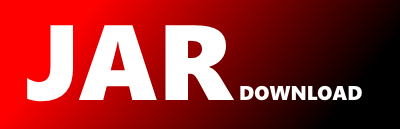
io.honnix.rkt.launcher.options.SeccompBuilder Maven / Gradle / Ivy
package io.honnix.rkt.launcher.options;
import io.norberg.automatter.AutoMatter;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import javax.annotation.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
public final class SeccompBuilder {
private PerImageOptions.Seccomp.Mode mode;
private String errno;
private List syscalls;
public SeccompBuilder() {
}
private SeccompBuilder(PerImageOptions.Seccomp v) {
this.mode = v.mode();
this.errno = v.errno();
List extends String> _syscalls = v.syscalls();
this.syscalls = (_syscalls == null) ? null : new ArrayList(_syscalls);
}
private SeccompBuilder(SeccompBuilder v) {
this.mode = v.mode;
this.errno = v.errno;
this.syscalls = (v.syscalls == null) ? null : new ArrayList(v.syscalls);
}
public PerImageOptions.Seccomp.Mode mode() {
return mode;
}
public SeccompBuilder mode(PerImageOptions.Seccomp.Mode mode) {
if (mode == null) {
throw new NullPointerException("mode");
}
this.mode = mode;
return this;
}
public String errno() {
return errno;
}
public SeccompBuilder errno(String errno) {
if (errno == null) {
throw new NullPointerException("errno");
}
this.errno = errno;
return this;
}
public List syscalls() {
if (this.syscalls == null) {
this.syscalls = new ArrayList();
}
return syscalls;
}
public SeccompBuilder syscalls(List extends String> syscalls) {
return syscalls((Collection extends String>) syscalls);
}
public SeccompBuilder syscalls(Collection extends String> syscalls) {
if (syscalls == null) {
throw new NullPointerException("syscalls");
}
for (String item : syscalls) {
if (item == null) {
throw new NullPointerException("syscalls: null item");
}
}
this.syscalls = new ArrayList(syscalls);
return this;
}
public SeccompBuilder syscalls(Iterable extends String> syscalls) {
if (syscalls == null) {
throw new NullPointerException("syscalls");
}
if (syscalls instanceof Collection) {
return syscalls((Collection extends String>) syscalls);
}
return syscalls(syscalls.iterator());
}
public SeccompBuilder syscalls(Iterator extends String> syscalls) {
if (syscalls == null) {
throw new NullPointerException("syscalls");
}
this.syscalls = new ArrayList();
while (syscalls.hasNext()) {
String item = syscalls.next();
if (item == null) {
throw new NullPointerException("syscalls: null item");
}
this.syscalls.add(item);
}
return this;
}
@SafeVarargs
public final SeccompBuilder syscalls(String... syscalls) {
if (syscalls == null) {
throw new NullPointerException("syscalls");
}
return syscalls(Arrays.asList(syscalls));
}
public SeccompBuilder addSyscall(String syscall) {
if (syscall == null) {
throw new NullPointerException("syscall");
}
if (this.syscalls == null) {
this.syscalls = new ArrayList();
}
syscalls.add(syscall);
return this;
}
public PerImageOptions.Seccomp build() {
List _syscalls = (syscalls != null) ? Collections.unmodifiableList(new ArrayList(syscalls)) : Collections.emptyList();
return new Value(mode, errno, _syscalls);
}
public static SeccompBuilder from(PerImageOptions.Seccomp v) {
return new SeccompBuilder(v);
}
public static SeccompBuilder from(SeccompBuilder v) {
return new SeccompBuilder(v);
}
private static final class Value implements PerImageOptions.Seccomp {
private final PerImageOptions.Seccomp.Mode mode;
private final String errno;
private final List syscalls;
private Value(@AutoMatter.Field("mode") PerImageOptions.Seccomp.Mode mode, @AutoMatter.Field("errno") String errno, @AutoMatter.Field("syscalls") List syscalls) {
if (mode == null) {
throw new NullPointerException("mode");
}
if (errno == null) {
throw new NullPointerException("errno");
}
this.mode = mode;
this.errno = errno;
this.syscalls = (syscalls != null) ? syscalls : Collections.emptyList();
}
@AutoMatter.Field
@Override
public PerImageOptions.Seccomp.Mode mode() {
return mode;
}
@AutoMatter.Field
@Override
public String errno() {
return errno;
}
@AutoMatter.Field
@Override
public List syscalls() {
return syscalls;
}
public SeccompBuilder builder() {
return new SeccompBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof PerImageOptions.Seccomp)) {
return false;
}
final PerImageOptions.Seccomp that = (PerImageOptions.Seccomp) o;
if (mode != null ? !mode.equals(that.mode()) : that.mode() != null) {
return false;
}
if (errno != null ? !errno.equals(that.errno()) : that.errno() != null) {
return false;
}
if (syscalls != null ? !syscalls.equals(that.syscalls()) : that.syscalls() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
long temp;
result = 31 * result + (mode != null ? mode.hashCode() : 0);
result = 31 * result + (errno != null ? errno.hashCode() : 0);
result = 31 * result + (syscalls != null ? syscalls.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "PerImageOptions.Seccomp{" +
"mode=" + mode +
", errno=" + errno +
", syscalls=" + syscalls +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy