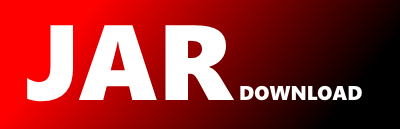
io.helidon.openapi.SnakeYAMLParserHelper Maven / Gradle / Ivy
/*
* Copyright (c) 2020 Oracle and/or its affiliates.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package io.helidon.openapi;
import java.math.BigDecimal;
import java.util.AbstractMap;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.BiFunction;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.smallrye.openapi.api.constants.OpenApiConstants;
import io.smallrye.openapi.api.models.ComponentsImpl;
import io.smallrye.openapi.api.models.ExtensibleImpl;
import io.smallrye.openapi.api.models.ExternalDocumentationImpl;
import io.smallrye.openapi.api.models.MapModel;
import io.smallrye.openapi.api.models.ModelImpl;
import io.smallrye.openapi.api.models.OpenAPIImpl;
import io.smallrye.openapi.api.models.OperationImpl;
import io.smallrye.openapi.api.models.PathItemImpl;
import io.smallrye.openapi.api.models.PathsImpl;
import io.smallrye.openapi.api.models.callbacks.CallbackImpl;
import io.smallrye.openapi.api.models.examples.ExampleImpl;
import io.smallrye.openapi.api.models.headers.HeaderImpl;
import io.smallrye.openapi.api.models.info.ContactImpl;
import io.smallrye.openapi.api.models.info.InfoImpl;
import io.smallrye.openapi.api.models.info.LicenseImpl;
import io.smallrye.openapi.api.models.links.LinkImpl;
import io.smallrye.openapi.api.models.media.ContentImpl;
import io.smallrye.openapi.api.models.media.DiscriminatorImpl;
import io.smallrye.openapi.api.models.media.EncodingImpl;
import io.smallrye.openapi.api.models.media.MediaTypeImpl;
import io.smallrye.openapi.api.models.media.SchemaImpl;
import io.smallrye.openapi.api.models.media.XMLImpl;
import io.smallrye.openapi.api.models.parameters.ParameterImpl;
import io.smallrye.openapi.api.models.parameters.RequestBodyImpl;
import io.smallrye.openapi.api.models.responses.APIResponseImpl;
import io.smallrye.openapi.api.models.responses.APIResponsesImpl;
import io.smallrye.openapi.api.models.security.OAuthFlowImpl;
import io.smallrye.openapi.api.models.security.OAuthFlowsImpl;
import io.smallrye.openapi.api.models.security.ScopesImpl;
import io.smallrye.openapi.api.models.security.SecurityRequirementImpl;
import io.smallrye.openapi.api.models.security.SecuritySchemeImpl;
import io.smallrye.openapi.api.models.servers.ServerImpl;
import io.smallrye.openapi.api.models.servers.ServerVariableImpl;
import io.smallrye.openapi.api.models.servers.ServerVariablesImpl;
import io.smallrye.openapi.api.models.tags.TagImpl;
import io.smallrye.openapi.runtime.util.ModelUtil;
import org.eclipse.microprofile.openapi.models.Components;
import org.eclipse.microprofile.openapi.models.Constructible;
import org.eclipse.microprofile.openapi.models.Extensible;
import org.eclipse.microprofile.openapi.models.ExternalDocumentation;
import org.eclipse.microprofile.openapi.models.OpenAPI;
import org.eclipse.microprofile.openapi.models.Operation;
import org.eclipse.microprofile.openapi.models.PathItem;
import org.eclipse.microprofile.openapi.models.Paths;
import org.eclipse.microprofile.openapi.models.Reference;
import org.eclipse.microprofile.openapi.models.callbacks.Callback;
import org.eclipse.microprofile.openapi.models.examples.Example;
import org.eclipse.microprofile.openapi.models.headers.Header;
import org.eclipse.microprofile.openapi.models.info.Contact;
import org.eclipse.microprofile.openapi.models.info.Info;
import org.eclipse.microprofile.openapi.models.info.License;
import org.eclipse.microprofile.openapi.models.links.Link;
import org.eclipse.microprofile.openapi.models.media.Content;
import org.eclipse.microprofile.openapi.models.media.Discriminator;
import org.eclipse.microprofile.openapi.models.media.Encoding;
import org.eclipse.microprofile.openapi.models.media.MediaType;
import org.eclipse.microprofile.openapi.models.media.Schema;
import org.eclipse.microprofile.openapi.models.media.XML;
import org.eclipse.microprofile.openapi.models.parameters.Parameter;
import org.eclipse.microprofile.openapi.models.parameters.RequestBody;
import org.eclipse.microprofile.openapi.models.responses.APIResponse;
import org.eclipse.microprofile.openapi.models.responses.APIResponses;
import org.eclipse.microprofile.openapi.models.security.OAuthFlow;
import org.eclipse.microprofile.openapi.models.security.OAuthFlows;
import org.eclipse.microprofile.openapi.models.security.Scopes;
import org.eclipse.microprofile.openapi.models.security.SecurityRequirement;
import org.eclipse.microprofile.openapi.models.security.SecurityScheme;
import org.eclipse.microprofile.openapi.models.servers.Server;
import org.eclipse.microprofile.openapi.models.servers.ServerVariable;
import org.eclipse.microprofile.openapi.models.servers.ServerVariables;
import org.eclipse.microprofile.openapi.models.tags.Tag;
import org.yaml.snakeyaml.TypeDescription;
class SnakeYAMLParserHelper {
private final Map, T> types = new HashMap<>();
private final Map, Class>> mapImplementingTypes = new HashMap<>();
private final Map, Class>> listImplementingTypes = new HashMap<>();
static SnakeYAMLParserHelper create(
BiFunction, Class>, T> factoryFunction) {
return new SnakeYAMLParserHelper(factoryFunction);
}
Map, T> types() {
return types;
}
Set, T>> entrySet() {
return types.entrySet();
}
Set> keySet() {
return types.keySet();
}
boolean containsKey(Class> type) {
return types.containsKey(type);
}
T get(Class> clazz) {
return types.get(clazz);
}
private SnakeYAMLParserHelper(BiFunction, Class>, T> factoryFunction) {
T td_Discriminator = factoryFunction.apply(Discriminator.class, DiscriminatorImpl.class);
td_Discriminator.addPropertyParameters("mapping", String.class, String.class);
types.put(td_Discriminator.getType(), td_Discriminator);
T td_Components = factoryFunction.apply(Components.class, ComponentsImpl.class);
td_Components.addPropertyParameters("schemas", String.class, Schema.class);
td_Components.addPropertyParameters("responses", String.class, APIResponse.class);
td_Components.addPropertyParameters("parameters", String.class, Parameter.class);
td_Components.addPropertyParameters("examples", String.class, Example.class);
td_Components.addPropertyParameters("requestBodies", String.class, RequestBody.class);
td_Components.addPropertyParameters("headers", String.class, Header.class);
td_Components.addPropertyParameters("securitySchemes", String.class, SecurityScheme.class);
td_Components.addPropertyParameters("links", String.class, Link.class);
td_Components.addPropertyParameters("callbacks", String.class, Callback.class);
types.put(td_Components.getType(), td_Components);
T td_Info = factoryFunction.apply(Info.class, InfoImpl.class);
types.put(td_Info.getType(), td_Info);
T td_Example = factoryFunction.apply(Example.class, ExampleImpl.class);
types.put(td_Example.getType(), td_Example);
T td_SecurityRequirement = factoryFunction.apply(SecurityRequirement.class, SecurityRequirementImpl.class);
td_SecurityRequirement.addPropertyParameters("schemes", String.class);
types.put(td_SecurityRequirement.getType(), td_SecurityRequirement);
T td_Paths = factoryFunction.apply(Paths.class, PathsImpl.class);
td_Paths.addPropertyParameters("pathItems", String.class, PathItem.class);
types.put(td_Paths.getType(), td_Paths);
T td_APIResponse = factoryFunction.apply(APIResponse.class, APIResponseImpl.class);
td_APIResponse.addPropertyParameters("headers", String.class, Header.class);
td_APIResponse.addPropertyParameters("links", String.class, Link.class);
types.put(td_APIResponse.getType(), td_APIResponse);
T td_OAuthFlow = factoryFunction.apply(OAuthFlow.class, OAuthFlowImpl.class);
td_OAuthFlow.addPropertyParameters("scopes", String.class, String.class);
types.put(td_OAuthFlow.getType(), td_OAuthFlow);
T td_MediaType = factoryFunction.apply(MediaType.class, MediaTypeImpl.class);
td_MediaType.addPropertyParameters("examples", String.class, Example.class);
td_MediaType.addPropertyParameters("encoding", String.class, Encoding.class);
types.put(td_MediaType.getType(), td_MediaType);
T td_License = factoryFunction.apply(License.class, LicenseImpl.class);
types.put(td_License.getType(), td_License);
T td_ServerVariable = factoryFunction.apply(ServerVariable.class, ServerVariableImpl.class);
td_ServerVariable.addPropertyParameters("enumeration", String.class);
types.put(td_ServerVariable.getType(), td_ServerVariable);
T td_Encoding = factoryFunction.apply(Encoding.class, EncodingImpl.class);
td_Encoding.addPropertyParameters("headers", String.class, Header.class);
types.put(td_Encoding.getType(), td_Encoding);
T td_PathItem = factoryFunction.apply(PathItem.class, PathItemImpl.class);
td_PathItem.addPropertyParameters("servers", Server.class);
td_PathItem.addPropertyParameters("parameters", Parameter.class);
types.put(td_PathItem.getType(), td_PathItem);
T td_Tag = factoryFunction.apply(Tag.class, TagImpl.class);
types.put(td_Tag.getType(), td_Tag);
T td_Operation = factoryFunction.apply(Operation.class, OperationImpl.class);
td_Operation.addPropertyParameters("tags", String.class);
td_Operation.addPropertyParameters("parameters", Parameter.class);
td_Operation.addPropertyParameters("callbacks", String.class, Callback.class);
td_Operation.addPropertyParameters("security", SecurityRequirement.class);
td_Operation.addPropertyParameters("servers", Server.class);
types.put(td_Operation.getType(), td_Operation);
T td_Schema = factoryFunction.apply(Schema.class, SchemaImpl.class);
td_Schema.addPropertyParameters("enumeration", Object.class);
td_Schema.addPropertyParameters("required", String.class);
td_Schema.addPropertyParameters("properties", String.class, Schema.class);
td_Schema.addPropertyParameters("allOf", Schema.class);
td_Schema.addPropertyParameters("anyOf", Schema.class);
td_Schema.addPropertyParameters("oneOf", Schema.class);
types.put(td_Schema.getType(), td_Schema);
T td_Header = factoryFunction.apply(Header.class, HeaderImpl.class);
td_Header.addPropertyParameters("examples", String.class, Example.class);
types.put(td_Header.getType(), td_Header);
T td_RequestBody = factoryFunction.apply(RequestBody.class, RequestBodyImpl.class);
types.put(td_RequestBody.getType(), td_RequestBody);
T td_Callback = factoryFunction.apply(Callback.class, CallbackImpl.class);
td_Callback.addPropertyParameters("pathItems", String.class, PathItem.class);
types.put(td_Callback.getType(), td_Callback);
T td_SecurityScheme = factoryFunction.apply(SecurityScheme.class, SecuritySchemeImpl.class);
types.put(td_SecurityScheme.getType(), td_SecurityScheme);
T td_Contact = factoryFunction.apply(Contact.class, ContactImpl.class);
types.put(td_Contact.getType(), td_Contact);
T td_Content = factoryFunction.apply(Content.class, ContentImpl.class);
td_Content.addPropertyParameters("mediaTypes", String.class, MediaType.class);
types.put(td_Content.getType(), td_Content);
T td_OAuthFlows = factoryFunction.apply(OAuthFlows.class, OAuthFlowsImpl.class);
types.put(td_OAuthFlows.getType(), td_OAuthFlows);
T td_ServerVariables = factoryFunction.apply(ServerVariables.class, ServerVariablesImpl.class);
td_ServerVariables.addPropertyParameters("serverVariables", String.class, ServerVariable.class);
types.put(td_ServerVariables.getType(), td_ServerVariables);
T td_Link = factoryFunction.apply(Link.class, LinkImpl.class);
td_Link.addPropertyParameters("parameters", String.class, Object.class);
types.put(td_Link.getType(), td_Link);
T td_Parameter = factoryFunction.apply(Parameter.class, ParameterImpl.class);
td_Parameter.addPropertyParameters("examples", String.class, Example.class);
types.put(td_Parameter.getType(), td_Parameter);
T td_Scopes = factoryFunction.apply(Scopes.class, ScopesImpl.class);
td_Scopes.addPropertyParameters("scopes", String.class, String.class);
types.put(td_Scopes.getType(), td_Scopes);
T td_OpenAPI = factoryFunction.apply(OpenAPI.class, OpenAPIImpl.class);
td_OpenAPI.addPropertyParameters("servers", Server.class);
td_OpenAPI.addPropertyParameters("security", SecurityRequirement.class);
td_OpenAPI.addPropertyParameters("tags", Tag.class);
types.put(td_OpenAPI.getType(), td_OpenAPI);
T td_APIResponses = factoryFunction.apply(APIResponses.class, APIResponsesImpl.class);
td_APIResponses.addPropertyParameters("APIResponses", String.class, APIResponse.class);
types.put(td_APIResponses.getType(), td_APIResponses);
T td_ExternalDocumentation = factoryFunction.apply(ExternalDocumentation.class, ExternalDocumentationImpl.class);
types.put(td_ExternalDocumentation.getType(), td_ExternalDocumentation);
T td_Server = factoryFunction.apply(Server.class, ServerImpl.class);
td_Server.addPropertyParameters("variables", String.class, ServerVariable.class);
types.put(td_Server.getType(), td_Server);
T td_XML = factoryFunction.apply(XML.class, XMLImpl.class);
types.put(td_XML.getType(), td_XML);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy