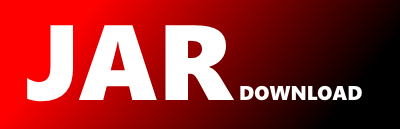
io.helidon.common.http.HashParameters Maven / Gradle / Ivy
/*
* Copyright (c) 2017, 2021 Oracle and/or its affiliates.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.helidon.common.http;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.TreeMap;
import java.util.concurrent.ConcurrentMap;
import java.util.concurrent.ConcurrentSkipListMap;
import java.util.function.Function;
/**
* A {@link ConcurrentSkipListMap} based {@link Parameters} implementation with
* case-insensitive keys and immutable {@link List} of values that needs to be copied on each write.
*/
public class HashParameters implements Parameters {
private static final List EMPTY_STRING_LIST = Collections.emptyList();
private final ConcurrentMap> content;
/**
* Creates a new instance.
*/
protected HashParameters() {
this((Parameters) null);
}
/**
* Creates a new instance from provided data.
* Initial data are copied.
*
* @param initialContent initial content.
*/
protected HashParameters(Map> initialContent) {
if (initialContent == null) {
content = new ConcurrentSkipListMap<>(String.CASE_INSENSITIVE_ORDER);
} else {
content = new ConcurrentSkipListMap<>(String.CASE_INSENSITIVE_ORDER);
for (Map.Entry> entry : initialContent.entrySet()) {
content.compute(
entry.getKey(),
(key, values) -> {
if (values == null) {
return Collections.unmodifiableList(new ArrayList<>(entry.getValue()));
} else {
values.addAll(entry.getValue());
return values;
}
}
);
}
}
}
/**
* Creates a new instance from provided data.
* Initial data is copied.
*
* @param initialContent initial content.
*/
protected HashParameters(Parameters initialContent) {
this(initialContent == null ? null : initialContent.toMap());
}
/**
* Creates a new empty instance {@link HashParameters}.
*
* @return a new instance of {@link HashParameters}.
*/
public static HashParameters create() {
return new HashParameters();
}
/**
* Creates a new instance {@link HashParameters} from provided data. Initial data is copied.
*
* @param initialContent initial content.
* @return a new instance of {@link HashParameters} initialized with the given content.
*/
public static HashParameters create(Map> initialContent) {
return new HashParameters(initialContent);
}
/**
* Creates a new instance {@link HashParameters} from provided data. Initial data is copied.
*
* @param initialContent initial content.
* @return a new instance of {@link HashParameters} initialized with the given content.
*/
public static HashParameters create(Parameters initialContent) {
return new HashParameters(initialContent);
}
/**
* Creates new instance of {@link HashParameters} as a concatenation of provided parameters.
* Values for keys found across the provided parameters are "concatenated" into a {@link List} entry for their respective key
* in the created {@link HashParameters} instance.
*
* @param parameters parameters to concatenate.
* @return a new instance of {@link HashParameters} that represents the concatenation of the provided parameters.
*/
public static HashParameters concat(Parameters... parameters) {
if (parameters == null || parameters.length == 0) {
return new HashParameters();
}
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy