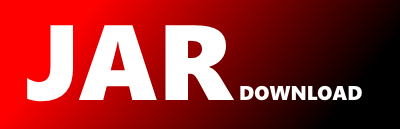
io.hypertrack.factory.HyperTrackFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hypertrack-java Show documentation
Show all versions of hypertrack-java Show documentation
A Java wrapper for the HyperTrack API https://hypertrack.io
package io.hypertrack.factory;
import com.google.gson.Gson;
import com.google.gson.internal.LinkedTreeMap;
import io.hypertrack.model.Driver;
import io.hypertrack.model.HyperTrackModel;
import io.hypertrack.model.Task;
import io.hypertrack.net.HyperTrackClient;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
/**
* Created by Arjun on 20/04/16.
*/
public abstract class HyperTrackFactory {
private HyperTrackClient client;
protected HyperTrackFactory(final HyperTrackClient client) {
this.client = client;
}
protected abstract T makeNew(Map params);
protected abstract String getModelUrl();
public T retrieve(String id) throws Exception {
return getAndCreateFromId(id);
}
public T create(Map params) throws Exception {
return postAndCreateFromParams(params);
}
public ArrayList list(Map filters) throws Exception {
return getListAndCreate(filters);
}
protected T getAndCreateFromId(String id) throws Exception {
String response = client.request(getModelUrl() + id + "/", "GET", null);
return createFromParams(getMapFromResponse(response));
}
protected T postAndCreateFromParams(Map params) throws Exception {
String response = client.request(getModelUrl(), "POST", params);
return createFromParams(getMapFromResponse(response));
}
protected ArrayList getListAndCreate(Map filters) throws Exception {
// TODO handle pagination
String response = client.request(getModelUrl(), "GET", filters);
Gson gson = new Gson();
Map responseMap = getMapFromResponse(response);
ArrayList results = (ArrayList)responseMap.get("results");
ArrayList objectList = new ArrayList<>();
for (LinkedTreeMap temp : results) {
Map params = new HashMap<>(temp);
objectList.add(createFromParams(params));
}
return objectList;
}
private T createFromParams(Map params) {
return makeNew(params);
}
private static Map getMapFromResponse(String response) {
Gson gson = new Gson();
Map map = new HashMap();
map = (Map)gson.fromJson(response, map.getClass());
return map;
}
public HyperTrackClient getClient() {
return client;
}
public void setClient(HyperTrackClient client) {
this.client = client;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy