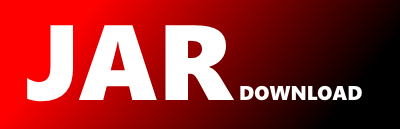
io.helidon.codegen.Codegen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of helidon-codegen Show documentation
Show all versions of helidon-codegen Show documentation
Code generation common utilities
The newest version!
/*
* Copyright (c) 2023, 2024 Oracle and/or its affiliates.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.helidon.codegen;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.IdentityHashMap;
import java.util.List;
import java.util.Map;
import java.util.ServiceLoader;
import java.util.Set;
import java.util.function.Predicate;
import java.util.stream.Collectors;
import io.helidon.codegen.classmodel.ClassModel;
import io.helidon.codegen.spi.CodegenExtension;
import io.helidon.codegen.spi.CodegenExtensionProvider;
import io.helidon.common.HelidonServiceLoader;
import io.helidon.common.types.TypeInfo;
import io.helidon.common.types.TypeName;
/**
* Central piece of code processing and generation.
* This type loads {@link io.helidon.codegen.spi.CodegenExtensionProvider extension providers}, and invokes
* each {@link io.helidon.codegen.spi.CodegenExtension} with appropriate types and annotations.
*/
public class Codegen {
private static final List EXTENSIONS =
HelidonServiceLoader.create(ServiceLoader.load(CodegenExtensionProvider.class,
Codegen.class.getClassLoader()))
.asList();
private static final Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy