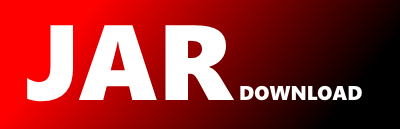
io.hyperfoil.controller.router.ApiRouter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hyperfoil-controller-api Show documentation
Show all versions of hyperfoil-controller-api Show documentation
Hyperfoil Controller RESTful API
package io.hyperfoil.controller.router;
import java.util.Date;
import java.util.List;
import java.util.Map;
import java.util.Collections;
import io.vertx.core.json.Json;
import io.vertx.ext.web.handler.BodyHandler;
import io.vertx.ext.web.Router;
import io.vertx.ext.web.RoutingContext;
import org.apache.logging.log4j.Logger;
import org.apache.logging.log4j.LogManager;
import io.hyperfoil.controller.model.*;
import io.hyperfoil.controller.ApiService;
public class ApiRouter {
private final ApiService service;
private static final Logger log = LogManager.getLogger(ApiRouter.class);
public ApiRouter(ApiService service, Router router) {
this.service = service;
router.route().handler(BodyHandler.create(System.getProperty("java.io.tmpdir")));
router.errorHandler(500, ctx -> {
log.error("Error processing {} {}", ctx.request().method(), ctx.request().uri(), ctx.failure());
});
router.get("/openapi").produces("text/vnd.yaml").handler(this::openApi);
router.get("/benchmark").produces("application/json").handler(this::listBenchmarks);
router.post("/benchmark").consumes("application/json").handler(this::addBenchmark$application_json);
router.post("/benchmark").consumes("text/uri-list").handler(this::addBenchmark$text_uri_list);
router.post("/benchmark").consumes("text/vnd.yaml").handler(this::addBenchmark$text_vnd_yaml);
router.post("/benchmark").consumes("application/java-serialized-object").handler(this::addBenchmark$application_java_serialized_object);
router.post("/benchmark").consumes("multipart/form-data").handler(this::addBenchmark$multipart_form_data);
router.get("/benchmark/:name").produces("text/vnd.yaml").handler(this::getBenchmark$text_vnd_yaml);
router.get("/benchmark/:name").produces("application/java-serialized-object").handler(this::getBenchmark$application_java_serialized_object);
router.get("/benchmark/:name/start").produces("application/json").handler(this::startBenchmark);
router.get("/benchmark/:name/files").produces("multipart/form-data").handler(this::getBenchmarkFiles);
router.get("/benchmark/:name/structure").produces("application/json").handler(this::getBenchmarkStructure);
router.get("/run").produces("application/json").handler(this::listRuns);
router.get("/run/:runId").produces("application/json").handler(this::getRun);
router.get("/run/:runId/agentCpu").produces("application/json").handler(this::agentCpu);
router.get("/run/:runId/kill").handler(this::killRun);
router.get("/run/:runId/report").produces("text/html").handler(this::createReport);
router.get("/run/:runId/sessions").produces("text/plain").handler(this::listSessions);
router.get("/run/:runId/sessions/recent").produces("application/json").handler(this::getRecentSessions);
router.get("/run/:runId/sessions/total").produces("application/json").handler(this::getTotalSessions);
router.get("/run/:runId/connections").produces("text/plain").handler(this::listConnections);
router.get("/run/:runId/connections/recent").produces("application/json").handler(this::getRecentConnections);
router.get("/run/:runId/connections/total").produces("application/json").handler(this::getTotalConnections);
router.get("/run/:runId/stats/all").produces("application/zip").handler(this::getAllStats$application_zip);
router.get("/run/:runId/stats/all").produces("application/json").handler(this::getAllStats$application_json);
router.get("/run/:runId/stats/all/json").produces("application/json").handler(this::getAllStatsJson);
router.get("/run/:runId/stats/all/csv").produces("application/zip").handler(this::getAllStatsCsv);
router.get("/run/:runId/stats/recent").produces("application/json").handler(this::getRecentStats);
router.get("/run/:runId/stats/total").produces("application/json").handler(this::getTotalStats);
router.get("/run/:runId/stats/histogram").produces("application/json").handler(this::getHistogramStats);
router.get("/run/:runId/file").produces("application/octet-stream").handler(this::getRunFile);
router.get("/run/:runId/benchmark").produces("text/vnd.yaml").handler(this::getBenchmarkForRun$text_vnd_yaml);
router.get("/run/:runId/benchmark").produces("application/java-serialized-object").handler(this::getBenchmarkForRun$application_java_serialized_object);
router.get("/agents").produces("application/json").handler(this::listAgents);
router.get("/log").produces("text/plain").handler(this::getControllerLog);
router.get("/log/:agent").produces("text/plain").handler(this::getAgentLog);
router.get("/shutdown").handler(this::shutdown);
router.get("/template").produces("application/json").handler(this::listTemplates);
router.get("/token").handler(this::getToken);
router.get("/version").produces("application/json").handler(this::getVersion);
}
private void openApi(RoutingContext ctx) {
service.openApi(ctx);
}
private void listBenchmarks(RoutingContext ctx) {
service.listBenchmarks(ctx);
}
private void addBenchmark$application_json(RoutingContext ctx) {
String _ifMatch = headerParam(ctx, "if-match", null);
String ifMatch = convert(_ifMatch, String.class);
String _storedFilesBenchmark = queryParam(ctx, "storedFilesBenchmark", null);
String storedFilesBenchmark = convert(_storedFilesBenchmark, String.class);
service.addBenchmark$application_json(ctx, ifMatch, storedFilesBenchmark);
}
private void addBenchmark$text_uri_list(RoutingContext ctx) {
String _ifMatch = headerParam(ctx, "if-match", null);
String ifMatch = convert(_ifMatch, String.class);
String _storedFilesBenchmark = queryParam(ctx, "storedFilesBenchmark", null);
String storedFilesBenchmark = convert(_storedFilesBenchmark, String.class);
service.addBenchmark$text_uri_list(ctx, ifMatch, storedFilesBenchmark);
}
private void addBenchmark$text_vnd_yaml(RoutingContext ctx) {
String _ifMatch = headerParam(ctx, "if-match", null);
String ifMatch = convert(_ifMatch, String.class);
String _storedFilesBenchmark = queryParam(ctx, "storedFilesBenchmark", null);
String storedFilesBenchmark = convert(_storedFilesBenchmark, String.class);
service.addBenchmark$text_vnd_yaml(ctx, ifMatch, storedFilesBenchmark);
}
private void addBenchmark$application_java_serialized_object(RoutingContext ctx) {
String _ifMatch = headerParam(ctx, "if-match", null);
String ifMatch = convert(_ifMatch, String.class);
String _storedFilesBenchmark = queryParam(ctx, "storedFilesBenchmark", null);
String storedFilesBenchmark = convert(_storedFilesBenchmark, String.class);
service.addBenchmark$application_java_serialized_object(ctx, ifMatch, storedFilesBenchmark);
}
private void addBenchmark$multipart_form_data(RoutingContext ctx) {
String _ifMatch = headerParam(ctx, "if-match", null);
String ifMatch = convert(_ifMatch, String.class);
String _storedFilesBenchmark = queryParam(ctx, "storedFilesBenchmark", null);
String storedFilesBenchmark = convert(_storedFilesBenchmark, String.class);
service.addBenchmark$multipart_form_data(ctx, ifMatch, storedFilesBenchmark);
}
private void getBenchmark$text_vnd_yaml(RoutingContext ctx) {
String _name = pathParam(ctx, "name", null);
if (_name == null) {
ctx.response().setStatusCode(400).end("path parameter 'name' was not set!");
return;
}
String name = convert(_name, String.class);
service.getBenchmark$text_vnd_yaml(ctx, name);
}
private void getBenchmark$application_java_serialized_object(RoutingContext ctx) {
String _name = pathParam(ctx, "name", null);
if (_name == null) {
ctx.response().setStatusCode(400).end("path parameter 'name' was not set!");
return;
}
String name = convert(_name, String.class);
service.getBenchmark$application_java_serialized_object(ctx, name);
}
private void startBenchmark(RoutingContext ctx) {
String _name = pathParam(ctx, "name", null);
if (_name == null) {
ctx.response().setStatusCode(400).end("path parameter 'name' was not set!");
return;
}
String name = convert(_name, String.class);
String _desc = queryParam(ctx, "desc", null);
String desc = convert(_desc, String.class);
String _xTriggerJob = headerParam(ctx, "x-trigger-job", null);
String xTriggerJob = convert(_xTriggerJob, String.class);
String _runId = queryParam(ctx, "runId", null);
String runId = convert(_runId, String.class);
String _templateParam = queryParam(ctx, "templateParam", null);
List templateParam = convert(_templateParam, List.class);
service.startBenchmark(ctx, name, desc, xTriggerJob, runId, templateParam);
}
private void getBenchmarkFiles(RoutingContext ctx) {
String _name = pathParam(ctx, "name", null);
if (_name == null) {
ctx.response().setStatusCode(400).end("path parameter 'name' was not set!");
return;
}
String name = convert(_name, String.class);
service.getBenchmarkFiles(ctx, name);
}
private void getBenchmarkStructure(RoutingContext ctx) {
String _name = pathParam(ctx, "name", null);
if (_name == null) {
ctx.response().setStatusCode(400).end("path parameter 'name' was not set!");
return;
}
String name = convert(_name, String.class);
String _maxCollectionSize = queryParam(ctx, "maxCollectionSize", "20");
int maxCollectionSize = convert(_maxCollectionSize, int.class);
String _templateParam = queryParam(ctx, "templateParam", null);
List templateParam = convert(_templateParam, List.class);
service.getBenchmarkStructure(ctx, name, maxCollectionSize, templateParam);
}
private void listRuns(RoutingContext ctx) {
String _details = queryParam(ctx, "details", "false");
boolean details = convert(_details, boolean.class);
service.listRuns(ctx, details);
}
private void getRun(RoutingContext ctx) {
String _runId = pathParam(ctx, "runId", null);
if (_runId == null) {
ctx.response().setStatusCode(400).end("path parameter 'runId' was not set!");
return;
}
String runId = convert(_runId, String.class);
service.getRun(ctx, runId);
}
private void agentCpu(RoutingContext ctx) {
String _runId = pathParam(ctx, "runId", null);
if (_runId == null) {
ctx.response().setStatusCode(400).end("path parameter 'runId' was not set!");
return;
}
String runId = convert(_runId, String.class);
service.agentCpu(ctx, runId);
}
private void killRun(RoutingContext ctx) {
String _runId = pathParam(ctx, "runId", null);
if (_runId == null) {
ctx.response().setStatusCode(400).end("path parameter 'runId' was not set!");
return;
}
String runId = convert(_runId, String.class);
service.killRun(ctx, runId);
}
private void createReport(RoutingContext ctx) {
String _runId = pathParam(ctx, "runId", null);
if (_runId == null) {
ctx.response().setStatusCode(400).end("path parameter 'runId' was not set!");
return;
}
String runId = convert(_runId, String.class);
String _source = queryParam(ctx, "source", null);
String source = convert(_source, String.class);
service.createReport(ctx, runId, source);
}
private void listSessions(RoutingContext ctx) {
String _runId = pathParam(ctx, "runId", null);
if (_runId == null) {
ctx.response().setStatusCode(400).end("path parameter 'runId' was not set!");
return;
}
String runId = convert(_runId, String.class);
String _inactive = queryParam(ctx, "inactive", "false");
boolean inactive = convert(_inactive, boolean.class);
service.listSessions(ctx, runId, inactive);
}
private void getRecentSessions(RoutingContext ctx) {
String _runId = pathParam(ctx, "runId", null);
if (_runId == null) {
ctx.response().setStatusCode(400).end("path parameter 'runId' was not set!");
return;
}
String runId = convert(_runId, String.class);
service.getRecentSessions(ctx, runId);
}
private void getTotalSessions(RoutingContext ctx) {
String _runId = pathParam(ctx, "runId", null);
if (_runId == null) {
ctx.response().setStatusCode(400).end("path parameter 'runId' was not set!");
return;
}
String runId = convert(_runId, String.class);
service.getTotalSessions(ctx, runId);
}
private void listConnections(RoutingContext ctx) {
String _runId = pathParam(ctx, "runId", null);
if (_runId == null) {
ctx.response().setStatusCode(400).end("path parameter 'runId' was not set!");
return;
}
String runId = convert(_runId, String.class);
service.listConnections(ctx, runId);
}
private void getRecentConnections(RoutingContext ctx) {
String _runId = pathParam(ctx, "runId", null);
if (_runId == null) {
ctx.response().setStatusCode(400).end("path parameter 'runId' was not set!");
return;
}
String runId = convert(_runId, String.class);
service.getRecentConnections(ctx, runId);
}
private void getTotalConnections(RoutingContext ctx) {
String _runId = pathParam(ctx, "runId", null);
if (_runId == null) {
ctx.response().setStatusCode(400).end("path parameter 'runId' was not set!");
return;
}
String runId = convert(_runId, String.class);
service.getTotalConnections(ctx, runId);
}
private void getAllStats$application_zip(RoutingContext ctx) {
String _runId = pathParam(ctx, "runId", null);
if (_runId == null) {
ctx.response().setStatusCode(400).end("path parameter 'runId' was not set!");
return;
}
String runId = convert(_runId, String.class);
service.getAllStats$application_zip(ctx, runId);
}
private void getAllStats$application_json(RoutingContext ctx) {
String _runId = pathParam(ctx, "runId", null);
if (_runId == null) {
ctx.response().setStatusCode(400).end("path parameter 'runId' was not set!");
return;
}
String runId = convert(_runId, String.class);
service.getAllStats$application_json(ctx, runId);
}
private void getAllStatsJson(RoutingContext ctx) {
String _runId = pathParam(ctx, "runId", null);
if (_runId == null) {
ctx.response().setStatusCode(400).end("path parameter 'runId' was not set!");
return;
}
String runId = convert(_runId, String.class);
service.getAllStatsJson(ctx, runId);
}
private void getAllStatsCsv(RoutingContext ctx) {
String _runId = pathParam(ctx, "runId", null);
if (_runId == null) {
ctx.response().setStatusCode(400).end("path parameter 'runId' was not set!");
return;
}
String runId = convert(_runId, String.class);
service.getAllStatsCsv(ctx, runId);
}
private void getRecentStats(RoutingContext ctx) {
String _runId = pathParam(ctx, "runId", null);
if (_runId == null) {
ctx.response().setStatusCode(400).end("path parameter 'runId' was not set!");
return;
}
String runId = convert(_runId, String.class);
service.getRecentStats(ctx, runId);
}
private void getTotalStats(RoutingContext ctx) {
String _runId = pathParam(ctx, "runId", null);
if (_runId == null) {
ctx.response().setStatusCode(400).end("path parameter 'runId' was not set!");
return;
}
String runId = convert(_runId, String.class);
service.getTotalStats(ctx, runId);
}
private void getHistogramStats(RoutingContext ctx) {
String _runId = pathParam(ctx, "runId", null);
if (_runId == null) {
ctx.response().setStatusCode(400).end("path parameter 'runId' was not set!");
return;
}
String runId = convert(_runId, String.class);
String _phase = queryParam(ctx, "phase", null);
if (_phase == null) {
ctx.response().setStatusCode(400).end("query parameter 'phase' was not set!");
return;
}
String phase = convert(_phase, String.class);
String _stepId = queryParam(ctx, "stepId", null);
if (_stepId == null) {
ctx.response().setStatusCode(400).end("query parameter 'stepId' was not set!");
return;
}
int stepId = convert(_stepId, int.class);
String _metric = queryParam(ctx, "metric", null);
if (_metric == null) {
ctx.response().setStatusCode(400).end("query parameter 'metric' was not set!");
return;
}
String metric = convert(_metric, String.class);
service.getHistogramStats(ctx, runId, phase, stepId, metric);
}
private void getRunFile(RoutingContext ctx) {
String _runId = pathParam(ctx, "runId", null);
if (_runId == null) {
ctx.response().setStatusCode(400).end("path parameter 'runId' was not set!");
return;
}
String runId = convert(_runId, String.class);
String _file = queryParam(ctx, "file", null);
if (_file == null) {
ctx.response().setStatusCode(400).end("query parameter 'file' was not set!");
return;
}
String file = convert(_file, String.class);
service.getRunFile(ctx, runId, file);
}
private void getBenchmarkForRun$text_vnd_yaml(RoutingContext ctx) {
String _runId = pathParam(ctx, "runId", null);
if (_runId == null) {
ctx.response().setStatusCode(400).end("path parameter 'runId' was not set!");
return;
}
String runId = convert(_runId, String.class);
service.getBenchmarkForRun$text_vnd_yaml(ctx, runId);
}
private void getBenchmarkForRun$application_java_serialized_object(RoutingContext ctx) {
String _runId = pathParam(ctx, "runId", null);
if (_runId == null) {
ctx.response().setStatusCode(400).end("path parameter 'runId' was not set!");
return;
}
String runId = convert(_runId, String.class);
service.getBenchmarkForRun$application_java_serialized_object(ctx, runId);
}
private void listAgents(RoutingContext ctx) {
service.listAgents(ctx);
}
private void getControllerLog(RoutingContext ctx) {
String _offset = queryParam(ctx, "offset", "0");
long offset = convert(_offset, long.class);
String _ifMatch = headerParam(ctx, "if-match", null);
String ifMatch = convert(_ifMatch, String.class);
service.getControllerLog(ctx, offset, ifMatch);
}
private void getAgentLog(RoutingContext ctx) {
String _agent = pathParam(ctx, "agent", null);
if (_agent == null) {
ctx.response().setStatusCode(400).end("path parameter 'agent' was not set!");
return;
}
String agent = convert(_agent, String.class);
String _offset = queryParam(ctx, "offset", "0");
long offset = convert(_offset, long.class);
String _ifMatch = headerParam(ctx, "if-match", null);
String ifMatch = convert(_ifMatch, String.class);
service.getAgentLog(ctx, agent, offset, ifMatch);
}
private void shutdown(RoutingContext ctx) {
String _force = queryParam(ctx, "force", "false");
boolean force = convert(_force, boolean.class);
service.shutdown(ctx, force);
}
private void listTemplates(RoutingContext ctx) {
service.listTemplates(ctx);
}
private void getToken(RoutingContext ctx) {
service.getToken(ctx);
}
private void getVersion(RoutingContext ctx) {
service.getVersion(ctx);
}
private String pathParam(RoutingContext ctx, String name, String defaultValue) {
return ctx.pathParam(name);
}
private String queryParam(RoutingContext ctx, String name, String defaultValue) {
List list = ctx.queryParam(name);
if (list == null || list.isEmpty())
return defaultValue;
return list.iterator().next();
}
private String headerParam(RoutingContext ctx, String name, String defaultValue) {
String value = ctx.request().getHeader(name);
return value == null ? defaultValue : value;
}
@SuppressWarnings("unchecked")
T convert(String value, Class type) {
if (type == String.class)
return (T) value;
if (type == boolean.class)
return (T) Boolean.valueOf(value);
if (type == int.class)
return (T) Integer.valueOf(value);
if (type == List.class) {
if (value == null)
return (T) Collections.emptyList();
if (value instanceof String)
return (T) Collections.singletonList(value);
}
if (value == null) {
if (type == Map.class)
return (T) Collections.emptyMap();
return null;
}
return Json.decodeValue(value, type);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy