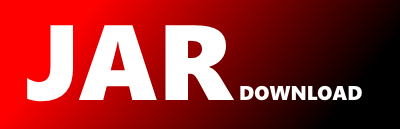
io.honnix.rkt.launcher.model.schema.RuntimeAppBuilder Maven / Gradle / Ivy
package io.honnix.rkt.launcher.model.schema;
import io.honnix.rkt.launcher.model.schema.type.Annotation;
import io.honnix.rkt.launcher.model.schema.type.App;
import io.honnix.rkt.launcher.model.schema.type.Mount;
import io.norberg.automatter.AutoMatter;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.util.Optional;
import javax.annotation.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
public final class RuntimeAppBuilder {
private String name;
private PodManifest.RuntimeImage image;
private Optional app;
private Optional readOnlyRootFS;
private List mounts;
private List annotations;
public RuntimeAppBuilder() {
this.app = Optional.empty();
this.readOnlyRootFS = Optional.empty();
}
private RuntimeAppBuilder(PodManifest.RuntimeApp v) {
this.name = v.name();
this.image = v.image();
this.app = v.app();
this.readOnlyRootFS = v.readOnlyRootFS();
List extends Mount> _mounts = v.mounts();
this.mounts = (_mounts == null) ? null : new ArrayList(_mounts);
List extends Annotation> _annotations = v.annotations();
this.annotations = (_annotations == null) ? null : new ArrayList(_annotations);
}
private RuntimeAppBuilder(RuntimeAppBuilder v) {
this.name = v.name;
this.image = v.image;
this.app = v.app;
this.readOnlyRootFS = v.readOnlyRootFS;
this.mounts = (v.mounts == null) ? null : new ArrayList(v.mounts);
this.annotations = (v.annotations == null) ? null : new ArrayList(v.annotations);
}
public String name() {
return name;
}
public RuntimeAppBuilder name(String name) {
if (name == null) {
throw new NullPointerException("name");
}
this.name = name;
return this;
}
public PodManifest.RuntimeImage image() {
return image;
}
public RuntimeAppBuilder image(PodManifest.RuntimeImage image) {
if (image == null) {
throw new NullPointerException("image");
}
this.image = image;
return this;
}
public Optional app() {
return app;
}
public RuntimeAppBuilder app(App app) {
return app(Optional.ofNullable(app));
}
@SuppressWarnings("unchecked")
public RuntimeAppBuilder app(Optional extends App> app) {
if (app == null) {
throw new NullPointerException("app");
}
this.app = (Optional)app;
return this;
}
public Optional readOnlyRootFS() {
return readOnlyRootFS;
}
public RuntimeAppBuilder readOnlyRootFS(Boolean readOnlyRootFS) {
return readOnlyRootFS(Optional.ofNullable(readOnlyRootFS));
}
@SuppressWarnings("unchecked")
public RuntimeAppBuilder readOnlyRootFS(Optional extends Boolean> readOnlyRootFS) {
if (readOnlyRootFS == null) {
throw new NullPointerException("readOnlyRootFS");
}
this.readOnlyRootFS = (Optional)readOnlyRootFS;
return this;
}
public List mounts() {
if (this.mounts == null) {
this.mounts = new ArrayList();
}
return mounts;
}
public RuntimeAppBuilder mounts(List extends Mount> mounts) {
return mounts((Collection extends Mount>) mounts);
}
public RuntimeAppBuilder mounts(Collection extends Mount> mounts) {
if (mounts == null) {
throw new NullPointerException("mounts");
}
for (Mount item : mounts) {
if (item == null) {
throw new NullPointerException("mounts: null item");
}
}
this.mounts = new ArrayList(mounts);
return this;
}
public RuntimeAppBuilder mounts(Iterable extends Mount> mounts) {
if (mounts == null) {
throw new NullPointerException("mounts");
}
if (mounts instanceof Collection) {
return mounts((Collection extends Mount>) mounts);
}
return mounts(mounts.iterator());
}
public RuntimeAppBuilder mounts(Iterator extends Mount> mounts) {
if (mounts == null) {
throw new NullPointerException("mounts");
}
this.mounts = new ArrayList();
while (mounts.hasNext()) {
Mount item = mounts.next();
if (item == null) {
throw new NullPointerException("mounts: null item");
}
this.mounts.add(item);
}
return this;
}
@SafeVarargs
public final RuntimeAppBuilder mounts(Mount... mounts) {
if (mounts == null) {
throw new NullPointerException("mounts");
}
return mounts(Arrays.asList(mounts));
}
public RuntimeAppBuilder addMount(Mount mount) {
if (mount == null) {
throw new NullPointerException("mount");
}
if (this.mounts == null) {
this.mounts = new ArrayList();
}
mounts.add(mount);
return this;
}
public List annotations() {
if (this.annotations == null) {
this.annotations = new ArrayList();
}
return annotations;
}
public RuntimeAppBuilder annotations(List extends Annotation> annotations) {
return annotations((Collection extends Annotation>) annotations);
}
public RuntimeAppBuilder annotations(Collection extends Annotation> annotations) {
if (annotations == null) {
throw new NullPointerException("annotations");
}
for (Annotation item : annotations) {
if (item == null) {
throw new NullPointerException("annotations: null item");
}
}
this.annotations = new ArrayList(annotations);
return this;
}
public RuntimeAppBuilder annotations(Iterable extends Annotation> annotations) {
if (annotations == null) {
throw new NullPointerException("annotations");
}
if (annotations instanceof Collection) {
return annotations((Collection extends Annotation>) annotations);
}
return annotations(annotations.iterator());
}
public RuntimeAppBuilder annotations(Iterator extends Annotation> annotations) {
if (annotations == null) {
throw new NullPointerException("annotations");
}
this.annotations = new ArrayList();
while (annotations.hasNext()) {
Annotation item = annotations.next();
if (item == null) {
throw new NullPointerException("annotations: null item");
}
this.annotations.add(item);
}
return this;
}
@SafeVarargs
public final RuntimeAppBuilder annotations(Annotation... annotations) {
if (annotations == null) {
throw new NullPointerException("annotations");
}
return annotations(Arrays.asList(annotations));
}
public RuntimeAppBuilder addAnnotation(Annotation annotation) {
if (annotation == null) {
throw new NullPointerException("annotation");
}
if (this.annotations == null) {
this.annotations = new ArrayList();
}
annotations.add(annotation);
return this;
}
public PodManifest.RuntimeApp build() {
List _mounts = (mounts != null) ? Collections.unmodifiableList(new ArrayList(mounts)) : Collections.emptyList();
List _annotations = (annotations != null) ? Collections.unmodifiableList(new ArrayList(annotations)) : Collections.emptyList();
return new Value(name, image, app, readOnlyRootFS, _mounts, _annotations);
}
public static RuntimeAppBuilder from(PodManifest.RuntimeApp v) {
return new RuntimeAppBuilder(v);
}
public static RuntimeAppBuilder from(RuntimeAppBuilder v) {
return new RuntimeAppBuilder(v);
}
private static final class Value implements PodManifest.RuntimeApp {
private final String name;
private final PodManifest.RuntimeImage image;
private final Optional app;
private final Optional readOnlyRootFS;
private final List mounts;
private final List annotations;
private Value(@AutoMatter.Field("name") String name, @AutoMatter.Field("image") PodManifest.RuntimeImage image, @AutoMatter.Field("app") Optional app, @AutoMatter.Field("readOnlyRootFS") Optional readOnlyRootFS, @AutoMatter.Field("mounts") List mounts, @AutoMatter.Field("annotations") List annotations) {
if (name == null) {
throw new NullPointerException("name");
}
if (image == null) {
throw new NullPointerException("image");
}
if (app == null) {
throw new NullPointerException("app");
}
if (readOnlyRootFS == null) {
throw new NullPointerException("readOnlyRootFS");
}
this.name = name;
this.image = image;
this.app = app;
this.readOnlyRootFS = readOnlyRootFS;
this.mounts = (mounts != null) ? mounts : Collections.emptyList();
this.annotations = (annotations != null) ? annotations : Collections.emptyList();
}
@AutoMatter.Field
@Override
public String name() {
return name;
}
@AutoMatter.Field
@Override
public PodManifest.RuntimeImage image() {
return image;
}
@AutoMatter.Field
@Override
public Optional app() {
return app;
}
@AutoMatter.Field
@Override
public Optional readOnlyRootFS() {
return readOnlyRootFS;
}
@AutoMatter.Field
@Override
public List mounts() {
return mounts;
}
@AutoMatter.Field
@Override
public List annotations() {
return annotations;
}
public RuntimeAppBuilder builder() {
return new RuntimeAppBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof PodManifest.RuntimeApp)) {
return false;
}
final PodManifest.RuntimeApp that = (PodManifest.RuntimeApp) o;
if (name != null ? !name.equals(that.name()) : that.name() != null) {
return false;
}
if (image != null ? !image.equals(that.image()) : that.image() != null) {
return false;
}
if (app != null ? !app.equals(that.app()) : that.app() != null) {
return false;
}
if (readOnlyRootFS != null ? !readOnlyRootFS.equals(that.readOnlyRootFS()) : that.readOnlyRootFS() != null) {
return false;
}
if (mounts != null ? !mounts.equals(that.mounts()) : that.mounts() != null) {
return false;
}
if (annotations != null ? !annotations.equals(that.annotations()) : that.annotations() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
long temp;
result = 31 * result + (name != null ? name.hashCode() : 0);
result = 31 * result + (image != null ? image.hashCode() : 0);
result = 31 * result + (app != null ? app.hashCode() : 0);
result = 31 * result + (readOnlyRootFS != null ? readOnlyRootFS.hashCode() : 0);
result = 31 * result + (mounts != null ? mounts.hashCode() : 0);
result = 31 * result + (annotations != null ? annotations.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "PodManifest.RuntimeApp{" +
"name=" + name +
", image=" + image +
", app=" + app +
", readOnlyRootFS=" + readOnlyRootFS +
", mounts=" + mounts +
", annotations=" + annotations +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy