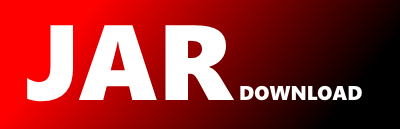
io.humble.video.Global Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of humble-video-noarch Show documentation
Show all versions of humble-video-noarch Show documentation
This is the main Humble Video Java library. It contains no native code, but all Java runtime code.
It must be paired up with the correct humble-video-arch-*.jar library for your OS. For most
users, depending on humble-video-all will work better.
The newest version!
/* ----------------------------------------------------------------------------
* This file was automatically generated by SWIG (http://www.swig.org).
* Version 2.0.6
*
* Do not make changes to this file unless you know what you are doing--modify
* the SWIG interface file instead.
* ----------------------------------------------------------------------------- */
package io.humble.video;
import io.humble.ferry.*;
/**
* A collection of static functions that refer to the entire package (like version getters).
*/
public class Global extends RefCounted {
// JNIHelper.swg: Start generated code
// >>>>>>>>>>>>>>>>>>>>>>>>>>>
/**
* This method is only here to use some references and remove
* a Eclipse compiler warning.
*/
@SuppressWarnings("unused")
private void noop()
{
Buffer.make(null, 1);
}
private volatile long swigCPtr;
/**
* Internal Only.
*/
protected Global(long cPtr, boolean cMemoryOwn) {
super(VideoJNI.Global_SWIGUpcast(cPtr), cMemoryOwn);
swigCPtr = cPtr;
}
/**
* Internal Only.
*/
protected Global(long cPtr, boolean cMemoryOwn,
java.util.concurrent.atomic.AtomicLong ref)
{
super(VideoJNI.Global_SWIGUpcast(cPtr),
cMemoryOwn, ref);
swigCPtr = cPtr;
}
/**
* Internal Only. Not part of public API.
*
* Get the raw value of the native object that obj is proxying for.
*
* @param obj The java proxy object for a native object.
* @return The raw pointer obj is proxying for.
*/
protected static long getCPtr(Global obj) {
if (obj == null) return 0;
return obj.getMyCPtr();
}
/**
* Internal Only. Not part of public API.
*
* Get the raw value of the native object that we're proxying for.
*
* @return The raw pointer we're proxying for.
*/
protected long getMyCPtr() {
if (swigCPtr == 0) throw new IllegalStateException("underlying native object already deleted");
return swigCPtr;
}
/**
* Create a new Global object that is actually referring to the
* exact same underlying native object.
*
* @return the new Java object.
*/
@Override
public Global copyReference() {
if (swigCPtr == 0)
return null;
else
return new Global(swigCPtr, swigCMemOwn, getJavaRefCount());
}
/**
* Compares two values, returning true if the underlying objects in native code are the same object.
*
* That means you can have two different Java objects, but when you do a comparison, you'll find out
* they are the EXACT same object.
*
* @return True if the underlying native object is the same. False otherwise.
*/
public boolean equals(Object obj) {
boolean equal = false;
if (obj instanceof Global)
equal = (((Global)obj).swigCPtr == this.swigCPtr);
return equal;
}
/**
* Get a hashable value for this object.
*
* @return the hashable value.
*/
public int hashCode() {
return (int)swigCPtr;
}
// <<<<<<<<<<<<<<<<<<<<<<<<<<<
// JNIHelper.swg: End generated code
/**
* The default unit of time that {@link MediaRaw} time stamps are represented in.
*/
public static final java.util.concurrent.TimeUnit DEFAULT_TIME_UNIT =
java.util.concurrent.TimeUnit.MICROSECONDS;
/**
* The default timebase used by media if not otherwise specified.
*/
public static Rational getDefaultTimeBase() {
long cPtr = VideoJNI.Global_getDefaultTimeBase();
return (cPtr == 0) ? null : new Rational(cPtr, false);
}
/**
* Get the major version number of this library.
* @return the major version number of this library or 0 if unknown.
*/
public static int getVersionMajor() {
return VideoJNI.Global_getVersionMajor();
}
/**
* Get the minor version number of this library.
* @return the minor version number of this library or 0 if unknown.
*/
public static int getVersionMinor() {
return VideoJNI.Global_getVersionMinor();
}
/**
* Get a string representation of the version of this library.
* @return the version of this library in string form.
*/
public static String getVersionStr() {
return VideoJNI.Global_getVersionStr();
}
/**
* Get the version of the FFMPEG libavformat library we are compiled against.
* @return the version.
*/
public static int getAVFormatVersion() {
return VideoJNI.Global_getAVFormatVersion();
}
/**
* Get the version of the FFMPEG libavformat library we are compiled against.
* @return the version.
*/
public static String getAVFormatVersionStr() {
return VideoJNI.Global_getAVFormatVersionStr();
}
/**
* Get the version of the FFMPEG libavcodec library we are compiled against.
* @return the version.
*/
public static int getAVCodecVersion() {
return VideoJNI.Global_getAVCodecVersion();
}
/**
* Get the version of the FFMPEG libavcodec library we are compiled against.
* @return the version.
*/
public static String getAVCodecVersionStr() {
return VideoJNI.Global_getAVCodecVersionStr();
}
/**
* Internal Only. Do not call.
* Methods using the C++ interface that will not necessarily
* create other Global object should call this. In general,
* unless you're extending Humble Video directly yourself, ordinary
* users of this library don't need to call this.
*
* It's main purpose is to ensure any FFmpeg required environment
* initialization functions are called, and any Humble Video required
* environmental contexts are set up.
*
*/
public static void init() {
VideoJNI.Global_init();
}
/**
* Internal Only. This method can be used to turn up or
* down FFmpeg's logging level.
* @param level An integer value for level. Lower numbers
* mean less logging. A negative number tells FFmpeg to
* shut up.
*/
public static void setFFmpegLoggingLevel(int level) {
VideoJNI.Global_setFFmpegLoggingLevel(level);
}
/**
* A value that means no time stamp is set for a given object.
* if the Media#getTimeStamp() method of an
* object returns this value it means the time stamp wasn't set.
*/
public final static long NO_PTS = VideoJNI.Global_NO_PTS_get();
/**
* The default time units per second that we use for decoded
* MediaRaw objects.
*
* This means that 1 tick of a time stamp is 1 Microsecond.
*/
public final static long DEFAULT_PTS_PER_SECOND = VideoJNI.Global_DEFAULT_PTS_PER_SECOND_get();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy