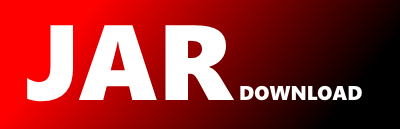
se.config.extensions.adoc Maven / Gradle / Ivy
///////////////////////////////////////////////////////////////////////////////
Copyright (c) 2018, 2024 Oracle and/or its affiliates.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
///////////////////////////////////////////////////////////////////////////////
= Extensions
:description: Helidon config extensions
:keywords: helidon, config
:feature-name: Config
:rootdir: {docdir}/../..
include::{rootdir}/includes/se.adoc[]
== Contents
- <>
- <>
- <>
- <>
- <>
- <>
- <>
- <>
- <>
== Overview
Developer-provided extensions influence how the config system behaves.
The xref:introduction.adoc#_getting_started[config system introduction] explains the design of the config
system and how its parts work together to read and parse config data, convert it
to Java types, fine-tune the look-up of config data, and reload and
reprocess data when it changes. _Config extensions_ provided by the application
modify and expand the way the config system performs these steps.
Each config extension implements one of the interfaces defined in the Configuration SPI:
//Once our asciidoc processing handles labeled lists, uncomment the following
//and use it instead of the bulleted list which follows:
//`ConfigSource`:: Loads raw configuration data from a given type of source and
//delegates to a `ConfigParser`, producing the in-memory data structure which
//represents the loaded and parsed configuration.
//`ConfigParser`:: Translates configuration content in a given format into the
//corresponding internal config data structures.
//`OverrideSource`:: Provides key/value pairs which override config values loaded
//from any `ConfigSource`, given the key but _ignoring_ the original value.
//`ConfigFilter`:: Transforms config `String` values returned from any value-type
//`Config` node, given the key _and_ the original value.
//`ConfigMapperProvider`:: Provides one or more ``ConfigMapper``s each of which
//converts a `Config` object tree to a Java type specific to the application.
//`PollingStrategy`:: Implements a custom technique for notifying the Config system
//when the data underlying a `ConfigSource` or `OverrideSource` has changed.
* `ConfigSource` - Loads raw configuration data from a given type of source and delegates to a `ConfigParser`, producing the in-memory data structure which represents the loaded and parsed configuration.
* `ConfigParser` - Translates configuration content in a given format into the corresponding internal config data structures.
* `OverrideSource` - Provides key/value pairs which override config values loaded from any `ConfigSource`, given the key and _ignoring_ the original value.
* `ConfigFilter` - Transforms config `String` values returned from any value-type
`Config` node, given the key _and_ the original value.
* `ConfigMapperProvider` - Provides one or more ``ConfigMapper``s each of which converts a `Config` object tree to a Java type specific to the application.
* `PollingStrategy` - Implements a custom technique to trigger polling of underlying sources for changes
* `ChangeWatcher` - Implements a custom technique to watch underlying sources for changes and notifying the config system of such a change
The extension mechanism of Config can also use Java `ServiceLoader`.
For this purpose, you implement providers that serve as factories for your implementation of an extension.
This is to support config profiles even for custom extensions.
Service providers:
* `ConfigMapperProvider` - support for config mappers, automatically discovered by the config system
* `ConfigFilter` - support for config filters, automatically discovered by the config system
* `ConfigParser` - support for config parsers, automatically discovered by the config system
* `ConfigSourceProvider` - support for named config sources, configurable through profiles
* `ChangeWatcherProvider` - support for named change watchers, configurable through profiles
* `OverrideSourceProvider` - support for named override sources, configurable through profiles
* `PollingStrategyProvider` - support for named polling strategies, configurable through profiles
* `RetryPolicyProvider` - support for retry policies, configurable through profiles
The config system itself implements several of these SPIs, as noted in the sections below.
== Configuring an Extension
You can configure a custom extension in two ways:
1. Manual configuration with builder
2. Automatic configuration using a Java service loader
=== Manual Configuration with Builder
The following example shows configuration of all possible extensions with `Config` (all custom extension have a name prefix `My`):
[source,java]
----
include::{sourcedir}/se/config/ExtensionsSnippets.java[tag=snippet_1, indent=0]
----
=== Automatic Configuration Using a Service Loader
The following extensions are loaded using a service loader for any configuration instance, and do not require an explicit setup:
* `ConfigParser` - each config parser on the classpath that implements `ConfigParserProvider` as a Java service loader service
* `ConfigFilter` - each filter on the classpath that implements `ConfigFilter` as a Java service loader service
Other extensions are only used from Java service loader when you use config profiles.
Mapping is done through the type configured in config profile, and the type defined by the extension provider interface.
For example for config sources, the interface defines the following methods (only subset shown):
[source,java]
----
include::{sourcedir}/se/config/ExtensionsSnippets.java[tag=snippet_2, indent=0]
----
Considering the following meta configuration (or config profile):
[source,yaml]
----
sources:
- type: "my-type"
properties:
my-config: "configuration"
----
The config system would iterate through all `ConfigSourceProvider` implementations found through Java `ServiceLoader` based on
their link:{common-javadoc-base-url}/io/helidon/common/Weight.html[weight].
First provider that returns `true` when `supports("my-type")` is called would be used, and an instance of a `ConfigSource`
created using `create("my-type", config)`, where `config` is located on the node of `properties` from config profile.
=== About Priority [[priority-info]]
The config system invokes extensions of a given type in priority order.
Developers can express the relative importance of an extension by annotating the service implementation class with
link:{common-javadoc-base-url}/io/helidon/common/Weight.html[`@Weight`].
The default value is 100. The higher the weight, the more important the extension is.
== ConfigSource SPI [[Config-SPI-ConfigSource]]
The config system includes built-in support for several types of sources
(for example, Java `String`, `Readable`, `Properties`, and `Map`
objects - see link:{config-javadoc-base-url}/io/helidon/config/ConfigSources.html[`ConfigSources`]).
Implement a link:{config-javadoc-base-url}/io/helidon/config/spi/ConfigSource.html[`ConfigSource`] to
load raw configuration data from a type of source that the config system does
not already support.
image::config/spi-ConfigSource.png[title="ConfigSource SPI",align="center"]
For config sources that work directly with config nodes, the following API is available.
These interfaces have an implementation provided by Helidon.
The interfaces `ConfigNode`, `ObjectNode`, `ValueNode` and
`ListNode` represent the in-memory data structure for loaded and parsed configuration data.
image::config/spi-node.png[title="ConfigNode SPI",align="center"]
For config sources that work return data (`NodeConfigSource` and `ParsableConfigSource`) a
`Content` must be returned that describes the loaded data.
The following diagram depicts the `Content` API.
image::config/spi-content.png[title="Content SPI",align="center"]
Some methods provided are not always mandatory, yet they are part of the APIs to simplify the overall class structure:
* ConfigContent.stamp() - this method is used by `PollingStrategy` to determine if content has been changed.
This can be always
`empty` for sources, that do not implement `PollableSource`
* ConfigParser.Content.charset() - this can return any `Charset` for media types that are binary
* ConfigParser.Content.mediaType() - this can be used to override media type (that would otherwise be "guessed" from the underlying source)
* ParsableSource.parser() - this can be used to override parser (that would otherwise be based on `mediaType`)
* ParsableSource.mediaType() - return the configured or "guessed" media type of this source, see
`io.helidon.common.media.type.MediaTypes`, if not returned, media type must be present on `Content`, or provided through media type mapping
== ConfigParser SPI [[Config-SPI-ConfigParser]]
The parsing step converts config data in some format into the corresponding in-memory representation of config ``ObjectNode``s.
The config system can already parse several data formats (for example Java `Properties`, YAML, and HOCON).
Implement the
link:{config-javadoc-base-url}/io/helidon/config/spi/ConfigParser.html[`ConfigParser`] SPI to allow the config system to handle additional formats.
image::config/spi-ConfigParser.png[title="ConfigParser SPI",align="center"]
The `ConfigParser.Content` interface defines operations on the content that is to be parsed by a `ConfigParser` implementation:
* `mediaType()` - Reports the media type of the content (if it is to override media type defined on the config source)
* `data()` - Provides the `InputStream` with config source data
* `charset()` - Defines the charset to use to parse the stream in case this is a text based media type, ignored by parsers of binary content
The application can register parsers for a builder by invoking `Config.Builder#addParser(ConfigParser)`.
The config system also uses the Java service loader mechanism to load automatically, for all builders, any parsers listed in the
`META-INF/services/io.helidon.config.spi.ConfigParser` resource on the runtime classpath.
Prevent automatic loading of parsers for a given builder by invoking `Config.Builder#disableParserServices()`.
`ConfigParser` accepts link:{common-javadoc-base-url}/io/helidon/common/Weight.html[`@Weight`].
See <>.
[source,listing]
.Example custom parser implementation listed in `META-INF/services/io.helidon.config.spi.ConfigParser`
----
my.module.MyConfigParser
----
== OverrideSource SPI [[Config-SPI-OverrideSource]]
When the application retrieves a configuration value the config system first uses
the relevant config sources and filters. It then applies any _overrides_ the
application has provided. Each override has:
* a `Predicate` (a boolean-valued function that operates on
the config key), and
* a replacement, _overriding_, `String` value the config system should use if the predicate evaluates to true.
To furnish overrides to the config system, implement the
link:{config-javadoc-base-url}/io/helidon/config/spi/OverrideSource.html[`OverrideSource`] SPI one or more times and pass instances of
those implementations to the config builder's
link:{config-javadoc-base-url}/io/helidon/config/Config.Builder.html#overrides-java.util.function.Supplier-[`overrides`] method.
The config system will apply the overrides returned from each
`OverrideSource` to each config key requested from a `Config` that is based on that `Config.Builder`.
To support custom override sources in config profiles, also implement the
link:{config-javadoc-base-url}/io/helidon/config/spi/OverrideSourceProvider.html[`OverrideSourceProvider`] service loader SPI
image::config/spi-OverrideSource.png[title="OverrideSource SPI",align="center"]
Note that override sources can also implement `PollableSource`, and `WatchableSource` to add change support.
== ConfigFilter SPI [[Config-SPI-ConfigFilter]]
Before returning a `String` from `Config.value()` the config system applies any _filters_ set up on the
`Config.Builder` used to create the config tree that contains the config node of interest.
The application provides filters as implementations of the
link:{config-javadoc-base-url}/io/helidon/config/spi/ConfigFilter.html[`ConfigFilter`] interface.
Each filter is a function which accepts a `Config.Key` and an input `String` value and returns a `String` value
the config system should use for that key going forward.
The filter can return the original value or return some other value.
The application registers filters and filter providers by passing `ConfigFilter`
implementations to one of the config builder
link:{config-javadoc-base-url}/io/helidon/config/Config.Builder.html[`addFilter` methods]. The config
system also uses the Java service loader mechanism to load
additional filters automatically, for all builders, using
the service interface described in the following table. Prevent a given
builder from using the automatically loaded filters by invoking the
link:{config-javadoc-base-url}/io/helidon/config/Config.Builder.html#disableFilterServices--[`disableFilterServices`]
method.
.Config SPI Interfaces for Filtering
|===
|Interface |Method |Usage
|link:{config-javadoc-base-url}/io/helidon/config/spi/ConfigFilter.html[`ConfigFilter`]
Accepts link:{common-javadoc-base-url}/io/helidon/common/Weight.html[`@Weight`]. See <>.
|`String apply(Config.Key key, String stringValue);`
|Accepts a key and the corresponding `String` value and
returns the `String` which the config system should use for that key.
|===
=== Initializing Filters
The `ConfigFilter` JavaDoc describes multiple methods for adding filters to a
`Config.Builder`. Some accept a `ConfigFilter` directly and some accept a provider
function which, when passed a `Config` instance, returns a `ConfigFilter`.
*_Neither a `ConfigFilter` nor a provider function which furnishes one should
access the `Config` instance passed to the provider function._*
Instead, implement the `ConfigFilter.init(Config)` method on the filter. The config
system invokes the filters' `init` methods according to the filters <>.
Recall that whenever any code invokes `Config.get`, the `Config` instance
invokes the `apply` method of _all_ registered filters. By the time the application
retrieves config this way the config system will have run the `init` method on all
the filters. _But note that when a filter's `init` method invokes `Config.get`, the
`init` methods of lower-priority filters will not yet have run._
image::config/spi-ConfigFilter.png[title="ConfigFilter SPI",align="center"]
== ConfigMapperProvider SPI [[Config-SPI-ConfigMapperProvider]]
The config system provides built-in mappings from `String` values to various Java
types. (See link:{config-javadoc-base-url}/io/helidon/config/ConfigMappers.html[`ConfigMappers`].)
To handle mappings to other types the application can register
custom mappers with the config system by implementing the
link:{config-javadoc-base-url}/io/helidon/config/spi/ConfigMapperProvider.html[`ConfigMapperProvider`]
SPI.
Such providers return a map, with entries in which:
* the key is the Java type (a `Class` object) the mapper produces, and
* the value is a `ConfigMapper` that converts the config in-memory
data structure into the type in the key.
The provider may also implement other methods for finer tuned conversion mechanisms:
* `genericTypeMappers()` returns a map with entries for specific `GenericType` conversions,
for example when the provider supports only mapping for `GenericType
© 2015 - 2025 Weber Informatics LLC | Privacy Policy