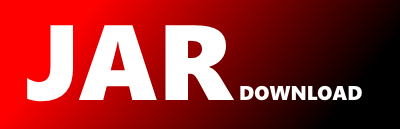
io.honnix.rkt.launcher.command.RunPreparedBuilder Maven / Gradle / Ivy
package io.honnix.rkt.launcher.command;
import io.honnix.rkt.launcher.options.RunPreparedOptions;
import io.norberg.automatter.AutoMatter;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.util.Optional;
import javax.annotation.Generated;
@Generated("io.norberg.automatter.processor.AutoMatterProcessor")
public final class RunPreparedBuilder {
private boolean daemonize;
private Optional options;
private List args;
public RunPreparedBuilder() {
this.options = Optional.empty();
}
private RunPreparedBuilder(RunPrepared v) {
this.daemonize = v.daemonize();
this.options = v.options();
List extends String> _args = v.args();
this.args = (_args == null) ? null : new ArrayList(_args);
}
private RunPreparedBuilder(RunPreparedBuilder v) {
this.daemonize = v.daemonize;
this.options = v.options;
this.args = (v.args == null) ? null : new ArrayList(v.args);
}
public boolean daemonize() {
return daemonize;
}
public RunPreparedBuilder daemonize(boolean daemonize) {
this.daemonize = daemonize;
return this;
}
public Optional options() {
return options;
}
public RunPreparedBuilder options(RunPreparedOptions options) {
return options(Optional.ofNullable(options));
}
@SuppressWarnings("unchecked")
public RunPreparedBuilder options(Optional extends RunPreparedOptions> options) {
if (options == null) {
throw new NullPointerException("options");
}
this.options = (Optional)options;
return this;
}
public List args() {
if (this.args == null) {
this.args = new ArrayList();
}
return args;
}
public RunPreparedBuilder args(List extends String> args) {
return args((Collection extends String>) args);
}
public RunPreparedBuilder args(Collection extends String> args) {
if (args == null) {
throw new NullPointerException("args");
}
for (String item : args) {
if (item == null) {
throw new NullPointerException("args: null item");
}
}
this.args = new ArrayList(args);
return this;
}
public RunPreparedBuilder args(Iterable extends String> args) {
if (args == null) {
throw new NullPointerException("args");
}
if (args instanceof Collection) {
return args((Collection extends String>) args);
}
return args(args.iterator());
}
public RunPreparedBuilder args(Iterator extends String> args) {
if (args == null) {
throw new NullPointerException("args");
}
this.args = new ArrayList();
while (args.hasNext()) {
String item = args.next();
if (item == null) {
throw new NullPointerException("args: null item");
}
this.args.add(item);
}
return this;
}
@SafeVarargs
public final RunPreparedBuilder args(String... args) {
if (args == null) {
throw new NullPointerException("args");
}
return args(Arrays.asList(args));
}
public RunPreparedBuilder addArg(String arg) {
if (arg == null) {
throw new NullPointerException("arg");
}
if (this.args == null) {
this.args = new ArrayList();
}
args.add(arg);
return this;
}
public RunPrepared build() {
List _args = (args != null) ? Collections.unmodifiableList(new ArrayList(args)) : Collections.emptyList();
return new Value(daemonize, options, _args);
}
public static RunPreparedBuilder from(RunPrepared v) {
return new RunPreparedBuilder(v);
}
public static RunPreparedBuilder from(RunPreparedBuilder v) {
return new RunPreparedBuilder(v);
}
private static final class Value implements RunPrepared {
private final boolean daemonize;
private final Optional options;
private final List args;
private Value(@AutoMatter.Field("daemonize") boolean daemonize, @AutoMatter.Field("options") Optional options, @AutoMatter.Field("args") List args) {
if (options == null) {
throw new NullPointerException("options");
}
this.daemonize = daemonize;
this.options = options;
this.args = (args != null) ? args : Collections.emptyList();
}
@AutoMatter.Field
@Override
public boolean daemonize() {
return daemonize;
}
@AutoMatter.Field
@Override
public Optional options() {
return options;
}
@AutoMatter.Field
@Override
public List args() {
return args;
}
public RunPreparedBuilder builder() {
return new RunPreparedBuilder(this);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof RunPrepared)) {
return false;
}
final RunPrepared that = (RunPrepared) o;
if (daemonize != that.daemonize()) {
return false;
}
if (options != null ? !options.equals(that.options()) : that.options() != null) {
return false;
}
if (args != null ? !args.equals(that.args()) : that.args() != null) {
return false;
}
return true;
}
@Override
public int hashCode() {
int result = 1;
long temp;
result = 31 * result + (daemonize ? 1231 : 1237);
result = 31 * result + (options != null ? options.hashCode() : 0);
result = 31 * result + (args != null ? args.hashCode() : 0);
return result;
}
@Override
public String toString() {
return "RunPrepared{" +
"daemonize=" + daemonize +
", options=" + options +
", args=" + args +
'}';
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy