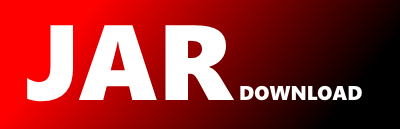
io.honnix.rkt.launcher.model.TrustedPubkeyBuilder Maven / Gradle / Ivy
package io.honnix.rkt.launcher.model;
import io.norberg.automatter.AutoMatter;
import javax.annotation.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
public final class TrustedPubkeyBuilder {
private String prefix;
private String key;
private String location;
public TrustedPubkeyBuilder() {
}
private TrustedPubkeyBuilder(TrustedPubkey v) {
this.prefix = v.prefix();
this.key = v.key();
this.location = v.location();
}
private TrustedPubkeyBuilder(TrustedPubkeyBuilder v) {
this.prefix = v.prefix;
this.key = v.key;
this.location = v.location;
}
public String prefix() {
return prefix;
}
public TrustedPubkeyBuilder prefix(String prefix) {
if (prefix == null) {
throw new NullPointerException("prefix");
}
this.prefix = prefix;
return this;
}
public String key() {
return key;
}
public TrustedPubkeyBuilder key(String key) {
if (key == null) {
throw new NullPointerException("key");
}
this.key = key;
return this;
}
public String location() {
return location;
}
public TrustedPubkeyBuilder location(String location) {
if (location == null) {
throw new NullPointerException("location");
}
this.location = location;
return this;
}
public TrustedPubkey build() {
return new Value(prefix, key, location);
}
public static TrustedPubkeyBuilder from(TrustedPubkey v) {
return new TrustedPubkeyBuilder(v);
}
public static TrustedPubkeyBuilder from(TrustedPubkeyBuilder v) {
return new TrustedPubkeyBuilder(v);
}
private static final class Value implements TrustedPubkey {
private final String prefix;
private final String key;
private final String location;
private Value(@AutoMatter.Field("prefix") String prefix, @AutoMatter.Field("key") String key, @AutoMatter.Field("location") String location) {
if (prefix == null) {
throw new NullPointerException("prefix");
}
if (key == null) {
throw new NullPointerException("key");
}
if (location == null) {
throw new NullPointerException("location");
}
this.prefix = prefix;
this.key = key;
this.location = location;
}
@AutoMatter.Field
@Override
public String prefix() {
return prefix;
}
@AutoMatter.Field
@Override
public String key() {
return key;
}
@AutoMatter.Field
@Override
public String location() {
return location;
}
public TrustedPubkeyBuilder builder() {
return new TrustedPubkeyBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof TrustedPubkey)) {
return false;
}
final TrustedPubkey that = (TrustedPubkey) o;
if (prefix != null ? !prefix.equals(that.prefix()) : that.prefix() != null) {
return false;
}
if (key != null ? !key.equals(that.key()) : that.key() != null) {
return false;
}
if (location != null ? !location.equals(that.location()) : that.location() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
long temp;
result = 31 * result + (prefix != null ? prefix.hashCode() : 0);
result = 31 * result + (key != null ? key.hashCode() : 0);
result = 31 * result + (location != null ? location.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "TrustedPubkey{" +
"prefix=" + prefix +
", key=" + key +
", location=" + location +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy