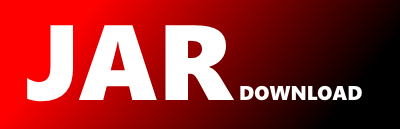
io.humble.video.Encoder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of humble-video-noarch Show documentation
Show all versions of humble-video-noarch Show documentation
This is the main Humble Video Java library. It contains no native code, but all Java runtime code.
It must be paired up with the correct humble-video-arch-*.jar library for your OS. For most
users, depending on humble-video-all will work better.
The newest version!
/* ----------------------------------------------------------------------------
* This file was automatically generated by SWIG (http://www.swig.org).
* Version 2.0.6
*
* Do not make changes to this file unless you know what you are doing--modify
* the SWIG interface file instead.
* ----------------------------------------------------------------------------- */
package io.humble.video;
import io.humble.ferry.*;
/**
* Encodes MediaAudio, MediaPicture or MediaSubtitle objects into MediaPacket objects.
*/
public class Encoder extends Coder {
// JNIHelper.swg: Start generated code
// >>>>>>>>>>>>>>>>>>>>>>>>>>>
/**
* This method is only here to use some references and remove
* a Eclipse compiler warning.
*/
@SuppressWarnings("unused")
private void noop()
{
Buffer.make(null, 1);
}
private volatile long swigCPtr;
/**
* Internal Only.
*/
protected Encoder(long cPtr, boolean cMemoryOwn) {
super(VideoJNI.Encoder_SWIGUpcast(cPtr), cMemoryOwn);
swigCPtr = cPtr;
}
/**
* Internal Only.
*/
protected Encoder(long cPtr, boolean cMemoryOwn,
java.util.concurrent.atomic.AtomicLong ref)
{
super(VideoJNI.Encoder_SWIGUpcast(cPtr),
cMemoryOwn, ref);
swigCPtr = cPtr;
}
/**
* Internal Only. Not part of public API.
*
* Get the raw value of the native object that obj is proxying for.
*
* @param obj The java proxy object for a native object.
* @return The raw pointer obj is proxying for.
*/
protected static long getCPtr(Encoder obj) {
if (obj == null) return 0;
return obj.getMyCPtr();
}
/**
* Internal Only. Not part of public API.
*
* Get the raw value of the native object that we're proxying for.
*
* @return The raw pointer we're proxying for.
*/
protected long getMyCPtr() {
if (swigCPtr == 0) throw new IllegalStateException("underlying native object already deleted");
return swigCPtr;
}
/**
* Create a new Encoder object that is actually referring to the
* exact same underlying native object.
*
* @return the new Java object.
*/
@Override
public Encoder copyReference() {
if (swigCPtr == 0)
return null;
else
return new Encoder(swigCPtr, swigCMemOwn, getJavaRefCount());
}
/**
* Compares two values, returning true if the underlying objects in native code are the same object.
*
* That means you can have two different Java objects, but when you do a comparison, you'll find out
* they are the EXACT same object.
*
* @return True if the underlying native object is the same. False otherwise.
*/
public boolean equals(Object obj) {
boolean equal = false;
if (obj instanceof Encoder)
equal = (((Encoder)obj).swigCPtr == this.swigCPtr);
return equal;
}
/**
* Get a hashable value for this object.
*
* @return the hashable value.
*/
public int hashCode() {
return (int)swigCPtr;
}
// <<<<<<<<<<<<<<<<<<<<<<<<<<<
// JNIHelper.swg: End generated code
/**
* the number of media object the encoder had to drop (i.e. skip
* encoding) in order to ensure that time stamp values are
* monotonically increasing. See https://code.google.com/p/xuggle/issues/detail?id=180
* for details on why this is.
*/
public long getNumDroppedFrames() {
return VideoJNI.Encoder_getNumDroppedFrames(swigCPtr, this);
}
/**
* Create a Encoder that will use the given Codec.
*
* @return a Encoder
* @throws InvalidArgument if codec is null or codec cannot decode.
*/
public static Encoder make(Codec codec) {
long cPtr = VideoJNI.Encoder_make__SWIG_0(Codec.getCPtr(codec), codec);
return (cPtr == 0) ? null : new Encoder(cPtr, false);
}
/**
* Creates a Encoder from a given Encoder
* @return a Encoder
* @throws InvalidArgument if src is null
*/
public static Encoder make(Coder src) {
long cPtr = VideoJNI.Encoder_make__SWIG_1(Coder.getCPtr(src), src);
return (cPtr == 0) ? null : new Encoder(cPtr, false);
}
/**
* Open this Coder, using the given bag of Codec-specific options.
*
* @param inputOptions If non-null, a bag of codec-specific options.
* @param unsetOptions If non-null, the bag will be emptied and then filled with
* the options in inputOptions
that were not set.
*/
public void open(KeyValueBag inputOptions, KeyValueBag unsetOptions) {
VideoJNI.Encoder_open(swigCPtr, this, KeyValueBag.getCPtr(inputOptions), inputOptions, KeyValueBag.getCPtr(unsetOptions), unsetOptions);
}
/**
* Encode the given MediaPicture using this encoder.
*
* The MediaPicture will allocate a buffer to use internally for this, and
* will free it when the frame destroys itself.
*
* Also, when done in order to flush the encoder, caller should call
* this method passing in 0 (null) for frame to tell the encoder
* to flush any data it was keeping a hold of.
*
* @param output [out] The packet to encode into. Caller should check
* MediaPacket.isComplete() after call to find out if we had enough
* information to encode a full packet.
* @param picture [in] The picture to encode
*
* Note: caller must ensure that output has sufficient space to
* contain a full packet. Alas, there is currently no way to
* query an encoder to find out the maximum packet size that
* can be output (bummer, I know). That leaves the caller two
* options. (1) You can call Packet.make() before each encode
* call, and then the encoder will automagically create the correct
* sized buffer for that call (but if you reuse the packet, it
* may be too small for the next caller). Or (2) you can call
* Packet.make(int) with a value that will be larger than your
* max packet size (in which case you can reuse the packet).
*/
public void encodeVideo(MediaPacket output, MediaPicture picture) {
VideoJNI.Encoder_encodeVideo(swigCPtr, this, MediaPacket.getCPtr(output), output, MediaPicture.getCPtr(picture), picture);
}
/**
* Encode the given MediaAudio using this encoder.
*
* Callers should call this repeatedly on a set of samples until
* we consume all the samples.
*
* Also, when done in order to flush the encoder, caller should call
* this method passing in 0 (null) for samples to tell the encoder
* to flush any data it was keeping a hold of.
*
* @param output [out] The packet to encode into. Caller should check
* MediaPacket.isComplete() after call to find out if we had enough
* information to encode a full packet.
* @param samples [in] The samples to consume
*
* Note: caller must ensure that output has sufficient space to
* contain a full packet. Alas, there is currently no way to
* query an encoder to find out the maximum packet size that
* can be output (bummer, I know). That leaves the caller two
* options. (1) You can call Packet.make() before each encode
* call, and then the encoder will automagically create the correct
* sized buffer for that call (but if you reuse the packet, it
* may be too small for the next caller). Or (2) you can call
* Packet.make(int) with a value that will be larger than your
* max packet size (in which case you can reuse the packet).
*/
public void encodeAudio(MediaPacket output, MediaAudio samples) {
VideoJNI.Encoder_encodeAudio(swigCPtr, this, MediaPacket.getCPtr(output), output, MediaAudio.getCPtr(samples), samples);
}
/**
* Encode the given Media using this encoder.
*
* Callers should call this repeatedly on a media object ntil
* we consume all the media.
*
* Also, when done in order to flush the encoder, caller should call
* this method passing in 0 (null) for media to tell the encoder
* to flush any data it was keeping a hold of.
*
* @param output [out] The packet to encode into. Caller should check
* MediaPacket.isComplete() after call to find out if we had enough
* information to encode a full packet.
*
*
* Note: caller must ensure that output has sufficient space to
* contain a full packet. Alas, there is currently no way to
* query an encoder to find out the maximum packet size that
* can be output (bummer, I know). That leaves the caller two
* options. (1) You can call Packet.make() before each encode
* call, and then the encoder will automagically create the correct
* sized buffer for that call (but if you reuse the packet, it
* may be too small for the next caller). Or (2) you can call
* Packet.make(int) with a value that will be larger than your
* max packet size (in which case you can reuse the packet).
*
* @throws throws an exception if getCodecType() and the underlying
* media object do not align (e.g. if you pass in a MediaPicture but the
* CodecType is audio data).
*/
public void encode(MediaPacket output, MediaSampled media) {
VideoJNI.Encoder_encode(swigCPtr, this, MediaPacket.getCPtr(output), output, MediaSampled.getCPtr(media), media);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy