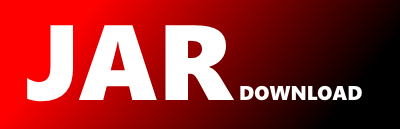
io.gsonfire.util.reflection.CachedReflectionFactory Maven / Gradle / Ivy
package io.gsonfire.util.reflection;
import java.lang.ref.SoftReference;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
/**
* Created by julio on 10/1/16.
*/
public class CachedReflectionFactory implements Factory {
private final ConcurrentMap> cache = new ConcurrentHashMap>();
@Override
public T get(Class clazz) {
SoftReference resultRef = (SoftReference) cache.get(clazz);
if(resultRef != null) {
T result = resultRef.get();
if (result != null) {
return result;
}
}
//We need to create the object
try {
T newObject = clazz.newInstance();
cache.putIfAbsent(clazz, new SoftReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy