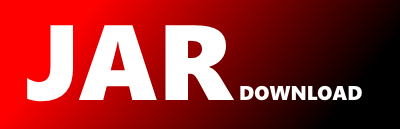
io.honnix.rkt.launcher.options.TrustOptionsBuilder Maven / Gradle / Ivy
package io.honnix.rkt.launcher.options;
import io.norberg.automatter.AutoMatter;
import java.util.Optional;
import javax.annotation.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
public final class TrustOptionsBuilder {
private Optional insecureAllowHttp;
private Optional prefix;
private Optional root;
public TrustOptionsBuilder() {
this.insecureAllowHttp = Optional.empty();
this.prefix = Optional.empty();
this.root = Optional.empty();
}
private TrustOptionsBuilder(TrustOptions v) {
this.insecureAllowHttp = v.insecureAllowHttp();
this.prefix = v.prefix();
this.root = v.root();
}
private TrustOptionsBuilder(TrustOptionsBuilder v) {
this.insecureAllowHttp = v.insecureAllowHttp;
this.prefix = v.prefix;
this.root = v.root;
}
public Optional insecureAllowHttp() {
return insecureAllowHttp;
}
public TrustOptionsBuilder insecureAllowHttp(Boolean insecureAllowHttp) {
return insecureAllowHttp(Optional.ofNullable(insecureAllowHttp));
}
@SuppressWarnings("unchecked")
public TrustOptionsBuilder insecureAllowHttp(Optional extends Boolean> insecureAllowHttp) {
if (insecureAllowHttp == null) {
throw new NullPointerException("insecureAllowHttp");
}
this.insecureAllowHttp = (Optional)insecureAllowHttp;
return this;
}
public Optional prefix() {
return prefix;
}
public TrustOptionsBuilder prefix(String prefix) {
return prefix(Optional.ofNullable(prefix));
}
@SuppressWarnings("unchecked")
public TrustOptionsBuilder prefix(Optional extends String> prefix) {
if (prefix == null) {
throw new NullPointerException("prefix");
}
this.prefix = (Optional)prefix;
return this;
}
public Optional root() {
return root;
}
public TrustOptionsBuilder root(Boolean root) {
return root(Optional.ofNullable(root));
}
@SuppressWarnings("unchecked")
public TrustOptionsBuilder root(Optional extends Boolean> root) {
if (root == null) {
throw new NullPointerException("root");
}
this.root = (Optional)root;
return this;
}
public TrustOptions build() {
return new Value(insecureAllowHttp, prefix, root);
}
public static TrustOptionsBuilder from(TrustOptions v) {
return new TrustOptionsBuilder(v);
}
public static TrustOptionsBuilder from(TrustOptionsBuilder v) {
return new TrustOptionsBuilder(v);
}
private static final class Value implements TrustOptions {
private final Optional insecureAllowHttp;
private final Optional prefix;
private final Optional root;
private Value(@AutoMatter.Field("insecureAllowHttp") Optional insecureAllowHttp, @AutoMatter.Field("prefix") Optional prefix, @AutoMatter.Field("root") Optional root) {
if (insecureAllowHttp == null) {
throw new NullPointerException("insecureAllowHttp");
}
if (prefix == null) {
throw new NullPointerException("prefix");
}
if (root == null) {
throw new NullPointerException("root");
}
this.insecureAllowHttp = insecureAllowHttp;
this.prefix = prefix;
this.root = root;
}
@AutoMatter.Field
@Override
public Optional insecureAllowHttp() {
return insecureAllowHttp;
}
@AutoMatter.Field
@Override
public Optional prefix() {
return prefix;
}
@AutoMatter.Field
@Override
public Optional root() {
return root;
}
public TrustOptionsBuilder builder() {
return new TrustOptionsBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof TrustOptions)) {
return false;
}
final TrustOptions that = (TrustOptions) o;
if (insecureAllowHttp != null ? !insecureAllowHttp.equals(that.insecureAllowHttp()) : that.insecureAllowHttp() != null) {
return false;
}
if (prefix != null ? !prefix.equals(that.prefix()) : that.prefix() != null) {
return false;
}
if (root != null ? !root.equals(that.root()) : that.root() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
long temp;
result = 31 * result + (insecureAllowHttp != null ? insecureAllowHttp.hashCode() : 0);
result = 31 * result + (prefix != null ? prefix.hashCode() : 0);
result = 31 * result + (root != null ? root.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "TrustOptions{" +
"insecureAllowHttp=" + insecureAllowHttp +
", prefix=" + prefix +
", root=" + root +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy