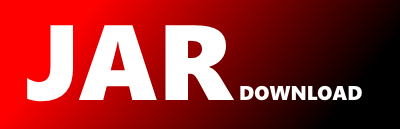
io.humble.ferry.JNIReference Maven / Gradle / Ivy
Go to download
This is the main Humble Video Java library. It contains no native code, but all Java runtime code.
It must be paired up with the correct humble-video-arch-*.jar library for your OS. For most
users, depending on humble-video-all will work better.
The newest version!
Please wait ...