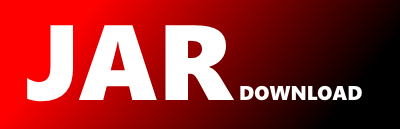
io.honnix.rkt.launcher.options.RunPreparedOptionsBuilder Maven / Gradle / Ivy
package io.honnix.rkt.launcher.options;
import io.honnix.rkt.launcher.model.Network;
import io.norberg.automatter.AutoMatter;
import java.util.List;
import java.util.Optional;
import javax.annotation.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
public final class RunPreparedOptionsBuilder {
private Optional> dns;
private Optional> dnsDomain;
private Optional> dnsOpt;
private Optional> dnsSearch;
private Optional hostname;
private Optional> hostsEntry;
private Optional mdsRegister;
private Optional> net;
public RunPreparedOptionsBuilder() {
this.dns = Optional.empty();
this.dnsDomain = Optional.empty();
this.dnsOpt = Optional.empty();
this.dnsSearch = Optional.empty();
this.hostname = Optional.empty();
this.hostsEntry = Optional.empty();
this.mdsRegister = Optional.empty();
this.net = Optional.empty();
}
private RunPreparedOptionsBuilder(RunPreparedOptions v) {
this.dns = v.dns();
this.dnsDomain = v.dnsDomain();
this.dnsOpt = v.dnsOpt();
this.dnsSearch = v.dnsSearch();
this.hostname = v.hostname();
this.hostsEntry = v.hostsEntry();
this.mdsRegister = v.mdsRegister();
this.net = v.net();
}
private RunPreparedOptionsBuilder(RunPreparedOptionsBuilder v) {
this.dns = v.dns;
this.dnsDomain = v.dnsDomain;
this.dnsOpt = v.dnsOpt;
this.dnsSearch = v.dnsSearch;
this.hostname = v.hostname;
this.hostsEntry = v.hostsEntry;
this.mdsRegister = v.mdsRegister;
this.net = v.net;
}
public Optional> dns() {
return dns;
}
public RunPreparedOptionsBuilder dns(List dns) {
return dns(Optional.ofNullable(dns));
}
@SuppressWarnings("unchecked")
public RunPreparedOptionsBuilder dns(Optional extends List> dns) {
if (dns == null) {
throw new NullPointerException("dns");
}
this.dns = (Optional>)dns;
return this;
}
public Optional> dnsDomain() {
return dnsDomain;
}
public RunPreparedOptionsBuilder dnsDomain(List dnsDomain) {
return dnsDomain(Optional.ofNullable(dnsDomain));
}
@SuppressWarnings("unchecked")
public RunPreparedOptionsBuilder dnsDomain(Optional extends List> dnsDomain) {
if (dnsDomain == null) {
throw new NullPointerException("dnsDomain");
}
this.dnsDomain = (Optional>)dnsDomain;
return this;
}
public Optional> dnsOpt() {
return dnsOpt;
}
public RunPreparedOptionsBuilder dnsOpt(List dnsOpt) {
return dnsOpt(Optional.ofNullable(dnsOpt));
}
@SuppressWarnings("unchecked")
public RunPreparedOptionsBuilder dnsOpt(Optional extends List> dnsOpt) {
if (dnsOpt == null) {
throw new NullPointerException("dnsOpt");
}
this.dnsOpt = (Optional>)dnsOpt;
return this;
}
public Optional> dnsSearch() {
return dnsSearch;
}
public RunPreparedOptionsBuilder dnsSearch(List dnsSearch) {
return dnsSearch(Optional.ofNullable(dnsSearch));
}
@SuppressWarnings("unchecked")
public RunPreparedOptionsBuilder dnsSearch(Optional extends List> dnsSearch) {
if (dnsSearch == null) {
throw new NullPointerException("dnsSearch");
}
this.dnsSearch = (Optional>)dnsSearch;
return this;
}
public Optional hostname() {
return hostname;
}
public RunPreparedOptionsBuilder hostname(String hostname) {
return hostname(Optional.ofNullable(hostname));
}
@SuppressWarnings("unchecked")
public RunPreparedOptionsBuilder hostname(Optional extends String> hostname) {
if (hostname == null) {
throw new NullPointerException("hostname");
}
this.hostname = (Optional)hostname;
return this;
}
public Optional> hostsEntry() {
return hostsEntry;
}
public RunPreparedOptionsBuilder hostsEntry(List hostsEntry) {
return hostsEntry(Optional.ofNullable(hostsEntry));
}
@SuppressWarnings("unchecked")
public RunPreparedOptionsBuilder hostsEntry(Optional extends List> hostsEntry) {
if (hostsEntry == null) {
throw new NullPointerException("hostsEntry");
}
this.hostsEntry = (Optional>)hostsEntry;
return this;
}
public Optional mdsRegister() {
return mdsRegister;
}
public RunPreparedOptionsBuilder mdsRegister(Boolean mdsRegister) {
return mdsRegister(Optional.ofNullable(mdsRegister));
}
@SuppressWarnings("unchecked")
public RunPreparedOptionsBuilder mdsRegister(Optional extends Boolean> mdsRegister) {
if (mdsRegister == null) {
throw new NullPointerException("mdsRegister");
}
this.mdsRegister = (Optional)mdsRegister;
return this;
}
public Optional> net() {
return net;
}
public RunPreparedOptionsBuilder net(List net) {
return net(Optional.ofNullable(net));
}
@SuppressWarnings("unchecked")
public RunPreparedOptionsBuilder net(Optional extends List> net) {
if (net == null) {
throw new NullPointerException("net");
}
this.net = (Optional>)net;
return this;
}
public RunPreparedOptions build() {
return new Value(dns, dnsDomain, dnsOpt, dnsSearch, hostname, hostsEntry, mdsRegister, net);
}
public static RunPreparedOptionsBuilder from(RunPreparedOptions v) {
return new RunPreparedOptionsBuilder(v);
}
public static RunPreparedOptionsBuilder from(RunPreparedOptionsBuilder v) {
return new RunPreparedOptionsBuilder(v);
}
private static final class Value implements RunPreparedOptions {
private final Optional> dns;
private final Optional> dnsDomain;
private final Optional> dnsOpt;
private final Optional> dnsSearch;
private final Optional hostname;
private final Optional> hostsEntry;
private final Optional mdsRegister;
private final Optional> net;
private Value(@AutoMatter.Field("dns") Optional> dns, @AutoMatter.Field("dnsDomain") Optional> dnsDomain, @AutoMatter.Field("dnsOpt") Optional> dnsOpt, @AutoMatter.Field("dnsSearch") Optional> dnsSearch, @AutoMatter.Field("hostname") Optional hostname, @AutoMatter.Field("hostsEntry") Optional> hostsEntry, @AutoMatter.Field("mdsRegister") Optional mdsRegister, @AutoMatter.Field("net") Optional> net) {
if (dns == null) {
throw new NullPointerException("dns");
}
if (dnsDomain == null) {
throw new NullPointerException("dnsDomain");
}
if (dnsOpt == null) {
throw new NullPointerException("dnsOpt");
}
if (dnsSearch == null) {
throw new NullPointerException("dnsSearch");
}
if (hostname == null) {
throw new NullPointerException("hostname");
}
if (hostsEntry == null) {
throw new NullPointerException("hostsEntry");
}
if (mdsRegister == null) {
throw new NullPointerException("mdsRegister");
}
if (net == null) {
throw new NullPointerException("net");
}
this.dns = dns;
this.dnsDomain = dnsDomain;
this.dnsOpt = dnsOpt;
this.dnsSearch = dnsSearch;
this.hostname = hostname;
this.hostsEntry = hostsEntry;
this.mdsRegister = mdsRegister;
this.net = net;
}
@AutoMatter.Field
@Override
public Optional> dns() {
return dns;
}
@AutoMatter.Field
@Override
public Optional> dnsDomain() {
return dnsDomain;
}
@AutoMatter.Field
@Override
public Optional> dnsOpt() {
return dnsOpt;
}
@AutoMatter.Field
@Override
public Optional> dnsSearch() {
return dnsSearch;
}
@AutoMatter.Field
@Override
public Optional hostname() {
return hostname;
}
@AutoMatter.Field
@Override
public Optional> hostsEntry() {
return hostsEntry;
}
@AutoMatter.Field
@Override
public Optional mdsRegister() {
return mdsRegister;
}
@AutoMatter.Field
@Override
public Optional> net() {
return net;
}
public RunPreparedOptionsBuilder builder() {
return new RunPreparedOptionsBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof RunPreparedOptions)) {
return false;
}
final RunPreparedOptions that = (RunPreparedOptions) o;
if (dns != null ? !dns.equals(that.dns()) : that.dns() != null) {
return false;
}
if (dnsDomain != null ? !dnsDomain.equals(that.dnsDomain()) : that.dnsDomain() != null) {
return false;
}
if (dnsOpt != null ? !dnsOpt.equals(that.dnsOpt()) : that.dnsOpt() != null) {
return false;
}
if (dnsSearch != null ? !dnsSearch.equals(that.dnsSearch()) : that.dnsSearch() != null) {
return false;
}
if (hostname != null ? !hostname.equals(that.hostname()) : that.hostname() != null) {
return false;
}
if (hostsEntry != null ? !hostsEntry.equals(that.hostsEntry()) : that.hostsEntry() != null) {
return false;
}
if (mdsRegister != null ? !mdsRegister.equals(that.mdsRegister()) : that.mdsRegister() != null) {
return false;
}
if (net != null ? !net.equals(that.net()) : that.net() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
long temp;
result = 31 * result + (dns != null ? dns.hashCode() : 0);
result = 31 * result + (dnsDomain != null ? dnsDomain.hashCode() : 0);
result = 31 * result + (dnsOpt != null ? dnsOpt.hashCode() : 0);
result = 31 * result + (dnsSearch != null ? dnsSearch.hashCode() : 0);
result = 31 * result + (hostname != null ? hostname.hashCode() : 0);
result = 31 * result + (hostsEntry != null ? hostsEntry.hashCode() : 0);
result = 31 * result + (mdsRegister != null ? mdsRegister.hashCode() : 0);
result = 31 * result + (net != null ? net.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "RunPreparedOptions{" +
"dns=" + dns +
", dnsDomain=" + dnsDomain +
", dnsOpt=" + dnsOpt +
", dnsSearch=" + dnsSearch +
", hostname=" + hostname +
", hostsEntry=" + hostsEntry +
", mdsRegister=" + mdsRegister +
", net=" + net +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy