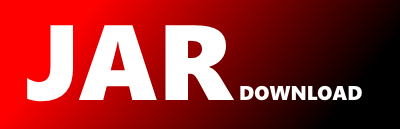
io.harness.cf.model.Segment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ff-java-server-sdk Show documentation
Show all versions of ff-java-server-sdk Show documentation
Harness Feature Flag Java Server SDK
The newest version!
/*
* Harness feature flag service client apis
* No description provided (generated by Openapi Generator https://github.com/openapitools/openapi-generator)
*
* The version of the OpenAPI document: 1.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package io.harness.cf.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.harness.cf.model.Clause;
import io.harness.cf.model.GroupServingRule;
import io.harness.cf.model.Tag;
import io.harness.cf.model.Target;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.io.Serializable;
import lombok.Builder;
import lombok.NoArgsConstructor;
import lombok.AllArgsConstructor;
/**
* A Target Group (Segment) response
*/
@ApiModel(description = "A Target Group (Segment) response")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T14:30:44.890314Z[GMT]")
@Builder
@NoArgsConstructor
@AllArgsConstructor
public class Segment implements Serializable {
private static final long serialVersionUID = 1L;
public static final String SERIALIZED_NAME_IDENTIFIER = "identifier";
@SerializedName(SERIALIZED_NAME_IDENTIFIER)
private String identifier;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_ENVIRONMENT = "environment";
@SerializedName(SERIALIZED_NAME_ENVIRONMENT)
private String environment;
public static final String SERIALIZED_NAME_TAGS = "tags";
@SerializedName(SERIALIZED_NAME_TAGS)
private List tags = null;
public static final String SERIALIZED_NAME_INCLUDED = "included";
@SerializedName(SERIALIZED_NAME_INCLUDED)
private List included = null;
public static final String SERIALIZED_NAME_EXCLUDED = "excluded";
@SerializedName(SERIALIZED_NAME_EXCLUDED)
private List excluded = null;
public static final String SERIALIZED_NAME_RULES = "rules";
@SerializedName(SERIALIZED_NAME_RULES)
private List rules = null;
public static final String SERIALIZED_NAME_SERVING_RULES = "servingRules";
@SerializedName(SERIALIZED_NAME_SERVING_RULES)
private List servingRules = null;
public static final String SERIALIZED_NAME_CREATED_AT = "createdAt";
@SerializedName(SERIALIZED_NAME_CREATED_AT)
private Long createdAt;
public static final String SERIALIZED_NAME_MODIFIED_AT = "modifiedAt";
@SerializedName(SERIALIZED_NAME_MODIFIED_AT)
private Long modifiedAt;
public static final String SERIALIZED_NAME_VERSION = "version";
@SerializedName(SERIALIZED_NAME_VERSION)
private Long version;
public Segment identifier(String identifier) {
this.identifier = identifier;
return this;
}
/**
* Unique identifier for the target group.
* @return identifier
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Unique identifier for the target group.")
public String getIdentifier() {
return identifier;
}
public void setIdentifier(String identifier) {
this.identifier = identifier;
}
public Segment name(String name) {
this.name = name;
return this;
}
/**
* Name of the target group.
* @return name
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "Beta Testers", required = true, value = "Name of the target group.")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Segment environment(String environment) {
this.environment = environment;
return this;
}
/**
* The environment this target group belongs to
* @return environment
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Production", value = "The environment this target group belongs to")
public String getEnvironment() {
return environment;
}
public void setEnvironment(String environment) {
this.environment = environment;
}
public Segment tags(List tags) {
this.tags = tags;
return this;
}
/**
* Tags for this target group
* @return tags
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Tags for this target group")
public List getTags() {
return tags;
}
public void setTags(List tags) {
this.tags = tags;
}
public Segment included(List included) {
this.included = included;
return this;
}
/**
* A list of Targets who belong to this target group
* @return included
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of Targets who belong to this target group")
public List getIncluded() {
return included;
}
public void setIncluded(List included) {
this.included = included;
}
public Segment excluded(List excluded) {
this.excluded = excluded;
return this;
}
/**
* A list of Targets who are excluded from this target group
* @return excluded
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of Targets who are excluded from this target group")
public List getExcluded() {
return excluded;
}
public void setExcluded(List excluded) {
this.excluded = excluded;
}
public Segment rules(List rules) {
this.rules = rules;
return this;
}
/**
* Get rules
* @return rules
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public List getRules() {
return rules;
}
public void setRules(List rules) {
this.rules = rules;
}
public Segment servingRules(List servingRules) {
this.servingRules = servingRules;
return this;
}
/**
* An array of rules that can cause a user to be included in this segment.
* @return servingRules
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "An array of rules that can cause a user to be included in this segment.")
public List getServingRules() {
return servingRules;
}
public void setServingRules(List servingRules) {
this.servingRules = servingRules;
}
public Segment createdAt(Long createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* The data and time in milliseconds when the group was created
* @return createdAt
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The data and time in milliseconds when the group was created")
public Long getCreatedAt() {
return createdAt;
}
public void setCreatedAt(Long createdAt) {
this.createdAt = createdAt;
}
public Segment modifiedAt(Long modifiedAt) {
this.modifiedAt = modifiedAt;
return this;
}
/**
* The data and time in milliseconds when the group was last modified
* @return modifiedAt
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The data and time in milliseconds when the group was last modified")
public Long getModifiedAt() {
return modifiedAt;
}
public void setModifiedAt(Long modifiedAt) {
this.modifiedAt = modifiedAt;
}
public Segment version(Long version) {
this.version = version;
return this;
}
/**
* The version of this group. Each time it is modified the version is incremented
* @return version
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "1", value = "The version of this group. Each time it is modified the version is incremented")
public Long getVersion() {
return version;
}
public void setVersion(Long version) {
this.version = version;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Segment segment = (Segment) o;
return Objects.equals(this.identifier, segment.identifier) &&
Objects.equals(this.name, segment.name) &&
Objects.equals(this.environment, segment.environment) &&
Objects.equals(this.tags, segment.tags) &&
Objects.equals(this.included, segment.included) &&
Objects.equals(this.excluded, segment.excluded) &&
Objects.equals(this.rules, segment.rules) &&
Objects.equals(this.servingRules, segment.servingRules) &&
Objects.equals(this.createdAt, segment.createdAt) &&
Objects.equals(this.modifiedAt, segment.modifiedAt) &&
Objects.equals(this.version, segment.version);
}
@Override
public int hashCode() {
return Objects.hash(identifier, name, environment, tags, included, excluded, rules, servingRules, createdAt, modifiedAt, version);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Segment {\n");
sb.append(" identifier: ").append(toIndentedString(identifier)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" environment: ").append(toIndentedString(environment)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" included: ").append(toIndentedString(included)).append("\n");
sb.append(" excluded: ").append(toIndentedString(excluded)).append("\n");
sb.append(" rules: ").append(toIndentedString(rules)).append("\n");
sb.append(" servingRules: ").append(toIndentedString(servingRules)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" modifiedAt: ").append(toIndentedString(modifiedAt)).append("\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy