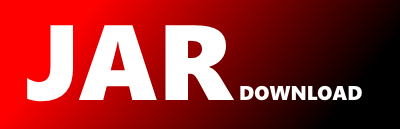
io.humble.video.customio.FfmpegIO Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of humble-video-noarch Show documentation
Show all versions of humble-video-noarch Show documentation
This is the main Humble Video Java library. It contains no native code, but all Java runtime code.
It must be paired up with the correct humble-video-arch-*.jar library for your OS. For most
users, depending on humble-video-all will work better.
The newest version!
/*******************************************************************************
* Copyright (c) 2013, Art Clarke. All rights reserved.
*
* This file is part of Humble-Video.
*
* Humble-Video is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* Humble-Video is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Humble-Video. If not, see .
*******************************************************************************/
package io.humble.video.customio;
import io.humble.video.customio.FfmpegIOHandle;
import io.humble.video.customio.IURLProtocolHandlerFactory;
import io.humble.video.customio.URLProtocolManager;
/**
* For Internal Use Only.
* This is the global (static) object that implements the Humble Video IO library.
*
* The following methods are not meant to be generally
* called by code (although they can be).
*
* You should never need to call this method. Calling {@link URLProtocolManager#registerFactory(String, IURLProtocolHandlerFactory)}
* should cause it to be instantiated.
*
* They are generally 'thread-aware' but not 'thread-safe', meaning if you use a handle
* on a thread, you are a responsible for making sure you don't
* reuse it on other threads.
*
* They forward into ffmpeg's URL read/write/seek capabilities
* and allow our test scripts to make sure our URLProtocolHandlers
* (and FFMPEG's native handlers) work as expected.
*
* Lastly this class, unlike other classes in the library, does not use SWIG to generate
* the Java objects, so you need to be careful not to change method names as the corresponding
* native code relies on specific naming and parameters.
*
* @author aclarke
*
*/
public class FfmpegIO
{
static
{
// this will load the one shared library
io.humble.ferry.Ferry.init();
FfmpegIO.init();
// And force the URLProtocolManager global
// object to be created.
URLProtocolManager.init();
}
/** Force a load of all native libraries; not normally needed */
public static void load() {}
/**
* Internal Only. Do not use.
*/
public native static void init();
/**
* This method is called by URLProtocolManager to register itself as a
* protocol manager for different FFMPEG protocols.
*
* @param protocol The protocol
* @param manager The manager for that protocol.
*/
static synchronized void registerProtocolHandler(String protocol,
URLProtocolManager manager)
{
native_registerProtocolHandler(protocol, manager);
}
public static int url_open(FfmpegIOHandle handle, String filename, int flags)
{
return native_url_open(handle, filename, flags);
}
public static int url_read(FfmpegIOHandle handle, byte[] buffer, int length)
{
return native_url_read(handle, buffer, length);
}
public static int url_close(FfmpegIOHandle handle)
{
return native_url_close(handle);
}
public static int url_write(FfmpegIOHandle handle, byte[] buffer, int length)
{
return native_url_write(handle, buffer, length);
}
public static long url_seek(FfmpegIOHandle handle, long position, int whence)
{
return native_url_seek(handle, position, whence);
}
private static native int native_registerProtocolHandler(
String urlPrefix, URLProtocolManager proto);
private static native int native_url_open(FfmpegIOHandle handle,
String filename, int flags);
private static native int native_url_read(FfmpegIOHandle handle,
byte[] buffer, int length);
private static native int native_url_write(FfmpegIOHandle handle,
byte[] buffer, int length);
private static native long native_url_seek(FfmpegIOHandle handle,
long position, int whence);
private static native int native_url_close(FfmpegIOHandle handle);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy