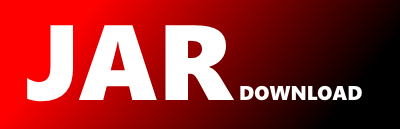
io.honnix.rkt.launcher.output.VersionOutputBuilder Maven / Gradle / Ivy
package io.honnix.rkt.launcher.output;
import io.norberg.automatter.AutoMatter;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import javax.annotation.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
public final class VersionOutputBuilder {
private String rktVersion;
private String appcVersion;
private String goVersion;
private String goOSArch;
private List features;
public VersionOutputBuilder() {
}
private VersionOutputBuilder(VersionOutput v) {
this.rktVersion = v.rktVersion();
this.appcVersion = v.appcVersion();
this.goVersion = v.goVersion();
this.goOSArch = v.goOSArch();
List extends String> _features = v.features();
this.features = (_features == null) ? null : new ArrayList(_features);
}
private VersionOutputBuilder(VersionOutputBuilder v) {
this.rktVersion = v.rktVersion;
this.appcVersion = v.appcVersion;
this.goVersion = v.goVersion;
this.goOSArch = v.goOSArch;
this.features = (v.features == null) ? null : new ArrayList(v.features);
}
public String rktVersion() {
return rktVersion;
}
public VersionOutputBuilder rktVersion(String rktVersion) {
if (rktVersion == null) {
throw new NullPointerException("rktVersion");
}
this.rktVersion = rktVersion;
return this;
}
public String appcVersion() {
return appcVersion;
}
public VersionOutputBuilder appcVersion(String appcVersion) {
if (appcVersion == null) {
throw new NullPointerException("appcVersion");
}
this.appcVersion = appcVersion;
return this;
}
public String goVersion() {
return goVersion;
}
public VersionOutputBuilder goVersion(String goVersion) {
if (goVersion == null) {
throw new NullPointerException("goVersion");
}
this.goVersion = goVersion;
return this;
}
public String goOSArch() {
return goOSArch;
}
public VersionOutputBuilder goOSArch(String goOSArch) {
if (goOSArch == null) {
throw new NullPointerException("goOSArch");
}
this.goOSArch = goOSArch;
return this;
}
public List features() {
if (this.features == null) {
this.features = new ArrayList();
}
return features;
}
public VersionOutputBuilder features(List extends String> features) {
return features((Collection extends String>) features);
}
public VersionOutputBuilder features(Collection extends String> features) {
if (features == null) {
throw new NullPointerException("features");
}
for (String item : features) {
if (item == null) {
throw new NullPointerException("features: null item");
}
}
this.features = new ArrayList(features);
return this;
}
public VersionOutputBuilder features(Iterable extends String> features) {
if (features == null) {
throw new NullPointerException("features");
}
if (features instanceof Collection) {
return features((Collection extends String>) features);
}
return features(features.iterator());
}
public VersionOutputBuilder features(Iterator extends String> features) {
if (features == null) {
throw new NullPointerException("features");
}
this.features = new ArrayList();
while (features.hasNext()) {
String item = features.next();
if (item == null) {
throw new NullPointerException("features: null item");
}
this.features.add(item);
}
return this;
}
@SafeVarargs
public final VersionOutputBuilder features(String... features) {
if (features == null) {
throw new NullPointerException("features");
}
return features(Arrays.asList(features));
}
public VersionOutputBuilder addFeature(String feature) {
if (feature == null) {
throw new NullPointerException("feature");
}
if (this.features == null) {
this.features = new ArrayList();
}
features.add(feature);
return this;
}
public VersionOutput build() {
List _features = (features != null) ? Collections.unmodifiableList(new ArrayList(features)) : Collections.emptyList();
return new Value(rktVersion, appcVersion, goVersion, goOSArch, _features);
}
public static VersionOutputBuilder from(VersionOutput v) {
return new VersionOutputBuilder(v);
}
public static VersionOutputBuilder from(VersionOutputBuilder v) {
return new VersionOutputBuilder(v);
}
private static final class Value implements VersionOutput {
private final String rktVersion;
private final String appcVersion;
private final String goVersion;
private final String goOSArch;
private final List features;
private Value(@AutoMatter.Field("rktVersion") String rktVersion, @AutoMatter.Field("appcVersion") String appcVersion, @AutoMatter.Field("goVersion") String goVersion, @AutoMatter.Field("goOSArch") String goOSArch, @AutoMatter.Field("features") List features) {
if (rktVersion == null) {
throw new NullPointerException("rktVersion");
}
if (appcVersion == null) {
throw new NullPointerException("appcVersion");
}
if (goVersion == null) {
throw new NullPointerException("goVersion");
}
if (goOSArch == null) {
throw new NullPointerException("goOSArch");
}
this.rktVersion = rktVersion;
this.appcVersion = appcVersion;
this.goVersion = goVersion;
this.goOSArch = goOSArch;
this.features = (features != null) ? features : Collections.emptyList();
}
@AutoMatter.Field
@Override
public String rktVersion() {
return rktVersion;
}
@AutoMatter.Field
@Override
public String appcVersion() {
return appcVersion;
}
@AutoMatter.Field
@Override
public String goVersion() {
return goVersion;
}
@AutoMatter.Field
@Override
public String goOSArch() {
return goOSArch;
}
@AutoMatter.Field
@Override
public List features() {
return features;
}
public VersionOutputBuilder builder() {
return new VersionOutputBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof VersionOutput)) {
return false;
}
final VersionOutput that = (VersionOutput) o;
if (rktVersion != null ? !rktVersion.equals(that.rktVersion()) : that.rktVersion() != null) {
return false;
}
if (appcVersion != null ? !appcVersion.equals(that.appcVersion()) : that.appcVersion() != null) {
return false;
}
if (goVersion != null ? !goVersion.equals(that.goVersion()) : that.goVersion() != null) {
return false;
}
if (goOSArch != null ? !goOSArch.equals(that.goOSArch()) : that.goOSArch() != null) {
return false;
}
if (features != null ? !features.equals(that.features()) : that.features() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
long temp;
result = 31 * result + (rktVersion != null ? rktVersion.hashCode() : 0);
result = 31 * result + (appcVersion != null ? appcVersion.hashCode() : 0);
result = 31 * result + (goVersion != null ? goVersion.hashCode() : 0);
result = 31 * result + (goOSArch != null ? goOSArch.hashCode() : 0);
result = 31 * result + (features != null ? features.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "VersionOutput{" +
"rktVersion=" + rktVersion +
", appcVersion=" + appcVersion +
", goVersion=" + goVersion +
", goOSArch=" + goOSArch +
", features=" + features +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy