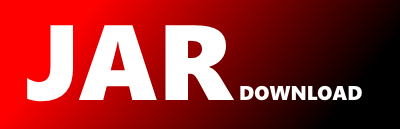
io.harness.cf.client.api.XmlFileMapStore Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ff-java-server-sdk Show documentation
Show all versions of ff-java-server-sdk Show documentation
Harness Feature Flag Java Server SDK
The newest version!
package io.harness.cf.client.api;
import io.harness.cf.client.common.Storage;
import io.harness.cf.client.logger.LogUtil;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.UnsupportedEncodingException;
import java.nio.file.Files;
import java.nio.file.NoSuchFileException;
import java.nio.file.Paths;
import java.util.*;
import lombok.NonNull;
import lombok.extern.slf4j.Slf4j;
@Slf4j
public class XmlFileMapStore implements Storage, AutoCloseable {
private final Properties map = new Properties();
private final String filename;
static {
LogUtil.setSystemProps();
}
public XmlFileMapStore(@NonNull String filename) {
this.filename = filename;
try (InputStream is = Files.newInputStream(Paths.get(filename))) {
map.loadFromXML(is);
log.info("FileMapStore initialized");
} catch (NoSuchFileException | InvalidPropertiesFormatException ignored) {
} catch (UnsupportedEncodingException ex) {
// As of 1.3.x we no longer support MapDB to reduce SDK size. If you still require it you can
// provide your own implementation via the Storage interface
throw new RuntimeException("Unsupported file format", ex);
} catch (Exception ex) {
throw new RuntimeException(ex);
}
}
@Override
public void set(@NonNull String key, @NonNull Object value) {
try {
map.put(key, value);
log.debug("FileMapStore stored successfully key {} with value {}", key, value);
} catch (UnsupportedOperationException
| ClassCastException
| NullPointerException
| IllegalArgumentException e) {
log.error(
"Exception was raised when storing the key {} with the error message {}",
key,
e.getMessage());
}
}
@Override
public Object get(@NonNull String key) {
try {
Object value = map.get(key);
log.debug("Key {} in store found with value {}", key, value);
return value;
} catch (ClassCastException | NullPointerException e) {
log.error(
"Exception was raised while getting the key {} with the error message {}",
key,
e.getMessage());
return null;
}
}
@Override
public void delete(@NonNull String key) {
try {
map.remove(key);
log.debug("FileMapStore successfully delete key {}", key);
} catch (UnsupportedOperationException | ClassCastException | NullPointerException e) {
log.error(
"Exception was raised while deleting the key {} with the error message {}",
key,
e.getMessage());
}
}
@Override
public List keys() {
final List keys = new ArrayList<>();
map.keySet().forEach(obj -> keys.add(obj.toString()));
return keys;
}
@Override
public void close() {
try (OutputStream os = Files.newOutputStream(Paths.get(filename))) {
map.storeToXML(
os, "Generated by " + XmlFileMapStore.class.getSimpleName() + " on " + new Date());
log.debug("FileMapStore closed");
} catch (IOException ex) {
throw new RuntimeException(ex);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy