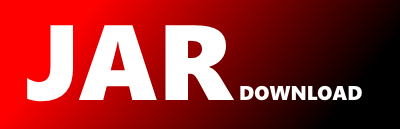
io.honnix.rkt.launcher.model.config.ConfigBuilder Maven / Gradle / Ivy
package io.honnix.rkt.launcher.model.config;
import io.norberg.automatter.AutoMatter;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.util.Optional;
import javax.annotation.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
public final class ConfigBuilder {
private List stage0;
private Optional> stage1;
public ConfigBuilder() {
this.stage1 = Optional.empty();
}
private ConfigBuilder(Config v) {
List extends Stage0Entry> _stage0 = v.stage0();
this.stage0 = (_stage0 == null) ? null : new ArrayList(_stage0);
this.stage1 = v.stage1();
}
private ConfigBuilder(ConfigBuilder v) {
this.stage0 = (v.stage0 == null) ? null : new ArrayList(v.stage0);
this.stage1 = v.stage1;
}
public List stage0() {
if (this.stage0 == null) {
this.stage0 = new ArrayList();
}
return stage0;
}
public ConfigBuilder stage0(List extends Stage0Entry> stage0) {
return stage0((Collection extends Stage0Entry>) stage0);
}
public ConfigBuilder stage0(Collection extends Stage0Entry> stage0) {
if (stage0 == null) {
throw new NullPointerException("stage0");
}
for (Stage0Entry item : stage0) {
if (item == null) {
throw new NullPointerException("stage0: null item");
}
}
this.stage0 = new ArrayList(stage0);
return this;
}
public ConfigBuilder stage0(Iterable extends Stage0Entry> stage0) {
if (stage0 == null) {
throw new NullPointerException("stage0");
}
if (stage0 instanceof Collection) {
return stage0((Collection extends Stage0Entry>) stage0);
}
return stage0(stage0.iterator());
}
public ConfigBuilder stage0(Iterator extends Stage0Entry> stage0) {
if (stage0 == null) {
throw new NullPointerException("stage0");
}
this.stage0 = new ArrayList();
while (stage0.hasNext()) {
Stage0Entry item = stage0.next();
if (item == null) {
throw new NullPointerException("stage0: null item");
}
this.stage0.add(item);
}
return this;
}
@SafeVarargs
public final ConfigBuilder stage0(Stage0Entry... stage0) {
if (stage0 == null) {
throw new NullPointerException("stage0");
}
return stage0(Arrays.asList(stage0));
}
public Optional> stage1() {
return stage1;
}
public ConfigBuilder stage1(List stage1) {
return stage1(Optional.ofNullable(stage1));
}
@SuppressWarnings("unchecked")
public ConfigBuilder stage1(Optional extends List> stage1) {
if (stage1 == null) {
throw new NullPointerException("stage1");
}
this.stage1 = (Optional>)stage1;
return this;
}
public Config build() {
List _stage0 = (stage0 != null) ? Collections.unmodifiableList(new ArrayList(stage0)) : Collections.emptyList();
return new Value(_stage0, stage1);
}
public static ConfigBuilder from(Config v) {
return new ConfigBuilder(v);
}
public static ConfigBuilder from(ConfigBuilder v) {
return new ConfigBuilder(v);
}
private static final class Value implements Config {
private final List stage0;
private final Optional> stage1;
private Value(@AutoMatter.Field("stage0") List stage0, @AutoMatter.Field("stage1") Optional> stage1) {
if (stage1 == null) {
throw new NullPointerException("stage1");
}
this.stage0 = (stage0 != null) ? stage0 : Collections.emptyList();
this.stage1 = stage1;
}
@AutoMatter.Field
@Override
public List stage0() {
return stage0;
}
@AutoMatter.Field
@Override
public Optional> stage1() {
return stage1;
}
public ConfigBuilder builder() {
return new ConfigBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof Config)) {
return false;
}
final Config that = (Config) o;
if (stage0 != null ? !stage0.equals(that.stage0()) : that.stage0() != null) {
return false;
}
if (stage1 != null ? !stage1.equals(that.stage1()) : that.stage1() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
long temp;
result = 31 * result + (stage0 != null ? stage0.hashCode() : 0);
result = 31 * result + (stage1 != null ? stage1.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "Config{" +
"stage0=" + stage0 +
", stage1=" + stage1 +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy