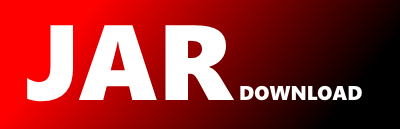
io.honnix.rkt.launcher.model.NetInfoBuilder Maven / Gradle / Ivy
package io.honnix.rkt.launcher.model;
import io.norberg.automatter.AutoMatter;
import javax.annotation.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
public final class NetInfoBuilder {
private String netName;
private String netConf;
private String pluginPath;
private String ifName;
private String ip;
private String args;
private String mask;
public NetInfoBuilder() {
}
private NetInfoBuilder(NetInfo v) {
this.netName = v.netName();
this.netConf = v.netConf();
this.pluginPath = v.pluginPath();
this.ifName = v.ifName();
this.ip = v.ip();
this.args = v.args();
this.mask = v.mask();
}
private NetInfoBuilder(NetInfoBuilder v) {
this.netName = v.netName;
this.netConf = v.netConf;
this.pluginPath = v.pluginPath;
this.ifName = v.ifName;
this.ip = v.ip;
this.args = v.args;
this.mask = v.mask;
}
public String netName() {
return netName;
}
public NetInfoBuilder netName(String netName) {
if (netName == null) {
throw new NullPointerException("netName");
}
this.netName = netName;
return this;
}
public String netConf() {
return netConf;
}
public NetInfoBuilder netConf(String netConf) {
if (netConf == null) {
throw new NullPointerException("netConf");
}
this.netConf = netConf;
return this;
}
public String pluginPath() {
return pluginPath;
}
public NetInfoBuilder pluginPath(String pluginPath) {
if (pluginPath == null) {
throw new NullPointerException("pluginPath");
}
this.pluginPath = pluginPath;
return this;
}
public String ifName() {
return ifName;
}
public NetInfoBuilder ifName(String ifName) {
if (ifName == null) {
throw new NullPointerException("ifName");
}
this.ifName = ifName;
return this;
}
public String ip() {
return ip;
}
public NetInfoBuilder ip(String ip) {
if (ip == null) {
throw new NullPointerException("ip");
}
this.ip = ip;
return this;
}
public String args() {
return args;
}
public NetInfoBuilder args(String args) {
if (args == null) {
throw new NullPointerException("args");
}
this.args = args;
return this;
}
public String mask() {
return mask;
}
public NetInfoBuilder mask(String mask) {
if (mask == null) {
throw new NullPointerException("mask");
}
this.mask = mask;
return this;
}
public NetInfo build() {
return new Value(netName, netConf, pluginPath, ifName, ip, args, mask);
}
public static NetInfoBuilder from(NetInfo v) {
return new NetInfoBuilder(v);
}
public static NetInfoBuilder from(NetInfoBuilder v) {
return new NetInfoBuilder(v);
}
private static final class Value implements NetInfo {
private final String netName;
private final String netConf;
private final String pluginPath;
private final String ifName;
private final String ip;
private final String args;
private final String mask;
private Value(@AutoMatter.Field("netName") String netName, @AutoMatter.Field("netConf") String netConf, @AutoMatter.Field("pluginPath") String pluginPath, @AutoMatter.Field("ifName") String ifName, @AutoMatter.Field("ip") String ip, @AutoMatter.Field("args") String args, @AutoMatter.Field("mask") String mask) {
if (netName == null) {
throw new NullPointerException("netName");
}
if (netConf == null) {
throw new NullPointerException("netConf");
}
if (pluginPath == null) {
throw new NullPointerException("pluginPath");
}
if (ifName == null) {
throw new NullPointerException("ifName");
}
if (ip == null) {
throw new NullPointerException("ip");
}
if (args == null) {
throw new NullPointerException("args");
}
if (mask == null) {
throw new NullPointerException("mask");
}
this.netName = netName;
this.netConf = netConf;
this.pluginPath = pluginPath;
this.ifName = ifName;
this.ip = ip;
this.args = args;
this.mask = mask;
}
@AutoMatter.Field
@Override
public String netName() {
return netName;
}
@AutoMatter.Field
@Override
public String netConf() {
return netConf;
}
@AutoMatter.Field
@Override
public String pluginPath() {
return pluginPath;
}
@AutoMatter.Field
@Override
public String ifName() {
return ifName;
}
@AutoMatter.Field
@Override
public String ip() {
return ip;
}
@AutoMatter.Field
@Override
public String args() {
return args;
}
@AutoMatter.Field
@Override
public String mask() {
return mask;
}
public NetInfoBuilder builder() {
return new NetInfoBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof NetInfo)) {
return false;
}
final NetInfo that = (NetInfo) o;
if (netName != null ? !netName.equals(that.netName()) : that.netName() != null) {
return false;
}
if (netConf != null ? !netConf.equals(that.netConf()) : that.netConf() != null) {
return false;
}
if (pluginPath != null ? !pluginPath.equals(that.pluginPath()) : that.pluginPath() != null) {
return false;
}
if (ifName != null ? !ifName.equals(that.ifName()) : that.ifName() != null) {
return false;
}
if (ip != null ? !ip.equals(that.ip()) : that.ip() != null) {
return false;
}
if (args != null ? !args.equals(that.args()) : that.args() != null) {
return false;
}
if (mask != null ? !mask.equals(that.mask()) : that.mask() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
long temp;
result = 31 * result + (netName != null ? netName.hashCode() : 0);
result = 31 * result + (netConf != null ? netConf.hashCode() : 0);
result = 31 * result + (pluginPath != null ? pluginPath.hashCode() : 0);
result = 31 * result + (ifName != null ? ifName.hashCode() : 0);
result = 31 * result + (ip != null ? ip.hashCode() : 0);
result = 31 * result + (args != null ? args.hashCode() : 0);
result = 31 * result + (mask != null ? mask.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "NetInfo{" +
"netName=" + netName +
", netConf=" + netConf +
", pluginPath=" + pluginPath +
", ifName=" + ifName +
", ip=" + ip +
", args=" + args +
", mask=" + mask +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy