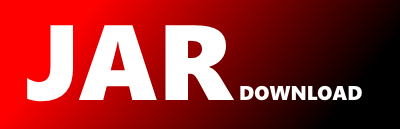
io.helidon.codegen.compiler.CompilerOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of helidon-codegen-compiler Show documentation
Show all versions of helidon-codegen-compiler Show documentation
Tools for compilation of Java
/*
* Copyright (c) 2024 Oracle and/or its affiliates.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.helidon.codegen.compiler;
import java.nio.file.Path;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import io.helidon.builder.api.Prototype;
import io.helidon.codegen.CodegenLogger;
import io.helidon.common.Errors;
import io.helidon.common.Generated;
/**
* Provides configuration to the javac compiler.
*
* @see #builder()
* @see #create()
*/
@Generated(value = "io.helidon.builder.codegen.BuilderCodegen", trigger = "io.helidon.codegen.compiler.CompilerOptionsBlueprint")
public interface CompilerOptions extends CompilerOptionsBlueprint, Prototype.Api {
/**
* Create a new fluent API builder to customize configuration.
*
* @return a new builder
*/
static CompilerOptions.Builder builder() {
return new CompilerOptions.Builder();
}
/**
* Create a new fluent API builder from an existing instance.
*
* @param instance an existing instance used as a base for the builder
* @return a builder based on an instance
*/
static CompilerOptions.Builder builder(CompilerOptions instance) {
return CompilerOptions.builder().from(instance);
}
/**
* Create a new instance with default values.
*
* @return a new instance
*/
static CompilerOptions create() {
return CompilerOptions.builder().buildPrototype();
}
/**
* Fluent API builder base for {@link CompilerOptions}.
*
* @param type of the builder extending this abstract builder
* @param type of the prototype interface that would be built by {@link #buildPrototype()}
*/
abstract class BuilderBase, PROTOTYPE extends CompilerOptions> implements Prototype.Builder {
private final List commandLineArguments = new ArrayList<>();
private final List classpath = new ArrayList<>();
private final List modulepath = new ArrayList<>();
private final List sourcepath = new ArrayList<>();
private boolean isClasspathMutated;
private boolean isCommandLineArgumentsMutated;
private boolean isModulepathMutated;
private boolean isSourcepathMutated;
private CodegenLogger logger = CodegenLogger.create(System.getLogger("io.helidon.codegen.compiler.Compiler"));
private Path outputDirectory;
private String source = "21";
private String target = "21";
/**
* Protected to support extensibility.
*/
protected BuilderBase() {
}
/**
* Update this builder from an existing prototype instance. This method disables automatic service discovery.
*
* @param prototype existing prototype to update this builder from
* @return updated builder instance
*/
public BUILDER from(CompilerOptions prototype) {
if (!isClasspathMutated) {
classpath.clear();
}
addClasspath(prototype.classpath());
if (!isModulepathMutated) {
modulepath.clear();
}
addModulepath(prototype.modulepath());
if (!isSourcepathMutated) {
sourcepath.clear();
}
addSourcepath(prototype.sourcepath());
if (!isCommandLineArgumentsMutated) {
commandLineArguments.clear();
}
addCommandLineArguments(prototype.commandLineArguments());
source(prototype.source());
target(prototype.target());
outputDirectory(prototype.outputDirectory());
logger(prototype.logger());
return self();
}
/**
* Update this builder from an existing prototype builder instance.
*
* @param builder existing builder prototype to update this builder from
* @return updated builder instance
*/
public BUILDER from(CompilerOptions.BuilderBase, ?> builder) {
if (isClasspathMutated) {
if (builder.isClasspathMutated) {
addClasspath(builder.classpath);
}
} else {
classpath.clear();
addClasspath(builder.classpath);
}
if (isModulepathMutated) {
if (builder.isModulepathMutated) {
addModulepath(builder.modulepath);
}
} else {
modulepath.clear();
addModulepath(builder.modulepath);
}
if (isSourcepathMutated) {
if (builder.isSourcepathMutated) {
addSourcepath(builder.sourcepath);
}
} else {
sourcepath.clear();
addSourcepath(builder.sourcepath);
}
if (isCommandLineArgumentsMutated) {
if (builder.isCommandLineArgumentsMutated) {
addCommandLineArguments(builder.commandLineArguments);
}
} else {
commandLineArguments.clear();
addCommandLineArguments(builder.commandLineArguments);
}
source(builder.source());
target(builder.target());
builder.outputDirectory().ifPresent(this::outputDirectory);
logger(builder.logger());
return self();
}
/**
* The classpath to pass to the compiler.
*
* @param classpath classpath
* @return updated builder instance
* @see #classpath()
*/
public BUILDER classpath(List extends Path> classpath) {
Objects.requireNonNull(classpath);
isClasspathMutated = true;
this.classpath.clear();
this.classpath.addAll(classpath);
return self();
}
/**
* The classpath to pass to the compiler.
*
* @param classpath classpath
* @return updated builder instance
* @see #classpath()
*/
public BUILDER addClasspath(List extends Path> classpath) {
Objects.requireNonNull(classpath);
isClasspathMutated = true;
this.classpath.addAll(classpath);
return self();
}
/**
* The classpath to pass to the compiler.
*
* @param classpath classpath
* @return updated builder instance
* @see #classpath()
*/
public BUILDER addClasspath(Path classpath) {
Objects.requireNonNull(classpath);
this.classpath.add(classpath);
isClasspathMutated = true;
return self();
}
/**
* The modulepath to pass to the compiler.
*
* @param modulepath the module path
* @return updated builder instance
* @see #modulepath()
*/
public BUILDER modulepath(List extends Path> modulepath) {
Objects.requireNonNull(modulepath);
isModulepathMutated = true;
this.modulepath.clear();
this.modulepath.addAll(modulepath);
return self();
}
/**
* The modulepath to pass to the compiler.
*
* @param modulepath the module path
* @return updated builder instance
* @see #modulepath()
*/
public BUILDER addModulepath(List extends Path> modulepath) {
Objects.requireNonNull(modulepath);
isModulepathMutated = true;
this.modulepath.addAll(modulepath);
return self();
}
/**
* The modulepath to pass to the compiler.
*
* @param modulepath the module path
* @return updated builder instance
* @see #modulepath()
*/
public BUILDER addModulepath(Path modulepath) {
Objects.requireNonNull(modulepath);
this.modulepath.add(modulepath);
isModulepathMutated = true;
return self();
}
/**
* The source path to pass to the compiler.
*
* @param sourcepath the source path
* @return updated builder instance
* @see #sourcepath()
*/
public BUILDER sourcepath(List extends Path> sourcepath) {
Objects.requireNonNull(sourcepath);
isSourcepathMutated = true;
this.sourcepath.clear();
this.sourcepath.addAll(sourcepath);
return self();
}
/**
* The source path to pass to the compiler.
*
* @param sourcepath the source path
* @return updated builder instance
* @see #sourcepath()
*/
public BUILDER addSourcepath(List extends Path> sourcepath) {
Objects.requireNonNull(sourcepath);
isSourcepathMutated = true;
this.sourcepath.addAll(sourcepath);
return self();
}
/**
* The source path to pass to the compiler.
*
* @param sourcepath the source path
* @return updated builder instance
* @see #sourcepath()
*/
public BUILDER addSourcepath(Path sourcepath) {
Objects.requireNonNull(sourcepath);
this.sourcepath.add(sourcepath);
isSourcepathMutated = true;
return self();
}
/**
* The command line arguments to pass to the compiler.
*
* @param commandLineArguments arguments
* @return updated builder instance
* @see #commandLineArguments()
*/
public BUILDER commandLineArguments(List extends String> commandLineArguments) {
Objects.requireNonNull(commandLineArguments);
isCommandLineArgumentsMutated = true;
this.commandLineArguments.clear();
this.commandLineArguments.addAll(commandLineArguments);
return self();
}
/**
* The command line arguments to pass to the compiler.
*
* @param commandLineArguments arguments
* @return updated builder instance
* @see #commandLineArguments()
*/
public BUILDER addCommandLineArguments(List extends String> commandLineArguments) {
Objects.requireNonNull(commandLineArguments);
isCommandLineArgumentsMutated = true;
this.commandLineArguments.addAll(commandLineArguments);
return self();
}
/**
* The command line arguments to pass to the compiler.
*
* @param commandLineArgument arguments
* @return updated builder instance
* @see #commandLineArguments()
*/
public BUILDER addCommandLineArgument(String commandLineArgument) {
Objects.requireNonNull(commandLineArgument);
this.commandLineArguments.add(commandLineArgument);
isCommandLineArgumentsMutated = true;
return self();
}
/**
* The compiler source version.
*
* @param source source version
* @return updated builder instance
* @see #source()
*/
public BUILDER source(String source) {
Objects.requireNonNull(source);
this.source = source;
return self();
}
/**
* The compiler target version.
*
* @param target target version
* @return updated builder instance
* @see #target()
*/
public BUILDER target(String target) {
Objects.requireNonNull(target);
this.target = target;
return self();
}
/**
* Target directory to generate class files to.
*
* @param outputDirectory output directory
* @return updated builder instance
* @see #outputDirectory()
*/
public BUILDER outputDirectory(Path outputDirectory) {
Objects.requireNonNull(outputDirectory);
this.outputDirectory = outputDirectory;
return self();
}
/**
* Logger to use, falls back to system logger.
*
* @param logger logger
* @return updated builder instance
* @see #logger()
*/
public BUILDER logger(CodegenLogger logger) {
Objects.requireNonNull(logger);
this.logger = logger;
return self();
}
/**
* The classpath to pass to the compiler.
*
* @return the classpath
*/
public List classpath() {
return classpath;
}
/**
* The modulepath to pass to the compiler.
*
* @return the modulepath
*/
public List modulepath() {
return modulepath;
}
/**
* The source path to pass to the compiler.
*
* @return the sourcepath
*/
public List sourcepath() {
return sourcepath;
}
/**
* The command line arguments to pass to the compiler.
*
* @return the command line arguments
*/
public List commandLineArguments() {
return commandLineArguments;
}
/**
* The compiler source version.
*
* @return the source
*/
public String source() {
return source;
}
/**
* The compiler target version.
*
* @return the target
*/
public String target() {
return target;
}
/**
* Target directory to generate class files to.
*
* @return the output directory
*/
public Optional outputDirectory() {
return Optional.ofNullable(outputDirectory);
}
/**
* Logger to use, falls back to system logger.
*
* @return the logger
*/
public CodegenLogger logger() {
return logger;
}
@Override
public String toString() {
return "CompilerOptionsBuilder{"
+ "classpath=" + classpath + ","
+ "modulepath=" + modulepath + ","
+ "sourcepath=" + sourcepath + ","
+ "commandLineArguments=" + commandLineArguments + ","
+ "source=" + source + ","
+ "target=" + target + ","
+ "outputDirectory=" + outputDirectory + ","
+ "logger=" + logger
+ "}";
}
/**
* Handles providers and decorators.
*/
protected void preBuildPrototype() {
}
/**
* Validates required properties.
*/
protected void validatePrototype() {
Errors.Collector collector = Errors.collector();
if (outputDirectory == null) {
collector.fatal(getClass(), "Property \"outputDirectory\" must not be null, but not set");
}
collector.collect().checkValid();
}
/**
* Generated implementation of the prototype, can be extended by descendant prototype implementations.
*/
protected static class CompilerOptionsImpl implements CompilerOptions {
private final CodegenLogger logger;
private final List commandLineArguments;
private final List classpath;
private final List modulepath;
private final List sourcepath;
private final Path outputDirectory;
private final String source;
private final String target;
/**
* Create an instance providing a builder.
*
* @param builder extending builder base of this prototype
*/
protected CompilerOptionsImpl(CompilerOptions.BuilderBase, ?> builder) {
this.classpath = List.copyOf(builder.classpath());
this.modulepath = List.copyOf(builder.modulepath());
this.sourcepath = List.copyOf(builder.sourcepath());
this.commandLineArguments = List.copyOf(builder.commandLineArguments());
this.source = builder.source();
this.target = builder.target();
this.outputDirectory = builder.outputDirectory().get();
this.logger = builder.logger();
}
@Override
public List classpath() {
return classpath;
}
@Override
public List modulepath() {
return modulepath;
}
@Override
public List sourcepath() {
return sourcepath;
}
@Override
public List commandLineArguments() {
return commandLineArguments;
}
@Override
public String source() {
return source;
}
@Override
public String target() {
return target;
}
@Override
public Path outputDirectory() {
return outputDirectory;
}
@Override
public CodegenLogger logger() {
return logger;
}
@Override
public String toString() {
return "CompilerOptions{"
+ "classpath=" + classpath + ","
+ "modulepath=" + modulepath + ","
+ "sourcepath=" + sourcepath + ","
+ "commandLineArguments=" + commandLineArguments + ","
+ "source=" + source + ","
+ "target=" + target + ","
+ "outputDirectory=" + outputDirectory + ","
+ "logger=" + logger
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (!(o instanceof CompilerOptions other)) {
return false;
}
return Objects.equals(classpath, other.classpath())
&& Objects.equals(modulepath, other.modulepath())
&& Objects.equals(sourcepath, other.sourcepath())
&& Objects.equals(commandLineArguments, other.commandLineArguments())
&& Objects.equals(source, other.source())
&& Objects.equals(target, other.target())
&& Objects.equals(outputDirectory, other.outputDirectory())
&& Objects.equals(logger, other.logger());
}
@Override
public int hashCode() {
return Objects.hash(classpath, modulepath, sourcepath, commandLineArguments, source, target, outputDirectory, logger);
}
}
}
/**
* Fluent API builder for {@link CompilerOptions}.
*/
class Builder extends CompilerOptions.BuilderBase implements io.helidon.common.Builder {
private Builder() {
}
@Override
public CompilerOptions buildPrototype() {
preBuildPrototype();
validatePrototype();
return new CompilerOptionsImpl(this);
}
@Override
public CompilerOptions build() {
return buildPrototype();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy