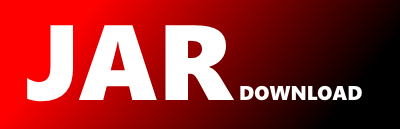
io.hypertrack.factory.ActionFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hypertrack-java Show documentation
Show all versions of hypertrack-java Show documentation
A Java wrapper for the HyperTrack API https://hypertrack.io
The newest version!
package io.hypertrack.factory;
import io.hypertrack.model.Action;
import io.hypertrack.net.HyperTrackClient;
import io.hypertrack.net.HyperTrackException;
import java.util.Map;
/**
* Factory class for Action model.
*/
public class ActionFactory extends HyperTrackFactory {
private static String modelUrl = "actions/";
public ActionFactory(HyperTrackClient client) {
super(client);
}
@Override
protected Action makeNew(Map params) {
return new Action(params);
}
@Override
protected String getModelUrl() {
return modelUrl;
}
/**
* Returns tracking url of the action.
*
* @param action action object
* @return tracking url of the action
*/
public String getTrackingUrl(Action action) {
return (String) action.getProperty("tracking_url");
}
/**
* Returns tracking url of the action.
*
* @param actionId id of the action
* @return tracking url of the action
*/
public String getTrackingUrl(String actionId) {
Action action = retrieve(actionId);
return getTrackingUrl(action);
}
/**
* Mark action as completed.
*
* @param action action object
* @param params params to be passed as POST body
*/
public void complete(Action action, Map params) {
String actionId = action.getId();
try {
String response = getClient().request(getInstanceUrl(actionId) + "complete/",
"POST", params);
Map responseMap = getMapFromResponse(response);
updateFromParams(action, responseMap);
} catch (final HyperTrackException e) {
e.printStackTrace();
throw new HyperTrackException("Could not complete action", e);
}
}
/**
* Mark action as canceled.
*
* @param action action object
* @param params params to be passed as POST body
*/
public void cancel(Action action, Map params) {
String actionId = action.getId();
try {
String response = getClient().request(getInstanceUrl(actionId) + "cancel/",
"POST", params);
Map responseMap = getMapFromResponse(response);
updateFromParams(action, responseMap);
} catch (final HyperTrackException e) {
e.printStackTrace();
throw new HyperTrackException("Could not cancel action", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy