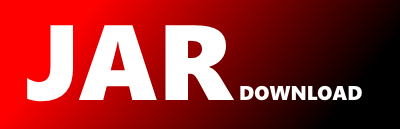
io.honnix.rkt.launcher.output.GcOutputBuilder Maven / Gradle / Ivy
package io.honnix.rkt.launcher.output;
import io.norberg.automatter.AutoMatter;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import javax.annotation.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
public final class GcOutputBuilder {
private List marked;
private List removed;
private List unremoved;
public GcOutputBuilder() {
}
private GcOutputBuilder(GcOutput v) {
List extends String> _marked = v.marked();
this.marked = (_marked == null) ? null : new ArrayList(_marked);
List extends String> _removed = v.removed();
this.removed = (_removed == null) ? null : new ArrayList(_removed);
List extends String> _unremoved = v.unremoved();
this.unremoved = (_unremoved == null) ? null : new ArrayList(_unremoved);
}
private GcOutputBuilder(GcOutputBuilder v) {
this.marked = (v.marked == null) ? null : new ArrayList(v.marked);
this.removed = (v.removed == null) ? null : new ArrayList(v.removed);
this.unremoved = (v.unremoved == null) ? null : new ArrayList(v.unremoved);
}
public List marked() {
if (this.marked == null) {
this.marked = new ArrayList();
}
return marked;
}
public GcOutputBuilder marked(List extends String> marked) {
return marked((Collection extends String>) marked);
}
public GcOutputBuilder marked(Collection extends String> marked) {
if (marked == null) {
throw new NullPointerException("marked");
}
for (String item : marked) {
if (item == null) {
throw new NullPointerException("marked: null item");
}
}
this.marked = new ArrayList(marked);
return this;
}
public GcOutputBuilder marked(Iterable extends String> marked) {
if (marked == null) {
throw new NullPointerException("marked");
}
if (marked instanceof Collection) {
return marked((Collection extends String>) marked);
}
return marked(marked.iterator());
}
public GcOutputBuilder marked(Iterator extends String> marked) {
if (marked == null) {
throw new NullPointerException("marked");
}
this.marked = new ArrayList();
while (marked.hasNext()) {
String item = marked.next();
if (item == null) {
throw new NullPointerException("marked: null item");
}
this.marked.add(item);
}
return this;
}
@SafeVarargs
public final GcOutputBuilder marked(String... marked) {
if (marked == null) {
throw new NullPointerException("marked");
}
return marked(Arrays.asList(marked));
}
public List removed() {
if (this.removed == null) {
this.removed = new ArrayList();
}
return removed;
}
public GcOutputBuilder removed(List extends String> removed) {
return removed((Collection extends String>) removed);
}
public GcOutputBuilder removed(Collection extends String> removed) {
if (removed == null) {
throw new NullPointerException("removed");
}
for (String item : removed) {
if (item == null) {
throw new NullPointerException("removed: null item");
}
}
this.removed = new ArrayList(removed);
return this;
}
public GcOutputBuilder removed(Iterable extends String> removed) {
if (removed == null) {
throw new NullPointerException("removed");
}
if (removed instanceof Collection) {
return removed((Collection extends String>) removed);
}
return removed(removed.iterator());
}
public GcOutputBuilder removed(Iterator extends String> removed) {
if (removed == null) {
throw new NullPointerException("removed");
}
this.removed = new ArrayList();
while (removed.hasNext()) {
String item = removed.next();
if (item == null) {
throw new NullPointerException("removed: null item");
}
this.removed.add(item);
}
return this;
}
@SafeVarargs
public final GcOutputBuilder removed(String... removed) {
if (removed == null) {
throw new NullPointerException("removed");
}
return removed(Arrays.asList(removed));
}
public List unremoved() {
if (this.unremoved == null) {
this.unremoved = new ArrayList();
}
return unremoved;
}
public GcOutputBuilder unremoved(List extends String> unremoved) {
return unremoved((Collection extends String>) unremoved);
}
public GcOutputBuilder unremoved(Collection extends String> unremoved) {
if (unremoved == null) {
throw new NullPointerException("unremoved");
}
for (String item : unremoved) {
if (item == null) {
throw new NullPointerException("unremoved: null item");
}
}
this.unremoved = new ArrayList(unremoved);
return this;
}
public GcOutputBuilder unremoved(Iterable extends String> unremoved) {
if (unremoved == null) {
throw new NullPointerException("unremoved");
}
if (unremoved instanceof Collection) {
return unremoved((Collection extends String>) unremoved);
}
return unremoved(unremoved.iterator());
}
public GcOutputBuilder unremoved(Iterator extends String> unremoved) {
if (unremoved == null) {
throw new NullPointerException("unremoved");
}
this.unremoved = new ArrayList();
while (unremoved.hasNext()) {
String item = unremoved.next();
if (item == null) {
throw new NullPointerException("unremoved: null item");
}
this.unremoved.add(item);
}
return this;
}
@SafeVarargs
public final GcOutputBuilder unremoved(String... unremoved) {
if (unremoved == null) {
throw new NullPointerException("unremoved");
}
return unremoved(Arrays.asList(unremoved));
}
public GcOutput build() {
List _marked = (marked != null) ? Collections.unmodifiableList(new ArrayList(marked)) : Collections.emptyList();
List _removed = (removed != null) ? Collections.unmodifiableList(new ArrayList(removed)) : Collections.emptyList();
List _unremoved = (unremoved != null) ? Collections.unmodifiableList(new ArrayList(unremoved)) : Collections.emptyList();
return new Value(_marked, _removed, _unremoved);
}
public static GcOutputBuilder from(GcOutput v) {
return new GcOutputBuilder(v);
}
public static GcOutputBuilder from(GcOutputBuilder v) {
return new GcOutputBuilder(v);
}
private static final class Value implements GcOutput {
private final List marked;
private final List removed;
private final List unremoved;
private Value(@AutoMatter.Field("marked") List marked, @AutoMatter.Field("removed") List removed, @AutoMatter.Field("unremoved") List unremoved) {
this.marked = (marked != null) ? marked : Collections.emptyList();
this.removed = (removed != null) ? removed : Collections.emptyList();
this.unremoved = (unremoved != null) ? unremoved : Collections.emptyList();
}
@AutoMatter.Field
@Override
public List marked() {
return marked;
}
@AutoMatter.Field
@Override
public List removed() {
return removed;
}
@AutoMatter.Field
@Override
public List unremoved() {
return unremoved;
}
public GcOutputBuilder builder() {
return new GcOutputBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof GcOutput)) {
return false;
}
final GcOutput that = (GcOutput) o;
if (marked != null ? !marked.equals(that.marked()) : that.marked() != null) {
return false;
}
if (removed != null ? !removed.equals(that.removed()) : that.removed() != null) {
return false;
}
if (unremoved != null ? !unremoved.equals(that.unremoved()) : that.unremoved() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
long temp;
result = 31 * result + (marked != null ? marked.hashCode() : 0);
result = 31 * result + (removed != null ? removed.hashCode() : 0);
result = 31 * result + (unremoved != null ? unremoved.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "GcOutput{" +
"marked=" + marked +
", removed=" + removed +
", unremoved=" + unremoved +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy