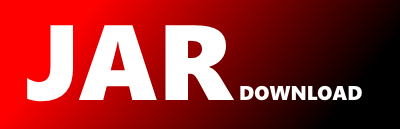
io.holunda.camunda.taskpool.api.business.AuthorizationChange.kt Maven / Gradle / Ivy
package io.holunda.camunda.taskpool.api.business
/**
* Change of authorization on the business object.
*/
sealed class AuthorizationChange {
companion object {
/**
* Adds a user.
*/
@JvmStatic
fun addUser(username: String): AuthorizationChange = AddAuthorization(authorizedUsers = listOf(username))
/**
* Removes a user.
*/
@JvmStatic
fun removeUser(username: String): AuthorizationChange = RemoveAuthorization(authorizedUsers = listOf(username))
/**
* Adds a group.
*/
@JvmStatic
fun addGroup(groupName: String): AuthorizationChange = AddAuthorization(authorizedGroups = listOf(groupName))
/**
* Removes a group.
*/
@JvmStatic
fun removeGroup(groupName: String): AuthorizationChange = RemoveAuthorization(authorizedGroups = listOf(groupName))
/**
* Applies authorizations to users.
* @return a list of authorized users.
*/
@JvmStatic
fun applyUserAuthorization(authorizedUsers: List, authorizationChanges: List): List {
val usersToRemove = authorizationChanges.filterIsInstance().flatMap { it.authorizedUsers }
val usersToAdd = authorizationChanges.filterIsInstance().flatMap { it.authorizedUsers }
val mutable = authorizedUsers.toMutableList()
mutable.addAll(usersToAdd)
mutable.removeAll(usersToRemove)
return mutable.toSet().toList()
}
/**
* Applies authorizations to groups.
* @return a list of authorized groups.
*/
@JvmStatic
fun applyGroupAuthorization(authorizedGroups: List, authorizationChanges: List): List {
val groupsToRemove = authorizationChanges.filterIsInstance().flatMap { it.authorizedGroups }
val groupsToAdd = authorizationChanges.filterIsInstance().flatMap { it.authorizedGroups }
val mutable = authorizedGroups.toMutableList()
mutable.addAll(groupsToAdd)
mutable.removeAll(groupsToRemove)
return mutable.toSet().toList()
}
}
}
/**
* Grants access to data entry.
*/
data class AddAuthorization(
/**
* List of authorized users to grant access.
*/
val authorizedUsers: List = listOf(),
/**
* List of authorized groups to grant access.
*/
val authorizedGroups: List = listOf()
) : AuthorizationChange()
/**
* Removes access to data entry.
*/
data class RemoveAuthorization(
/**
* List of authorized users to grant access.
*/
val authorizedUsers: List = listOf(),
/**
* List of authorized groups to grant access.
*/
val authorizedGroups: List = listOf()
) : AuthorizationChange()
© 2015 - 2024 Weber Informatics LLC | Privacy Policy