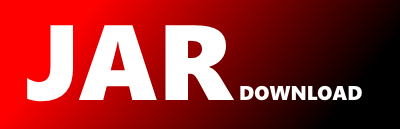
io.honeycomb.beeline.spring.beans.BeelineRestTemplateInterceptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of beeline-spring-boot-starter Show documentation
Show all versions of beeline-spring-boot-starter Show documentation
Spring Boot Starter module to auto-configure Spring Boot with the Honeycomb Beeline for Java
package io.honeycomb.beeline.spring.beans;
import io.honeycomb.beeline.tracing.Span;
import io.honeycomb.beeline.tracing.Tracer;
import io.honeycomb.beeline.tracing.propagation.HttpClientPropagator;
import io.honeycomb.beeline.tracing.propagation.HttpHeaderV1PropagationCodec;
import io.honeycomb.beeline.tracing.propagation.Propagation;
import io.honeycomb.beeline.tracing.propagation.PropagationCodec;
import io.honeycomb.libhoney.utils.Assert;
import org.springframework.boot.web.client.RestTemplateCustomizer;
import org.springframework.http.HttpRequest;
import org.springframework.http.client.ClientHttpRequestExecution;
import org.springframework.http.client.ClientHttpRequestInterceptor;
import org.springframework.http.client.ClientHttpResponse;
import java.io.IOException;
import java.util.Map;
import java.util.Optional;
import static io.honeycomb.beeline.spring.utils.InstrumentationConstants.HTTP_CLIENT_SPAN_NAME;
import static io.honeycomb.beeline.spring.utils.InstrumentationConstants.REST_TEMPLATE_INSTRUMENTATION_NAME;
import static io.honeycomb.beeline.spring.utils.MoreTraceFieldConstants.*;
public class BeelineRestTemplateInterceptor implements ClientHttpRequestInterceptor, BeelineInstrumentation {
private final HttpClientPropagator httpClientPropagator;
public BeelineRestTemplateInterceptor(final Tracer tracer) {
this(tracer, Propagation.honeycombHeaderV1());
}
public BeelineRestTemplateInterceptor(final Tracer tracer, PropagationCodec
© 2015 - 2024 Weber Informatics LLC | Privacy Policy