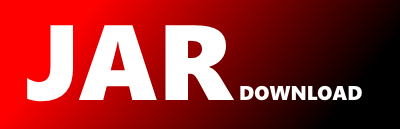
io.harness.cf.model.Target Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ff-java-server-sdk Show documentation
Show all versions of ff-java-server-sdk Show documentation
Harness Feature Flag Java Server SDK
The newest version!
/*
* Harness feature flag service client apis
* No description provided (generated by Openapi Generator https://github.com/openapitools/openapi-generator)
*
* The version of the OpenAPI document: 1.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package io.harness.cf.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.harness.cf.model.Segment;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.io.Serializable;
import lombok.Builder;
import lombok.NoArgsConstructor;
import lombok.AllArgsConstructor;
/**
* A Target object
*/
@ApiModel(description = "A Target object")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T14:30:44.890314Z[GMT]")
@Builder
@NoArgsConstructor
@AllArgsConstructor
public class Target implements Serializable {
private static final long serialVersionUID = 1L;
public static final String SERIALIZED_NAME_IDENTIFIER = "identifier";
@SerializedName(SERIALIZED_NAME_IDENTIFIER)
private String identifier;
public static final String SERIALIZED_NAME_ACCOUNT = "account";
@SerializedName(SERIALIZED_NAME_ACCOUNT)
private String account;
public static final String SERIALIZED_NAME_ORG = "org";
@SerializedName(SERIALIZED_NAME_ORG)
private String org;
public static final String SERIALIZED_NAME_ENVIRONMENT = "environment";
@SerializedName(SERIALIZED_NAME_ENVIRONMENT)
private String environment;
public static final String SERIALIZED_NAME_PROJECT = "project";
@SerializedName(SERIALIZED_NAME_PROJECT)
private String project;
public static final String SERIALIZED_NAME_NAME = "name";
@SerializedName(SERIALIZED_NAME_NAME)
private String name;
public static final String SERIALIZED_NAME_ANONYMOUS = "anonymous";
@SerializedName(SERIALIZED_NAME_ANONYMOUS)
private Boolean anonymous;
public static final String SERIALIZED_NAME_ATTRIBUTES = "attributes";
@SerializedName(SERIALIZED_NAME_ATTRIBUTES)
private Object attributes;
public static final String SERIALIZED_NAME_CREATED_AT = "createdAt";
@SerializedName(SERIALIZED_NAME_CREATED_AT)
private Long createdAt;
public static final String SERIALIZED_NAME_SEGMENTS = "segments";
@SerializedName(SERIALIZED_NAME_SEGMENTS)
private List segments = null;
public Target identifier(String identifier) {
this.identifier = identifier;
return this;
}
/**
* The unique identifier for this target
* @return identifier
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "john-doe", required = true, value = "The unique identifier for this target")
public String getIdentifier() {
return identifier;
}
public void setIdentifier(String identifier) {
this.identifier = identifier;
}
public Target account(String account) {
this.account = account;
return this;
}
/**
* The account ID that the target belongs to
* @return account
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "abcXDdffdaffd", required = true, value = "The account ID that the target belongs to")
public String getAccount() {
return account;
}
public void setAccount(String account) {
this.account = account;
}
public Target org(String org) {
this.org = org;
return this;
}
/**
* The identifier for the organization that the target belongs to
* @return org
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The identifier for the organization that the target belongs to")
public String getOrg() {
return org;
}
public void setOrg(String org) {
this.org = org;
}
public Target environment(String environment) {
this.environment = environment;
return this;
}
/**
* The identifier for the environment that the target belongs to
* @return environment
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The identifier for the environment that the target belongs to")
public String getEnvironment() {
return environment;
}
public void setEnvironment(String environment) {
this.environment = environment;
}
public Target project(String project) {
this.project = project;
return this;
}
/**
* The identifier for the project that this target belongs to
* @return project
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "The identifier for the project that this target belongs to")
public String getProject() {
return project;
}
public void setProject(String project) {
this.project = project;
}
public Target name(String name) {
this.name = name;
return this;
}
/**
* The name of this Target
* @return name
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "John Doe", required = true, value = "The name of this Target")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Target anonymous(Boolean anonymous) {
this.anonymous = anonymous;
return this;
}
/**
* Indicates if this target is anonymous
* @return anonymous
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Indicates if this target is anonymous")
public Boolean getAnonymous() {
return anonymous;
}
public void setAnonymous(Boolean anonymous) {
this.anonymous = anonymous;
}
public Target attributes(Object attributes) {
this.attributes = attributes;
return this;
}
/**
* a JSON representation of the attributes for this target
* @return attributes
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "{\"age\":20,\"location\":\"Belfast\"}", value = "a JSON representation of the attributes for this target")
public Object getAttributes() {
return attributes;
}
public void setAttributes(Object attributes) {
this.attributes = attributes;
}
public Target createdAt(Long createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* The date and time in milliseconds when this Target was created
* @return createdAt
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The date and time in milliseconds when this Target was created")
public Long getCreatedAt() {
return createdAt;
}
public void setCreatedAt(Long createdAt) {
this.createdAt = createdAt;
}
public Target segments(List segments) {
this.segments = segments;
return this;
}
/**
* A list of Target Groups (Segments) that this Target belongs to
* @return segments
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of Target Groups (Segments) that this Target belongs to")
public List getSegments() {
return segments;
}
public void setSegments(List segments) {
this.segments = segments;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Target target = (Target) o;
return Objects.equals(this.identifier, target.identifier) &&
Objects.equals(this.account, target.account) &&
Objects.equals(this.org, target.org) &&
Objects.equals(this.environment, target.environment) &&
Objects.equals(this.project, target.project) &&
Objects.equals(this.name, target.name) &&
Objects.equals(this.anonymous, target.anonymous) &&
Objects.equals(this.attributes, target.attributes) &&
Objects.equals(this.createdAt, target.createdAt) &&
Objects.equals(this.segments, target.segments);
}
@Override
public int hashCode() {
return Objects.hash(identifier, account, org, environment, project, name, anonymous, attributes, createdAt, segments);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Target {\n");
sb.append(" identifier: ").append(toIndentedString(identifier)).append("\n");
sb.append(" account: ").append(toIndentedString(account)).append("\n");
sb.append(" org: ").append(toIndentedString(org)).append("\n");
sb.append(" environment: ").append(toIndentedString(environment)).append("\n");
sb.append(" project: ").append(toIndentedString(project)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" anonymous: ").append(toIndentedString(anonymous)).append("\n");
sb.append(" attributes: ").append(toIndentedString(attributes)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" segments: ").append(toIndentedString(segments)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy