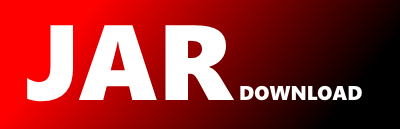
io.honnix.rkt.launcher.model.config.DockerAuthBuilder Maven / Gradle / Ivy
package io.honnix.rkt.launcher.model.config;
import io.norberg.automatter.AutoMatter;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import javax.annotation.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
public final class DockerAuthBuilder {
private String rktVersion;
private String rktKind;
private List registries;
private Stage0Entry.DockerAuth.BasicCredentials credentials;
public DockerAuthBuilder() {
}
private DockerAuthBuilder(Stage0Entry.DockerAuth v) {
this.rktVersion = v.rktVersion();
this.rktKind = v.rktKind();
List extends String> _registries = v.registries();
this.registries = (_registries == null) ? null : new ArrayList(_registries);
this.credentials = v.credentials();
}
private DockerAuthBuilder(DockerAuthBuilder v) {
this.rktVersion = v.rktVersion;
this.rktKind = v.rktKind;
this.registries = (v.registries == null) ? null : new ArrayList(v.registries);
this.credentials = v.credentials;
}
public String rktVersion() {
return rktVersion;
}
public DockerAuthBuilder rktVersion(String rktVersion) {
if (rktVersion == null) {
throw new NullPointerException("rktVersion");
}
this.rktVersion = rktVersion;
return this;
}
public String rktKind() {
return rktKind;
}
public DockerAuthBuilder rktKind(String rktKind) {
if (rktKind == null) {
throw new NullPointerException("rktKind");
}
this.rktKind = rktKind;
return this;
}
public List registries() {
if (this.registries == null) {
this.registries = new ArrayList();
}
return registries;
}
public DockerAuthBuilder registries(List extends String> registries) {
return registries((Collection extends String>) registries);
}
public DockerAuthBuilder registries(Collection extends String> registries) {
if (registries == null) {
throw new NullPointerException("registries");
}
for (String item : registries) {
if (item == null) {
throw new NullPointerException("registries: null item");
}
}
this.registries = new ArrayList(registries);
return this;
}
public DockerAuthBuilder registries(Iterable extends String> registries) {
if (registries == null) {
throw new NullPointerException("registries");
}
if (registries instanceof Collection) {
return registries((Collection extends String>) registries);
}
return registries(registries.iterator());
}
public DockerAuthBuilder registries(Iterator extends String> registries) {
if (registries == null) {
throw new NullPointerException("registries");
}
this.registries = new ArrayList();
while (registries.hasNext()) {
String item = registries.next();
if (item == null) {
throw new NullPointerException("registries: null item");
}
this.registries.add(item);
}
return this;
}
@SafeVarargs
public final DockerAuthBuilder registries(String... registries) {
if (registries == null) {
throw new NullPointerException("registries");
}
return registries(Arrays.asList(registries));
}
public DockerAuthBuilder addRegistry(String registry) {
if (registry == null) {
throw new NullPointerException("registry");
}
if (this.registries == null) {
this.registries = new ArrayList();
}
registries.add(registry);
return this;
}
public Stage0Entry.DockerAuth.BasicCredentials credentials() {
return credentials;
}
public DockerAuthBuilder credentials(Stage0Entry.DockerAuth.BasicCredentials credentials) {
if (credentials == null) {
throw new NullPointerException("credentials");
}
this.credentials = credentials;
return this;
}
public Stage0Entry.DockerAuth build() {
List _registries = (registries != null) ? Collections.unmodifiableList(new ArrayList(registries)) : Collections.emptyList();
return new Value(rktVersion, rktKind, _registries, credentials);
}
public static DockerAuthBuilder from(Stage0Entry.DockerAuth v) {
return new DockerAuthBuilder(v);
}
public static DockerAuthBuilder from(DockerAuthBuilder v) {
return new DockerAuthBuilder(v);
}
private static final class Value implements Stage0Entry.DockerAuth {
private final String rktVersion;
private final String rktKind;
private final List registries;
private final Stage0Entry.DockerAuth.BasicCredentials credentials;
private Value(@AutoMatter.Field("rktVersion") String rktVersion, @AutoMatter.Field("rktKind") String rktKind, @AutoMatter.Field("registries") List registries, @AutoMatter.Field("credentials") Stage0Entry.DockerAuth.BasicCredentials credentials) {
if (rktVersion == null) {
throw new NullPointerException("rktVersion");
}
if (rktKind == null) {
throw new NullPointerException("rktKind");
}
if (credentials == null) {
throw new NullPointerException("credentials");
}
this.rktVersion = rktVersion;
this.rktKind = rktKind;
this.registries = (registries != null) ? registries : Collections.emptyList();
this.credentials = credentials;
}
@AutoMatter.Field
@Override
public String rktVersion() {
return rktVersion;
}
@AutoMatter.Field
@Override
public String rktKind() {
return rktKind;
}
@AutoMatter.Field
@Override
public List registries() {
return registries;
}
@AutoMatter.Field
@Override
public Stage0Entry.DockerAuth.BasicCredentials credentials() {
return credentials;
}
public DockerAuthBuilder builder() {
return new DockerAuthBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof Stage0Entry.DockerAuth)) {
return false;
}
final Stage0Entry.DockerAuth that = (Stage0Entry.DockerAuth) o;
if (rktVersion != null ? !rktVersion.equals(that.rktVersion()) : that.rktVersion() != null) {
return false;
}
if (rktKind != null ? !rktKind.equals(that.rktKind()) : that.rktKind() != null) {
return false;
}
if (registries != null ? !registries.equals(that.registries()) : that.registries() != null) {
return false;
}
if (credentials != null ? !credentials.equals(that.credentials()) : that.credentials() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
long temp;
result = 31 * result + (rktVersion != null ? rktVersion.hashCode() : 0);
result = 31 * result + (rktKind != null ? rktKind.hashCode() : 0);
result = 31 * result + (registries != null ? registries.hashCode() : 0);
result = 31 * result + (credentials != null ? credentials.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "Stage0Entry.DockerAuth{" +
"rktVersion=" + rktVersion +
", rktKind=" + rktKind +
", registries=" + registries +
", credentials=" + credentials +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy