protobuf.Duration value) {
if (maxEjectionTimeJitterBuilder_ == null) {
if (((bitField0_ & 0x00200000) != 0) &&
maxEjectionTimeJitter_ != null &&
maxEjectionTimeJitter_ != com.google.protobuf.Duration.getDefaultInstance()) {
getMaxEjectionTimeJitterBuilder().mergeFrom(value);
} else {
maxEjectionTimeJitter_ = value;
}
} else {
maxEjectionTimeJitterBuilder_.mergeFrom(value);
}
if (maxEjectionTimeJitter_ != null) {
bitField0_ |= 0x00200000;
onChanged();
}
return this;
}
/**
*
* The maximum amount of jitter to add to the ejection time, in order to prevent
* a 'thundering herd' effect where all proxies try to reconnect to host at the same time.
* See :ref:`max_ejection_time_jitter<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* Defaults to 0s.
*
*
* .google.protobuf.Duration max_ejection_time_jitter = 22;
*/
public Builder clearMaxEjectionTimeJitter() {
bitField0_ = (bitField0_ & ~0x00200000);
maxEjectionTimeJitter_ = null;
if (maxEjectionTimeJitterBuilder_ != null) {
maxEjectionTimeJitterBuilder_.dispose();
maxEjectionTimeJitterBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The maximum amount of jitter to add to the ejection time, in order to prevent
* a 'thundering herd' effect where all proxies try to reconnect to host at the same time.
* See :ref:`max_ejection_time_jitter<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* Defaults to 0s.
*
*
* .google.protobuf.Duration max_ejection_time_jitter = 22;
*/
public com.google.protobuf.Duration.Builder getMaxEjectionTimeJitterBuilder() {
bitField0_ |= 0x00200000;
onChanged();
return getMaxEjectionTimeJitterFieldBuilder().getBuilder();
}
/**
*
* The maximum amount of jitter to add to the ejection time, in order to prevent
* a 'thundering herd' effect where all proxies try to reconnect to host at the same time.
* See :ref:`max_ejection_time_jitter<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* Defaults to 0s.
*
*
* .google.protobuf.Duration max_ejection_time_jitter = 22;
*/
public com.google.protobuf.DurationOrBuilder getMaxEjectionTimeJitterOrBuilder() {
if (maxEjectionTimeJitterBuilder_ != null) {
return maxEjectionTimeJitterBuilder_.getMessageOrBuilder();
} else {
return maxEjectionTimeJitter_ == null ?
com.google.protobuf.Duration.getDefaultInstance() : maxEjectionTimeJitter_;
}
}
/**
*
* The maximum amount of jitter to add to the ejection time, in order to prevent
* a 'thundering herd' effect where all proxies try to reconnect to host at the same time.
* See :ref:`max_ejection_time_jitter<envoy_v3_api_field_config.cluster.v3.OutlierDetection.base_ejection_time>`
* Defaults to 0s.
*
*
* .google.protobuf.Duration max_ejection_time_jitter = 22;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>
getMaxEjectionTimeJitterFieldBuilder() {
if (maxEjectionTimeJitterBuilder_ == null) {
maxEjectionTimeJitterBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>(
getMaxEjectionTimeJitter(),
getParentForChildren(),
isClean());
maxEjectionTimeJitter_ = null;
}
return maxEjectionTimeJitterBuilder_;
}
private com.google.protobuf.BoolValue successfulActiveHealthCheckUnejectHost_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder> successfulActiveHealthCheckUnejectHostBuilder_;
/**
*
* If active health checking is enabled and a host is ejected by outlier detection, a successful active health check
* unejects the host by default and considers it as healthy. Unejection also clears all the outlier detection counters.
* To change this default behavior set this config to ``false`` where active health checking will not uneject the host.
* Defaults to true.
*
*
* .google.protobuf.BoolValue successful_active_health_check_uneject_host = 23;
* @return Whether the successfulActiveHealthCheckUnejectHost field is set.
*/
public boolean hasSuccessfulActiveHealthCheckUnejectHost() {
return ((bitField0_ & 0x00400000) != 0);
}
/**
*
* If active health checking is enabled and a host is ejected by outlier detection, a successful active health check
* unejects the host by default and considers it as healthy. Unejection also clears all the outlier detection counters.
* To change this default behavior set this config to ``false`` where active health checking will not uneject the host.
* Defaults to true.
*
*
* .google.protobuf.BoolValue successful_active_health_check_uneject_host = 23;
* @return The successfulActiveHealthCheckUnejectHost.
*/
public com.google.protobuf.BoolValue getSuccessfulActiveHealthCheckUnejectHost() {
if (successfulActiveHealthCheckUnejectHostBuilder_ == null) {
return successfulActiveHealthCheckUnejectHost_ == null ? com.google.protobuf.BoolValue.getDefaultInstance() : successfulActiveHealthCheckUnejectHost_;
} else {
return successfulActiveHealthCheckUnejectHostBuilder_.getMessage();
}
}
/**
*
* If active health checking is enabled and a host is ejected by outlier detection, a successful active health check
* unejects the host by default and considers it as healthy. Unejection also clears all the outlier detection counters.
* To change this default behavior set this config to ``false`` where active health checking will not uneject the host.
* Defaults to true.
*
*
* .google.protobuf.BoolValue successful_active_health_check_uneject_host = 23;
*/
public Builder setSuccessfulActiveHealthCheckUnejectHost(com.google.protobuf.BoolValue value) {
if (successfulActiveHealthCheckUnejectHostBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
successfulActiveHealthCheckUnejectHost_ = value;
} else {
successfulActiveHealthCheckUnejectHostBuilder_.setMessage(value);
}
bitField0_ |= 0x00400000;
onChanged();
return this;
}
/**
*
* If active health checking is enabled and a host is ejected by outlier detection, a successful active health check
* unejects the host by default and considers it as healthy. Unejection also clears all the outlier detection counters.
* To change this default behavior set this config to ``false`` where active health checking will not uneject the host.
* Defaults to true.
*
*
* .google.protobuf.BoolValue successful_active_health_check_uneject_host = 23;
*/
public Builder setSuccessfulActiveHealthCheckUnejectHost(
com.google.protobuf.BoolValue.Builder builderForValue) {
if (successfulActiveHealthCheckUnejectHostBuilder_ == null) {
successfulActiveHealthCheckUnejectHost_ = builderForValue.build();
} else {
successfulActiveHealthCheckUnejectHostBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00400000;
onChanged();
return this;
}
/**
*
* If active health checking is enabled and a host is ejected by outlier detection, a successful active health check
* unejects the host by default and considers it as healthy. Unejection also clears all the outlier detection counters.
* To change this default behavior set this config to ``false`` where active health checking will not uneject the host.
* Defaults to true.
*
*
* .google.protobuf.BoolValue successful_active_health_check_uneject_host = 23;
*/
public Builder mergeSuccessfulActiveHealthCheckUnejectHost(com.google.protobuf.BoolValue value) {
if (successfulActiveHealthCheckUnejectHostBuilder_ == null) {
if (((bitField0_ & 0x00400000) != 0) &&
successfulActiveHealthCheckUnejectHost_ != null &&
successfulActiveHealthCheckUnejectHost_ != com.google.protobuf.BoolValue.getDefaultInstance()) {
getSuccessfulActiveHealthCheckUnejectHostBuilder().mergeFrom(value);
} else {
successfulActiveHealthCheckUnejectHost_ = value;
}
} else {
successfulActiveHealthCheckUnejectHostBuilder_.mergeFrom(value);
}
if (successfulActiveHealthCheckUnejectHost_ != null) {
bitField0_ |= 0x00400000;
onChanged();
}
return this;
}
/**
*
* If active health checking is enabled and a host is ejected by outlier detection, a successful active health check
* unejects the host by default and considers it as healthy. Unejection also clears all the outlier detection counters.
* To change this default behavior set this config to ``false`` where active health checking will not uneject the host.
* Defaults to true.
*
*
* .google.protobuf.BoolValue successful_active_health_check_uneject_host = 23;
*/
public Builder clearSuccessfulActiveHealthCheckUnejectHost() {
bitField0_ = (bitField0_ & ~0x00400000);
successfulActiveHealthCheckUnejectHost_ = null;
if (successfulActiveHealthCheckUnejectHostBuilder_ != null) {
successfulActiveHealthCheckUnejectHostBuilder_.dispose();
successfulActiveHealthCheckUnejectHostBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* If active health checking is enabled and a host is ejected by outlier detection, a successful active health check
* unejects the host by default and considers it as healthy. Unejection also clears all the outlier detection counters.
* To change this default behavior set this config to ``false`` where active health checking will not uneject the host.
* Defaults to true.
*
*
* .google.protobuf.BoolValue successful_active_health_check_uneject_host = 23;
*/
public com.google.protobuf.BoolValue.Builder getSuccessfulActiveHealthCheckUnejectHostBuilder() {
bitField0_ |= 0x00400000;
onChanged();
return getSuccessfulActiveHealthCheckUnejectHostFieldBuilder().getBuilder();
}
/**
*
* If active health checking is enabled and a host is ejected by outlier detection, a successful active health check
* unejects the host by default and considers it as healthy. Unejection also clears all the outlier detection counters.
* To change this default behavior set this config to ``false`` where active health checking will not uneject the host.
* Defaults to true.
*
*
* .google.protobuf.BoolValue successful_active_health_check_uneject_host = 23;
*/
public com.google.protobuf.BoolValueOrBuilder getSuccessfulActiveHealthCheckUnejectHostOrBuilder() {
if (successfulActiveHealthCheckUnejectHostBuilder_ != null) {
return successfulActiveHealthCheckUnejectHostBuilder_.getMessageOrBuilder();
} else {
return successfulActiveHealthCheckUnejectHost_ == null ?
com.google.protobuf.BoolValue.getDefaultInstance() : successfulActiveHealthCheckUnejectHost_;
}
}
/**
*
* If active health checking is enabled and a host is ejected by outlier detection, a successful active health check
* unejects the host by default and considers it as healthy. Unejection also clears all the outlier detection counters.
* To change this default behavior set this config to ``false`` where active health checking will not uneject the host.
* Defaults to true.
*
*
* .google.protobuf.BoolValue successful_active_health_check_uneject_host = 23;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder>
getSuccessfulActiveHealthCheckUnejectHostFieldBuilder() {
if (successfulActiveHealthCheckUnejectHostBuilder_ == null) {
successfulActiveHealthCheckUnejectHostBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder>(
getSuccessfulActiveHealthCheckUnejectHost(),
getParentForChildren(),
isClean());
successfulActiveHealthCheckUnejectHost_ = null;
}
return successfulActiveHealthCheckUnejectHostBuilder_;
}
private java.util.List monitors_ =
java.util.Collections.emptyList();
private void ensureMonitorsIsMutable() {
if (!((bitField0_ & 0x00800000) != 0)) {
monitors_ = new java.util.ArrayList(monitors_);
bitField0_ |= 0x00800000;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig, io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig.Builder, io.envoyproxy.envoy.config.core.v3.TypedExtensionConfigOrBuilder> monitorsBuilder_;
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
public java.util.List getMonitorsList() {
if (monitorsBuilder_ == null) {
return java.util.Collections.unmodifiableList(monitors_);
} else {
return monitorsBuilder_.getMessageList();
}
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
public int getMonitorsCount() {
if (monitorsBuilder_ == null) {
return monitors_.size();
} else {
return monitorsBuilder_.getCount();
}
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
public io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig getMonitors(int index) {
if (monitorsBuilder_ == null) {
return monitors_.get(index);
} else {
return monitorsBuilder_.getMessage(index);
}
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
public Builder setMonitors(
int index, io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig value) {
if (monitorsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMonitorsIsMutable();
monitors_.set(index, value);
onChanged();
} else {
monitorsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
public Builder setMonitors(
int index, io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig.Builder builderForValue) {
if (monitorsBuilder_ == null) {
ensureMonitorsIsMutable();
monitors_.set(index, builderForValue.build());
onChanged();
} else {
monitorsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
public Builder addMonitors(io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig value) {
if (monitorsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMonitorsIsMutable();
monitors_.add(value);
onChanged();
} else {
monitorsBuilder_.addMessage(value);
}
return this;
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
public Builder addMonitors(
int index, io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig value) {
if (monitorsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMonitorsIsMutable();
monitors_.add(index, value);
onChanged();
} else {
monitorsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
public Builder addMonitors(
io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig.Builder builderForValue) {
if (monitorsBuilder_ == null) {
ensureMonitorsIsMutable();
monitors_.add(builderForValue.build());
onChanged();
} else {
monitorsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
public Builder addMonitors(
int index, io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig.Builder builderForValue) {
if (monitorsBuilder_ == null) {
ensureMonitorsIsMutable();
monitors_.add(index, builderForValue.build());
onChanged();
} else {
monitorsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
public Builder addAllMonitors(
java.lang.Iterable extends io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig> values) {
if (monitorsBuilder_ == null) {
ensureMonitorsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, monitors_);
onChanged();
} else {
monitorsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
public Builder clearMonitors() {
if (monitorsBuilder_ == null) {
monitors_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00800000);
onChanged();
} else {
monitorsBuilder_.clear();
}
return this;
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
public Builder removeMonitors(int index) {
if (monitorsBuilder_ == null) {
ensureMonitorsIsMutable();
monitors_.remove(index);
onChanged();
} else {
monitorsBuilder_.remove(index);
}
return this;
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
public io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig.Builder getMonitorsBuilder(
int index) {
return getMonitorsFieldBuilder().getBuilder(index);
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
public io.envoyproxy.envoy.config.core.v3.TypedExtensionConfigOrBuilder getMonitorsOrBuilder(
int index) {
if (monitorsBuilder_ == null) {
return monitors_.get(index); } else {
return monitorsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
public java.util.List extends io.envoyproxy.envoy.config.core.v3.TypedExtensionConfigOrBuilder>
getMonitorsOrBuilderList() {
if (monitorsBuilder_ != null) {
return monitorsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(monitors_);
}
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
public io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig.Builder addMonitorsBuilder() {
return getMonitorsFieldBuilder().addBuilder(
io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig.getDefaultInstance());
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
public io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig.Builder addMonitorsBuilder(
int index) {
return getMonitorsFieldBuilder().addBuilder(
index, io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig.getDefaultInstance());
}
/**
*
* Set of host's passive monitors.
* [#not-implemented-hide:]
*
*
* repeated .envoy.config.core.v3.TypedExtensionConfig monitors = 24;
*/
public java.util.List
getMonitorsBuilderList() {
return getMonitorsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig, io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig.Builder, io.envoyproxy.envoy.config.core.v3.TypedExtensionConfigOrBuilder>
getMonitorsFieldBuilder() {
if (monitorsBuilder_ == null) {
monitorsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig, io.envoyproxy.envoy.config.core.v3.TypedExtensionConfig.Builder, io.envoyproxy.envoy.config.core.v3.TypedExtensionConfigOrBuilder>(
monitors_,
((bitField0_ & 0x00800000) != 0),
getParentForChildren(),
isClean());
monitors_ = null;
}
return monitorsBuilder_;
}
private com.google.protobuf.BoolValue alwaysEjectOneHost_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder> alwaysEjectOneHostBuilder_;
/**
*
* If enabled, at least one host is ejected regardless of the value of :ref:`max_ejection_percent<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_percent>`.
* Defaults to false.
*
*
* .google.protobuf.BoolValue always_eject_one_host = 25;
* @return Whether the alwaysEjectOneHost field is set.
*/
public boolean hasAlwaysEjectOneHost() {
return ((bitField0_ & 0x01000000) != 0);
}
/**
*
* If enabled, at least one host is ejected regardless of the value of :ref:`max_ejection_percent<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_percent>`.
* Defaults to false.
*
*
* .google.protobuf.BoolValue always_eject_one_host = 25;
* @return The alwaysEjectOneHost.
*/
public com.google.protobuf.BoolValue getAlwaysEjectOneHost() {
if (alwaysEjectOneHostBuilder_ == null) {
return alwaysEjectOneHost_ == null ? com.google.protobuf.BoolValue.getDefaultInstance() : alwaysEjectOneHost_;
} else {
return alwaysEjectOneHostBuilder_.getMessage();
}
}
/**
*
* If enabled, at least one host is ejected regardless of the value of :ref:`max_ejection_percent<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_percent>`.
* Defaults to false.
*
*
* .google.protobuf.BoolValue always_eject_one_host = 25;
*/
public Builder setAlwaysEjectOneHost(com.google.protobuf.BoolValue value) {
if (alwaysEjectOneHostBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
alwaysEjectOneHost_ = value;
} else {
alwaysEjectOneHostBuilder_.setMessage(value);
}
bitField0_ |= 0x01000000;
onChanged();
return this;
}
/**
*
* If enabled, at least one host is ejected regardless of the value of :ref:`max_ejection_percent<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_percent>`.
* Defaults to false.
*
*
* .google.protobuf.BoolValue always_eject_one_host = 25;
*/
public Builder setAlwaysEjectOneHost(
com.google.protobuf.BoolValue.Builder builderForValue) {
if (alwaysEjectOneHostBuilder_ == null) {
alwaysEjectOneHost_ = builderForValue.build();
} else {
alwaysEjectOneHostBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x01000000;
onChanged();
return this;
}
/**
*
* If enabled, at least one host is ejected regardless of the value of :ref:`max_ejection_percent<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_percent>`.
* Defaults to false.
*
*
* .google.protobuf.BoolValue always_eject_one_host = 25;
*/
public Builder mergeAlwaysEjectOneHost(com.google.protobuf.BoolValue value) {
if (alwaysEjectOneHostBuilder_ == null) {
if (((bitField0_ & 0x01000000) != 0) &&
alwaysEjectOneHost_ != null &&
alwaysEjectOneHost_ != com.google.protobuf.BoolValue.getDefaultInstance()) {
getAlwaysEjectOneHostBuilder().mergeFrom(value);
} else {
alwaysEjectOneHost_ = value;
}
} else {
alwaysEjectOneHostBuilder_.mergeFrom(value);
}
if (alwaysEjectOneHost_ != null) {
bitField0_ |= 0x01000000;
onChanged();
}
return this;
}
/**
*
* If enabled, at least one host is ejected regardless of the value of :ref:`max_ejection_percent<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_percent>`.
* Defaults to false.
*
*
* .google.protobuf.BoolValue always_eject_one_host = 25;
*/
public Builder clearAlwaysEjectOneHost() {
bitField0_ = (bitField0_ & ~0x01000000);
alwaysEjectOneHost_ = null;
if (alwaysEjectOneHostBuilder_ != null) {
alwaysEjectOneHostBuilder_.dispose();
alwaysEjectOneHostBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* If enabled, at least one host is ejected regardless of the value of :ref:`max_ejection_percent<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_percent>`.
* Defaults to false.
*
*
* .google.protobuf.BoolValue always_eject_one_host = 25;
*/
public com.google.protobuf.BoolValue.Builder getAlwaysEjectOneHostBuilder() {
bitField0_ |= 0x01000000;
onChanged();
return getAlwaysEjectOneHostFieldBuilder().getBuilder();
}
/**
*
* If enabled, at least one host is ejected regardless of the value of :ref:`max_ejection_percent<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_percent>`.
* Defaults to false.
*
*
* .google.protobuf.BoolValue always_eject_one_host = 25;
*/
public com.google.protobuf.BoolValueOrBuilder getAlwaysEjectOneHostOrBuilder() {
if (alwaysEjectOneHostBuilder_ != null) {
return alwaysEjectOneHostBuilder_.getMessageOrBuilder();
} else {
return alwaysEjectOneHost_ == null ?
com.google.protobuf.BoolValue.getDefaultInstance() : alwaysEjectOneHost_;
}
}
/**
*
* If enabled, at least one host is ejected regardless of the value of :ref:`max_ejection_percent<envoy_v3_api_field_config.cluster.v3.OutlierDetection.max_ejection_percent>`.
* Defaults to false.
*
*
* .google.protobuf.BoolValue always_eject_one_host = 25;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder>
getAlwaysEjectOneHostFieldBuilder() {
if (alwaysEjectOneHostBuilder_ == null) {
alwaysEjectOneHostBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder>(
getAlwaysEjectOneHost(),
getParentForChildren(),
isClean());
alwaysEjectOneHost_ = null;
}
return alwaysEjectOneHostBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:envoy.config.cluster.v3.OutlierDetection)
}
// @@protoc_insertion_point(class_scope:envoy.config.cluster.v3.OutlierDetection)
private static final io.envoyproxy.envoy.config.cluster.v3.OutlierDetection DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.envoyproxy.envoy.config.cluster.v3.OutlierDetection();
}
public static io.envoyproxy.envoy.config.cluster.v3.OutlierDetection getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public OutlierDetection parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.envoyproxy.envoy.config.cluster.v3.OutlierDetection getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy