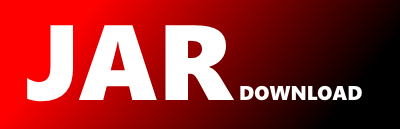
io.honnix.rkt.launcher.RktLauncher Maven / Gradle / Ivy
/*-
* -\-\-
* rkt-launcher
* --
*
* --
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* -/-/-
*/
package io.honnix.rkt.launcher;
import com.google.common.collect.ImmutableList;
import io.honnix.rkt.launcher.command.Command;
import io.honnix.rkt.launcher.command.Daemonizable;
import io.honnix.rkt.launcher.exception.RktException;
import io.honnix.rkt.launcher.exception.RktLauncherException;
import io.honnix.rkt.launcher.options.Options;
import io.honnix.rkt.launcher.output.Output;
import java.io.IOException;
import java.util.List;
import java.util.Objects;
import java.util.function.Supplier;
import org.zeroturnaround.exec.ProcessExecutor;
/**
* The rkt launcher forking rkt
process.
*/
public class RktLauncher {
private static final String DEFAULT_RKT = "rkt";
private static final List DEFAULT_DAEMON =
ImmutableList.of("systemd-run", "--slice=machine");
private static final Supplier DEFAULT_PROCESS_EXECUTOR_SUPPLIER =
ProcessExecutor::new;
private final RktLauncherConfig rktLauncherConfig;
private final Supplier processExecutorSupplier;
public RktLauncher(final RktLauncherConfig rktLauncherConfig) {
this(rktLauncherConfig, DEFAULT_PROCESS_EXECUTOR_SUPPLIER);
}
/**
* Used by unit test.
*/
RktLauncher(final RktLauncherConfig rktLauncherConfig,
final Supplier processExecutorSupplier) {
this.rktLauncherConfig = Objects.requireNonNull(rktLauncherConfig);
this.processExecutorSupplier = Objects.requireNonNull(processExecutorSupplier);
}
/**
* Run rkt
command.
*
* @param command the rkt
command
* @param type of the command options
* @param type of the command output
* @return the command output
* @throws RktLauncherException if failed to fork rkt
process for any reason
* @throws RktException if rkt
returns exit code other than 0
*/
public S run(final Command command)
throws RktLauncherException, RktException {
final ProcessExecutor processExecutor = processExecutorSupplier.get();
processExecutor.readOutput(true);
try {
return command.parse(
processExecutor.command(buildCompleteCommand(command)).executeNoTimeout());
} catch (IOException | InterruptedException e) {
throw new RktLauncherException("failed executing command " + command.getClass(), e);
}
}
private List buildCompleteCommand(
final Command command) {
final ImmutableList.Builder builder = ImmutableList.builder();
if (command instanceof Daemonizable && ((Daemonizable) command).daemonize()) {
builder.addAll(rktLauncherConfig.daemon().orElse(DEFAULT_DAEMON));
}
return builder
.add(rktLauncherConfig.rkt().orElse(DEFAULT_RKT))
.addAll(rktLauncherConfig.globalOptions().map(Options::asList).orElse(ImmutableList.of()))
.addAll(command.asList())
.build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy