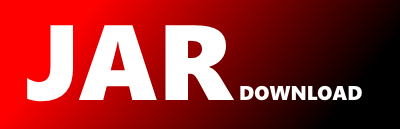
io.holunda.camunda.taskpool.cockpit.rest.model.TaskDto Maven / Gradle / Ivy
package io.holunda.camunda.taskpool.cockpit.rest.model;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.validation.Valid;
import javax.validation.constraints.*;
/**
* TaskDto
*/
@javax.annotation.Generated(value = "io.swagger.codegen.languages.SpringCodegen", date = "2019-04-02T18:15:46.103+02:00")
public class TaskDto {
@JsonProperty("id")
private String id = null;
@JsonProperty("formKey")
private String formKey = null;
@JsonProperty("processName")
private String processName = null;
@JsonProperty("taskDefinitionKey")
private String taskDefinitionKey = null;
@JsonProperty("processDefinitionKey")
private String processDefinitionKey = null;
@JsonProperty("applicationName")
private String applicationName = null;
@JsonProperty("tenantId")
private String tenantId = null;
@JsonProperty("name")
private String name = null;
@JsonProperty("description")
private String description = null;
@JsonProperty("candidateGroups")
private List candidateGroups = new ArrayList<>();
@JsonProperty("candidateUsers")
private List candidateUsers = new ArrayList<>();
@JsonProperty("assignee")
private String assignee = null;
@JsonProperty("createTime")
private OffsetDateTime createTime = null;
@JsonProperty("dueDate")
private OffsetDateTime dueDate = null;
@JsonProperty("businessKey")
private String businessKey = null;
@JsonProperty("priority")
private Integer priority = null;
@JsonProperty("payload")
private Map payload = null;
public TaskDto id(String id) {
this.id = id;
return this;
}
/**
* Id of the user task.
* @return id
**/
@ApiModelProperty(required = true, value = "Id of the user task.")
@NotNull
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public TaskDto formKey(String formKey) {
this.formKey = formKey;
return this;
}
/**
* Form key of the task (as defined in the process model).
* @return formKey
**/
@ApiModelProperty(value = "Form key of the task (as defined in the process model).")
public String getFormKey() {
return formKey;
}
public void setFormKey(String formKey) {
this.formKey = formKey;
}
public TaskDto processName(String processName) {
this.processName = processName;
return this;
}
/**
* Name of the process.
* @return processName
**/
@ApiModelProperty(required = true, value = "Name of the process.")
@NotNull
public String getProcessName() {
return processName;
}
public void setProcessName(String processName) {
this.processName = processName;
}
public TaskDto taskDefinitionKey(String taskDefinitionKey) {
this.taskDefinitionKey = taskDefinitionKey;
return this;
}
/**
* Task definition key from BPMN.
* @return taskDefinitionKey
**/
@ApiModelProperty(value = "Task definition key from BPMN.")
public String getTaskDefinitionKey() {
return taskDefinitionKey;
}
public void setTaskDefinitionKey(String taskDefinitionKey) {
this.taskDefinitionKey = taskDefinitionKey;
}
public TaskDto processDefinitionKey(String processDefinitionKey) {
this.processDefinitionKey = processDefinitionKey;
return this;
}
/**
* Process definition key from BPMN.
* @return processDefinitionKey
**/
@ApiModelProperty(value = "Process definition key from BPMN.")
public String getProcessDefinitionKey() {
return processDefinitionKey;
}
public void setProcessDefinitionKey(String processDefinitionKey) {
this.processDefinitionKey = processDefinitionKey;
}
public TaskDto applicationName(String applicationName) {
this.applicationName = applicationName;
return this;
}
/**
* Application name.
* @return applicationName
**/
@ApiModelProperty(value = "Application name.")
public String getApplicationName() {
return applicationName;
}
public void setApplicationName(String applicationName) {
this.applicationName = applicationName;
}
public TaskDto tenantId(String tenantId) {
this.tenantId = tenantId;
return this;
}
/**
* Tenant Id.
* @return tenantId
**/
@ApiModelProperty(value = "Tenant Id.")
public String getTenantId() {
return tenantId;
}
public void setTenantId(String tenantId) {
this.tenantId = tenantId;
}
public TaskDto name(String name) {
this.name = name;
return this;
}
/**
* Action to execute.
* @return name
**/
@ApiModelProperty(required = true, value = "Action to execute.")
@NotNull
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public TaskDto description(String description) {
this.description = description;
return this;
}
/**
* Description of the user task.
* @return description
**/
@ApiModelProperty(required = true, value = "Description of the user task.")
@NotNull
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public TaskDto candidateGroups(List candidateGroups) {
this.candidateGroups = candidateGroups;
return this;
}
public TaskDto addCandidateGroupsItem(String candidateGroupsItem) {
this.candidateGroups.add(candidateGroupsItem);
return this;
}
/**
* Set of candidate groups represented by their group ids.
* @return candidateGroups
**/
@ApiModelProperty(required = true, value = "Set of candidate groups represented by their group ids.")
@NotNull
public List getCandidateGroups() {
return candidateGroups;
}
public void setCandidateGroups(List candidateGroups) {
this.candidateGroups = candidateGroups;
}
public TaskDto candidateUsers(List candidateUsers) {
this.candidateUsers = candidateUsers;
return this;
}
public TaskDto addCandidateUsersItem(String candidateUsersItem) {
this.candidateUsers.add(candidateUsersItem);
return this;
}
/**
* Set of candidate users represented by their user ids.
* @return candidateUsers
**/
@ApiModelProperty(required = true, value = "Set of candidate users represented by their user ids.")
@NotNull
public List getCandidateUsers() {
return candidateUsers;
}
public void setCandidateUsers(List candidateUsers) {
this.candidateUsers = candidateUsers;
}
public TaskDto assignee(String assignee) {
this.assignee = assignee;
return this;
}
/**
* User name of assigned user.
* @return assignee
**/
@ApiModelProperty(value = "User name of assigned user.")
public String getAssignee() {
return assignee;
}
public void setAssignee(String assignee) {
this.assignee = assignee;
}
public TaskDto createTime(OffsetDateTime createTime) {
this.createTime = createTime;
return this;
}
/**
* Creation date and time of the task.
* @return createTime
**/
@ApiModelProperty(required = true, value = "Creation date and time of the task.")
@NotNull
@Valid
public OffsetDateTime getCreateTime() {
return createTime;
}
public void setCreateTime(OffsetDateTime createTime) {
this.createTime = createTime;
}
public TaskDto dueDate(OffsetDateTime dueDate) {
this.dueDate = dueDate;
return this;
}
/**
* Due date and time of the task.
* @return dueDate
**/
@ApiModelProperty(required = true, value = "Due date and time of the task.")
@NotNull
@Valid
public OffsetDateTime getDueDate() {
return dueDate;
}
public void setDueDate(OffsetDateTime dueDate) {
this.dueDate = dueDate;
}
public TaskDto businessKey(String businessKey) {
this.businessKey = businessKey;
return this;
}
/**
* Business Key of the process.
* @return businessKey
**/
@ApiModelProperty(required = true, value = "Business Key of the process.")
@NotNull
public String getBusinessKey() {
return businessKey;
}
public void setBusinessKey(String businessKey) {
this.businessKey = businessKey;
}
public TaskDto priority(Integer priority) {
this.priority = priority;
return this;
}
/**
* Priority of the task.
* @return priority
**/
@ApiModelProperty(value = "Priority of the task.")
public Integer getPriority() {
return priority;
}
public void setPriority(Integer priority) {
this.priority = priority;
}
public TaskDto payload(Map payload) {
this.payload = payload;
return this;
}
public TaskDto putPayloadItem(String key, Object payloadItem) {
if (this.payload == null) {
this.payload = new HashMap<>();
}
this.payload.put(key, payloadItem);
return this;
}
/**
* Get payload
* @return payload
**/
@ApiModelProperty(value = "")
public Map getPayload() {
return payload;
}
public void setPayload(Map payload) {
this.payload = payload;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TaskDto task = (TaskDto) o;
return Objects.equals(this.id, task.id) &&
Objects.equals(this.formKey, task.formKey) &&
Objects.equals(this.processName, task.processName) &&
Objects.equals(this.taskDefinitionKey, task.taskDefinitionKey) &&
Objects.equals(this.processDefinitionKey, task.processDefinitionKey) &&
Objects.equals(this.applicationName, task.applicationName) &&
Objects.equals(this.tenantId, task.tenantId) &&
Objects.equals(this.name, task.name) &&
Objects.equals(this.description, task.description) &&
Objects.equals(this.candidateGroups, task.candidateGroups) &&
Objects.equals(this.candidateUsers, task.candidateUsers) &&
Objects.equals(this.assignee, task.assignee) &&
Objects.equals(this.createTime, task.createTime) &&
Objects.equals(this.dueDate, task.dueDate) &&
Objects.equals(this.businessKey, task.businessKey) &&
Objects.equals(this.priority, task.priority) &&
Objects.equals(this.payload, task.payload);
}
@Override
public int hashCode() {
return Objects.hash(id, formKey, processName, taskDefinitionKey, processDefinitionKey, applicationName, tenantId, name, description, candidateGroups, candidateUsers, assignee, createTime, dueDate, businessKey, priority, payload);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TaskDto {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" formKey: ").append(toIndentedString(formKey)).append("\n");
sb.append(" processName: ").append(toIndentedString(processName)).append("\n");
sb.append(" taskDefinitionKey: ").append(toIndentedString(taskDefinitionKey)).append("\n");
sb.append(" processDefinitionKey: ").append(toIndentedString(processDefinitionKey)).append("\n");
sb.append(" applicationName: ").append(toIndentedString(applicationName)).append("\n");
sb.append(" tenantId: ").append(toIndentedString(tenantId)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" candidateGroups: ").append(toIndentedString(candidateGroups)).append("\n");
sb.append(" candidateUsers: ").append(toIndentedString(candidateUsers)).append("\n");
sb.append(" assignee: ").append(toIndentedString(assignee)).append("\n");
sb.append(" createTime: ").append(toIndentedString(createTime)).append("\n");
sb.append(" dueDate: ").append(toIndentedString(dueDate)).append("\n");
sb.append(" businessKey: ").append(toIndentedString(businessKey)).append("\n");
sb.append(" priority: ").append(toIndentedString(priority)).append("\n");
sb.append(" payload: ").append(toIndentedString(payload)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy