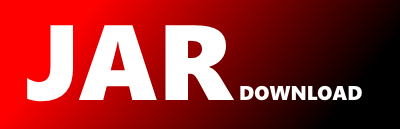
io.helidon.webserver.observe.ObserveFeatureConfig Maven / Gradle / Ivy
/*
* Copyright (c) 2024 Oracle and/or its affiliates.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.helidon.webserver.observe;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.ServiceLoader;
import java.util.function.Consumer;
import java.util.function.Supplier;
import io.helidon.builder.api.Prototype;
import io.helidon.common.Generated;
import io.helidon.common.HelidonServiceLoader;
import io.helidon.common.config.Config;
import io.helidon.cors.CrossOriginConfig;
import io.helidon.webserver.observe.spi.ObserveProvider;
import io.helidon.webserver.observe.spi.Observer;
/**
* Configuration for observability feature itself.
*
* @see #builder()
* @see #create()
*/
@Generated(value = "io.helidon.builder.codegen.BuilderCodegen", trigger = "io.helidon.webserver.observe.ObserveFeatureConfigBlueprint")
public interface ObserveFeatureConfig extends ObserveFeatureConfigBlueprint, Prototype.Api {
/**
* Create a new fluent API builder to customize configuration.
*
* @return a new builder
*/
static ObserveFeatureConfig.Builder builder() {
return new ObserveFeatureConfig.Builder();
}
/**
* Create a new fluent API builder from an existing instance.
*
* @param instance an existing instance used as a base for the builder
* @return a builder based on an instance
*/
static ObserveFeatureConfig.Builder builder(ObserveFeatureConfig instance) {
return ObserveFeatureConfig.builder().from(instance);
}
/**
* Create a new instance from configuration.
*
* @param config used to configure the new instance
* @return a new instance configured from configuration
*/
static ObserveFeatureConfig create(Config config) {
return ObserveFeatureConfig.builder().config(config).buildPrototype();
}
/**
* Create a new instance with default values.
*
* @return a new instance
*/
static ObserveFeatureConfig create() {
return ObserveFeatureConfig.builder().buildPrototype();
}
/**
* Fluent API builder base for {@link ObserveFeature}.
*
* @param type of the builder extending this abstract builder
* @param type of the prototype interface that would be built by {@link #buildPrototype()}
*/
abstract class BuilderBase, PROTOTYPE extends ObserveFeatureConfig> implements Prototype.ConfiguredBuilder {
private final List observers = new ArrayList<>();
private final List sockets = new ArrayList<>();
private boolean enabled = true;
private boolean isObserversMutated;
private boolean isSocketsMutated;
private boolean observersDiscoverServices = true;
private Config config;
private CrossOriginConfig cors = CrossOriginConfig.create();
private double weight = 80.0;
private String endpoint = "/observe";
private String name = "observe";
/**
* Protected to support extensibility.
*/
protected BuilderBase() {
}
/**
* Update this builder from an existing prototype instance. This method disables automatic service discovery.
*
* @param prototype existing prototype to update this builder from
* @return updated builder instance
*/
public BUILDER from(ObserveFeatureConfig prototype) {
cors(prototype.cors());
enabled(prototype.enabled());
endpoint(prototype.endpoint());
weight(prototype.weight());
if (!isObserversMutated) {
observers.clear();
}
addObservers(prototype.observers());
observersDiscoverServices = false;
this.config = prototype.config().orElse(null);
if (!isSocketsMutated) {
sockets.clear();
}
addSockets(prototype.sockets());
name(prototype.name());
return self();
}
/**
* Update this builder from an existing prototype builder instance.
*
* @param builder existing builder prototype to update this builder from
* @return updated builder instance
*/
public BUILDER from(ObserveFeatureConfig.BuilderBase, ?> builder) {
cors(builder.cors());
enabled(builder.enabled());
endpoint(builder.endpoint());
weight(builder.weight());
if (isObserversMutated) {
if (builder.isObserversMutated) {
addObservers(builder.observers);
}
} else {
observers.clear();
addObservers(builder.observers);
}
observersDiscoverServices = builder.observersDiscoverServices;
builder.config().ifPresent(this::config);
if (isSocketsMutated) {
if (builder.isSocketsMutated) {
addSockets(builder.sockets);
}
} else {
sockets.clear();
addSockets(builder.sockets);
}
name(builder.name());
return self();
}
/**
* Update builder from configuration (node of this type).
* If a value is present in configuration, it would override currently configured values.
*
* @param config configuration instance used to obtain values to update this builder
* @return updated builder instance
*/
@Override
public BUILDER config(Config config) {
Objects.requireNonNull(config);
this.config = config;
config.get("cors").map(CrossOriginConfig::create).ifPresent(this::cors);
config.get("enabled").as(Boolean.class).ifPresent(this::enabled);
config.get("endpoint").as(String.class).ifPresent(this::endpoint);
config.get("weight").as(Double.class).ifPresent(this::weight);
config.get("sockets").asList(String.class).ifPresent(this::sockets);
return self();
}
/**
* Cors support inherited by each observe provider, unless explicitly configured.
*
* @param cors cors support to use
* @return updated builder instance
* @see #cors()
*/
public BUILDER cors(CrossOriginConfig cors) {
Objects.requireNonNull(cors);
this.cors = cors;
return self();
}
/**
* Cors support inherited by each observe provider, unless explicitly configured.
*
* @param consumer consumer of builder for
* cors support to use
* @return updated builder instance
* @see #cors()
*/
public BUILDER cors(Consumer consumer) {
Objects.requireNonNull(consumer);
var builder = CrossOriginConfig.builder();
consumer.accept(builder);
this.cors(builder.build());
return self();
}
/**
* Cors support inherited by each observe provider, unless explicitly configured.
*
* @param supplier supplier of
* cors support to use
* @return updated builder instance
* @see #cors()
*/
public BUILDER cors(Supplier extends CrossOriginConfig> supplier) {
Objects.requireNonNull(supplier);
this.cors(supplier.get());
return self();
}
/**
* Whether the observe support is enabled.
*
* @param enabled {@code false} to disable observe feature
* @return updated builder instance
* @see #enabled()
*/
public BUILDER enabled(boolean enabled) {
this.enabled = enabled;
return self();
}
/**
* Root endpoint to use for observe providers. By default, all observe endpoint are under this root endpoint.
*
* Example:
*
* If root endpoint is {@code /observe} (the default), and default health endpoint is {@code health} (relative),
* health endpoint would be {@code /observe/health}.
*
* @param endpoint endpoint to use
* @return updated builder instance
* @see #endpoint()
*/
public BUILDER endpoint(String endpoint) {
Objects.requireNonNull(endpoint);
this.endpoint = endpoint;
return self();
}
/**
* Change the weight of this feature. This may change the order of registration of this feature.
* By default, observability weight is {@value ObserveFeature#WEIGHT} so it is registered after routing.
*
* @param weight weight to use
* @return updated builder instance
* @see #weight()
*/
public BUILDER weight(double weight) {
this.weight = weight;
return self();
}
/**
* Observers to use with this observe features.
* Each observer type is registered only once, unless it uses a custom name (default name is the same as the type).
*
* @param discoverServices whether to discover implementations through service loader
* @return updated builder instance
* @see #observers()
*/
public BUILDER observersDiscoverServices(boolean discoverServices) {
this.observersDiscoverServices = discoverServices;
return self();
}
/**
* Observers to use with this observe features.
* Each observer type is registered only once, unless it uses a custom name (default name is the same as the type).
*
* @param observers list of observers to use in this feature
* @return updated builder instance
* @see #observers()
*/
public BUILDER observers(List extends Observer> observers) {
Objects.requireNonNull(observers);
isObserversMutated = true;
this.observers.clear();
this.observers.addAll(observers);
return self();
}
/**
* Observers to use with this observe features.
* Each observer type is registered only once, unless it uses a custom name (default name is the same as the type).
*
* @param observers list of observers to use in this feature
* @return updated builder instance
* @see #observers()
*/
public BUILDER addObservers(List extends Observer> observers) {
Objects.requireNonNull(observers);
isObserversMutated = true;
this.observers.addAll(observers);
return self();
}
/**
* Observers to use with this observe features.
* Each observer type is registered only once, unless it uses a custom name (default name is the same as the type).
*
* @param observer list of observers to use in this feature
* @return updated builder instance
* @see #observers()
*/
public BUILDER addObserver(Observer observer) {
Objects.requireNonNull(observer);
this.observers.add(observer);
isObserversMutated = true;
return self();
}
/**
* Sockets the observability endpoint should be exposed on. If not defined, defaults to the default socket
* ({@value io.helidon.webserver.WebServer#DEFAULT_SOCKET_NAME}.
* Each observer may have its own configuration of sockets that are relevant to it, this only controls the endpoints!
*
* @param sockets list of sockets to register observe endpoint on
* @return updated builder instance
* @see #sockets()
*/
public BUILDER sockets(List extends String> sockets) {
Objects.requireNonNull(sockets);
isSocketsMutated = true;
this.sockets.clear();
this.sockets.addAll(sockets);
return self();
}
/**
* Sockets the observability endpoint should be exposed on. If not defined, defaults to the default socket
* ({@value io.helidon.webserver.WebServer#DEFAULT_SOCKET_NAME}.
* Each observer may have its own configuration of sockets that are relevant to it, this only controls the endpoints!
*
* @param sockets list of sockets to register observe endpoint on
* @return updated builder instance
* @see #sockets()
*/
public BUILDER addSockets(List extends String> sockets) {
Objects.requireNonNull(sockets);
isSocketsMutated = true;
this.sockets.addAll(sockets);
return self();
}
/**
* Name of this instance.
*
* @param name instance name
* @return updated builder instance
* @see #name()
*/
public BUILDER name(String name) {
Objects.requireNonNull(name);
this.name = name;
return self();
}
/**
* Cors support inherited by each observe provider, unless explicitly configured.
*
* @return the cors
*/
public CrossOriginConfig cors() {
return cors;
}
/**
* Whether the observe support is enabled.
*
* @return the enabled
*/
public boolean enabled() {
return enabled;
}
/**
* Root endpoint to use for observe providers. By default, all observe endpoint are under this root endpoint.
*
* Example:
*
* If root endpoint is {@code /observe} (the default), and default health endpoint is {@code health} (relative),
* health endpoint would be {@code /observe/health}.
*
* @return the endpoint
*/
public String endpoint() {
return endpoint;
}
/**
* Change the weight of this feature. This may change the order of registration of this feature.
* By default, observability weight is {@value ObserveFeature#WEIGHT} so it is registered after routing.
*
* @return the weight
*/
public double weight() {
return weight;
}
/**
* Observers to use with this observe features.
* Each observer type is registered only once, unless it uses a custom name (default name is the same as the type).
*
* @return the observers
*/
public List observers() {
return observers;
}
/**
* Sockets the observability endpoint should be exposed on. If not defined, defaults to the default socket
* ({@value io.helidon.webserver.WebServer#DEFAULT_SOCKET_NAME}.
* Each observer may have its own configuration of sockets that are relevant to it, this only controls the endpoints!
*
* @return the sockets
*/
public List sockets() {
return sockets;
}
/**
* Name of this instance.
*
* @return the name
*/
public String name() {
return name;
}
/**
* If this instance was configured, this would be the config instance used.
*
* @return config node used to configure this builder, or empty if not configured
*/
public Optional config() {
return Optional.ofNullable(config);
}
@Override
public String toString() {
return "ObserveFeatureConfigBuilder{"
+ "cors=" + cors + ","
+ "enabled=" + enabled + ","
+ "endpoint=" + endpoint + ","
+ "weight=" + weight + ","
+ "observers=" + observers + ","
+ "config=" + config + ","
+ "sockets=" + sockets + ","
+ "name=" + name
+ "}";
}
/**
* Handles providers and decorators.
*/
@SuppressWarnings("unchecked")
protected void preBuildPrototype() {
var config = this.config == null ? Config.empty() : this.config;
{
var serviceLoader = HelidonServiceLoader.create(ServiceLoader.load(ObserveProvider.class));
this.addObservers(discoverServices(config, "observers", serviceLoader, ObserveProvider.class, Observer.class, observersDiscoverServices, observers));
}
}
/**
* Validates required properties.
*/
protected void validatePrototype() {
}
/**
* Generated implementation of the prototype, can be extended by descendant prototype implementations.
*/
protected static class ObserveFeatureConfigImpl implements ObserveFeatureConfig, Supplier {
private final boolean enabled;
private final CrossOriginConfig cors;
private final double weight;
private final List observers;
private final List sockets;
private final Optional config;
private final String endpoint;
private final String name;
/**
* Create an instance providing a builder.
*
* @param builder extending builder base of this prototype
*/
protected ObserveFeatureConfigImpl(ObserveFeatureConfig.BuilderBase, ?> builder) {
this.cors = builder.cors();
this.enabled = builder.enabled();
this.endpoint = builder.endpoint();
this.weight = builder.weight();
this.observers = List.copyOf(builder.observers());
this.config = builder.config();
this.sockets = List.copyOf(builder.sockets());
this.name = builder.name();
}
@Override
public ObserveFeature build() {
return ObserveFeature.create(this);
}
@Override
public ObserveFeature get() {
return build();
}
@Override
public CrossOriginConfig cors() {
return cors;
}
@Override
public boolean enabled() {
return enabled;
}
@Override
public String endpoint() {
return endpoint;
}
@Override
public double weight() {
return weight;
}
@Override
public List observers() {
return observers;
}
@Override
public Optional config() {
return config;
}
@Override
public List sockets() {
return sockets;
}
@Override
public String name() {
return name;
}
@Override
public String toString() {
return "ObserveFeatureConfig{"
+ "cors=" + cors + ","
+ "enabled=" + enabled + ","
+ "endpoint=" + endpoint + ","
+ "weight=" + weight + ","
+ "observers=" + observers + ","
+ "config=" + config + ","
+ "sockets=" + sockets + ","
+ "name=" + name
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (!(o instanceof ObserveFeatureConfig other)) {
return false;
}
return Objects.equals(cors, other.cors())
&& enabled == other.enabled()
&& Objects.equals(endpoint, other.endpoint())
&& weight == other.weight()
&& Objects.equals(observers, other.observers())
&& Objects.equals(config, other.config())
&& Objects.equals(sockets, other.sockets())
&& Objects.equals(name, other.name());
}
@Override
public int hashCode() {
return Objects.hash(cors, enabled, endpoint, weight, observers, config, sockets, name);
}
}
}
/**
* Fluent API builder for {@link ObserveFeature}.
*/
class Builder extends ObserveFeatureConfig.BuilderBase implements io.helidon.common.Builder {
private Builder() {
}
@Override
public ObserveFeatureConfig buildPrototype() {
preBuildPrototype();
validatePrototype();
return new ObserveFeatureConfigImpl(this);
}
@Override
public ObserveFeature build() {
return ObserveFeature.create(this.buildPrototype());
}
}
}