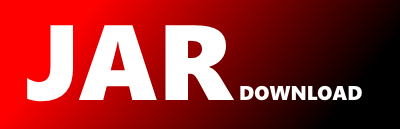
io.harness.cf.model.VariationMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ff-java-server-sdk Show documentation
Show all versions of ff-java-server-sdk Show documentation
Harness Feature Flag Java Server SDK
The newest version!
/*
* Harness feature flag service client apis
* No description provided (generated by Openapi Generator https://github.com/openapitools/openapi-generator)
*
* The version of the OpenAPI document: 1.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package io.harness.cf.model;
import java.util.Objects;
import java.util.Arrays;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import io.harness.cf.model.TargetMap;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.io.Serializable;
import lombok.Builder;
import lombok.NoArgsConstructor;
import lombok.AllArgsConstructor;
/**
* A mapping of variations to targets and target groups (segments). The targets listed here should receive this variation.
*/
@ApiModel(description = "A mapping of variations to targets and target groups (segments). The targets listed here should receive this variation.")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-10-04T14:30:44.890314Z[GMT]")
@Builder
@NoArgsConstructor
@AllArgsConstructor
public class VariationMap implements Serializable {
private static final long serialVersionUID = 1L;
public static final String SERIALIZED_NAME_VARIATION = "variation";
@SerializedName(SERIALIZED_NAME_VARIATION)
private String variation;
public static final String SERIALIZED_NAME_TARGETS = "targets";
@SerializedName(SERIALIZED_NAME_TARGETS)
private List targets = null;
public static final String SERIALIZED_NAME_TARGET_SEGMENTS = "targetSegments";
@SerializedName(SERIALIZED_NAME_TARGET_SEGMENTS)
private List targetSegments = null;
public VariationMap variation(String variation) {
this.variation = variation;
return this;
}
/**
* The variation identifier
* @return variation
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "off-variation", required = true, value = "The variation identifier")
public String getVariation() {
return variation;
}
public void setVariation(String variation) {
this.variation = variation;
}
public VariationMap targets(List targets) {
this.targets = targets;
return this;
}
/**
* A list of target mappings
* @return targets
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of target mappings")
public List getTargets() {
return targets;
}
public void setTargets(List targets) {
this.targets = targets;
}
public VariationMap targetSegments(List targetSegments) {
this.targetSegments = targetSegments;
return this;
}
/**
* A list of target groups (segments)
* @return targetSegments
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "A list of target groups (segments)")
public List getTargetSegments() {
return targetSegments;
}
public void setTargetSegments(List targetSegments) {
this.targetSegments = targetSegments;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
VariationMap variationMap = (VariationMap) o;
return Objects.equals(this.variation, variationMap.variation) &&
Objects.equals(this.targets, variationMap.targets) &&
Objects.equals(this.targetSegments, variationMap.targetSegments);
}
@Override
public int hashCode() {
return Objects.hash(variation, targets, targetSegments);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class VariationMap {\n");
sb.append(" variation: ").append(toIndentedString(variation)).append("\n");
sb.append(" targets: ").append(toIndentedString(targets)).append("\n");
sb.append(" targetSegments: ").append(toIndentedString(targetSegments)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy