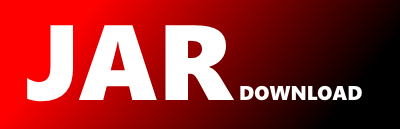
io.honnix.rkt.launcher.model.PodBuilder Maven / Gradle / Ivy
package io.honnix.rkt.launcher.model;
import io.norberg.automatter.AutoMatter;
import java.time.Instant;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
public final class PodBuilder {
private String name;
private String state;
private Optional> networks;
private Optional> appNames;
private Optional startedAt;
private Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy